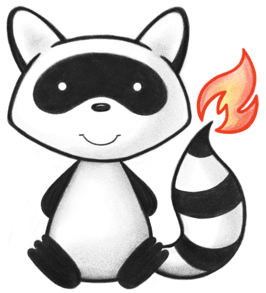
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Money40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.ContactDetail40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.UsageContext40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Date40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 014import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Decimal40_50; 015import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 016import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 017import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 018import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r4.model.ChargeItemDefinition; 021import org.hl7.fhir.r5.model.Enumeration; 022import org.hl7.fhir.r5.model.MonetaryComponent; 023 024/* 025 Copyright (c) 2011+, HL7, Inc. 026 All rights reserved. 027 028 Redistribution and use in source and binary forms, with or without modification, 029 are permitted provided that the following conditions are met: 030 031 * Redistributions of source code must retain the above copyright notice, this 032 list of conditions and the following disclaimer. 033 * Redistributions in binary form must reproduce the above copyright notice, 034 this list of conditions and the following disclaimer in the documentation 035 and/or other materials provided with the distribution. 036 * Neither the name of HL7 nor the names of its contributors may be used to 037 endorse or promote products derived from this software without specific 038 prior written permission. 039 040 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 041 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 042 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 043 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 044 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 045 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 046 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 047 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 048 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 049 POSSIBILITY OF SUCH DAMAGE. 050 051*/ 052// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 053public class ChargeItemDefinition40_50 { 054 055 public static org.hl7.fhir.r5.model.ChargeItemDefinition convertChargeItemDefinition(org.hl7.fhir.r4.model.ChargeItemDefinition src) throws FHIRException { 056 if (src == null) 057 return null; 058 org.hl7.fhir.r5.model.ChargeItemDefinition tgt = new org.hl7.fhir.r5.model.ChargeItemDefinition(); 059 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 060 if (src.hasUrl()) 061 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 062 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 063 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 064 if (src.hasVersion()) 065 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 066 if (src.hasTitle()) 067 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 068 for (org.hl7.fhir.r4.model.UriType t : src.getDerivedFromUri()) tgt.getDerivedFromUri().add(Uri40_50.convertUri(t)); 069 for (org.hl7.fhir.r4.model.CanonicalType t : src.getPartOf()) 070 tgt.getPartOf().add(Canonical40_50.convertCanonical(t)); 071 for (org.hl7.fhir.r4.model.CanonicalType t : src.getReplaces()) 072 tgt.getReplaces().add(Canonical40_50.convertCanonical(t)); 073 if (src.hasStatus()) 074 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 075 if (src.hasExperimental()) 076 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 077 if (src.hasDate()) 078 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 079 if (src.hasPublisher()) 080 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 081 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 082 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 083 if (src.hasDescription()) 084 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 085 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 086 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 087 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 088 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 089 if (src.hasCopyright()) 090 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 091 if (src.hasApprovalDate()) 092 tgt.setApprovalDateElement(Date40_50.convertDate(src.getApprovalDateElement())); 093 if (src.hasLastReviewDate()) 094 tgt.setLastReviewDateElement(Date40_50.convertDate(src.getLastReviewDateElement())); 095 if (src.hasEffectivePeriod()) 096 tgt.setEffectivePeriod(Period40_50.convertPeriod(src.getEffectivePeriod())); 097 if (src.hasCode()) 098 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 099 for (org.hl7.fhir.r4.model.Reference t : src.getInstance()) tgt.addInstance(Reference40_50.convertReference(t)); 100 for (org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent t : src.getApplicability()) 101 tgt.addApplicability(convertChargeItemDefinitionApplicabilityComponent(t)); 102 for (org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent t : src.getPropertyGroup()) 103 tgt.addPropertyGroup(convertChargeItemDefinitionPropertyGroupComponent(t)); 104 return tgt; 105 } 106 107 public static org.hl7.fhir.r4.model.ChargeItemDefinition convertChargeItemDefinition(org.hl7.fhir.r5.model.ChargeItemDefinition src) throws FHIRException { 108 if (src == null) 109 return null; 110 org.hl7.fhir.r4.model.ChargeItemDefinition tgt = new org.hl7.fhir.r4.model.ChargeItemDefinition(); 111 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 112 if (src.hasUrl()) 113 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 114 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 115 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 116 if (src.hasVersion()) 117 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 118 if (src.hasTitle()) 119 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 120 for (org.hl7.fhir.r5.model.UriType t : src.getDerivedFromUri()) tgt.getDerivedFromUri().add(Uri40_50.convertUri(t)); 121 for (org.hl7.fhir.r5.model.CanonicalType t : src.getPartOf()) 122 tgt.getPartOf().add(Canonical40_50.convertCanonical(t)); 123 for (org.hl7.fhir.r5.model.CanonicalType t : src.getReplaces()) 124 tgt.getReplaces().add(Canonical40_50.convertCanonical(t)); 125 if (src.hasStatus()) 126 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 127 if (src.hasExperimental()) 128 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 129 if (src.hasDate()) 130 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 131 if (src.hasPublisher()) 132 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 133 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 134 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 135 if (src.hasDescription()) 136 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 137 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 138 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 139 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 140 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 141 if (src.hasCopyright()) 142 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 143 if (src.hasApprovalDate()) 144 tgt.setApprovalDateElement(Date40_50.convertDate(src.getApprovalDateElement())); 145 if (src.hasLastReviewDate()) 146 tgt.setLastReviewDateElement(Date40_50.convertDate(src.getLastReviewDateElement())); 147 if (src.hasEffectivePeriod()) 148 tgt.setEffectivePeriod(Period40_50.convertPeriod(src.getEffectivePeriod())); 149 if (src.hasCode()) 150 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 151 for (org.hl7.fhir.r5.model.Reference t : src.getInstance()) tgt.addInstance(Reference40_50.convertReference(t)); 152 for (org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent t : src.getApplicability()) 153 tgt.addApplicability(convertChargeItemDefinitionApplicabilityComponent(t)); 154 for (org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent t : src.getPropertyGroup()) 155 tgt.addPropertyGroup(convertChargeItemDefinitionPropertyGroupComponent(t)); 156 return tgt; 157 } 158 159 public static org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent convertChargeItemDefinitionApplicabilityComponent(org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent src) throws FHIRException { 160 if (src == null) 161 return null; 162 org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent tgt = new org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent(); 163 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 164 if (src.hasDescription()) 165 tgt.getCondition().setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 166 if (src.hasLanguage()) 167 tgt.getCondition().setLanguage(src.getLanguage()); 168 if (src.hasExpression()) 169 tgt.getCondition().setExpressionElement(String40_50.convertString(src.getExpressionElement())); 170 return tgt; 171 } 172 173 public static org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent convertChargeItemDefinitionApplicabilityComponent(org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent src) throws FHIRException { 174 if (src == null) 175 return null; 176 org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent tgt = new org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent(); 177 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 178 if (src.getCondition().hasDescription()) 179 tgt.setDescriptionElement(String40_50.convertString(src.getCondition().getDescriptionElement())); 180 if (src.getCondition().hasLanguage()) 181 tgt.setLanguageElement(String40_50.convertString(src.getCondition().getLanguageElement())); 182 if (src.getCondition().hasExpression()) 183 tgt.setExpressionElement(String40_50.convertString(src.getCondition().getExpressionElement())); 184 return tgt; 185 } 186 187 public static org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent convertChargeItemDefinitionPropertyGroupComponent(org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent src) throws FHIRException { 188 if (src == null) 189 return null; 190 org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent tgt = new org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent(); 191 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 192 for (org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent t : src.getApplicability()) 193 tgt.addApplicability(convertChargeItemDefinitionApplicabilityComponent(t)); 194 for (org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent t : src.getPriceComponent()) 195 tgt.addPriceComponent(convertChargeItemDefinitionPropertyGroupPriceComponentComponent(t)); 196 return tgt; 197 } 198 199 public static org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent convertChargeItemDefinitionPropertyGroupComponent(org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent src) throws FHIRException { 200 if (src == null) 201 return null; 202 org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent tgt = new org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent(); 203 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 204 for (org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent t : src.getApplicability()) 205 tgt.addApplicability(convertChargeItemDefinitionApplicabilityComponent(t)); 206 for (org.hl7.fhir.r5.model.MonetaryComponent t : src.getPriceComponent()) 207 tgt.addPriceComponent(convertChargeItemDefinitionPropertyGroupPriceComponentComponent(t)); 208 return tgt; 209 } 210 211 public static org.hl7.fhir.r5.model.MonetaryComponent convertChargeItemDefinitionPropertyGroupPriceComponentComponent(org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent src) throws FHIRException { 212 if (src == null) 213 return null; 214 org.hl7.fhir.r5.model.MonetaryComponent tgt = new org.hl7.fhir.r5.model.MonetaryComponent(); 215 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 216 if (src.hasType()) 217 tgt.setTypeElement(convertChargeItemDefinitionPriceComponentType(src.getTypeElement())); 218 if (src.hasCode()) 219 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 220 if (src.hasFactor()) 221 tgt.setFactorElement(Decimal40_50.convertDecimal(src.getFactorElement())); 222 if (src.hasAmount()) 223 tgt.setAmount(Money40_50.convertMoney(src.getAmount())); 224 return tgt; 225 } 226 227 public static org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent convertChargeItemDefinitionPropertyGroupPriceComponentComponent(org.hl7.fhir.r5.model.MonetaryComponent src) throws FHIRException { 228 if (src == null) 229 return null; 230 org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent tgt = new org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent(); 231 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 232 if (src.hasType()) 233 tgt.setTypeElement(convertChargeItemDefinitionPriceComponentType(src.getTypeElement())); 234 if (src.hasCode()) 235 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 236 if (src.hasFactor()) 237 tgt.setFactorElement(Decimal40_50.convertDecimal(src.getFactorElement())); 238 if (src.hasAmount()) 239 tgt.setAmount(Money40_50.convertMoney(src.getAmount())); 240 return tgt; 241 } 242 243 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MonetaryComponent.PriceComponentType> convertChargeItemDefinitionPriceComponentType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPriceComponentType> src) throws FHIRException { 244 if (src == null || src.isEmpty()) 245 return null; 246 Enumeration<MonetaryComponent.PriceComponentType> tgt = new Enumeration<>(new MonetaryComponent.PriceComponentTypeEnumFactory()); 247 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 248 if (src.getValue() == null) { 249 tgt.setValue(null); 250 } else { 251 switch (src.getValue()) { 252 case BASE: 253 tgt.setValue(MonetaryComponent.PriceComponentType.BASE); 254 break; 255 case SURCHARGE: 256 tgt.setValue(MonetaryComponent.PriceComponentType.SURCHARGE); 257 break; 258 case DEDUCTION: 259 tgt.setValue(MonetaryComponent.PriceComponentType.DEDUCTION); 260 break; 261 case DISCOUNT: 262 tgt.setValue(MonetaryComponent.PriceComponentType.DISCOUNT); 263 break; 264 case TAX: 265 tgt.setValue(MonetaryComponent.PriceComponentType.TAX); 266 break; 267 case INFORMATIONAL: 268 tgt.setValue(MonetaryComponent.PriceComponentType.INFORMATIONAL); 269 break; 270 default: 271 tgt.setValue(MonetaryComponent.PriceComponentType.NULL); 272 break; 273 } 274 } 275 return tgt; 276 } 277 278 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ChargeItemDefinition.ChargeItemDefinitionPriceComponentType> convertChargeItemDefinitionPriceComponentType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MonetaryComponent.PriceComponentType> src) throws FHIRException { 279 if (src == null || src.isEmpty()) 280 return null; 281 org.hl7.fhir.r4.model.Enumeration<ChargeItemDefinition.ChargeItemDefinitionPriceComponentType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ChargeItemDefinition.ChargeItemDefinitionPriceComponentTypeEnumFactory()); 282 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 283 if (src.getValue() == null) { 284 tgt.setValue(null); 285 } else { 286 switch (src.getValue()) { 287 case BASE: 288 tgt.setValue(ChargeItemDefinition.ChargeItemDefinitionPriceComponentType.BASE); 289 break; 290 case SURCHARGE: 291 tgt.setValue(ChargeItemDefinition.ChargeItemDefinitionPriceComponentType.SURCHARGE); 292 break; 293 case DEDUCTION: 294 tgt.setValue(ChargeItemDefinition.ChargeItemDefinitionPriceComponentType.DEDUCTION); 295 break; 296 case DISCOUNT: 297 tgt.setValue(ChargeItemDefinition.ChargeItemDefinitionPriceComponentType.DISCOUNT); 298 break; 299 case TAX: 300 tgt.setValue(ChargeItemDefinition.ChargeItemDefinitionPriceComponentType.TAX); 301 break; 302 case INFORMATIONAL: 303 tgt.setValue(ChargeItemDefinition.ChargeItemDefinitionPriceComponentType.INFORMATIONAL); 304 break; 305 default: 306 tgt.setValue(ChargeItemDefinition.ChargeItemDefinitionPriceComponentType.NULL); 307 break; 308 } 309 } 310 return tgt; 311 } 312}