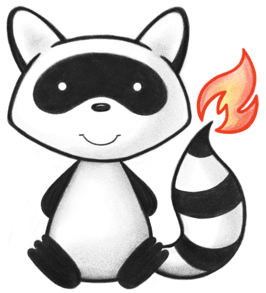
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Narrative40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.CodeableReference; 013import org.hl7.fhir.r5.model.RelatedArtifact; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class Composition40_50 { 045 046 public static org.hl7.fhir.r5.model.Composition convertComposition(org.hl7.fhir.r4.model.Composition src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.Composition tgt = new org.hl7.fhir.r5.model.Composition(); 050 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 051 if (src.hasIdentifier()) 052 tgt.addIdentifier(Identifier40_50.convertIdentifier(src.getIdentifier())); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertCompositionStatus(src.getStatusElement())); 055 if (src.hasType()) 056 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 057 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 058 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 059 if (src.hasSubject()) 060 tgt.addSubject(Reference40_50.convertReference(src.getSubject())); 061 if (src.hasEncounter()) 062 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 063 if (src.hasDate()) 064 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 065 for (org.hl7.fhir.r4.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference40_50.convertReference(t)); 066 if (src.hasTitle()) 067 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 068 if (src.hasConfidentiality()) 069 tgt.getMeta().addSecurity().setCode(src.getConfidentiality().toCode()); 070 for (org.hl7.fhir.r4.model.Composition.CompositionAttesterComponent t : src.getAttester()) 071 tgt.addAttester(convertCompositionAttesterComponent(t)); 072 if (src.hasCustodian()) 073 tgt.setCustodian(Reference40_50.convertReference(src.getCustodian())); 074 for (org.hl7.fhir.r4.model.Composition.CompositionRelatesToComponent t : src.getRelatesTo()) 075 tgt.addRelatesTo(convertCompositionRelatesToComponent(t)); 076 for (org.hl7.fhir.r4.model.Composition.CompositionEventComponent t : src.getEvent()) 077 tgt.addEvent(convertCompositionEventComponent(t)); 078 for (org.hl7.fhir.r4.model.Composition.SectionComponent t : src.getSection()) 079 tgt.addSection(convertSectionComponent(t)); 080 return tgt; 081 } 082 083 public static org.hl7.fhir.r4.model.Composition convertComposition(org.hl7.fhir.r5.model.Composition src) throws FHIRException { 084 if (src == null) 085 return null; 086 org.hl7.fhir.r4.model.Composition tgt = new org.hl7.fhir.r4.model.Composition(); 087 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 088 if (src.hasIdentifier()) 089 tgt.setIdentifier(Identifier40_50.convertIdentifier(src.getIdentifierFirstRep())); 090 if (src.hasStatus()) 091 tgt.setStatusElement(convertCompositionStatus(src.getStatusElement())); 092 if (src.hasType()) 093 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 094 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 095 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 096 if (src.hasSubject()) 097 tgt.setSubject(Reference40_50.convertReference(src.getSubjectFirstRep())); 098 if (src.hasEncounter()) 099 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 100 if (src.hasDate()) 101 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 102 for (org.hl7.fhir.r5.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference40_50.convertReference(t)); 103 if (src.hasTitle()) 104 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 105 if (src.getMeta().hasSecurity()) 106 tgt.setConfidentialityElement(convertDocumentConfidentiality(src.getMeta().getSecurityFirstRep())); 107 for (org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent t : src.getAttester()) 108 tgt.addAttester(convertCompositionAttesterComponent(t)); 109 if (src.hasCustodian()) 110 tgt.setCustodian(Reference40_50.convertReference(src.getCustodian())); 111 for (RelatedArtifact t : src.getRelatesTo()) 112 tgt.addRelatesTo(convertCompositionRelatesToComponent(t)); 113 for (org.hl7.fhir.r5.model.Composition.CompositionEventComponent t : src.getEvent()) 114 tgt.addEvent(convertCompositionEventComponent(t)); 115 for (org.hl7.fhir.r5.model.Composition.SectionComponent t : src.getSection()) 116 tgt.addSection(convertSectionComponent(t)); 117 return tgt; 118 } 119 120 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> convertCompositionStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.CompositionStatus> src) throws FHIRException { 121 if (src == null || src.isEmpty()) 122 return null; 123 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.CompositionStatusEnumFactory()); 124 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 125 switch (src.getValue()) { 126 case PRELIMINARY: 127 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.PRELIMINARY); 128 break; 129 case FINAL: 130 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.FINAL); 131 break; 132 case AMENDED: 133 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.AMENDED); 134 break; 135 case ENTEREDINERROR: 136 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.ENTEREDINERROR); 137 break; 138 default: 139 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.CompositionStatus.NULL); 140 break; 141 } 142 return tgt; 143 } 144 145 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.CompositionStatus> convertCompositionStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> src) throws FHIRException { 146 if (src == null || src.isEmpty()) 147 return null; 148 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.CompositionStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Composition.CompositionStatusEnumFactory()); 149 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 150 switch (src.getValue()) { 151 case PRELIMINARY: 152 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionStatus.PRELIMINARY); 153 break; 154 case FINAL: 155 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionStatus.FINAL); 156 break; 157 case AMENDED: 158 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionStatus.AMENDED); 159 break; 160 case ENTEREDINERROR: 161 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionStatus.ENTEREDINERROR); 162 break; 163 default: 164 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionStatus.NULL); 165 break; 166 } 167 return tgt; 168 } 169 170 static public org.hl7.fhir.r5.model.Coding convertDocumentConfidentiality(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.DocumentConfidentiality> src) throws FHIRException { 171 if (src == null || src.isEmpty()) 172 return null; 173 org.hl7.fhir.r5.model.Coding tgt = new org.hl7.fhir.r5.model.Coding(); 174 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 175 tgt.setCode(src.getValue().toCode()); 176 return tgt; 177 } 178 179 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.DocumentConfidentiality> convertDocumentConfidentiality(org.hl7.fhir.r5.model.Coding src) throws FHIRException { 180 if (src == null || src.isEmpty()) 181 return null; 182 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.DocumentConfidentiality> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Composition.DocumentConfidentialityEnumFactory()); 183 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 184 switch (src.getCode()) { 185 case "U": 186 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentConfidentiality.U); 187 break; 188 case "L": 189 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentConfidentiality.L); 190 break; 191 case "M": 192 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentConfidentiality.M); 193 break; 194 case "N": 195 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentConfidentiality.N); 196 break; 197 case "R": 198 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentConfidentiality.R); 199 break; 200 case "V": 201 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentConfidentiality.V); 202 break; 203 default: 204 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentConfidentiality.NULL); 205 break; 206 } 207 return tgt; 208 } 209 210 public static org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent convertCompositionAttesterComponent(org.hl7.fhir.r4.model.Composition.CompositionAttesterComponent src) throws FHIRException { 211 if (src == null) 212 return null; 213 org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent tgt = new org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent(); 214 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 215 if (src.hasMode()) 216 tgt.setMode(convertCompositionAttestationMode(src.getModeElement())); 217 if (src.hasTime()) 218 tgt.setTimeElement(DateTime40_50.convertDateTime(src.getTimeElement())); 219 if (src.hasParty()) 220 tgt.setParty(Reference40_50.convertReference(src.getParty())); 221 return tgt; 222 } 223 224 public static org.hl7.fhir.r4.model.Composition.CompositionAttesterComponent convertCompositionAttesterComponent(org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent src) throws FHIRException { 225 if (src == null) 226 return null; 227 org.hl7.fhir.r4.model.Composition.CompositionAttesterComponent tgt = new org.hl7.fhir.r4.model.Composition.CompositionAttesterComponent(); 228 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 229 if (src.hasMode()) 230 tgt.setModeElement(convertCompositionAttestationMode(src.getMode())); 231 if (src.hasTime()) 232 tgt.setTimeElement(DateTime40_50.convertDateTime(src.getTimeElement())); 233 if (src.hasParty()) 234 tgt.setParty(Reference40_50.convertReference(src.getParty())); 235 return tgt; 236 } 237 238 static public org.hl7.fhir.r5.model.CodeableConcept convertCompositionAttestationMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.CompositionAttestationMode> src) throws FHIRException { 239 if (src == null || src.isEmpty()) 240 return null; 241 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 242 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 243 switch (src.getValue()) { 244 case PERSONAL: 245 tgt.addCoding().setSystem("http://hl7.org/fhir/composition-attestation-mode").setCode("personal"); 246 break; 247 case PROFESSIONAL: 248 tgt.addCoding().setSystem("http://hl7.org/fhir/composition-attestation-mode").setCode("professional"); 249 break; 250 case LEGAL: 251 tgt.addCoding().setSystem("http://hl7.org/fhir/composition-attestation-mode").setCode("legal"); 252 break; 253 case OFFICIAL: 254 tgt.addCoding().setSystem("http://hl7.org/fhir/composition-attestation-mode").setCode("official"); 255 break; 256 default: 257 break; 258 } 259 return tgt; 260 } 261 262 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.CompositionAttestationMode> convertCompositionAttestationMode(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 263 if (src == null || src.isEmpty()) 264 return null; 265 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.CompositionAttestationMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Composition.CompositionAttestationModeEnumFactory()); 266 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 267 switch (src.getCode("http://hl7.org/fhir/composition-attestation-mode")) { 268 case "personal": 269 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionAttestationMode.PERSONAL); 270 break; 271 case "professional": 272 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionAttestationMode.PROFESSIONAL); 273 break; 274 case "legal": 275 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionAttestationMode.LEGAL); 276 break; 277 case "official": 278 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionAttestationMode.OFFICIAL); 279 break; 280 default: 281 tgt.setValue(org.hl7.fhir.r4.model.Composition.CompositionAttestationMode.NULL); 282 break; 283 } 284 return tgt; 285 } 286 287 public static org.hl7.fhir.r5.model.RelatedArtifact convertCompositionRelatesToComponent(org.hl7.fhir.r4.model.Composition.CompositionRelatesToComponent src) throws FHIRException { 288 if (src == null) 289 return null; 290 org.hl7.fhir.r5.model.RelatedArtifact tgt = new org.hl7.fhir.r5.model.RelatedArtifact(); 291 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 292 if (src.hasCode()) 293 tgt.setTypeElement(convertDocumentRelationshipType(src.getCodeElement())); 294 if (src.hasTargetReference()) 295 tgt.setResourceReference(Reference40_50.convertReference(src.getTargetReference())); 296 return tgt; 297 } 298 299 public static org.hl7.fhir.r4.model.Composition.CompositionRelatesToComponent convertCompositionRelatesToComponent(org.hl7.fhir.r5.model.RelatedArtifact src) throws FHIRException { 300 if (src == null) 301 return null; 302 org.hl7.fhir.r4.model.Composition.CompositionRelatesToComponent tgt = new org.hl7.fhir.r4.model.Composition.CompositionRelatesToComponent(); 303 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 304 if (src.hasType()) 305 tgt.setCodeElement(convertDocumentRelationshipType(src.getTypeElement())); 306 if (src.hasResourceReference()) 307 tgt.setTarget(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getResourceReference())); 308 return tgt; 309 } 310 311 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> convertDocumentRelationshipType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.DocumentRelationshipType> src) throws FHIRException { 312 if (src == null || src.isEmpty()) 313 return null; 314 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactTypeEnumFactory()); 315 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 316 switch (src.getValue()) { 317 case REPLACES: 318 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.REPLACES); 319 break; 320 case TRANSFORMS: 321 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.TRANSFORMS); 322 break; 323 case SIGNS: 324 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.SIGNS); 325 break; 326 case APPENDS: 327 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.APPENDS); 328 break; 329 default: 330 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.NULL); 331 break; 332 } 333 return tgt; 334 } 335 336 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> src) throws FHIRException { 337 if (src == null || src.isEmpty()) 338 return null; 339 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.DocumentRelationshipType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Composition.DocumentRelationshipTypeEnumFactory()); 340 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 341 switch (src.getValue()) { 342 case REPLACES: 343 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentRelationshipType.REPLACES); 344 break; 345 case TRANSFORMS: 346 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentRelationshipType.TRANSFORMS); 347 break; 348 case SIGNS: 349 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentRelationshipType.SIGNS); 350 break; 351 case APPENDS: 352 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentRelationshipType.APPENDS); 353 break; 354 default: 355 tgt.setValue(org.hl7.fhir.r4.model.Composition.DocumentRelationshipType.NULL); 356 break; 357 } 358 return tgt; 359 } 360 361 public static org.hl7.fhir.r5.model.Composition.CompositionEventComponent convertCompositionEventComponent(org.hl7.fhir.r4.model.Composition.CompositionEventComponent src) throws FHIRException { 362 if (src == null) 363 return null; 364 org.hl7.fhir.r5.model.Composition.CompositionEventComponent tgt = new org.hl7.fhir.r5.model.Composition.CompositionEventComponent(); 365 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 366 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCode()) 367 tgt.addDetail().setConcept(CodeableConcept40_50.convertCodeableConcept(t)); 368 if (src.hasPeriod()) 369 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 370 for (org.hl7.fhir.r4.model.Reference t : src.getDetail()) tgt.addDetail().setReference(Reference40_50.convertReference(t)); 371 return tgt; 372 } 373 374 public static org.hl7.fhir.r4.model.Composition.CompositionEventComponent convertCompositionEventComponent(org.hl7.fhir.r5.model.Composition.CompositionEventComponent src) throws FHIRException { 375 if (src == null) 376 return null; 377 org.hl7.fhir.r4.model.Composition.CompositionEventComponent tgt = new org.hl7.fhir.r4.model.Composition.CompositionEventComponent(); 378 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 379 if (src.hasPeriod()) 380 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 381 for (CodeableReference t : src.getDetail()) { 382 if (t.hasConcept()) { 383 tgt.addCode(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 384 } 385 if (t.hasReference()) { 386 tgt.addDetail(Reference40_50.convertReference(t.getReference())); 387 } 388 } 389 return tgt; 390 } 391 392 public static org.hl7.fhir.r5.model.Composition.SectionComponent convertSectionComponent(org.hl7.fhir.r4.model.Composition.SectionComponent src) throws FHIRException { 393 if (src == null) 394 return null; 395 org.hl7.fhir.r5.model.Composition.SectionComponent tgt = new org.hl7.fhir.r5.model.Composition.SectionComponent(); 396 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 397 if (src.hasTitle()) 398 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 399 if (src.hasCode()) 400 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 401 for (org.hl7.fhir.r4.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference40_50.convertReference(t)); 402 if (src.hasFocus()) 403 tgt.setFocus(Reference40_50.convertReference(src.getFocus())); 404 if (src.hasText()) 405 tgt.setText(Narrative40_50.convertNarrative(src.getText())); 406// if (src.hasMode()) 407// tgt.setModeElement(convertSectionMode(src.getModeElement())); 408 if (src.hasOrderedBy()) 409 tgt.setOrderedBy(CodeableConcept40_50.convertCodeableConcept(src.getOrderedBy())); 410 for (org.hl7.fhir.r4.model.Reference t : src.getEntry()) tgt.addEntry(Reference40_50.convertReference(t)); 411 if (src.hasEmptyReason()) 412 tgt.setEmptyReason(CodeableConcept40_50.convertCodeableConcept(src.getEmptyReason())); 413 for (org.hl7.fhir.r4.model.Composition.SectionComponent t : src.getSection()) 414 tgt.addSection(convertSectionComponent(t)); 415 return tgt; 416 } 417 418 public static org.hl7.fhir.r4.model.Composition.SectionComponent convertSectionComponent(org.hl7.fhir.r5.model.Composition.SectionComponent src) throws FHIRException { 419 if (src == null) 420 return null; 421 org.hl7.fhir.r4.model.Composition.SectionComponent tgt = new org.hl7.fhir.r4.model.Composition.SectionComponent(); 422 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 423 if (src.hasTitle()) 424 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 425 if (src.hasCode()) 426 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 427 for (org.hl7.fhir.r5.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference40_50.convertReference(t)); 428 if (src.hasFocus()) 429 tgt.setFocus(Reference40_50.convertReference(src.getFocus())); 430 if (src.hasText()) 431 tgt.setText(Narrative40_50.convertNarrative(src.getText())); 432// if (src.hasMode()) 433// tgt.setModeElement(convertSectionMode(src.getModeElement())); 434 if (src.hasOrderedBy()) 435 tgt.setOrderedBy(CodeableConcept40_50.convertCodeableConcept(src.getOrderedBy())); 436 for (org.hl7.fhir.r5.model.Reference t : src.getEntry()) tgt.addEntry(Reference40_50.convertReference(t)); 437 if (src.hasEmptyReason()) 438 tgt.setEmptyReason(CodeableConcept40_50.convertCodeableConcept(src.getEmptyReason())); 439 for (org.hl7.fhir.r5.model.Composition.SectionComponent t : src.getSection()) 440 tgt.addSection(convertSectionComponent(t)); 441 return tgt; 442 } 443 444 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ListMode> convertSectionMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.SectionMode> src) throws FHIRException { 445 if (src == null || src.isEmpty()) 446 return null; 447 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ListMode> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ListModeEnumFactory()); 448 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 449 switch (src.getValue()) { 450 case WORKING: 451 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ListMode.WORKING); 452 break; 453 case SNAPSHOT: 454 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ListMode.SNAPSHOT); 455 break; 456 case CHANGES: 457 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ListMode.CHANGES); 458 break; 459 default: 460 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ListMode.NULL); 461 break; 462 } 463 return tgt; 464 } 465 466 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.SectionMode> convertSectionMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ListMode> src) throws FHIRException { 467 if (src == null || src.isEmpty()) 468 return null; 469 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Composition.SectionMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Composition.SectionModeEnumFactory()); 470 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 471 switch (src.getValue()) { 472 case WORKING: 473 tgt.setValue(org.hl7.fhir.r4.model.Composition.SectionMode.WORKING); 474 break; 475 case SNAPSHOT: 476 tgt.setValue(org.hl7.fhir.r4.model.Composition.SectionMode.SNAPSHOT); 477 break; 478 case CHANGES: 479 tgt.setValue(org.hl7.fhir.r4.model.Composition.SectionMode.CHANGES); 480 break; 481 default: 482 tgt.setValue(org.hl7.fhir.r4.model.Composition.SectionMode.NULL); 483 break; 484 } 485 return tgt; 486 } 487}