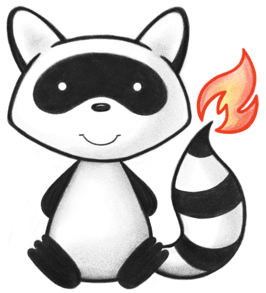
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.ContactDetail40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.UsageContext40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Code40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 014import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 015import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 016import org.hl7.fhir.exceptions.FHIRException; 017import org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence; 018import org.hl7.fhir.r5.model.*; 019import org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship; 020import org.hl7.fhir.r5.utils.ToolingExtensions; 021import org.hl7.fhir.utilities.CanonicalPair; 022 023/* 024 Copyright (c) 2011+, HL7, Inc. 025 All rights reserved. 026 027 Redistribution and use in source and binary forms, with or without modification, 028 are permitted provided that the following conditions are met: 029 030 * Redistributions of source code must retain the above copyright notice, this 031 list of conditions and the following disclaimer. 032 * Redistributions in binary form must reproduce the above copyright notice, 033 this list of conditions and the following disclaimer in the documentation 034 and/or other materials provided with the distribution. 035 * Neither the name of HL7 nor the names of its contributors may be used to 036 endorse or promote products derived from this software without specific 037 prior written permission. 038 039 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 040 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 041 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 042 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 043 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 044 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 045 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 046 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 047 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 048 POSSIBILITY OF SUCH DAMAGE. 049 050*/ 051// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 052public class ConceptMap40_50 { 053 054 public static org.hl7.fhir.r5.model.ConceptMap convertConceptMap(org.hl7.fhir.r4.model.ConceptMap src) throws FHIRException { 055 if (src == null) 056 return null; 057 org.hl7.fhir.r5.model.ConceptMap tgt = new org.hl7.fhir.r5.model.ConceptMap(); 058 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 059 if (src.hasUrl()) 060 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 061 if (src.hasIdentifier()) 062 tgt.addIdentifier(Identifier40_50.convertIdentifier(src.getIdentifier())); 063 if (src.hasVersion()) 064 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 065 if (src.hasName()) 066 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 067 if (src.hasTitle()) 068 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 069 if (src.hasStatus()) 070 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 071 if (src.hasExperimental()) 072 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 073 if (src.hasDate()) 074 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 075 if (src.hasPublisher()) 076 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 077 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 078 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 079 if (src.hasDescription()) 080 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 081 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 082 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 083 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 084 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 085 if (src.hasPurpose()) 086 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 087 if (src.hasCopyright()) 088 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 089 if (src.hasSource()) 090 tgt.setSourceScope(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getSource())); 091 if (src.hasTarget()) 092 tgt.setTargetScope(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getTarget())); 093 for (org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent t : src.getGroup()) 094 tgt.addGroup(convertConceptMapGroupComponent(t, tgt)); 095 return tgt; 096 } 097 098 public static org.hl7.fhir.r4.model.ConceptMap convertConceptMap(org.hl7.fhir.r5.model.ConceptMap src) throws FHIRException { 099 if (src == null) 100 return null; 101 org.hl7.fhir.r4.model.ConceptMap tgt = new org.hl7.fhir.r4.model.ConceptMap(); 102 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 103 if (src.hasUrl()) 104 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 105 if (src.hasIdentifier()) 106 tgt.setIdentifier(Identifier40_50.convertIdentifier(src.getIdentifierFirstRep())); 107 if (src.hasVersion()) 108 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 109 if (src.hasName()) 110 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 111 if (src.hasTitle()) 112 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 113 if (src.hasStatus()) 114 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 115 if (src.hasExperimental()) 116 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 117 if (src.hasDate()) 118 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 119 if (src.hasPublisher()) 120 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 121 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 122 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 123 if (src.hasDescription()) 124 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 125 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 126 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 127 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 128 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 129 if (src.hasPurpose()) 130 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 131 if (src.hasCopyright()) 132 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 133 if (src.hasSourceScope()) 134 tgt.setSource(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getSourceScope())); 135 if (src.hasTargetScope()) 136 tgt.setTarget(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getTargetScope())); 137 for (org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent t : src.getGroup()) 138 tgt.addGroup(convertConceptMapGroupComponent(t, src)); 139 return tgt; 140 } 141 142 public static org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent convertConceptMapGroupComponent(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 143 if (src == null) 144 return null; 145 org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent(); 146 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 147 if (src.hasSource() || src.hasSourceVersion()) 148 tgt.setSourceElement(convertUriAndVersionToCanonical(src.getSourceElement(), src.getSourceVersionElement())); 149 if (src.hasTarget() || src.hasTargetVersion()) 150 tgt.setTargetElement(convertUriAndVersionToCanonical(src.getTargetElement(), src.getTargetVersionElement())); 151 for (org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent t : src.getElement()) 152 tgt.addElement(convertSourceElementComponent(t, tgtMap)); 153 if (src.hasUnmapped()) 154 tgt.setUnmapped(convertConceptMapGroupUnmappedComponent(src.getUnmapped())); 155 return tgt; 156 } 157 158 private static CanonicalType convertUriAndVersionToCanonical(org.hl7.fhir.r4.model.UriType srcUri, org.hl7.fhir.r4.model.StringType srcVersion) { 159 if (srcUri == null && srcVersion == null) 160 return null; 161 org.hl7.fhir.r5.model.CanonicalType tgt = new org.hl7.fhir.r5.model.CanonicalType(); 162 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(srcUri == null ? srcVersion : srcUri, tgt); 163 if (srcUri.hasValue()) { 164 if (srcVersion.hasValue()) { 165 tgt.setValue(srcUri.getValue() + "|" + srcVersion.getValue()); 166 } else { 167 tgt.setValue(srcUri.getValue()); 168 } 169 } 170 return tgt; 171 } 172 173 public static org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent convertConceptMapGroupComponent(org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupComponent src, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 174 if (src == null) 175 return null; 176 org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupComponent(); 177 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 178 if (src.hasSource()) { 179 CanonicalPair cp = new CanonicalPair(src.getSource()); 180 tgt.setSource(cp.getUrl()); 181 tgt.setSourceVersion(cp.getVersion()); 182 } 183 if (src.hasTarget()) { 184 CanonicalPair cp = new CanonicalPair(src.getTarget()); 185 tgt.setTarget(cp.getUrl()); 186 tgt.setTargetVersion(cp.getVersion()); 187 } 188 for (org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent t : src.getElement()) 189 tgt.addElement(convertSourceElementComponent(t, srcMap)); 190 if (src.hasUnmapped()) 191 tgt.setUnmapped(convertConceptMapGroupUnmappedComponent(src.getUnmapped())); 192 return tgt; 193 } 194 195 public static org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent convertSourceElementComponent(org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 196 if (src == null) 197 return null; 198 org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent(); 199 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 200 if (src.hasCode()) 201 tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 202 if (src.hasDisplay()) 203 tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 204 for (org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent t : src.getTarget()) { 205 if (t.getEquivalence() == org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.UNMATCHED) { 206 tgt.setNoMap(true); 207 if (t.hasComment()) { 208 if (tgt.hasExtension(ToolingExtensions.EXT_CM_NOMAP_COMMENT)) { 209 throw new FHIRException("A source can only have one 'unmatched' relationship. Consider using 'disjoint' "); 210 } 211 tgt.addExtension(ToolingExtensions.EXT_CM_NOMAP_COMMENT, ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(t.getCommentElement())); 212 } 213 } else { 214 tgt.addTarget(convertTargetElementComponent(t, tgtMap)); 215 } 216 } 217 return tgt; 218 } 219 220 public static org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent convertSourceElementComponent(org.hl7.fhir.r5.model.ConceptMap.SourceElementComponent src, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 221 if (src == null) 222 return null; 223 org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.SourceElementComponent(); 224 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt, ToolingExtensions.EXT_CM_NOMAP_COMMENT); 225 if (src.hasCode()) 226 tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 227 if (src.hasDisplay()) 228 tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 229 if (src.hasNoMap() && src.getNoMap() == true) { 230 org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent t = new org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent(); 231 t.setEquivalence(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.UNMATCHED); 232 if (src.hasExtension(ToolingExtensions.EXT_CM_NOMAP_COMMENT)) { 233 t.setCommentElement((org.hl7.fhir.r4.model.StringType) ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getExtensionByUrl(ToolingExtensions.EXT_CM_NOMAP_COMMENT).getValue())); 234 } 235 tgt.addTarget(t); 236 } else { 237 for (org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent t : src.getTarget()) 238 tgt.addTarget(convertTargetElementComponent(t, srcMap)); 239 } 240 return tgt; 241 } 242 243 public static org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 244 if (src == null) 245 return null; 246 org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent(); 247 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 248 if (src.hasCode()) 249 tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 250 if (src.hasDisplay()) 251 tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 252 if (src.hasEquivalence()) 253 tgt.setRelationshipElement(convertConceptMapRelationship(src.getEquivalenceElement(), tgt)); 254 if (src.hasComment()) 255 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 256 for (org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 257 tgt.addDependsOn(convertOtherElementComponent(t, tgtMap)); 258 for (org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent t : src.getProduct()) 259 tgt.addProduct(convertOtherElementComponent(t, tgtMap)); 260 return tgt; 261 } 262 263 public static org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent convertTargetElementComponent(org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent src, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 264 if (src == null) 265 return null; 266 org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.TargetElementComponent(); 267 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt, VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE); 268 if (src.hasCode()) 269 tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 270 if (src.hasDisplay()) 271 tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 272 if (src.hasRelationship()) 273 tgt.setEquivalenceElement(convertConceptMapEquivalence(src.getRelationshipElement(), src)); 274 else 275 tgt.setEquivalence(ConceptMapEquivalence.RELATEDTO); 276 if (src.hasComment()) 277 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 278 for (org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent t : src.getDependsOn()) 279 tgt.addDependsOn(convertOtherElementComponent(t, srcMap)); 280 for (org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent t : src.getProduct()) 281 tgt.addProduct(convertOtherElementComponent(t, srcMap)); 282 return tgt; 283 } 284 285 public static org.hl7.fhir.r4.model.Enumeration<ConceptMapEquivalence> convertConceptMapEquivalence(Enumeration<ConceptMapRelationship> src, org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent ccm) throws FHIRException { 286 if (src == null || src.isEmpty()) 287 return null; 288 org.hl7.fhir.r4.model.Enumeration<ConceptMapEquivalence> tgt = new org.hl7.fhir.r4.model.Enumeration<ConceptMapEquivalence>(new org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalenceEnumFactory()); 289 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 290 if (ccm.hasExtension(VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE)) { 291 tgt.setValueAsString(ccm.getExtensionString(VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE)); 292 } else { 293 if (src.getValue() == null) { 294 tgt.setValue(null); 295 } else { 296 switch (src.getValue()) { 297 case RELATEDTO: 298 tgt.setValue(ConceptMapEquivalence.RELATEDTO); 299 break; 300 case EQUIVALENT: 301 tgt.setValue(ConceptMapEquivalence.EQUIVALENT); 302 break; 303 case SOURCEISNARROWERTHANTARGET: 304 tgt.setValue(ConceptMapEquivalence.WIDER); 305 break; 306 case SOURCEISBROADERTHANTARGET: 307 tgt.setValue(ConceptMapEquivalence.NARROWER); 308 break; 309 case NOTRELATEDTO: 310 tgt.setValue(ConceptMapEquivalence.DISJOINT); 311 break; 312 default: 313 tgt.setValue(ConceptMapEquivalence.NULL); 314 break; 315 } 316 } 317 } 318 return tgt; 319 } 320 321 public static Enumeration<ConceptMapRelationship> convertConceptMapRelationship(org.hl7.fhir.r4.model.Enumeration<ConceptMapEquivalence> src, org.hl7.fhir.r5.model.ConceptMap.TargetElementComponent tgtCtxt) throws FHIRException { 322 if (src == null || src.isEmpty()) 323 return null; 324 Enumeration<ConceptMapRelationship> tgt = new Enumeration<ConceptMapRelationship>(new Enumerations.ConceptMapRelationshipEnumFactory()); 325 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 326 ToolingExtensions.setCodeExtensionMod(tgtCtxt, VersionConvertorConstants.EXT_OLD_CONCEPTMAP_EQUIVALENCE, src.getValueAsString()); 327 if (src.getValue() == null) { 328 tgt.setValue(null); 329 } else { 330 switch (src.getValue()) { 331 case EQUIVALENT: 332 tgt.setValue(ConceptMapRelationship.EQUIVALENT); 333 break; 334 case EQUAL: 335 tgt.setValue(ConceptMapRelationship.EQUIVALENT); 336 break; 337 case WIDER: 338 tgt.setValue(ConceptMapRelationship.SOURCEISNARROWERTHANTARGET); 339 break; 340 case SUBSUMES: 341 tgt.setValue(ConceptMapRelationship.SOURCEISNARROWERTHANTARGET); 342 break; 343 case NARROWER: 344 tgt.setValue(ConceptMapRelationship.SOURCEISBROADERTHANTARGET); 345 break; 346 case SPECIALIZES: 347 tgt.setValue(ConceptMapRelationship.SOURCEISBROADERTHANTARGET); 348 break; 349 case RELATEDTO: 350 tgt.setValue(ConceptMapRelationship.RELATEDTO); 351 break; 352 case INEXACT: 353 tgt.setValue(ConceptMapRelationship.RELATEDTO); 354 break; 355 case UNMATCHED: 356 tgt.setValue(ConceptMapRelationship.NULL); 357 break; 358 case DISJOINT: 359 tgt.setValue(ConceptMapRelationship.NOTRELATEDTO); 360 break; 361 default: 362 tgt.setValue(ConceptMapRelationship.NULL); 363 break; 364 } 365 } 366 return tgt; 367 } 368 369 public static org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent src, org.hl7.fhir.r5.model.ConceptMap tgtMap) throws FHIRException { 370 if (src == null) 371 return null; 372 org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent(); 373 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 374 if (src.hasProperty()) 375 tgt.setAttribute(tgtMap.registerAttribute(src.getProperty())); 376 if (src.hasSystem()) { 377 tgt.setValue(new Coding().setSystem(src.getSystem()).setCode(src.getValue()).setDisplay(src.getDisplay())); 378 } else if (src.hasValueElement()) { 379 tgt.setValue(String40_50.convertString(src.getValueElement())); 380 } 381 return tgt; 382 } 383 384 public static org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent convertOtherElementComponent(org.hl7.fhir.r5.model.ConceptMap.OtherElementComponent src, org.hl7.fhir.r5.model.ConceptMap srcMap) throws FHIRException { 385 if (src == null) 386 return null; 387 org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.OtherElementComponent(); 388 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 389 if (src.hasAttribute()) 390 tgt.setProperty(srcMap.getAttributeUri(src.getAttribute())); 391 392 if (src.hasValueCoding()) { 393 tgt.setSystem(src.getValueCoding().getSystem()); 394 tgt.setValue(src.getValueCoding().getCode()); 395 tgt.setDisplay(src.getValueCoding().getDisplay()); 396 } else if (src.hasValue()) { 397 tgt.setValue(src.getValue().primitiveValue()); 398 } 399 400 return tgt; 401 } 402 403 public static org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupUnmappedComponent convertConceptMapGroupUnmappedComponent(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedComponent src) throws FHIRException { 404 if (src == null) 405 return null; 406 org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupUnmappedComponent tgt = new org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupUnmappedComponent(); 407 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 408 if (src.hasMode()) 409 tgt.setModeElement(convertConceptMapGroupUnmappedMode(src.getModeElement())); 410 if (src.hasCode()) 411 tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 412 if (src.hasDisplay()) 413 tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 414 if (src.hasUrl()) 415 tgt.setOtherMapElement(Canonical40_50.convertCanonical(src.getUrlElement())); 416 return tgt; 417 } 418 419 public static org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedComponent convertConceptMapGroupUnmappedComponent(org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupUnmappedComponent src) throws FHIRException { 420 if (src == null) 421 return null; 422 org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedComponent tgt = new org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedComponent(); 423 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 424 if (src.hasMode()) 425 tgt.setModeElement(convertConceptMapGroupUnmappedMode(src.getModeElement())); 426 if (src.hasCode()) 427 tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 428 if (src.hasDisplay()) 429 tgt.setDisplayElement(String40_50.convertString(src.getDisplayElement())); 430 if (src.hasOtherMap()) 431 tgt.setUrlElement(Canonical40_50.convertCanonical(src.getOtherMapElement())); 432 return tgt; 433 } 434 435 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupUnmappedMode> convertConceptMapGroupUnmappedMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode> src) throws FHIRException { 436 if (src == null || src.isEmpty()) 437 return null; 438 Enumeration<ConceptMap.ConceptMapGroupUnmappedMode> tgt = new Enumeration<>(new ConceptMap.ConceptMapGroupUnmappedModeEnumFactory()); 439 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 440 if (src.getValue() == null) { 441 tgt.setValue(null); 442 } else { 443 switch (src.getValue()) { 444 case PROVIDED: 445 tgt.setValue(ConceptMap.ConceptMapGroupUnmappedMode.USESOURCECODE); 446 break; 447 case FIXED: 448 tgt.setValue(ConceptMap.ConceptMapGroupUnmappedMode.FIXED); 449 break; 450 case OTHERMAP: 451 tgt.setValue(ConceptMap.ConceptMapGroupUnmappedMode.OTHERMAP); 452 break; 453 default: 454 tgt.setValue(ConceptMap.ConceptMapGroupUnmappedMode.NULL); 455 break; 456 } 457 } 458 return tgt; 459 } 460 461 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode> convertConceptMapGroupUnmappedMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ConceptMap.ConceptMapGroupUnmappedMode> src) throws FHIRException { 462 if (src == null || src.isEmpty()) 463 return null; 464 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedModeEnumFactory()); 465 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 466 if (src.getValue() == null) { 467 tgt.setValue(null); 468 } else { 469 switch (src.getValue()) { 470 case USESOURCECODE: 471 tgt.setValue(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode.PROVIDED); 472 break; 473 case FIXED: 474 tgt.setValue(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode.FIXED); 475 break; 476 case OTHERMAP: 477 tgt.setValue(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode.OTHERMAP); 478 break; 479 default: 480 tgt.setValue(org.hl7.fhir.r4.model.ConceptMap.ConceptMapGroupUnmappedMode.NULL); 481 break; 482 } 483 } 484 return tgt; 485 } 486}