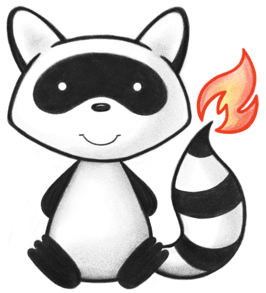
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import java.util.ArrayList; 004import java.util.List; 005 006import org.hl7.fhir.convertors.context.ConversionContext40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Annotation40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.CodeableConcept; 014import org.hl7.fhir.r5.model.CodeableReference; 015import org.hl7.fhir.r5.model.Coding; 016 017/* 018 Copyright (c) 2011+, HL7, Inc. 019 All rights reserved. 020 021 Redistribution and use in source and binary forms, with or without modification, 022 are permitted provided that the following conditions are met: 023 024 * Redistributions of source code must retain the above copyright notice, this 025 list of conditions and the following disclaimer. 026 * Redistributions in binary form must reproduce the above copyright notice, 027 this list of conditions and the following disclaimer in the documentation 028 and/or other materials provided with the distribution. 029 * Neither the name of HL7 nor the names of its contributors may be used to 030 endorse or promote products derived from this software without specific 031 prior written permission. 032 033 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 034 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 035 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 036 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 037 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 038 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 039 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 040 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 041 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 042 POSSIBILITY OF SUCH DAMAGE. 043 044*/ 045// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 046public class Condition40_50 { 047 048 public static org.hl7.fhir.r5.model.Condition convertCondition(org.hl7.fhir.r4.model.Condition src) throws FHIRException { 049 if (src == null) 050 return null; 051 org.hl7.fhir.r5.model.Condition tgt = new org.hl7.fhir.r5.model.Condition(); 052 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 053 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 054 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 055 if (src.hasClinicalStatus()) 056 tgt.setClinicalStatus(CodeableConcept40_50.convertCodeableConcept(src.getClinicalStatus())); 057 if (src.hasVerificationStatus()) 058 tgt.setVerificationStatus(CodeableConcept40_50.convertCodeableConcept(src.getVerificationStatus())); 059 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 060 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 061 if (src.hasSeverity()) 062 tgt.setSeverity(CodeableConcept40_50.convertCodeableConcept(src.getSeverity())); 063 if (src.hasCode()) 064 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 065 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getBodySite()) 066 tgt.addBodySite(CodeableConcept40_50.convertCodeableConcept(t)); 067 if (src.hasSubject()) 068 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 069 if (src.hasEncounter()) 070 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 071 if (src.hasOnset()) 072 tgt.setOnset(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOnset())); 073 if (src.hasAbatement()) 074 tgt.setAbatement(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getAbatement())); 075 if (src.hasRecordedDate()) 076 tgt.setRecordedDateElement(DateTime40_50.convertDateTime(src.getRecordedDateElement())); 077 if (src.hasRecorder()) 078 tgt.addParticipant().setFunction(new CodeableConcept(new Coding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "author", "Author"))).setActor(Reference40_50.convertReference(src.getRecorder())); 079 if (src.hasAsserter()) 080 tgt.addParticipant().setFunction(new CodeableConcept(new Coding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "informant", "Informant"))).setActor(Reference40_50.convertReference(src.getAsserter())); 081 for (org.hl7.fhir.r4.model.Condition.ConditionStageComponent t : src.getStage()) 082 tgt.addStage(convertConditionStageComponent(t)); 083 for (org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent t : src.getEvidence()) 084 tgt.getEvidence().addAll(convertConditionEvidenceComponent(t)); 085 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 086 return tgt; 087 } 088 089 public static org.hl7.fhir.r4.model.Condition convertCondition(org.hl7.fhir.r5.model.Condition src) throws FHIRException { 090 if (src == null) 091 return null; 092 org.hl7.fhir.r4.model.Condition tgt = new org.hl7.fhir.r4.model.Condition(); 093 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 094 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 095 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 096 if (src.hasClinicalStatus()) 097 tgt.setClinicalStatus(CodeableConcept40_50.convertCodeableConcept(src.getClinicalStatus())); 098 if (src.hasVerificationStatus()) 099 tgt.setVerificationStatus(CodeableConcept40_50.convertCodeableConcept(src.getVerificationStatus())); 100 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 101 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 102 if (src.hasSeverity()) 103 tgt.setSeverity(CodeableConcept40_50.convertCodeableConcept(src.getSeverity())); 104 if (src.hasCode()) 105 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 106 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getBodySite()) 107 tgt.addBodySite(CodeableConcept40_50.convertCodeableConcept(t)); 108 if (src.hasSubject()) 109 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 110 if (src.hasEncounter()) 111 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 112 if (src.hasOnset()) 113 tgt.setOnset(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOnset())); 114 if (src.hasAbatement()) 115 tgt.setAbatement(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getAbatement())); 116 if (src.hasRecordedDate()) 117 tgt.setRecordedDateElement(DateTime40_50.convertDateTime(src.getRecordedDateElement())); 118 for (org.hl7.fhir.r5.model.Condition.ConditionParticipantComponent t : src.getParticipant()) { 119 if (t.getFunction().hasCoding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "author")) 120 tgt.setRecorder(Reference40_50.convertReference(t.getActor())); 121 if (t.getFunction().hasCoding("http://terminology.hl7.org/CodeSystem/provenance-participant-type", "informant")) 122 tgt.setAsserter(Reference40_50.convertReference(t.getActor())); 123 } 124 for (org.hl7.fhir.r5.model.Condition.ConditionStageComponent t : src.getStage()) 125 tgt.addStage(convertConditionStageComponent(t)); 126 for (CodeableReference t : src.getEvidence()) 127 tgt.addEvidence(convertConditionEvidenceComponent(t)); 128 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 129 return tgt; 130 } 131 132 public static org.hl7.fhir.r5.model.Condition.ConditionStageComponent convertConditionStageComponent(org.hl7.fhir.r4.model.Condition.ConditionStageComponent src) throws FHIRException { 133 if (src == null) 134 return null; 135 org.hl7.fhir.r5.model.Condition.ConditionStageComponent tgt = new org.hl7.fhir.r5.model.Condition.ConditionStageComponent(); 136 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 137 if (src.hasSummary()) 138 tgt.setSummary(CodeableConcept40_50.convertCodeableConcept(src.getSummary())); 139 for (org.hl7.fhir.r4.model.Reference t : src.getAssessment()) tgt.addAssessment(Reference40_50.convertReference(t)); 140 if (src.hasType()) 141 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 142 return tgt; 143 } 144 145 public static org.hl7.fhir.r4.model.Condition.ConditionStageComponent convertConditionStageComponent(org.hl7.fhir.r5.model.Condition.ConditionStageComponent src) throws FHIRException { 146 if (src == null) 147 return null; 148 org.hl7.fhir.r4.model.Condition.ConditionStageComponent tgt = new org.hl7.fhir.r4.model.Condition.ConditionStageComponent(); 149 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 150 if (src.hasSummary()) 151 tgt.setSummary(CodeableConcept40_50.convertCodeableConcept(src.getSummary())); 152 for (org.hl7.fhir.r5.model.Reference t : src.getAssessment()) tgt.addAssessment(Reference40_50.convertReference(t)); 153 if (src.hasType()) 154 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 155 return tgt; 156 } 157 158 public static List<org.hl7.fhir.r5.model.CodeableReference> convertConditionEvidenceComponent(org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent src) throws FHIRException { 159 if (src == null) 160 return null; 161 List<org.hl7.fhir.r5.model.CodeableReference> list = new ArrayList<>(); 162 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCode()) { 163 org.hl7.fhir.r5.model.CodeableReference tgt = new org.hl7.fhir.r5.model.CodeableReference(); 164 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 165 tgt.setConcept(CodeableConcept40_50.convertCodeableConcept(t)); 166 list.add(tgt); 167 } 168 for (org.hl7.fhir.r4.model.Reference t : src.getDetail()) { 169 org.hl7.fhir.r5.model.CodeableReference tgt = new org.hl7.fhir.r5.model.CodeableReference(); 170 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 171 tgt.setReference(Reference40_50.convertReference(t)); 172 list.add(tgt); 173 } 174 return list; 175 } 176 177 public static org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent convertConditionEvidenceComponent(org.hl7.fhir.r5.model.CodeableReference src) throws FHIRException { 178 if (src == null) 179 return null; 180 org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent tgt = new org.hl7.fhir.r4.model.Condition.ConditionEvidenceComponent(); 181 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 182 if (src.hasConcept()) 183 tgt.addCode(CodeableConcept40_50.convertCodeableConcept(src.getConcept())); 184 if (src.hasReference()) 185 tgt.addDetail(Reference40_50.convertReference(src.getReference())); 186 return tgt; 187 } 188 189}