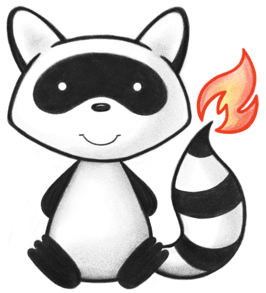
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Attachment40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Coding40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 012import org.hl7.fhir.exceptions.FHIRException; 013 014/* 015 Copyright (c) 2011+, HL7, Inc. 016 All rights reserved. 017 018 Redistribution and use in source and binary forms, with or without modification, 019 are permitted provided that the following conditions are met: 020 021 * Redistributions of source code must retain the above copyright notice, this 022 list of conditions and the following disclaimer. 023 * Redistributions in binary form must reproduce the above copyright notice, 024 this list of conditions and the following disclaimer in the documentation 025 and/or other materials provided with the distribution. 026 * Neither the name of HL7 nor the names of its contributors may be used to 027 endorse or promote products derived from this software without specific 028 prior written permission. 029 030 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 031 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 032 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 033 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 034 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 035 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 036 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 037 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 038 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 039 POSSIBILITY OF SUCH DAMAGE. 040 041*/ 042// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 043public class Consent40_50 { 044 045 public static org.hl7.fhir.r5.model.Consent convertConsent(org.hl7.fhir.r4.model.Consent src) throws FHIRException { 046 if (src == null) 047 return null; 048 org.hl7.fhir.r5.model.Consent tgt = new org.hl7.fhir.r5.model.Consent(); 049 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 050 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 051 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 052 if (src.hasStatus()) 053 tgt.setStatusElement(convertConsentState(src.getStatusElement())); 054// if (src.hasScope()) 055// tgt.setScope(CodeableConcept40_50.convertCodeableConcept(src.getScope())); 056 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 057 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 058 if (src.hasPatient()) 059 tgt.setSubject(Reference40_50.convertReference(src.getPatient())); 060 if (src.hasDateTime()) 061 tgt.setDateElement(DateTime40_50.convertDateTimeToDate(src.getDateTimeElement())); 062 for (org.hl7.fhir.r4.model.Reference t : src.getPerformer()) tgt.addGrantee(Reference40_50.convertReference(t)); 063 for (org.hl7.fhir.r4.model.Reference t : src.getOrganization()) tgt.addManager(Reference40_50.convertReference(t)); 064 if (src.hasSourceAttachment()) 065 tgt.addSourceAttachment(Attachment40_50.convertAttachment(src.getSourceAttachment())); 066 if (src.hasSourceReference()) 067 tgt.addSourceReference(Reference40_50.convertReference(src.getSourceReference())); 068// for (org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent t : src.getPolicy()) 069// tgt.addPolicy(convertConsentPolicyComponent(t)); 070// if (src.hasPolicyRule()) 071// tgt.setPolicyRule(CodeableConcept40_50.convertCodeableConcept(src.getPolicyRule())); 072 for (org.hl7.fhir.r4.model.Consent.ConsentVerificationComponent t : src.getVerification()) 073 tgt.addVerification(convertConsentVerificationComponent(t)); 074 if (src.hasProvision()) 075 tgt.addProvision(convertprovisionComponent(src.getProvision())); 076 return tgt; 077 } 078 079 public static org.hl7.fhir.r4.model.Consent convertConsent(org.hl7.fhir.r5.model.Consent src) throws FHIRException { 080 if (src == null) 081 return null; 082 org.hl7.fhir.r4.model.Consent tgt = new org.hl7.fhir.r4.model.Consent(); 083 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 084 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 085 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 086 if (src.hasStatus()) 087 tgt.setStatusElement(convertConsentState(src.getStatusElement())); 088// if (src.hasScope()) 089// tgt.setScope(CodeableConcept40_50.convertCodeableConcept(src.getScope())); 090 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 091 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 092 if (src.hasSubject()) 093 tgt.setPatient(Reference40_50.convertReference(src.getSubject())); 094 if (src.hasDate()) 095 tgt.setDateTimeElement(DateTime40_50.convertDateToDateTime(src.getDateElement())); 096 for (org.hl7.fhir.r5.model.Reference t : src.getGrantee()) tgt.addPerformer(Reference40_50.convertReference(t)); 097 for (org.hl7.fhir.r5.model.Reference t : src.getManager()) tgt.addOrganization(Reference40_50.convertReference(t)); 098 for (org.hl7.fhir.r5.model.Reference t : src.getController()) 099 tgt.addOrganization(Reference40_50.convertReference(t)); 100 if (src.hasSourceAttachment()) 101 tgt.setSource(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getSourceAttachmentFirstRep())); 102 if (src.hasSourceReference()) 103 tgt.setSource(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getSourceReferenceFirstRep())); 104// for (org.hl7.fhir.r5.model.Consent.ConsentPolicyComponent t : src.getPolicy()) 105// tgt.addPolicy(convertConsentPolicyComponent(t)); 106// if (src.hasPolicyRule()) 107// tgt.setPolicyRule(CodeableConcept40_50.convertCodeableConcept(src.getPolicyRule())); 108 for (org.hl7.fhir.r5.model.Consent.ConsentVerificationComponent t : src.getVerification()) 109 tgt.addVerification(convertConsentVerificationComponent(t)); 110 if (src.hasProvision()) 111 tgt.setProvision(convertprovisionComponent(src.getProvisionFirstRep())); 112 return tgt; 113 } 114 115 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Consent.ConsentState> convertConsentState(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentState> src) throws FHIRException { 116 if (src == null || src.isEmpty()) 117 return null; 118 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Consent.ConsentState> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Consent.ConsentStateEnumFactory()); 119 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 120 switch (src.getValue()) { 121 case DRAFT: 122 tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentState.DRAFT); 123 break; 124 case PROPOSED: 125 tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentState.DRAFT); 126 break; 127 case ACTIVE: 128 tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentState.ACTIVE); 129 break; 130 case REJECTED: 131 tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentState.INACTIVE); 132 break; 133 case INACTIVE: 134 tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentState.INACTIVE); 135 break; 136 case ENTEREDINERROR: 137 tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentState.ENTEREDINERROR); 138 break; 139 default: 140 tgt.setValue(org.hl7.fhir.r5.model.Consent.ConsentState.NULL); 141 break; 142 } 143 return tgt; 144 } 145 146 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentState> convertConsentState(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Consent.ConsentState> src) throws FHIRException { 147 if (src == null || src.isEmpty()) 148 return null; 149 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentState> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Consent.ConsentStateEnumFactory()); 150 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 151 switch (src.getValue()) { 152 case DRAFT: 153 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.DRAFT); 154 break; 155 case ACTIVE: 156 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.ACTIVE); 157 break; 158 case INACTIVE: 159 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.INACTIVE); 160 break; 161 case ENTEREDINERROR: 162 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.ENTEREDINERROR); 163 break; 164 default: 165 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentState.NULL); 166 break; 167 } 168 return tgt; 169 } 170 171// public static org.hl7.fhir.r5.model.Consent.ConsentPolicyComponent convertConsentPolicyComponent(org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent src) throws FHIRException { 172// if (src == null) 173// return null; 174// org.hl7.fhir.r5.model.Consent.ConsentPolicyComponent tgt = new org.hl7.fhir.r5.model.Consent.ConsentPolicyComponent(); 175// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 176// if (src.hasAuthority()) 177// tgt.setAuthorityElement(Uri40_50.convertUri(src.getAuthorityElement())); 178// if (src.hasUri()) 179// tgt.setUriElement(Uri40_50.convertUri(src.getUriElement())); 180// return tgt; 181// } 182// 183// public static org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent convertConsentPolicyComponent(org.hl7.fhir.r5.model.Consent.ConsentPolicyComponent src) throws FHIRException { 184// if (src == null) 185// return null; 186// org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent tgt = new org.hl7.fhir.r4.model.Consent.ConsentPolicyComponent(); 187// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 188// if (src.hasAuthority()) 189// tgt.setAuthorityElement(Uri40_50.convertUri(src.getAuthorityElement())); 190// if (src.hasUri()) 191// tgt.setUriElement(Uri40_50.convertUri(src.getUriElement())); 192// return tgt; 193// } 194 195 public static org.hl7.fhir.r5.model.Consent.ConsentVerificationComponent convertConsentVerificationComponent(org.hl7.fhir.r4.model.Consent.ConsentVerificationComponent src) throws FHIRException { 196 if (src == null) 197 return null; 198 org.hl7.fhir.r5.model.Consent.ConsentVerificationComponent tgt = new org.hl7.fhir.r5.model.Consent.ConsentVerificationComponent(); 199 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 200 if (src.hasVerified()) 201 tgt.setVerifiedElement(Boolean40_50.convertBoolean(src.getVerifiedElement())); 202 if (src.hasVerifiedWith()) 203 tgt.setVerifiedWith(Reference40_50.convertReference(src.getVerifiedWith())); 204 if (src.hasVerificationDate()) 205 tgt.getVerificationDate().add(DateTime40_50.convertDateTime(src.getVerificationDateElement())); 206 return tgt; 207 } 208 209 public static org.hl7.fhir.r4.model.Consent.ConsentVerificationComponent convertConsentVerificationComponent(org.hl7.fhir.r5.model.Consent.ConsentVerificationComponent src) throws FHIRException { 210 if (src == null) 211 return null; 212 org.hl7.fhir.r4.model.Consent.ConsentVerificationComponent tgt = new org.hl7.fhir.r4.model.Consent.ConsentVerificationComponent(); 213 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 214 if (src.hasVerified()) 215 tgt.setVerifiedElement(Boolean40_50.convertBoolean(src.getVerifiedElement())); 216 if (src.hasVerifiedWith()) 217 tgt.setVerifiedWith(Reference40_50.convertReference(src.getVerifiedWith())); 218 if (src.hasVerificationDate()) 219 tgt.setVerificationDateElement(DateTime40_50.convertDateTime(src.getVerificationDate().get(0))); 220 return tgt; 221 } 222 223 public static org.hl7.fhir.r5.model.Consent.ProvisionComponent convertprovisionComponent(org.hl7.fhir.r4.model.Consent.provisionComponent src) throws FHIRException { 224 if (src == null) 225 return null; 226 org.hl7.fhir.r5.model.Consent.ProvisionComponent tgt = new org.hl7.fhir.r5.model.Consent.ProvisionComponent(); 227 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 228// if (src.hasType()) 229// tgt.setTypeElement(convertConsentProvisionType(src.getTypeElement())); 230 if (src.hasPeriod()) 231 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 232 for (org.hl7.fhir.r4.model.Consent.provisionActorComponent t : src.getActor()) 233 tgt.addActor(convertprovisionActorComponent(t)); 234 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getAction()) 235 tgt.addAction(CodeableConcept40_50.convertCodeableConcept(t)); 236 for (org.hl7.fhir.r4.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding40_50.convertCoding(t)); 237 for (org.hl7.fhir.r4.model.Coding t : src.getPurpose()) tgt.addPurpose(Coding40_50.convertCoding(t)); 238// for (org.hl7.fhir.r4.model.Coding t : src.getClass_()) tgt.addClass_(Coding40_50.convertCoding(t)); 239 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCode()) 240 tgt.addCode(CodeableConcept40_50.convertCodeableConcept(t)); 241 if (src.hasDataPeriod()) 242 tgt.setDataPeriod(Period40_50.convertPeriod(src.getDataPeriod())); 243 for (org.hl7.fhir.r4.model.Consent.provisionDataComponent t : src.getData()) 244 tgt.addData(convertprovisionDataComponent(t)); 245 for (org.hl7.fhir.r4.model.Consent.provisionComponent t : src.getProvision()) 246 tgt.addProvision(convertprovisionComponent(t)); 247 return tgt; 248 } 249 250 public static org.hl7.fhir.r4.model.Consent.provisionComponent convertprovisionComponent(org.hl7.fhir.r5.model.Consent.ProvisionComponent src) throws FHIRException { 251 if (src == null) 252 return null; 253 org.hl7.fhir.r4.model.Consent.provisionComponent tgt = new org.hl7.fhir.r4.model.Consent.provisionComponent(); 254 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 255// if (src.hasType()) 256// tgt.setTypeElement(convertConsentProvisionType(src.getTypeElement())); 257 if (src.hasPeriod()) 258 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 259 for (org.hl7.fhir.r5.model.Consent.ProvisionActorComponent t : src.getActor()) 260 tgt.addActor(convertprovisionActorComponent(t)); 261 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getAction()) 262 tgt.addAction(CodeableConcept40_50.convertCodeableConcept(t)); 263 for (org.hl7.fhir.r5.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding40_50.convertCoding(t)); 264 for (org.hl7.fhir.r5.model.Coding t : src.getPurpose()) tgt.addPurpose(Coding40_50.convertCoding(t)); 265// for (org.hl7.fhir.r5.model.Coding t : src.getClass_()) tgt.addClass_(Coding40_50.convertCoding(t)); 266 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCode()) 267 tgt.addCode(CodeableConcept40_50.convertCodeableConcept(t)); 268 if (src.hasDataPeriod()) 269 tgt.setDataPeriod(Period40_50.convertPeriod(src.getDataPeriod())); 270 for (org.hl7.fhir.r5.model.Consent.ProvisionDataComponent t : src.getData()) 271 tgt.addData(convertprovisionDataComponent(t)); 272 for (org.hl7.fhir.r5.model.Consent.ProvisionComponent t : src.getProvision()) 273 tgt.addProvision(convertprovisionComponent(t)); 274 return tgt; 275 } 276 277 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ConsentProvisionType> convertConsentProvisionType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentProvisionType> src) throws FHIRException { 278 if (src == null || src.isEmpty()) 279 return null; 280 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ConsentProvisionType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ConsentProvisionTypeEnumFactory()); 281 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 282 switch (src.getValue()) { 283 case DENY: 284 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConsentProvisionType.DENY); 285 break; 286 case PERMIT: 287 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConsentProvisionType.PERMIT); 288 break; 289 default: 290 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConsentProvisionType.NULL); 291 break; 292 } 293 return tgt; 294 } 295 296 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentProvisionType> convertConsentProvisionType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ConsentProvisionType> src) throws FHIRException { 297 if (src == null || src.isEmpty()) 298 return null; 299 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentProvisionType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Consent.ConsentProvisionTypeEnumFactory()); 300 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 301 switch (src.getValue()) { 302 case DENY: 303 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentProvisionType.DENY); 304 break; 305 case PERMIT: 306 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentProvisionType.PERMIT); 307 break; 308 default: 309 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentProvisionType.NULL); 310 break; 311 } 312 return tgt; 313 } 314 315 public static org.hl7.fhir.r5.model.Consent.ProvisionActorComponent convertprovisionActorComponent(org.hl7.fhir.r4.model.Consent.provisionActorComponent src) throws FHIRException { 316 if (src == null) 317 return null; 318 org.hl7.fhir.r5.model.Consent.ProvisionActorComponent tgt = new org.hl7.fhir.r5.model.Consent.ProvisionActorComponent(); 319 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 320 if (src.hasRole()) 321 tgt.setRole(CodeableConcept40_50.convertCodeableConcept(src.getRole())); 322 if (src.hasReference()) 323 tgt.setReference(Reference40_50.convertReference(src.getReference())); 324 return tgt; 325 } 326 327 public static org.hl7.fhir.r4.model.Consent.provisionActorComponent convertprovisionActorComponent(org.hl7.fhir.r5.model.Consent.ProvisionActorComponent src) throws FHIRException { 328 if (src == null) 329 return null; 330 org.hl7.fhir.r4.model.Consent.provisionActorComponent tgt = new org.hl7.fhir.r4.model.Consent.provisionActorComponent(); 331 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 332 if (src.hasRole()) 333 tgt.setRole(CodeableConcept40_50.convertCodeableConcept(src.getRole())); 334 if (src.hasReference()) 335 tgt.setReference(Reference40_50.convertReference(src.getReference())); 336 return tgt; 337 } 338 339 public static org.hl7.fhir.r5.model.Consent.ProvisionDataComponent convertprovisionDataComponent(org.hl7.fhir.r4.model.Consent.provisionDataComponent src) throws FHIRException { 340 if (src == null) 341 return null; 342 org.hl7.fhir.r5.model.Consent.ProvisionDataComponent tgt = new org.hl7.fhir.r5.model.Consent.ProvisionDataComponent(); 343 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 344 if (src.hasMeaning()) 345 tgt.setMeaningElement(convertConsentDataMeaning(src.getMeaningElement())); 346 if (src.hasReference()) 347 tgt.setReference(Reference40_50.convertReference(src.getReference())); 348 return tgt; 349 } 350 351 public static org.hl7.fhir.r4.model.Consent.provisionDataComponent convertprovisionDataComponent(org.hl7.fhir.r5.model.Consent.ProvisionDataComponent src) throws FHIRException { 352 if (src == null) 353 return null; 354 org.hl7.fhir.r4.model.Consent.provisionDataComponent tgt = new org.hl7.fhir.r4.model.Consent.provisionDataComponent(); 355 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 356 if (src.hasMeaning()) 357 tgt.setMeaningElement(convertConsentDataMeaning(src.getMeaningElement())); 358 if (src.hasReference()) 359 tgt.setReference(Reference40_50.convertReference(src.getReference())); 360 return tgt; 361 } 362 363 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaning> convertConsentDataMeaning(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentDataMeaning> src) throws FHIRException { 364 if (src == null || src.isEmpty()) 365 return null; 366 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaning> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaningEnumFactory()); 367 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 368 switch (src.getValue()) { 369 case INSTANCE: 370 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaning.INSTANCE); 371 break; 372 case RELATED: 373 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaning.RELATED); 374 break; 375 case DEPENDENTS: 376 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaning.DEPENDENTS); 377 break; 378 case AUTHOREDBY: 379 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaning.AUTHOREDBY); 380 break; 381 default: 382 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaning.NULL); 383 break; 384 } 385 return tgt; 386 } 387 388 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentDataMeaning> convertConsentDataMeaning(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ConsentDataMeaning> src) throws FHIRException { 389 if (src == null || src.isEmpty()) 390 return null; 391 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Consent.ConsentDataMeaning> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Consent.ConsentDataMeaningEnumFactory()); 392 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 393 switch (src.getValue()) { 394 case INSTANCE: 395 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.INSTANCE); 396 break; 397 case RELATED: 398 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.RELATED); 399 break; 400 case DEPENDENTS: 401 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.DEPENDENTS); 402 break; 403 case AUTHOREDBY: 404 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.AUTHOREDBY); 405 break; 406 default: 407 tgt.setValue(org.hl7.fhir.r4.model.Consent.ConsentDataMeaning.NULL); 408 break; 409 } 410 return tgt; 411 } 412}