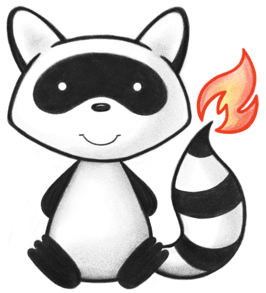
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Annotation40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r4.model.DeviceRequest; 013import org.hl7.fhir.r5.model.CodeableReference; 014import org.hl7.fhir.r5.model.Enumeration; 015import org.hl7.fhir.r5.model.Enumerations; 016 017/* 018 Copyright (c) 2011+, HL7, Inc. 019 All rights reserved. 020 021 Redistribution and use in source and binary forms, with or without modification, 022 are permitted provided that the following conditions are met: 023 024 * Redistributions of source code must retain the above copyright notice, this 025 list of conditions and the following disclaimer. 026 * Redistributions in binary form must reproduce the above copyright notice, 027 this list of conditions and the following disclaimer in the documentation 028 and/or other materials provided with the distribution. 029 * Neither the name of HL7 nor the names of its contributors may be used to 030 endorse or promote products derived from this software without specific 031 prior written permission. 032 033 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 034 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 035 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 036 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 037 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 038 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 039 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 040 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 041 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 042 POSSIBILITY OF SUCH DAMAGE. 043 044*/ 045// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 046public class DeviceRequest40_50 { 047 048 public static org.hl7.fhir.r5.model.DeviceRequest convertDeviceRequest(org.hl7.fhir.r4.model.DeviceRequest src) throws FHIRException { 049 if (src == null) 050 return null; 051 org.hl7.fhir.r5.model.DeviceRequest tgt = new org.hl7.fhir.r5.model.DeviceRequest(); 052 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 053 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 054 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 055 for (org.hl7.fhir.r4.model.CanonicalType t : src.getInstantiatesCanonical()) 056 tgt.getInstantiatesCanonical().add(Canonical40_50.convertCanonical(t)); 057 for (org.hl7.fhir.r4.model.UriType t : src.getInstantiatesUri()) 058 tgt.getInstantiatesUri().add(Uri40_50.convertUri(t)); 059 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 060// for (org.hl7.fhir.r4.model.Reference t : src.getPriorRequest()) 061// tgt.addPriorRequest(Reference40_50.convertReference(t)); 062 if (src.hasGroupIdentifier()) 063 tgt.setGroupIdentifier(Identifier40_50.convertIdentifier(src.getGroupIdentifier())); 064 if (src.hasStatus()) 065 tgt.setStatusElement(convertDeviceRequestStatus(src.getStatusElement())); 066 if (src.hasIntent()) 067 tgt.setIntentElement(convertRequestIntent(src.getIntentElement())); 068 if (src.hasPriority()) 069 tgt.setPriorityElement(convertRequestPriority(src.getPriorityElement())); 070 if (src.hasCodeCodeableConcept()) 071 tgt.getCode().setConcept(CodeableConcept40_50.convertCodeableConcept(src.getCodeCodeableConcept())); 072 else if (src.hasCodeReference()) 073 tgt.getCode().setReference(Reference40_50.convertReference(src.getCodeReference())); 074 075 for (org.hl7.fhir.r4.model.DeviceRequest.DeviceRequestParameterComponent t : src.getParameter()) 076 tgt.addParameter(convertDeviceRequestParameterComponent(t)); 077 if (src.hasSubject()) 078 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 079 if (src.hasEncounter()) 080 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 081 if (src.hasOccurrence()) 082 tgt.setOccurrence(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOccurrence())); 083 if (src.hasAuthoredOn()) 084 tgt.setAuthoredOnElement(DateTime40_50.convertDateTime(src.getAuthoredOnElement())); 085 if (src.hasRequester()) 086 tgt.setRequester(Reference40_50.convertReference(src.getRequester())); 087 if (src.hasPerformerType()) 088 tgt.getPerformer().setConcept(CodeableConcept40_50.convertCodeableConcept(src.getPerformerType())); 089 if (src.hasPerformer()) 090 tgt.getPerformer().setReference(Reference40_50.convertReference(src.getPerformer())); 091 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 092 tgt.addReason(CodeableConcept40_50.convertCodeableConceptToCodeableReference(t)); 093 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 094 tgt.addReason(Reference40_50.convertReferenceToCodeableReference(t)); 095 for (org.hl7.fhir.r4.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference40_50.convertReference(t)); 096 for (org.hl7.fhir.r4.model.Reference t : src.getSupportingInfo()) 097 tgt.addSupportingInfo(Reference40_50.convertReference(t)); 098 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 099 for (org.hl7.fhir.r4.model.Reference t : src.getRelevantHistory()) 100 tgt.addRelevantHistory(Reference40_50.convertReference(t)); 101 return tgt; 102 } 103 104 public static org.hl7.fhir.r4.model.DeviceRequest convertDeviceRequest(org.hl7.fhir.r5.model.DeviceRequest src) throws FHIRException { 105 if (src == null) 106 return null; 107 org.hl7.fhir.r4.model.DeviceRequest tgt = new org.hl7.fhir.r4.model.DeviceRequest(); 108 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 109 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 110 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 111 for (org.hl7.fhir.r5.model.CanonicalType t : src.getInstantiatesCanonical()) 112 tgt.getInstantiatesCanonical().add(Canonical40_50.convertCanonical(t)); 113 for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesUri()) 114 tgt.getInstantiatesUri().add(Uri40_50.convertUri(t)); 115 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 116// for (org.hl7.fhir.r5.model.Reference t : src.getPriorRequest()) 117// tgt.addPriorRequest(Reference40_50.convertReference(t)); 118 if (src.hasGroupIdentifier()) 119 tgt.setGroupIdentifier(Identifier40_50.convertIdentifier(src.getGroupIdentifier())); 120 if (src.hasStatus()) 121 tgt.setStatusElement(convertDeviceRequestStatus(src.getStatusElement())); 122 if (src.hasIntent()) 123 tgt.setIntentElement(convertRequestIntent(src.getIntentElement())); 124 if (src.hasPriority()) 125 tgt.setPriorityElement(convertRequestPriority(src.getPriorityElement())); 126 if (src.getCode().hasConcept()) 127 tgt.setCode(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getCode().getConcept())); 128 if (src.getCode().hasReference()) 129 tgt.setCode(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getCode().getReference())); 130 for (org.hl7.fhir.r5.model.DeviceRequest.DeviceRequestParameterComponent t : src.getParameter()) 131 tgt.addParameter(convertDeviceRequestParameterComponent(t)); 132 if (src.hasSubject()) 133 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 134 if (src.hasEncounter()) 135 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 136 if (src.hasOccurrence()) 137 tgt.setOccurrence(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOccurrence())); 138 if (src.hasAuthoredOn()) 139 tgt.setAuthoredOnElement(DateTime40_50.convertDateTime(src.getAuthoredOnElement())); 140 if (src.hasRequester()) 141 tgt.setRequester(Reference40_50.convertReference(src.getRequester())); 142 if (src.getPerformer().hasConcept()) 143 tgt.setPerformerType(CodeableConcept40_50.convertCodeableConcept(src.getPerformer().getConcept())); 144 if (src.getPerformer().hasReference()) 145 tgt.setPerformer(Reference40_50.convertReference(src.getPerformer().getReference())); 146 for (CodeableReference t : src.getReason()) 147 if (t.hasConcept()) 148 tgt.addReasonCode(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 149 for (CodeableReference t : src.getReason()) 150 if (t.hasReference()) 151 tgt.addReasonReference(Reference40_50.convertReference(t.getReference())); 152 for (org.hl7.fhir.r5.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference40_50.convertReference(t)); 153 for (org.hl7.fhir.r5.model.Reference t : src.getSupportingInfo()) 154 tgt.addSupportingInfo(Reference40_50.convertReference(t)); 155 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 156 for (org.hl7.fhir.r5.model.Reference t : src.getRelevantHistory()) 157 tgt.addRelevantHistory(Reference40_50.convertReference(t)); 158 return tgt; 159 } 160 161 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> convertDeviceRequestStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DeviceRequest.DeviceRequestStatus> src) throws FHIRException { 162 if (src == null || src.isEmpty()) 163 return null; 164 Enumeration<Enumerations.RequestStatus> tgt = new Enumeration<>(new Enumerations.RequestStatusEnumFactory()); 165 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 166 if (src.getValue() == null) { 167 tgt.setValue(null); 168 } else { 169 switch (src.getValue()) { 170 case DRAFT: 171 tgt.setValue(Enumerations.RequestStatus.DRAFT); 172 break; 173 case ACTIVE: 174 tgt.setValue(Enumerations.RequestStatus.ACTIVE); 175 break; 176 case ONHOLD: 177 tgt.setValue(Enumerations.RequestStatus.ONHOLD); 178 break; 179 case REVOKED: 180 tgt.setValue(Enumerations.RequestStatus.REVOKED); 181 break; 182 case COMPLETED: 183 tgt.setValue(Enumerations.RequestStatus.COMPLETED); 184 break; 185 case ENTEREDINERROR: 186 tgt.setValue(Enumerations.RequestStatus.ENTEREDINERROR); 187 break; 188 case UNKNOWN: 189 tgt.setValue(Enumerations.RequestStatus.UNKNOWN); 190 break; 191 default: 192 tgt.setValue(Enumerations.RequestStatus.NULL); 193 break; 194 } 195 } 196 return tgt; 197 } 198 199 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DeviceRequest.DeviceRequestStatus> convertDeviceRequestStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> src) throws FHIRException { 200 if (src == null || src.isEmpty()) 201 return null; 202 org.hl7.fhir.r4.model.Enumeration<DeviceRequest.DeviceRequestStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new DeviceRequest.DeviceRequestStatusEnumFactory()); 203 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 204 if (src.getValue() == null) { 205 tgt.setValue(null); 206 } else { 207 switch (src.getValue()) { 208 case DRAFT: 209 tgt.setValue(DeviceRequest.DeviceRequestStatus.DRAFT); 210 break; 211 case ACTIVE: 212 tgt.setValue(DeviceRequest.DeviceRequestStatus.ACTIVE); 213 break; 214 case ONHOLD: 215 tgt.setValue(DeviceRequest.DeviceRequestStatus.ONHOLD); 216 break; 217 case REVOKED: 218 tgt.setValue(DeviceRequest.DeviceRequestStatus.REVOKED); 219 break; 220 case COMPLETED: 221 tgt.setValue(DeviceRequest.DeviceRequestStatus.COMPLETED); 222 break; 223 case ENTEREDINERROR: 224 tgt.setValue(DeviceRequest.DeviceRequestStatus.ENTEREDINERROR); 225 break; 226 case UNKNOWN: 227 tgt.setValue(DeviceRequest.DeviceRequestStatus.UNKNOWN); 228 break; 229 default: 230 tgt.setValue(DeviceRequest.DeviceRequestStatus.NULL); 231 break; 232 } 233 } 234 return tgt; 235 } 236 237 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestIntent> convertRequestIntent(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DeviceRequest.RequestIntent> src) throws FHIRException { 238 if (src == null || src.isEmpty()) 239 return null; 240 Enumeration<Enumerations.RequestIntent> tgt = new Enumeration<>(new Enumerations.RequestIntentEnumFactory()); 241 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 242 if (src.getValue() == null) { 243 tgt.setValue(null); 244 } else { 245 switch (src.getValue()) { 246 case PROPOSAL: 247 tgt.setValue(Enumerations.RequestIntent.PROPOSAL); 248 break; 249 case PLAN: 250 tgt.setValue(Enumerations.RequestIntent.PLAN); 251 break; 252 case DIRECTIVE: 253 tgt.setValue(Enumerations.RequestIntent.DIRECTIVE); 254 break; 255 case ORDER: 256 tgt.setValue(Enumerations.RequestIntent.ORDER); 257 break; 258 case ORIGINALORDER: 259 tgt.setValue(Enumerations.RequestIntent.ORIGINALORDER); 260 break; 261 case REFLEXORDER: 262 tgt.setValue(Enumerations.RequestIntent.REFLEXORDER); 263 break; 264 case FILLERORDER: 265 tgt.setValue(Enumerations.RequestIntent.FILLERORDER); 266 break; 267 case INSTANCEORDER: 268 tgt.setValue(Enumerations.RequestIntent.INSTANCEORDER); 269 break; 270 case OPTION: 271 tgt.setValue(Enumerations.RequestIntent.OPTION); 272 break; 273 default: 274 tgt.setValue(Enumerations.RequestIntent.NULL); 275 break; 276 } 277 } 278 return tgt; 279 } 280 281 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DeviceRequest.RequestIntent> convertRequestIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestIntent> src) throws FHIRException { 282 if (src == null || src.isEmpty()) 283 return null; 284 org.hl7.fhir.r4.model.Enumeration<DeviceRequest.RequestIntent> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new DeviceRequest.RequestIntentEnumFactory()); 285 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 286 if (src.getValue() == null) { 287 tgt.setValue(null); 288 } else { 289 switch (src.getValue()) { 290 case PROPOSAL: 291 tgt.setValue(DeviceRequest.RequestIntent.PROPOSAL); 292 break; 293 case PLAN: 294 tgt.setValue(DeviceRequest.RequestIntent.PLAN); 295 break; 296 case DIRECTIVE: 297 tgt.setValue(DeviceRequest.RequestIntent.DIRECTIVE); 298 break; 299 case ORDER: 300 tgt.setValue(DeviceRequest.RequestIntent.ORDER); 301 break; 302 case ORIGINALORDER: 303 tgt.setValue(DeviceRequest.RequestIntent.ORIGINALORDER); 304 break; 305 case REFLEXORDER: 306 tgt.setValue(DeviceRequest.RequestIntent.REFLEXORDER); 307 break; 308 case FILLERORDER: 309 tgt.setValue(DeviceRequest.RequestIntent.FILLERORDER); 310 break; 311 case INSTANCEORDER: 312 tgt.setValue(DeviceRequest.RequestIntent.INSTANCEORDER); 313 break; 314 case OPTION: 315 tgt.setValue(DeviceRequest.RequestIntent.OPTION); 316 break; 317 default: 318 tgt.setValue(DeviceRequest.RequestIntent.NULL); 319 break; 320 } 321 } 322 return tgt; 323 } 324 325 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertRequestPriority(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DeviceRequest.RequestPriority> src) throws FHIRException { 326 if (src == null || src.isEmpty()) 327 return null; 328 Enumeration<Enumerations.RequestPriority> tgt = new Enumeration<>(new Enumerations.RequestPriorityEnumFactory()); 329 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 330 if (src.getValue() == null) { 331 tgt.setValue(null); 332 } else { 333 switch (src.getValue()) { 334 case ROUTINE: 335 tgt.setValue(Enumerations.RequestPriority.ROUTINE); 336 break; 337 case URGENT: 338 tgt.setValue(Enumerations.RequestPriority.URGENT); 339 break; 340 case ASAP: 341 tgt.setValue(Enumerations.RequestPriority.ASAP); 342 break; 343 case STAT: 344 tgt.setValue(Enumerations.RequestPriority.STAT); 345 break; 346 default: 347 tgt.setValue(Enumerations.RequestPriority.NULL); 348 break; 349 } 350 } 351 return tgt; 352 } 353 354 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DeviceRequest.RequestPriority> convertRequestPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 355 if (src == null || src.isEmpty()) 356 return null; 357 org.hl7.fhir.r4.model.Enumeration<DeviceRequest.RequestPriority> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new DeviceRequest.RequestPriorityEnumFactory()); 358 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 359 if (src.getValue() == null) { 360 tgt.setValue(null); 361 } else { 362 switch (src.getValue()) { 363 case ROUTINE: 364 tgt.setValue(DeviceRequest.RequestPriority.ROUTINE); 365 break; 366 case URGENT: 367 tgt.setValue(DeviceRequest.RequestPriority.URGENT); 368 break; 369 case ASAP: 370 tgt.setValue(DeviceRequest.RequestPriority.ASAP); 371 break; 372 case STAT: 373 tgt.setValue(DeviceRequest.RequestPriority.STAT); 374 break; 375 default: 376 tgt.setValue(DeviceRequest.RequestPriority.NULL); 377 break; 378 } 379 } 380 return tgt; 381 } 382 383 public static org.hl7.fhir.r5.model.DeviceRequest.DeviceRequestParameterComponent convertDeviceRequestParameterComponent(org.hl7.fhir.r4.model.DeviceRequest.DeviceRequestParameterComponent src) throws FHIRException { 384 if (src == null) 385 return null; 386 org.hl7.fhir.r5.model.DeviceRequest.DeviceRequestParameterComponent tgt = new org.hl7.fhir.r5.model.DeviceRequest.DeviceRequestParameterComponent(); 387 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 388 if (src.hasCode()) 389 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 390 if (src.hasValue()) 391 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 392 return tgt; 393 } 394 395 public static org.hl7.fhir.r4.model.DeviceRequest.DeviceRequestParameterComponent convertDeviceRequestParameterComponent(org.hl7.fhir.r5.model.DeviceRequest.DeviceRequestParameterComponent src) throws FHIRException { 396 if (src == null) 397 return null; 398 org.hl7.fhir.r4.model.DeviceRequest.DeviceRequestParameterComponent tgt = new org.hl7.fhir.r4.model.DeviceRequest.DeviceRequestParameterComponent(); 399 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 400 if (src.hasCode()) 401 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 402 if (src.hasValue()) 403 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 404 return tgt; 405 } 406}