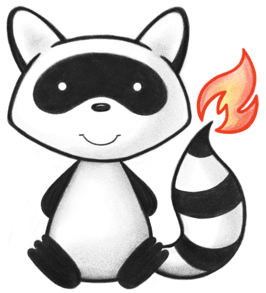
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Attachment40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Instant40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.DiagnosticReport; 012import org.hl7.fhir.r5.model.Enumeration; 013 014/* 015 Copyright (c) 2011+, HL7, Inc. 016 All rights reserved. 017 018 Redistribution and use in source and binary forms, with or without modification, 019 are permitted provided that the following conditions are met: 020 021 * Redistributions of source code must retain the above copyright notice, this 022 list of conditions and the following disclaimer. 023 * Redistributions in binary form must reproduce the above copyright notice, 024 this list of conditions and the following disclaimer in the documentation 025 and/or other materials provided with the distribution. 026 * Neither the name of HL7 nor the names of its contributors may be used to 027 endorse or promote products derived from this software without specific 028 prior written permission. 029 030 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 031 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 032 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 033 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 034 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 035 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 036 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 037 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 038 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 039 POSSIBILITY OF SUCH DAMAGE. 040 041*/ 042// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 043public class DiagnosticReport40_50 { 044 045 public static org.hl7.fhir.r5.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.r4.model.DiagnosticReport src) throws FHIRException { 046 if (src == null) 047 return null; 048 org.hl7.fhir.r5.model.DiagnosticReport tgt = new org.hl7.fhir.r5.model.DiagnosticReport(); 049 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 050 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 051 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 052 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 055 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 056 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 057 if (src.hasCode()) 058 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 059 if (src.hasSubject()) 060 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 061 if (src.hasEncounter()) 062 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 063 if (src.hasEffective()) 064 tgt.setEffective(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getEffective())); 065 if (src.hasIssued()) 066 tgt.setIssuedElement(Instant40_50.convertInstant(src.getIssuedElement())); 067 for (org.hl7.fhir.r4.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference40_50.convertReference(t)); 068 for (org.hl7.fhir.r4.model.Reference t : src.getResultsInterpreter()) 069 tgt.addResultsInterpreter(Reference40_50.convertReference(t)); 070 for (org.hl7.fhir.r4.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference40_50.convertReference(t)); 071 for (org.hl7.fhir.r4.model.Reference t : src.getResult()) tgt.addResult(Reference40_50.convertReference(t)); 072 for (org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent t : src.getMedia()) 073 tgt.addMedia(convertDiagnosticReportMediaComponent(t)); 074 if (src.hasConclusion()) 075 tgt.setConclusionElement(String40_50.convertStringToMarkdown(src.getConclusionElement())); 076 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getConclusionCode()) 077 tgt.addConclusionCode(CodeableConcept40_50.convertCodeableConcept(t)); 078 for (org.hl7.fhir.r4.model.Attachment t : src.getPresentedForm()) 079 tgt.addPresentedForm(Attachment40_50.convertAttachment(t)); 080 return tgt; 081 } 082 083 public static org.hl7.fhir.r4.model.DiagnosticReport convertDiagnosticReport(org.hl7.fhir.r5.model.DiagnosticReport src) throws FHIRException { 084 if (src == null) 085 return null; 086 org.hl7.fhir.r4.model.DiagnosticReport tgt = new org.hl7.fhir.r4.model.DiagnosticReport(); 087 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 088 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 089 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 090 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 091 if (src.hasStatus()) 092 tgt.setStatusElement(convertDiagnosticReportStatus(src.getStatusElement())); 093 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 094 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 095 if (src.hasCode()) 096 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 097 if (src.hasSubject()) 098 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 099 if (src.hasEncounter()) 100 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 101 if (src.hasEffective()) 102 tgt.setEffective(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getEffective())); 103 if (src.hasIssued()) 104 tgt.setIssuedElement(Instant40_50.convertInstant(src.getIssuedElement())); 105 for (org.hl7.fhir.r5.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference40_50.convertReference(t)); 106 for (org.hl7.fhir.r5.model.Reference t : src.getResultsInterpreter()) 107 tgt.addResultsInterpreter(Reference40_50.convertReference(t)); 108 for (org.hl7.fhir.r5.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference40_50.convertReference(t)); 109 for (org.hl7.fhir.r5.model.Reference t : src.getResult()) tgt.addResult(Reference40_50.convertReference(t)); 110 for (org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent t : src.getMedia()) 111 tgt.addMedia(convertDiagnosticReportMediaComponent(t)); 112 if (src.hasConclusion()) 113 tgt.setConclusionElement(String40_50.convertString(src.getConclusionElement())); 114 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getConclusionCode()) 115 tgt.addConclusionCode(CodeableConcept40_50.convertCodeableConcept(t)); 116 for (org.hl7.fhir.r5.model.Attachment t : src.getPresentedForm()) 117 tgt.addPresentedForm(Attachment40_50.convertAttachment(t)); 118 return tgt; 119 } 120 121 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 122 if (src == null || src.isEmpty()) 123 return null; 124 Enumeration<DiagnosticReport.DiagnosticReportStatus> tgt = new Enumeration<>(new DiagnosticReport.DiagnosticReportStatusEnumFactory()); 125 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 126 if (src.getValue() == null) { 127 tgt.setValue(null); 128 } else { 129 switch (src.getValue()) { 130 case REGISTERED: 131 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.REGISTERED); 132 break; 133 case PARTIAL: 134 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PARTIAL); 135 break; 136 case PRELIMINARY: 137 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.PRELIMINARY); 138 break; 139 case FINAL: 140 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.FINAL); 141 break; 142 case AMENDED: 143 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.AMENDED); 144 break; 145 case CORRECTED: 146 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CORRECTED); 147 break; 148 case APPENDED: 149 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.APPENDED); 150 break; 151 case CANCELLED: 152 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.CANCELLED); 153 break; 154 case ENTEREDINERROR: 155 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 156 break; 157 case UNKNOWN: 158 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.UNKNOWN); 159 break; 160 default: 161 tgt.setValue(DiagnosticReport.DiagnosticReportStatus.NULL); 162 break; 163 } 164 } 165 return tgt; 166 } 167 168 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus> convertDiagnosticReportStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportStatus> src) throws FHIRException { 169 if (src == null || src.isEmpty()) 170 return null; 171 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatusEnumFactory()); 172 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 173 if (src.getValue() == null) { 174 tgt.setValue(null); 175 } else { 176 switch (src.getValue()) { 177 case REGISTERED: 178 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.REGISTERED); 179 break; 180 case PARTIAL: 181 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.PARTIAL); 182 break; 183 case PRELIMINARY: 184 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.PRELIMINARY); 185 break; 186 case FINAL: 187 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.FINAL); 188 break; 189 case AMENDED: 190 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.AMENDED); 191 break; 192 case CORRECTED: 193 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.CORRECTED); 194 break; 195 case APPENDED: 196 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.APPENDED); 197 break; 198 case CANCELLED: 199 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.CANCELLED); 200 break; 201 case ENTEREDINERROR: 202 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.ENTEREDINERROR); 203 break; 204 case UNKNOWN: 205 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.UNKNOWN); 206 break; 207 default: 208 tgt.setValue(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportStatus.NULL); 209 break; 210 } 211 } 212 return tgt; 213 } 214 215 public static org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent convertDiagnosticReportMediaComponent(org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent src) throws FHIRException { 216 if (src == null) 217 return null; 218 org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent tgt = new org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent(); 219 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 220 if (src.hasComment()) 221 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 222 if (src.hasLink()) 223 tgt.setLink(Reference40_50.convertReference(src.getLink())); 224 return tgt; 225 } 226 227 public static org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent convertDiagnosticReportMediaComponent(org.hl7.fhir.r5.model.DiagnosticReport.DiagnosticReportMediaComponent src) throws FHIRException { 228 if (src == null) 229 return null; 230 org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent tgt = new org.hl7.fhir.r4.model.DiagnosticReport.DiagnosticReportMediaComponent(); 231 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 232 if (src.hasComment()) 233 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 234 if (src.hasLink()) 235 tgt.setLink(Reference40_50.convertReference(src.getLink())); 236 return tgt; 237 } 238}