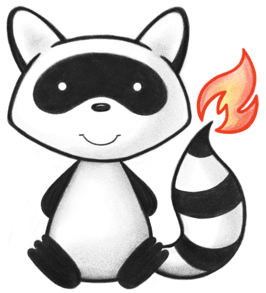
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Attachment40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Instant40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r4.model.DocumentReference; 014import org.hl7.fhir.r5.model.CodeableConcept; 015import org.hl7.fhir.r5.model.CodeableReference; 016import org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceAttesterComponent; 017import org.hl7.fhir.r5.model.Enumeration; 018import org.hl7.fhir.r5.model.Enumerations; 019 020/* 021 Copyright (c) 2011+, HL7, Inc. 022 All rights reserved. 023 024 Redistribution and use in source and binary forms, with or without modification, 025 are permitted provided that the following conditions are met: 026 027 * Redistributions of source code must retain the above copyright notice, this 028 list of conditions and the following disclaimer. 029 * Redistributions in binary form must reproduce the above copyright notice, 030 this list of conditions and the following disclaimer in the documentation 031 and/or other materials provided with the distribution. 032 * Neither the name of HL7 nor the names of its contributors may be used to 033 endorse or promote products derived from this software without specific 034 prior written permission. 035 036 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 037 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 038 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 039 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 040 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 041 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 042 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 043 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 044 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 045 POSSIBILITY OF SUCH DAMAGE. 046 047*/ 048// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 049public class DocumentReference40_50 { 050 051 public static org.hl7.fhir.r5.model.DocumentReference convertDocumentReference(org.hl7.fhir.r4.model.DocumentReference src) throws FHIRException { 052 if (src == null) 053 return null; 054 org.hl7.fhir.r5.model.DocumentReference tgt = new org.hl7.fhir.r5.model.DocumentReference(); 055 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 056 if (src.hasMasterIdentifier()) 057 tgt.addIdentifier(Identifier40_50.convertIdentifier(src.getMasterIdentifier())); 058 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 059 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 060 if (src.hasStatus()) 061 tgt.setStatusElement(Enumerations40_50.convertDocumentReferenceStatus(src.getStatusElement())); 062 if (src.hasDocStatus()) 063 tgt.setDocStatusElement(convertReferredDocumentStatus(src.getDocStatusElement())); 064 if (src.hasType()) 065 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 066 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 067 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 068 if (src.hasSubject()) 069 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 070 if (src.hasDate()) 071 tgt.setDateElement(Instant40_50.convertInstant(src.getDateElement())); 072 for (org.hl7.fhir.r4.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference40_50.convertReference(t)); 073 if (src.hasAuthenticator()) 074 tgt.addAttester().setMode(new org.hl7.fhir.r5.model.CodeableConcept().addCoding(new org.hl7.fhir.r5.model.Coding("http://hl7.org/fhir/composition-attestation-mode","official", "Official"))) 075 .setParty(Reference40_50.convertReference(src.getAuthenticator())); 076 if (src.hasCustodian()) 077 tgt.setCustodian(Reference40_50.convertReference(src.getCustodian())); 078 for (org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 079 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 080 if (src.hasDescription()) 081 tgt.setDescriptionElement(MarkDown40_50.convertStringToMarkdown(src.getDescriptionElement())); 082 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSecurityLabel()) 083 tgt.addSecurityLabel(CodeableConcept40_50.convertCodeableConcept(t)); 084 for (org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 085 tgt.addContent(convertDocumentReferenceContentComponent(t)); 086 if (src.hasContext()) 087 convertDocumentReferenceContextComponent(src.getContext(), tgt); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.r4.model.DocumentReference convertDocumentReference(org.hl7.fhir.r5.model.DocumentReference src) throws FHIRException { 092 if (src == null) 093 return null; 094 org.hl7.fhir.r4.model.DocumentReference tgt = new org.hl7.fhir.r4.model.DocumentReference(); 095 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 096// if (src.hasMasterIdentifier()) 097// tgt.setMasterIdentifier(convertIdentifier(src.getMasterIdentifier())); 098 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 099 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 100 if (src.hasStatus()) 101 tgt.setStatusElement(Enumerations40_50.convertDocumentReferenceStatus(src.getStatusElement())); 102 if (src.hasDocStatus()) 103 tgt.setDocStatusElement(convertReferredDocumentStatus(src.getDocStatusElement())); 104 if (src.hasType()) 105 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 106 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 107 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 108 if (src.hasSubject()) 109 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 110 if (src.hasDate()) 111 tgt.setDateElement(Instant40_50.convertInstant(src.getDateElement())); 112 for (org.hl7.fhir.r5.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference40_50.convertReference(t)); 113 for (DocumentReferenceAttesterComponent t : src.getAttester()) { 114 if (t.getMode().hasCoding("http://hl7.org/fhir/composition-attestation-mode", "official")) 115 tgt.setAuthenticator(Reference40_50.convertReference(t.getParty())); 116 } 117 if (src.hasCustodian()) 118 tgt.setCustodian(Reference40_50.convertReference(src.getCustodian())); 119 for (org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 120 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 121 if (src.hasDescription()) 122 tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 123 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSecurityLabel()) 124 tgt.addSecurityLabel(CodeableConcept40_50.convertCodeableConcept(t)); 125 for (org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 126 tgt.addContent(convertDocumentReferenceContentComponent(t)); 127 convertDocumentReferenceContextComponent(src, tgt.getContext()); 128 return tgt; 129 } 130 131 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> convertReferredDocumentStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DocumentReference.ReferredDocumentStatus> src) throws FHIRException { 132 if (src == null || src.isEmpty()) 133 return null; 134 Enumeration<Enumerations.CompositionStatus> tgt = new Enumeration<>(new Enumerations.CompositionStatusEnumFactory()); 135 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 136 if (src.getValue() == null) { 137 tgt.setValue(null); 138 } else { 139 switch (src.getValue()) { 140 case PRELIMINARY: 141 tgt.setValue(Enumerations.CompositionStatus.PRELIMINARY); 142 break; 143 case FINAL: 144 tgt.setValue(Enumerations.CompositionStatus.FINAL); 145 break; 146 case AMENDED: 147 tgt.setValue(Enumerations.CompositionStatus.AMENDED); 148 break; 149 case ENTEREDINERROR: 150 tgt.setValue(Enumerations.CompositionStatus.ENTEREDINERROR); 151 break; 152 default: 153 tgt.setValue(Enumerations.CompositionStatus.NULL); 154 break; 155 } 156 } 157 return tgt; 158 } 159 160 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DocumentReference.ReferredDocumentStatus> convertReferredDocumentStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> src) throws FHIRException { 161 if (src == null || src.isEmpty()) 162 return null; 163 org.hl7.fhir.r4.model.Enumeration<DocumentReference.ReferredDocumentStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new DocumentReference.ReferredDocumentStatusEnumFactory()); 164 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 165 if (src.getValue() == null) { 166 tgt.setValue(null); 167 } else { 168 switch (src.getValue()) { 169 case PRELIMINARY: 170 tgt.setValue(DocumentReference.ReferredDocumentStatus.PRELIMINARY); 171 break; 172 case FINAL: 173 tgt.setValue(DocumentReference.ReferredDocumentStatus.FINAL); 174 break; 175 case AMENDED: 176 tgt.setValue(DocumentReference.ReferredDocumentStatus.AMENDED); 177 break; 178 case ENTEREDINERROR: 179 tgt.setValue(DocumentReference.ReferredDocumentStatus.ENTEREDINERROR); 180 break; 181 default: 182 tgt.setValue(DocumentReference.ReferredDocumentStatus.NULL); 183 break; 184 } 185 } 186 return tgt; 187 } 188 189 public static org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 190 if (src == null) 191 return null; 192 org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent(); 193 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 194 if (src.hasCode()) 195 tgt.setCode(convertDocumentRelationshipType(src.getCodeElement())); 196 if (src.hasTarget()) 197 tgt.setTarget(Reference40_50.convertReference(src.getTarget())); 198 return tgt; 199 } 200 201 public static org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 202 if (src == null) 203 return null; 204 org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceRelatesToComponent(); 205 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 206 if (src.hasCode()) 207 tgt.setCodeElement(convertDocumentRelationshipType(src.getCode())); 208 if (src.hasTarget()) 209 tgt.setTarget(Reference40_50.convertReference(src.getTarget())); 210 return tgt; 211 } 212 213 static public org.hl7.fhir.r5.model.CodeableConcept convertDocumentRelationshipType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType> src) throws FHIRException { 214 if (src == null || src.isEmpty()) 215 return null; 216 CodeableConcept tgt = new CodeableConcept(); 217 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 218 if (src.getValue() == null) { 219 // Add nothing 220 } else { 221 switch (src.getValue()) { 222 case REPLACES: 223 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("replaces"); 224 break; 225 case TRANSFORMS: 226 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("transforms"); 227 break; 228 case SIGNS: 229 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("signs"); 230 break; 231 case APPENDS: 232 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("appends"); 233 break; 234 default: 235 break; 236 } 237 } 238 return tgt; 239 } 240 241 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 242 if (src == null || src.isEmpty()) 243 return null; 244 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipTypeEnumFactory()); 245 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 246 switch (src.getCode("http://hl7.org/fhir/document-relationship-type")) { 247 case "replaces": 248 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.REPLACES); 249 break; 250 case "transforms": 251 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.TRANSFORMS); 252 break; 253 case "signs": 254 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.SIGNS); 255 break; 256 case "appends": 257 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.APPENDS); 258 break; 259 default: 260 tgt.setValue(org.hl7.fhir.r4.model.DocumentReference.DocumentRelationshipType.NULL); 261 break; 262 } 263 return tgt; 264 } 265 266 public static org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 267 if (src == null) 268 return null; 269 org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent(); 270 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 271 if (src.hasAttachment()) 272 tgt.setAttachment(Attachment40_50.convertAttachment(src.getAttachment())); 273 return tgt; 274 } 275 276 public static org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 277 if (src == null) 278 return null; 279 org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContentComponent(); 280 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 281 if (src.hasAttachment()) 282 tgt.setAttachment(Attachment40_50.convertAttachment(src.getAttachment())); 283 return tgt; 284 } 285 286 public static void convertDocumentReferenceContextComponent(org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContextComponent src, org.hl7.fhir.r5.model.DocumentReference tgt) throws FHIRException { 287 for (org.hl7.fhir.r4.model.Reference t : src.getEncounter()) tgt.addContext(Reference40_50.convertReference(t)); 288 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getEvent()) 289 tgt.addEvent(new CodeableReference().setConcept(CodeableConcept40_50.convertCodeableConcept(t))); 290 if (src.hasPeriod()) 291 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 292 if (src.hasFacilityType()) 293 tgt.setFacilityType(CodeableConcept40_50.convertCodeableConcept(src.getFacilityType())); 294 if (src.hasPracticeSetting()) 295 tgt.setPracticeSetting(CodeableConcept40_50.convertCodeableConcept(src.getPracticeSetting())); 296// if (src.hasSourcePatientInfo()) 297// tgt.setSourcePatientInfo(Reference40_50.convertReference(src.getSourcePatientInfo())); 298// for (org.hl7.fhir.r4.model.Reference t : src.getRelated()) tgt.addRelated(Reference40_50.convertReference(t)); 299 } 300 301 public static void convertDocumentReferenceContextComponent(org.hl7.fhir.r5.model.DocumentReference src, org.hl7.fhir.r4.model.DocumentReference.DocumentReferenceContextComponent tgt) throws FHIRException { 302 for (org.hl7.fhir.r5.model.Reference t : src.getContext()) tgt.addEncounter(Reference40_50.convertReference(t)); 303 for (CodeableReference t : src.getEvent()) 304 if (t.hasConcept()) 305 tgt.addEvent(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 306 if (src.hasPeriod()) 307 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 308 if (src.hasFacilityType()) 309 tgt.setFacilityType(CodeableConcept40_50.convertCodeableConcept(src.getFacilityType())); 310 if (src.hasPracticeSetting()) 311 tgt.setPracticeSetting(CodeableConcept40_50.convertCodeableConcept(src.getPracticeSetting())); 312// if (src.hasSourcePatientInfo()) 313// tgt.setSourcePatientInfo(Reference40_50.convertReference(src.getSourcePatientInfo())); 314// for (org.hl7.fhir.r5.model.Reference t : src.getRelated()) tgt.addRelated(Reference40_50.convertReference(t)); 315 } 316}