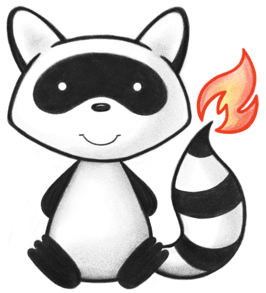
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Coding40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Duration40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r4.model.Encounter; 012import org.hl7.fhir.r5.model.CodeableReference; 013import org.hl7.fhir.r5.model.Encounter.ReasonComponent; 014import org.hl7.fhir.r5.model.Enumeration; 015import org.hl7.fhir.r5.model.Enumerations; 016 017/* 018 Copyright (c) 2011+, HL7, Inc. 019 All rights reserved. 020 021 Redistribution and use in source and binary forms, with or without modification, 022 are permitted provided that the following conditions are met: 023 024 * Redistributions of source code must retain the above copyright notice, this 025 list of conditions and the following disclaimer. 026 * Redistributions in binary form must reproduce the above copyright notice, 027 this list of conditions and the following disclaimer in the documentation 028 and/or other materials provided with the distribution. 029 * Neither the name of HL7 nor the names of its contributors may be used to 030 endorse or promote products derived from this software without specific 031 prior written permission. 032 033 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 034 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 035 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 036 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 037 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 038 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 039 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 040 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 041 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 042 POSSIBILITY OF SUCH DAMAGE. 043 044*/ 045// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 046public class Encounter40_50 { 047 048 public static org.hl7.fhir.r5.model.Encounter convertEncounter(org.hl7.fhir.r4.model.Encounter src) throws FHIRException { 049 if (src == null) 050 return null; 051 org.hl7.fhir.r5.model.Encounter tgt = new org.hl7.fhir.r5.model.Encounter(); 052 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 053 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 054 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 055 if (src.hasStatus()) 056 tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 057// for (org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent t : src.getStatusHistory()) 058// tgt.addStatusHistory(convertStatusHistoryComponent(t)); 059 if (src.hasClass_()) 060 tgt.addClass_(new org.hl7.fhir.r5.model.CodeableConcept().addCoding(Coding40_50.convertCoding(src.getClass_()))); 061// for (org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent t : src.getClassHistory()) 062// tgt.addClassHistory(convertClassHistoryComponent(t)); 063 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 064 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 065 if (src.hasServiceType()) 066 tgt.addServiceType(new CodeableReference(CodeableConcept40_50.convertCodeableConcept(src.getServiceType()))); 067 if (src.hasPriority()) 068 tgt.setPriority(CodeableConcept40_50.convertCodeableConcept(src.getPriority())); 069 if (src.hasSubject()) 070 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 071 for (org.hl7.fhir.r4.model.Reference t : src.getEpisodeOfCare()) 072 tgt.addEpisodeOfCare(Reference40_50.convertReference(t)); 073 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 074 for (org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent t : src.getParticipant()) 075 tgt.addParticipant(convertEncounterParticipantComponent(t)); 076 for (org.hl7.fhir.r4.model.Reference t : src.getAppointment()) 077 tgt.addAppointment(Reference40_50.convertReference(t)); 078 if (src.hasPeriod()) 079 tgt.setActualPeriod(Period40_50.convertPeriod(src.getPeriod())); 080 if (src.hasLength()) 081 tgt.setLength(Duration40_50.convertDuration(src.getLength())); 082 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 083 tgt.addReason().addValue(CodeableConcept40_50.convertCodeableConceptToCodeableReference(t)); 084 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 085 tgt.addReason().addValue(Reference40_50.convertReferenceToCodeableReference(t)); 086 for (org.hl7.fhir.r4.model.Encounter.DiagnosisComponent t : src.getDiagnosis()) 087 tgt.addDiagnosis(convertDiagnosisComponent(t)); 088 for (org.hl7.fhir.r4.model.Reference t : src.getAccount()) tgt.addAccount(Reference40_50.convertReference(t)); 089 if (src.hasHospitalization()) 090 tgt.setAdmission(convertEncounterHospitalizationComponent(src.getHospitalization(), tgt)); 091 for (org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent t : src.getLocation()) 092 tgt.addLocation(convertEncounterLocationComponent(t)); 093 if (src.hasServiceProvider()) 094 tgt.setServiceProvider(Reference40_50.convertReference(src.getServiceProvider())); 095 if (src.hasPartOf()) 096 tgt.setPartOf(Reference40_50.convertReference(src.getPartOf())); 097 return tgt; 098 } 099 100 public static org.hl7.fhir.r4.model.Encounter convertEncounter(org.hl7.fhir.r5.model.Encounter src) throws FHIRException { 101 if (src == null) 102 return null; 103 org.hl7.fhir.r4.model.Encounter tgt = new org.hl7.fhir.r4.model.Encounter(); 104 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 105 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 106 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 107 if (src.hasStatus()) 108 tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 109// for (org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent t : src.getStatusHistory()) 110// tgt.addStatusHistory(convertStatusHistoryComponent(t)); 111 if (src.hasClass_()) 112 tgt.setClass_(Coding40_50.convertCoding(src.getClass_FirstRep().getCodingFirstRep())); 113// for (org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent t : src.getClassHistory()) 114// tgt.addClassHistory(convertClassHistoryComponent(t)); 115 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 116 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 117 if (src.getServiceTypeFirstRep().hasConcept()) 118 tgt.setServiceType(CodeableConcept40_50.convertCodeableConcept(src.getServiceTypeFirstRep().getConcept())); 119 if (src.hasPriority()) 120 tgt.setPriority(CodeableConcept40_50.convertCodeableConcept(src.getPriority())); 121 if (src.hasSubject()) 122 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 123 for (org.hl7.fhir.r5.model.Reference t : src.getEpisodeOfCare()) 124 tgt.addEpisodeOfCare(Reference40_50.convertReference(t)); 125 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 126 for (org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent t : src.getParticipant()) 127 tgt.addParticipant(convertEncounterParticipantComponent(t)); 128 for (org.hl7.fhir.r5.model.Reference t : src.getAppointment()) 129 tgt.addAppointment(Reference40_50.convertReference(t)); 130 if (src.hasActualPeriod()) 131 tgt.setPeriod(Period40_50.convertPeriod(src.getActualPeriod())); 132 if (src.hasLength()) 133 tgt.setLength(Duration40_50.convertDuration(src.getLength())); 134 for (ReasonComponent t1 : src.getReason()) 135 for (CodeableReference t : t1.getValue()) 136 if (t.hasConcept()) 137 tgt.addReasonCode(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 138 for (ReasonComponent t1 : src.getReason()) 139 for (CodeableReference t : t1.getValue()) 140 if (t.hasReference()) 141 tgt.addReasonReference(Reference40_50.convertReference(t.getReference())); 142 for (org.hl7.fhir.r5.model.Encounter.DiagnosisComponent t : src.getDiagnosis()) 143 tgt.addDiagnosis(convertDiagnosisComponent(t)); 144 for (org.hl7.fhir.r5.model.Reference t : src.getAccount()) tgt.addAccount(Reference40_50.convertReference(t)); 145 if (src.hasAdmission() || src.hasDietPreference() || src.hasSpecialArrangement() || src.hasSpecialCourtesy()) 146 tgt.setHospitalization(convertEncounterHospitalizationComponent(src.getAdmission(), src)); 147 for (org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent t : src.getLocation()) 148 tgt.addLocation(convertEncounterLocationComponent(t)); 149 if (src.hasServiceProvider()) 150 tgt.setServiceProvider(Reference40_50.convertReference(src.getServiceProvider())); 151 if (src.hasPartOf()) 152 tgt.setPartOf(Reference40_50.convertReference(src.getPartOf())); 153 return tgt; 154 } 155 156 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EncounterStatus> convertEncounterStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterStatus> src) throws FHIRException { 157 if (src == null || src.isEmpty()) 158 return null; 159 Enumeration<Enumerations.EncounterStatus> tgt = new Enumeration<>(new Enumerations.EncounterStatusEnumFactory()); 160 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 161 if (src.getValue() == null) { 162 tgt.setValue(null); 163 } else { 164 switch (src.getValue()) { 165 case PLANNED: 166 tgt.setValue(Enumerations.EncounterStatus.PLANNED); 167 break; 168 case ARRIVED: 169 tgt.setValue(Enumerations.EncounterStatus.INPROGRESS); 170 break; 171 case TRIAGED: 172 tgt.setValue(Enumerations.EncounterStatus.INPROGRESS); 173 break; 174 case INPROGRESS: 175 tgt.setValue(Enumerations.EncounterStatus.INPROGRESS); 176 break; 177 case ONLEAVE: 178 tgt.setValue(Enumerations.EncounterStatus.INPROGRESS); 179 break; 180 case FINISHED: 181 tgt.setValue(Enumerations.EncounterStatus.COMPLETED); 182 break; 183 case CANCELLED: 184 tgt.setValue(Enumerations.EncounterStatus.CANCELLED); 185 break; 186 case ENTEREDINERROR: 187 tgt.setValue(Enumerations.EncounterStatus.ENTEREDINERROR); 188 break; 189 case UNKNOWN: 190 tgt.setValue(Enumerations.EncounterStatus.UNKNOWN); 191 break; 192 default: 193 tgt.setValue(Enumerations.EncounterStatus.NULL); 194 break; 195 } 196 } 197 return tgt; 198 } 199 200 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterStatus> convertEncounterStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EncounterStatus> src) throws FHIRException { 201 if (src == null || src.isEmpty()) 202 return null; 203 org.hl7.fhir.r4.model.Enumeration<Encounter.EncounterStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new Encounter.EncounterStatusEnumFactory()); 204 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 205 if (src.getValue() == null) { 206 tgt.setValue(null); 207 } else { 208 switch (src.getValue()) { 209 case PLANNED: 210 tgt.setValue(Encounter.EncounterStatus.PLANNED); 211 break; 212 case INPROGRESS: 213 tgt.setValue(Encounter.EncounterStatus.INPROGRESS); 214 break; 215 case CANCELLED: 216 tgt.setValue(Encounter.EncounterStatus.CANCELLED); 217 break; 218 case COMPLETED: 219 tgt.setValue(Encounter.EncounterStatus.FINISHED); 220 break; 221 case ENTEREDINERROR: 222 tgt.setValue(Encounter.EncounterStatus.ENTEREDINERROR); 223 break; 224 case UNKNOWN: 225 tgt.setValue(Encounter.EncounterStatus.UNKNOWN); 226 break; 227 default: 228 tgt.setValue(Encounter.EncounterStatus.NULL); 229 break; 230 } 231 } 232 return tgt; 233 } 234 235// public static org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent convertStatusHistoryComponent(org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent src) throws FHIRException { 236// if (src == null) 237// return null; 238// org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent tgt = new org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent(); 239// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 240// if (src.hasStatus()) 241// tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 242// if (src.hasPeriod()) 243// tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 244// return tgt; 245// } 246// 247// public static org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent convertStatusHistoryComponent(org.hl7.fhir.r5.model.Encounter.StatusHistoryComponent src) throws FHIRException { 248// if (src == null) 249// return null; 250// org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent tgt = new org.hl7.fhir.r4.model.Encounter.StatusHistoryComponent(); 251// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 252// if (src.hasStatus()) 253// tgt.setStatusElement(convertEncounterStatus(src.getStatusElement())); 254// if (src.hasPeriod()) 255// tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 256// return tgt; 257// } 258// 259// public static org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent convertClassHistoryComponent(org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent src) throws FHIRException { 260// if (src == null) 261// return null; 262// org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent tgt = new org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent(); 263// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 264// if (src.hasClass_()) 265// tgt.setClass_(Coding40_50.convertCoding(src.getClass_())); 266// if (src.hasPeriod()) 267// tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 268// return tgt; 269// } 270// 271// public static org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent convertClassHistoryComponent(org.hl7.fhir.r5.model.Encounter.ClassHistoryComponent src) throws FHIRException { 272// if (src == null) 273// return null; 274// org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent tgt = new org.hl7.fhir.r4.model.Encounter.ClassHistoryComponent(); 275// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 276// if (src.hasClass_()) 277// tgt.setClass_(Coding40_50.convertCoding(src.getClass_())); 278// if (src.hasPeriod()) 279// tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 280// return tgt; 281// } 282 283 public static org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent convertEncounterParticipantComponent(org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent src) throws FHIRException { 284 if (src == null) 285 return null; 286 org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent tgt = new org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent(); 287 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 288 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 289 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 290 if (src.hasPeriod()) 291 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 292 if (src.hasIndividual()) 293 tgt.setActor(Reference40_50.convertReference(src.getIndividual())); 294 return tgt; 295 } 296 297 public static org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent convertEncounterParticipantComponent(org.hl7.fhir.r5.model.Encounter.EncounterParticipantComponent src) throws FHIRException { 298 if (src == null) 299 return null; 300 org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent tgt = new org.hl7.fhir.r4.model.Encounter.EncounterParticipantComponent(); 301 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 302 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 303 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 304 if (src.hasPeriod()) 305 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 306 if (src.hasActor()) 307 tgt.setIndividual(Reference40_50.convertReference(src.getActor())); 308 return tgt; 309 } 310 311 public static org.hl7.fhir.r5.model.Encounter.DiagnosisComponent convertDiagnosisComponent(org.hl7.fhir.r4.model.Encounter.DiagnosisComponent src) throws FHIRException { 312 if (src == null) 313 return null; 314 org.hl7.fhir.r5.model.Encounter.DiagnosisComponent tgt = new org.hl7.fhir.r5.model.Encounter.DiagnosisComponent(); 315 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 316 if (src.hasCondition()) 317 tgt.addCondition(Reference40_50.convertReferenceToCodeableReference(src.getCondition())); 318 if (src.hasUse()) 319 tgt.addUse(CodeableConcept40_50.convertCodeableConcept(src.getUse())); 320// if (src.hasRank()) 321// tgt.setRankElement(PositiveInt40_50.convertPositiveInt(src.getRankElement())); 322 return tgt; 323 } 324 325 public static org.hl7.fhir.r4.model.Encounter.DiagnosisComponent convertDiagnosisComponent(org.hl7.fhir.r5.model.Encounter.DiagnosisComponent src) throws FHIRException { 326 if (src == null) 327 return null; 328 org.hl7.fhir.r4.model.Encounter.DiagnosisComponent tgt = new org.hl7.fhir.r4.model.Encounter.DiagnosisComponent(); 329 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 330 if (src.hasCondition() && src.getConditionFirstRep().hasReference()) 331 tgt.setCondition(Reference40_50.convertReference(src.getConditionFirstRep().getReference())); 332 if (src.hasUse()) 333 tgt.setUse(CodeableConcept40_50.convertCodeableConcept(src.getUseFirstRep())); 334// if (src.hasRank()) 335// tgt.setRankElement(PositiveInt40_50.convertPositiveInt(src.getRankElement())); 336 return tgt; 337 } 338 339 public static org.hl7.fhir.r5.model.Encounter.EncounterAdmissionComponent convertEncounterHospitalizationComponent(org.hl7.fhir.r4.model.Encounter.EncounterHospitalizationComponent src, org.hl7.fhir.r5.model.Encounter tgte) throws FHIRException { 340 if (src == null) 341 return null; 342 org.hl7.fhir.r5.model.Encounter.EncounterAdmissionComponent tgt = new org.hl7.fhir.r5.model.Encounter.EncounterAdmissionComponent(); 343 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 344 if (src.hasPreAdmissionIdentifier()) 345 tgt.setPreAdmissionIdentifier(Identifier40_50.convertIdentifier(src.getPreAdmissionIdentifier())); 346 if (src.hasOrigin()) 347 tgt.setOrigin(Reference40_50.convertReference(src.getOrigin())); 348 if (src.hasAdmitSource()) 349 tgt.setAdmitSource(CodeableConcept40_50.convertCodeableConcept(src.getAdmitSource())); 350 if (src.hasReAdmission()) 351 tgt.setReAdmission(CodeableConcept40_50.convertCodeableConcept(src.getReAdmission())); 352 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getDietPreference()) 353 tgte.addDietPreference(CodeableConcept40_50.convertCodeableConcept(t)); 354 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialCourtesy()) 355 tgte.addSpecialCourtesy(CodeableConcept40_50.convertCodeableConcept(t)); 356 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialArrangement()) 357 tgte.addSpecialArrangement(CodeableConcept40_50.convertCodeableConcept(t)); 358 if (src.hasDestination()) 359 tgt.setDestination(Reference40_50.convertReference(src.getDestination())); 360 if (src.hasDischargeDisposition()) 361 tgt.setDischargeDisposition(CodeableConcept40_50.convertCodeableConcept(src.getDischargeDisposition())); 362 return tgt; 363 } 364 365 public static org.hl7.fhir.r4.model.Encounter.EncounterHospitalizationComponent convertEncounterHospitalizationComponent(org.hl7.fhir.r5.model.Encounter.EncounterAdmissionComponent src, org.hl7.fhir.r5.model.Encounter srce) throws FHIRException { 366 if (src == null) 367 return null; 368 org.hl7.fhir.r4.model.Encounter.EncounterHospitalizationComponent tgt = new org.hl7.fhir.r4.model.Encounter.EncounterHospitalizationComponent(); 369 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 370 if (src.hasPreAdmissionIdentifier()) 371 tgt.setPreAdmissionIdentifier(Identifier40_50.convertIdentifier(src.getPreAdmissionIdentifier())); 372 if (src.hasOrigin()) 373 tgt.setOrigin(Reference40_50.convertReference(src.getOrigin())); 374 if (src.hasAdmitSource()) 375 tgt.setAdmitSource(CodeableConcept40_50.convertCodeableConcept(src.getAdmitSource())); 376 if (src.hasReAdmission()) 377 tgt.setReAdmission(CodeableConcept40_50.convertCodeableConcept(src.getReAdmission())); 378 for (org.hl7.fhir.r5.model.CodeableConcept t : srce.getDietPreference()) 379 tgt.addDietPreference(CodeableConcept40_50.convertCodeableConcept(t)); 380 for (org.hl7.fhir.r5.model.CodeableConcept t : srce.getSpecialCourtesy()) 381 tgt.addSpecialCourtesy(CodeableConcept40_50.convertCodeableConcept(t)); 382 for (org.hl7.fhir.r5.model.CodeableConcept t : srce.getSpecialArrangement()) 383 tgt.addSpecialArrangement(CodeableConcept40_50.convertCodeableConcept(t)); 384 if (src.hasDestination()) 385 tgt.setDestination(Reference40_50.convertReference(src.getDestination())); 386 if (src.hasDischargeDisposition()) 387 tgt.setDischargeDisposition(CodeableConcept40_50.convertCodeableConcept(src.getDischargeDisposition())); 388 return tgt; 389 } 390 391 public static org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent convertEncounterLocationComponent(org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent src) throws FHIRException { 392 if (src == null) 393 return null; 394 org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent tgt = new org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent(); 395 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 396 if (src.hasLocation()) 397 tgt.setLocation(Reference40_50.convertReference(src.getLocation())); 398 if (src.hasStatus()) 399 tgt.setStatusElement(convertEncounterLocationStatus(src.getStatusElement())); 400 if (src.hasPhysicalType()) 401 tgt.setForm(CodeableConcept40_50.convertCodeableConcept(src.getPhysicalType())); 402 if (src.hasPeriod()) 403 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 404 return tgt; 405 } 406 407 public static org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent convertEncounterLocationComponent(org.hl7.fhir.r5.model.Encounter.EncounterLocationComponent src) throws FHIRException { 408 if (src == null) 409 return null; 410 org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent tgt = new org.hl7.fhir.r4.model.Encounter.EncounterLocationComponent(); 411 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 412 if (src.hasLocation()) 413 tgt.setLocation(Reference40_50.convertReference(src.getLocation())); 414 if (src.hasStatus()) 415 tgt.setStatusElement(convertEncounterLocationStatus(src.getStatusElement())); 416 if (src.hasForm()) 417 tgt.setPhysicalType(CodeableConcept40_50.convertCodeableConcept(src.getForm())); 418 if (src.hasPeriod()) 419 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 420 return tgt; 421 } 422 423 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus> convertEncounterLocationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus> src) throws FHIRException { 424 if (src == null || src.isEmpty()) 425 return null; 426 Enumeration<org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus> tgt = new Enumeration<>(new org.hl7.fhir.r5.model.Encounter.EncounterLocationStatusEnumFactory()); 427 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 428 if (src.getValue() == null) { 429 tgt.setValue(null); 430 } else { 431 switch (src.getValue()) { 432 case PLANNED: 433 tgt.setValue(org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus.PLANNED); 434 break; 435 case ACTIVE: 436 tgt.setValue(org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus.ACTIVE); 437 break; 438 case RESERVED: 439 tgt.setValue(org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus.RESERVED); 440 break; 441 case COMPLETED: 442 tgt.setValue(org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus.COMPLETED); 443 break; 444 default: 445 tgt.setValue(org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus.NULL); 446 break; 447 } 448 } 449 return tgt; 450 } 451 452 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Encounter.EncounterLocationStatus> convertEncounterLocationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Encounter.EncounterLocationStatus> src) throws FHIRException { 453 if (src == null || src.isEmpty()) 454 return null; 455 org.hl7.fhir.r4.model.Enumeration<Encounter.EncounterLocationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new Encounter.EncounterLocationStatusEnumFactory()); 456 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 457 if (src.getValue() == null) { 458 tgt.setValue(null); 459 } else { 460 switch (src.getValue()) { 461 case PLANNED: 462 tgt.setValue(Encounter.EncounterLocationStatus.PLANNED); 463 break; 464 case ACTIVE: 465 tgt.setValue(Encounter.EncounterLocationStatus.ACTIVE); 466 break; 467 case RESERVED: 468 tgt.setValue(Encounter.EncounterLocationStatus.RESERVED); 469 break; 470 case COMPLETED: 471 tgt.setValue(Encounter.EncounterLocationStatus.COMPLETED); 472 break; 473 default: 474 tgt.setValue(Encounter.EncounterLocationStatus.NULL); 475 break; 476 } 477 } 478 return tgt; 479 } 480}