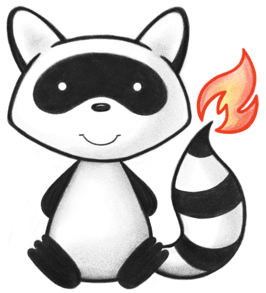
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Extension40_50; 005import org.hl7.fhir.exceptions.FHIRException; 006import org.hl7.fhir.r5.model.DocumentReference; 007import org.hl7.fhir.r5.model.Enumeration; 008import org.hl7.fhir.r5.model.Enumerations; 009import org.hl7.fhir.r5.model.PaymentReconciliation; 010 011/* 012 Copyright (c) 2011+, HL7, Inc. 013 All rights reserved. 014 015 Redistribution and use in source and binary forms, with or without modification, 016 are permitted provided that the following conditions are met: 017 018 * Redistributions of source code must retain the above copyright notice, this 019 list of conditions and the following disclaimer. 020 * Redistributions in binary form must reproduce the above copyright notice, 021 this list of conditions and the following disclaimer in the documentation 022 and/or other materials provided with the distribution. 023 * Neither the name of HL7 nor the names of its contributors may be used to 024 endorse or promote products derived from this software without specific 025 prior written permission. 026 027 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 028 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 029 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 030 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 031 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 032 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 033 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 034 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 035 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 036 POSSIBILITY OF SUCH DAMAGE. 037 038*/ 039// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 040public class Enumerations40_50 { 041 042 public static void copyEnumeration(org.hl7.fhir.r4.model.Enumeration<?> src, org.hl7.fhir.r5.model.Enumeration<?> tgt) throws FHIRException { 043 if (src.hasId()) tgt.setId(src.getId()); 044 for (org.hl7.fhir.r4.model.Extension e : src.getExtension()) { 045 tgt.addExtension(Extension40_50.convertExtension(e)); 046 } 047 } 048 049 public static void copyEnumeration(org.hl7.fhir.r5.model.Enumeration<?> src, org.hl7.fhir.r4.model.Enumeration<?> tgt) throws FHIRException { 050 if (src.hasId()) tgt.setId(src.getId()); 051 for (org.hl7.fhir.r5.model.Extension e : src.getExtension()) { 052 tgt.addExtension(Extension40_50.convertExtension(e)); 053 } 054 } 055 056 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> src) throws FHIRException { 057 if (src == null || src.isEmpty()) 058 return null; 059 Enumeration<Enumerations.BindingStrength> tgt = new Enumeration<>(new Enumerations.BindingStrengthEnumFactory()); 060 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 061 if (src.getValue() == null) { 062 tgt.setValue(null); 063 } else { 064 switch (src.getValue()) { 065 case REQUIRED: 066 tgt.setValue(Enumerations.BindingStrength.REQUIRED); 067 break; 068 case EXTENSIBLE: 069 tgt.setValue(Enumerations.BindingStrength.EXTENSIBLE); 070 break; 071 case PREFERRED: 072 tgt.setValue(Enumerations.BindingStrength.PREFERRED); 073 break; 074 case EXAMPLE: 075 tgt.setValue(Enumerations.BindingStrength.EXAMPLE); 076 break; 077 default: 078 tgt.setValue(Enumerations.BindingStrength.NULL); 079 break; 080 } 081 } 082 return tgt; 083 } 084 085 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.BindingStrength> src) throws FHIRException { 086 if (src == null || src.isEmpty()) 087 return null; 088 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.BindingStrengthEnumFactory()); 089 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 090 if (src.getValue() == null) { 091 tgt.setValue(null); 092 } else { 093 switch (src.getValue()) { 094 case REQUIRED: 095 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.REQUIRED); 096 break; 097 case EXTENSIBLE: 098 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.EXTENSIBLE); 099 break; 100 case PREFERRED: 101 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.PREFERRED); 102 break; 103 case EXAMPLE: 104 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.EXAMPLE); 105 break; 106 default: 107 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.BindingStrength.NULL); 108 break; 109 } 110 } 111 return tgt; 112 } 113 114 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> convertPublicationStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> src) throws FHIRException { 115 if (src == null || src.isEmpty()) 116 return null; 117 Enumeration<Enumerations.PublicationStatus> tgt = new Enumeration<>(new Enumerations.PublicationStatusEnumFactory()); 118 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 119 if (src.getValue() == null) { 120 tgt.setValue(null); 121 } else { 122 switch (src.getValue()) { 123 case DRAFT: 124 tgt.setValue(Enumerations.PublicationStatus.DRAFT); 125 break; 126 case ACTIVE: 127 tgt.setValue(Enumerations.PublicationStatus.ACTIVE); 128 break; 129 case RETIRED: 130 tgt.setValue(Enumerations.PublicationStatus.RETIRED); 131 break; 132 case UNKNOWN: 133 tgt.setValue(Enumerations.PublicationStatus.UNKNOWN); 134 break; 135 default: 136 tgt.setValue(Enumerations.PublicationStatus.NULL); 137 break; 138 } 139 } 140 return tgt; 141 } 142 143 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> convertPublicationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> src) throws FHIRException { 144 if (src == null || src.isEmpty()) 145 return null; 146 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.PublicationStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.PublicationStatusEnumFactory()); 147 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 148 if (src.getValue() == null) { 149 tgt.setValue(null); 150 } else { 151 switch (src.getValue()) { 152 case DRAFT: 153 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.DRAFT); 154 break; 155 case ACTIVE: 156 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.ACTIVE); 157 break; 158 case RETIRED: 159 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.RETIRED); 160 break; 161 case UNKNOWN: 162 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.UNKNOWN); 163 break; 164 default: 165 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.PublicationStatus.NULL); 166 break; 167 } 168 } 169 return tgt; 170 } 171 172 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FHIRVersion> convertFHIRVersion(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.FHIRVersion> src) throws FHIRException { 173 if (src == null || src.isEmpty()) 174 return null; 175 Enumeration<Enumerations.FHIRVersion> tgt = new Enumeration<>(new Enumerations.FHIRVersionEnumFactory()); 176 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 177 if (src.getValue() == null) { 178 tgt.setValue(null); 179 } else { 180 switch (src.getValue()) { 181 case _0_01: 182 tgt.setValue(Enumerations.FHIRVersion._0_01); 183 break; 184 case _0_05: 185 tgt.setValue(Enumerations.FHIRVersion._0_05); 186 break; 187 case _0_06: 188 tgt.setValue(Enumerations.FHIRVersion._0_06); 189 break; 190 case _0_11: 191 tgt.setValue(Enumerations.FHIRVersion._0_11); 192 break; 193 case _0_0_80: 194 tgt.setValue(Enumerations.FHIRVersion._0_0_80); 195 break; 196 case _0_0_81: 197 tgt.setValue(Enumerations.FHIRVersion._0_0_81); 198 break; 199 case _0_0_82: 200 tgt.setValue(Enumerations.FHIRVersion._0_0_82); 201 break; 202 case _0_4_0: 203 tgt.setValue(Enumerations.FHIRVersion._0_4_0); 204 break; 205 case _0_5_0: 206 tgt.setValue(Enumerations.FHIRVersion._0_5_0); 207 break; 208 case _1_0_0: 209 tgt.setValue(Enumerations.FHIRVersion._1_0_0); 210 break; 211 case _1_0_1: 212 tgt.setValue(Enumerations.FHIRVersion._1_0_1); 213 break; 214 case _1_0_2: 215 tgt.setValue(Enumerations.FHIRVersion._1_0_2); 216 break; 217 case _1_1_0: 218 tgt.setValue(Enumerations.FHIRVersion._1_1_0); 219 break; 220 case _1_4_0: 221 tgt.setValue(Enumerations.FHIRVersion._1_4_0); 222 break; 223 case _1_6_0: 224 tgt.setValue(Enumerations.FHIRVersion._1_6_0); 225 break; 226 case _1_8_0: 227 tgt.setValue(Enumerations.FHIRVersion._1_8_0); 228 break; 229 case _3_0_0: 230 tgt.setValue(Enumerations.FHIRVersion._3_0_0); 231 break; 232 case _3_0_1: 233 tgt.setValue(Enumerations.FHIRVersion._3_0_1); 234 break; 235 case _3_0_2: 236 tgt.setValue(Enumerations.FHIRVersion._3_0_2); 237 break; 238 case _3_3_0: 239 tgt.setValue(Enumerations.FHIRVersion._3_3_0); 240 break; 241 case _3_5_0: 242 tgt.setValue(Enumerations.FHIRVersion._3_5_0); 243 break; 244 case _4_0_0: 245 tgt.setValue(Enumerations.FHIRVersion._4_0_0); 246 break; 247 case _4_0_1: 248 tgt.setValue(Enumerations.FHIRVersion._4_0_1); 249 break; 250 case _4_1_0: 251 tgt.setValue(Enumerations.FHIRVersion._4_1_0); 252 break; 253 case _4_3_0: 254 tgt.setValue(Enumerations.FHIRVersion._4_3_0); 255 break; 256 case _5_0_0: 257 tgt.setValue(Enumerations.FHIRVersion._5_0_0); 258 break; 259 case _5_0_0SNAPSHOT1: 260 tgt.setValue(Enumerations.FHIRVersion._5_0_0SNAPSHOT1); 261 break; 262 case _5_0_0SNAPSHOT2: 263 tgt.setValue(Enumerations.FHIRVersion._5_0_0SNAPSHOT2); 264 break; 265 case _5_0_0BALLOT: 266 tgt.setValue(Enumerations.FHIRVersion._5_0_0BALLOT); 267 break; 268 case _5_0_0SNAPSHOT3: 269 tgt.setValue(Enumerations.FHIRVersion._5_0_0SNAPSHOT3); 270 break; 271 case _5_0_0DRAFTFINAL: 272 tgt.setValue(Enumerations.FHIRVersion._5_0_0DRAFTFINAL); 273 break; 274 case _6_0_0: 275 tgt.setValue(Enumerations.FHIRVersion._6_0_0); 276 break; 277 case _6_0_0_BALLOT1: 278 tgt.setValue(Enumerations.FHIRVersion._6_0_0_BALLOT1); 279 break; 280 case _6_0_0_BALLOT2: 281 tgt.setValue(Enumerations.FHIRVersion._6_0_0_BALLOT2); 282 break; 283 case _6_0_0_BALLOT3: 284 tgt.setValue(Enumerations.FHIRVersion._6_0_0_BALLOT3); 285 break; 286 default: 287 tgt.setValue(Enumerations.FHIRVersion.NULL); 288 break; 289 } 290 } 291 return tgt; 292 } 293 294 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.FHIRVersion> convertFHIRVersion(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FHIRVersion> src) throws FHIRException { 295 if (src == null || src.isEmpty()) 296 return null; 297 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.FHIRVersion> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.FHIRVersionEnumFactory()); 298 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 299 if (src.getValue() == null) { 300 tgt.setValue(null); 301 } else { 302 switch (src.getValue()) { 303 case _0_01: 304 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_01); 305 break; 306 case _0_05: 307 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_05); 308 break; 309 case _0_06: 310 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_06); 311 break; 312 case _0_11: 313 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_11); 314 break; 315 case _0_0_80: 316 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_0_80); 317 break; 318 case _0_0_81: 319 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_0_81); 320 break; 321 case _0_0_82: 322 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_0_82); 323 break; 324 case _0_4_0: 325 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_4_0); 326 break; 327 case _0_5_0: 328 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._0_5_0); 329 break; 330 case _1_0_0: 331 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._1_0_0); 332 break; 333 case _1_0_1: 334 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._1_0_1); 335 break; 336 case _1_0_2: 337 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._1_0_2); 338 break; 339 case _1_1_0: 340 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._1_1_0); 341 break; 342 case _1_4_0: 343 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._1_4_0); 344 break; 345 case _1_6_0: 346 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._1_6_0); 347 break; 348 case _1_8_0: 349 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._1_8_0); 350 break; 351 case _3_0_0: 352 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._3_0_0); 353 break; 354 case _3_0_1: 355 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._3_0_1); 356 break; 357 case _3_0_2: 358 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._3_0_2); 359 break; 360 case _3_3_0: 361 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._3_3_0); 362 break; 363 case _3_5_0: 364 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._3_5_0); 365 break; 366 case _4_0_0: 367 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._4_0_0); 368 break; 369 case _4_0_1: 370 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._4_0_1); 371 break; 372 case _4_1_0: 373 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._4_1_0); 374 break; 375 case _4_3_0: 376 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._4_3_0); 377 break; 378 case _5_0_0: 379 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._5_0_0); 380 break; 381 case _5_0_0SNAPSHOT1: 382 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._5_0_0SNAPSHOT1); 383 break; 384 case _5_0_0SNAPSHOT2: 385 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._5_0_0SNAPSHOT2); 386 break; 387 case _5_0_0BALLOT: 388 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._5_0_0BALLOT); 389 break; 390 case _5_0_0SNAPSHOT3: 391 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._5_0_0SNAPSHOT3); 392 break; 393 case _5_0_0DRAFTFINAL: 394 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._5_0_0DRAFTFINAL); 395 break; 396 case _6_0_0: 397 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._6_0_0); 398 break; 399 case _6_0_0_BALLOT1: 400 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._6_0_0_BALLOT1); 401 break; 402 case _6_0_0_BALLOT2: 403 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._6_0_0_BALLOT2); 404 break; 405 case _6_0_0_BALLOT3: 406 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion._6_0_0_BALLOT3); 407 break; 408 409 default: 410 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.FHIRVersion.NULL); 411 break; 412 } 413 } 414 return tgt; 415 } 416 417 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> src) throws FHIRException { 418 if (src == null || src.isEmpty()) 419 return null; 420 Enumeration<Enumerations.SearchParamType> tgt = new Enumeration<>(new Enumerations.SearchParamTypeEnumFactory()); 421 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 422 if (src.getValue() == null) { 423 tgt.setValue(null); 424 } else { 425 switch (src.getValue()) { 426 case NUMBER: 427 tgt.setValue(Enumerations.SearchParamType.NUMBER); 428 break; 429 case DATE: 430 tgt.setValue(Enumerations.SearchParamType.DATE); 431 break; 432 case STRING: 433 tgt.setValue(Enumerations.SearchParamType.STRING); 434 break; 435 case TOKEN: 436 tgt.setValue(Enumerations.SearchParamType.TOKEN); 437 break; 438 case REFERENCE: 439 tgt.setValue(Enumerations.SearchParamType.REFERENCE); 440 break; 441 case COMPOSITE: 442 tgt.setValue(Enumerations.SearchParamType.COMPOSITE); 443 break; 444 case QUANTITY: 445 tgt.setValue(Enumerations.SearchParamType.QUANTITY); 446 break; 447 case URI: 448 tgt.setValue(Enumerations.SearchParamType.URI); 449 break; 450 case SPECIAL: 451 tgt.setValue(Enumerations.SearchParamType.SPECIAL); 452 break; 453 default: 454 tgt.setValue(Enumerations.SearchParamType.NULL); 455 break; 456 } 457 } 458 return tgt; 459 } 460 461 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchParamType> src) throws FHIRException { 462 if (src == null || src.isEmpty()) 463 return null; 464 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.SearchParamTypeEnumFactory()); 465 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 466 if (src.getValue() == null) { 467 tgt.setValue(null); 468 } else { 469 switch (src.getValue()) { 470 case NUMBER: 471 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.NUMBER); 472 break; 473 case DATE: 474 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.DATE); 475 break; 476 case STRING: 477 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.STRING); 478 break; 479 case TOKEN: 480 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.TOKEN); 481 break; 482 case REFERENCE: 483 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.REFERENCE); 484 break; 485 case COMPOSITE: 486 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.COMPOSITE); 487 break; 488 case QUANTITY: 489 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.QUANTITY); 490 break; 491 case URI: 492 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.URI); 493 break; 494 case SPECIAL: 495 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.SPECIAL); 496 break; 497 default: 498 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.SearchParamType.NULL); 499 break; 500 } 501 } 502 return tgt; 503 } 504 505 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.PaymentReconciliation.NoteType> convertNoteType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.NoteType> src) throws FHIRException { 506 if (src == null || src.isEmpty()) 507 return null; 508 Enumeration<PaymentReconciliation.NoteType> tgt = new Enumeration<>(new PaymentReconciliation.NoteTypeEnumFactory()); 509 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 510 if (src.getValue() == null) { 511 tgt.setValue(null); 512 } else { 513 switch (src.getValue()) { 514 case DISPLAY: 515 tgt.setValue(PaymentReconciliation.NoteType.DISPLAY); 516 break; 517 case PRINT: 518 tgt.setValue(PaymentReconciliation.NoteType.PRINT); 519 break; 520 case PRINTOPER: 521 tgt.setValue(PaymentReconciliation.NoteType.PRINTOPER); 522 break; 523 default: 524 tgt.setValue(PaymentReconciliation.NoteType.NULL); 525 break; 526 } 527 } 528 return tgt; 529 } 530 531 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.NoteType> convertNoteType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.PaymentReconciliation.NoteType> src) throws FHIRException { 532 if (src == null || src.isEmpty()) 533 return null; 534 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.NoteType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.NoteTypeEnumFactory()); 535 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 536 if (src.getValue() == null) { 537 tgt.setValue(null); 538 } else { 539 switch (src.getValue()) { 540 case DISPLAY: 541 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.NoteType.DISPLAY); 542 break; 543 case PRINT: 544 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.NoteType.PRINT); 545 break; 546 case PRINTOPER: 547 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.NoteType.PRINTOPER); 548 break; 549 default: 550 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.NoteType.NULL); 551 break; 552 } 553 } 554 return tgt; 555 } 556 557 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship> convertConceptMapRelationship(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence> src) throws FHIRException { 558 if (src == null || src.isEmpty()) 559 return null; 560 Enumeration<Enumerations.ConceptMapRelationship> tgt = new Enumeration<>(new Enumerations.ConceptMapRelationshipEnumFactory()); 561 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 562 if (src.getValue() == null) { 563 tgt.setValue(null); 564 } else { 565 switch (src.getValue()) { 566 case RELATEDTO: 567 tgt.setValue(Enumerations.ConceptMapRelationship.RELATEDTO); 568 break; 569 case EQUIVALENT: 570 tgt.setValue(Enumerations.ConceptMapRelationship.EQUIVALENT); 571 break; 572 case EQUAL: 573 tgt.setValue(Enumerations.ConceptMapRelationship.EQUIVALENT); 574 break; 575 case WIDER: 576 tgt.setValue(Enumerations.ConceptMapRelationship.SOURCEISNARROWERTHANTARGET); 577 break; 578 case SUBSUMES: 579 tgt.setValue(Enumerations.ConceptMapRelationship.SOURCEISNARROWERTHANTARGET); 580 break; 581 case NARROWER: 582 tgt.setValue(Enumerations.ConceptMapRelationship.SOURCEISBROADERTHANTARGET); 583 break; 584 case SPECIALIZES: 585 tgt.setValue(Enumerations.ConceptMapRelationship.SOURCEISBROADERTHANTARGET); 586 break; 587 case INEXACT: 588 tgt.setValue(Enumerations.ConceptMapRelationship.RELATEDTO); 589 break; 590 case UNMATCHED: 591 tgt.setValue(Enumerations.ConceptMapRelationship.NULL); 592 break; 593 case DISJOINT: 594 tgt.setValue(Enumerations.ConceptMapRelationship.NOTRELATEDTO); 595 break; 596 default: 597 tgt.setValue(Enumerations.ConceptMapRelationship.NULL); 598 break; 599 } 600 } 601 return tgt; 602 } 603 604 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence> convertConceptMapEquivalence(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ConceptMapRelationship> src) throws FHIRException { 605 if (src == null || src.isEmpty()) 606 return null; 607 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalenceEnumFactory()); 608 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 609 if (src.getValue() == null) { 610 tgt.setValue(null); 611 } else { 612 switch (src.getValue()) { 613 case RELATEDTO: 614 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.RELATEDTO); 615 break; 616 case EQUIVALENT: 617 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.EQUIVALENT); 618 break; 619 case SOURCEISNARROWERTHANTARGET: 620 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.WIDER); 621 break; 622 case SOURCEISBROADERTHANTARGET: 623 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.NARROWER); 624 break; 625 case NOTRELATEDTO: 626 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.DISJOINT); 627 break; 628 default: 629 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.ConceptMapEquivalence.NULL); 630 break; 631 } 632 } 633 return tgt; 634 } 635 636 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus> src) throws FHIRException { 637 if (src == null || src.isEmpty()) 638 return null; 639 Enumeration<DocumentReference.DocumentReferenceStatus> tgt = new Enumeration<>(new DocumentReference.DocumentReferenceStatusEnumFactory()); 640 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 641 if (src.getValue() == null) { 642 tgt.setValue(null); 643 } else { 644 switch (src.getValue()) { 645 case CURRENT: 646 tgt.setValue(DocumentReference.DocumentReferenceStatus.CURRENT); 647 break; 648 case SUPERSEDED: 649 tgt.setValue(DocumentReference.DocumentReferenceStatus.SUPERSEDED); 650 break; 651 case ENTEREDINERROR: 652 tgt.setValue(DocumentReference.DocumentReferenceStatus.ENTEREDINERROR); 653 break; 654 default: 655 tgt.setValue(DocumentReference.DocumentReferenceStatus.NULL); 656 break; 657 } 658 } 659 return tgt; 660 } 661 662 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> src) throws FHIRException { 663 if (src == null || src.isEmpty()) 664 return null; 665 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatusEnumFactory()); 666 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 667 if (src.getValue() == null) { 668 tgt.setValue(null); 669 } else { 670 switch (src.getValue()) { 671 case CURRENT: 672 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus.CURRENT); 673 break; 674 case SUPERSEDED: 675 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus.SUPERSEDED); 676 break; 677 case ENTEREDINERROR: 678 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus.ENTEREDINERROR); 679 break; 680 default: 681 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.DocumentReferenceStatus.NULL); 682 break; 683 } 684 } 685 return tgt; 686 } 687 688 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.AdministrativeGender> src) throws FHIRException { 689 if (src == null || src.isEmpty()) 690 return null; 691 Enumeration<Enumerations.AdministrativeGender> tgt = new Enumeration<>(new Enumerations.AdministrativeGenderEnumFactory()); 692 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 693 if (src.getValue() == null) { 694 tgt.setValue(null); 695 } else { 696 switch (src.getValue()) { 697 case MALE: 698 tgt.setValue(Enumerations.AdministrativeGender.MALE); 699 break; 700 case FEMALE: 701 tgt.setValue(Enumerations.AdministrativeGender.FEMALE); 702 break; 703 case OTHER: 704 tgt.setValue(Enumerations.AdministrativeGender.OTHER); 705 break; 706 case UNKNOWN: 707 tgt.setValue(Enumerations.AdministrativeGender.UNKNOWN); 708 break; 709 default: 710 tgt.setValue(Enumerations.AdministrativeGender.NULL); 711 break; 712 } 713 } 714 return tgt; 715 } 716 717 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.AdministrativeGender> src) throws FHIRException { 718 if (src == null || src.isEmpty()) 719 return null; 720 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Enumerations.AdministrativeGenderEnumFactory()); 721 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 722 if (src.getValue() == null) { 723 tgt.setValue(null); 724 } else { 725 switch (src.getValue()) { 726 case MALE: 727 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.MALE); 728 break; 729 case FEMALE: 730 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.FEMALE); 731 break; 732 case OTHER: 733 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.OTHER); 734 break; 735 case UNKNOWN: 736 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.UNKNOWN); 737 break; 738 default: 739 tgt.setValue(org.hl7.fhir.r4.model.Enumerations.AdministrativeGender.NULL); 740 break; 741 } 742 } 743 return tgt; 744 } 745 746}