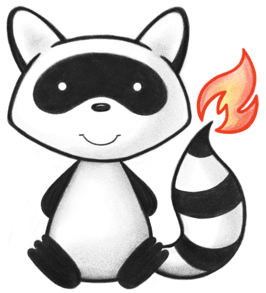
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Attachment40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.ContactPoint40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r4.model.HealthcareService; 014import org.hl7.fhir.r5.model.Enumeration; 015import org.hl7.fhir.r5.model.Enumerations; 016import org.hl7.fhir.r5.model.ExtendedContactDetail; 017 018/* 019 Copyright (c) 2011+, HL7, Inc. 020 All rights reserved. 021 022 Redistribution and use in source and binary forms, with or without modification, 023 are permitted provided that the following conditions are met: 024 025 * Redistributions of source code must retain the above copyright notice, this 026 list of conditions and the following disclaimer. 027 * Redistributions in binary form must reproduce the above copyright notice, 028 this list of conditions and the following disclaimer in the documentation 029 and/or other materials provided with the distribution. 030 * Neither the name of HL7 nor the names of its contributors may be used to 031 endorse or promote products derived from this software without specific 032 prior written permission. 033 034 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 035 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 036 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 037 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 038 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 039 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 040 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 041 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 042 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 043 POSSIBILITY OF SUCH DAMAGE. 044 045*/ 046// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 047public class HealthcareService40_50 { 048 049 public static org.hl7.fhir.r5.model.HealthcareService convertHealthcareService(org.hl7.fhir.r4.model.HealthcareService src) throws FHIRException { 050 if (src == null) 051 return null; 052 org.hl7.fhir.r5.model.HealthcareService tgt = new org.hl7.fhir.r5.model.HealthcareService(); 053 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 056 if (src.hasActive()) 057 tgt.setActiveElement(Boolean40_50.convertBoolean(src.getActiveElement())); 058 if (src.hasProvidedBy()) 059 tgt.setProvidedBy(Reference40_50.convertReference(src.getProvidedBy())); 060 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 061 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 062 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 063 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 064 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSpecialty()) 065 tgt.addSpecialty(CodeableConcept40_50.convertCodeableConcept(t)); 066 for (org.hl7.fhir.r4.model.Reference t : src.getLocation()) tgt.addLocation(Reference40_50.convertReference(t)); 067 if (src.hasName()) 068 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 069 if (src.hasComment()) 070 tgt.setCommentElement(String40_50.convertStringToMarkdown(src.getCommentElement())); 071 if (src.hasExtraDetails()) 072 tgt.setExtraDetailsElement(MarkDown40_50.convertMarkdown(src.getExtraDetailsElement())); 073 if (src.hasPhoto()) 074 tgt.setPhoto(Attachment40_50.convertAttachment(src.getPhoto())); 075 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 076 tgt.getContactFirstRep().addTelecom(ContactPoint40_50.convertContactPoint(t)); 077 for (org.hl7.fhir.r4.model.Reference t : src.getCoverageArea()) 078 tgt.addCoverageArea(Reference40_50.convertReference(t)); 079 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getServiceProvisionCode()) 080 tgt.addServiceProvisionCode(CodeableConcept40_50.convertCodeableConcept(t)); 081 for (org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceEligibilityComponent t : src.getEligibility()) 082 tgt.addEligibility(convertHealthcareServiceEligibilityComponent(t)); 083 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getProgram()) 084 tgt.addProgram(CodeableConcept40_50.convertCodeableConcept(t)); 085 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCharacteristic()) 086 tgt.addCharacteristic(CodeableConcept40_50.convertCodeableConcept(t)); 087 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCommunication()) 088 tgt.addCommunication(CodeableConcept40_50.convertCodeableConcept(t)); 089 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReferralMethod()) 090 tgt.addReferralMethod(CodeableConcept40_50.convertCodeableConcept(t)); 091 if (src.hasAppointmentRequired()) 092 tgt.setAppointmentRequiredElement(Boolean40_50.convertBoolean(src.getAppointmentRequiredElement())); 093// for (org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent t : src.getAvailableTime()) 094// tgt.addAvailableTime(convertHealthcareServiceAvailableTimeComponent(t)); 095// for (org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent t : src.getNotAvailable()) 096// tgt.addNotAvailable(convertHealthcareServiceNotAvailableComponent(t)); 097// if (src.hasAvailabilityExceptions()) 098// tgt.setAvailabilityExceptionsElement(String40_50.convertString(src.getAvailabilityExceptionsElement())); 099 for (org.hl7.fhir.r4.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference40_50.convertReference(t)); 100 return tgt; 101 } 102 103 public static org.hl7.fhir.r4.model.HealthcareService convertHealthcareService(org.hl7.fhir.r5.model.HealthcareService src) throws FHIRException { 104 if (src == null) 105 return null; 106 org.hl7.fhir.r4.model.HealthcareService tgt = new org.hl7.fhir.r4.model.HealthcareService(); 107 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 108 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 109 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 110 if (src.hasActive()) 111 tgt.setActiveElement(Boolean40_50.convertBoolean(src.getActiveElement())); 112 if (src.hasProvidedBy()) 113 tgt.setProvidedBy(Reference40_50.convertReference(src.getProvidedBy())); 114 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 115 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 116 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 117 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 118 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSpecialty()) 119 tgt.addSpecialty(CodeableConcept40_50.convertCodeableConcept(t)); 120 for (org.hl7.fhir.r5.model.Reference t : src.getLocation()) tgt.addLocation(Reference40_50.convertReference(t)); 121 if (src.hasName()) 122 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 123 if (src.hasComment()) 124 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 125 if (src.hasExtraDetails()) 126 tgt.setExtraDetailsElement(MarkDown40_50.convertMarkdown(src.getExtraDetailsElement())); 127 if (src.hasPhoto()) 128 tgt.setPhoto(Attachment40_50.convertAttachment(src.getPhoto())); 129 for (ExtendedContactDetail t1 : src.getContact()) 130 for (org.hl7.fhir.r5.model.ContactPoint t : t1.getTelecom()) 131 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 132 for (org.hl7.fhir.r5.model.Reference t : src.getCoverageArea()) 133 tgt.addCoverageArea(Reference40_50.convertReference(t)); 134 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getServiceProvisionCode()) 135 tgt.addServiceProvisionCode(CodeableConcept40_50.convertCodeableConcept(t)); 136 for (org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceEligibilityComponent t : src.getEligibility()) 137 tgt.addEligibility(convertHealthcareServiceEligibilityComponent(t)); 138 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getProgram()) 139 tgt.addProgram(CodeableConcept40_50.convertCodeableConcept(t)); 140 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCharacteristic()) 141 tgt.addCharacteristic(CodeableConcept40_50.convertCodeableConcept(t)); 142 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCommunication()) 143 tgt.addCommunication(CodeableConcept40_50.convertCodeableConcept(t)); 144 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getReferralMethod()) 145 tgt.addReferralMethod(CodeableConcept40_50.convertCodeableConcept(t)); 146 if (src.hasAppointmentRequired()) 147 tgt.setAppointmentRequiredElement(Boolean40_50.convertBoolean(src.getAppointmentRequiredElement())); 148// for (org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent t : src.getAvailableTime()) 149// tgt.addAvailableTime(convertHealthcareServiceAvailableTimeComponent(t)); 150// for (org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent t : src.getNotAvailable()) 151// tgt.addNotAvailable(convertHealthcareServiceNotAvailableComponent(t)); 152// if (src.hasAvailabilityExceptions()) 153// tgt.setAvailabilityExceptionsElement(String40_50.convertString(src.getAvailabilityExceptionsElement())); 154 for (org.hl7.fhir.r5.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference40_50.convertReference(t)); 155 return tgt; 156 } 157 158 public static org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceEligibilityComponent convertHealthcareServiceEligibilityComponent(org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceEligibilityComponent src) throws FHIRException { 159 if (src == null) 160 return null; 161 org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceEligibilityComponent tgt = new org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceEligibilityComponent(); 162 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 163 if (src.hasCode()) 164 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 165 if (src.hasComment()) 166 tgt.setCommentElement(MarkDown40_50.convertMarkdown(src.getCommentElement())); 167 return tgt; 168 } 169 170 public static org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceEligibilityComponent convertHealthcareServiceEligibilityComponent(org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceEligibilityComponent src) throws FHIRException { 171 if (src == null) 172 return null; 173 org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceEligibilityComponent tgt = new org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceEligibilityComponent(); 174 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 175 if (src.hasCode()) 176 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 177 if (src.hasComment()) 178 tgt.setCommentElement(MarkDown40_50.convertMarkdown(src.getCommentElement())); 179 return tgt; 180 } 181 182// public static org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent convertHealthcareServiceAvailableTimeComponent(org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent src) throws FHIRException { 183// if (src == null) 184// return null; 185// org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent tgt = new org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent(); 186// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 187// tgt.setDaysOfWeek(src.getDaysOfWeek().stream() 188// .map(HealthcareService40_50::convertDaysOfWeek) 189// .collect(Collectors.toList())); 190// if (src.hasAllDay()) 191// tgt.setAllDayElement(Boolean40_50.convertBoolean(src.getAllDayElement())); 192// if (src.hasAvailableStartTime()) 193// tgt.setAvailableStartTimeElement(Time40_50.convertTime(src.getAvailableStartTimeElement())); 194// if (src.hasAvailableEndTime()) 195// tgt.setAvailableEndTimeElement(Time40_50.convertTime(src.getAvailableEndTimeElement())); 196// return tgt; 197// } 198 199// public static org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent convertHealthcareServiceAvailableTimeComponent(org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceAvailableTimeComponent src) throws FHIRException { 200// if (src == null) 201// return null; 202// org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent tgt = new org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceAvailableTimeComponent(); 203// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 204// tgt.setDaysOfWeek(src.getDaysOfWeek().stream() 205// .map(HealthcareService40_50::convertDaysOfWeek) 206// .collect(Collectors.toList())); 207// if (src.hasAllDay()) 208// tgt.setAllDayElement(Boolean40_50.convertBoolean(src.getAllDayElement())); 209// if (src.hasAvailableStartTime()) 210// tgt.setAvailableStartTimeElement(Time40_50.convertTime(src.getAvailableStartTimeElement())); 211// if (src.hasAvailableEndTime()) 212// tgt.setAvailableEndTimeElement(Time40_50.convertTime(src.getAvailableEndTimeElement())); 213// return tgt; 214// } 215 216 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> convertDaysOfWeek(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.HealthcareService.DaysOfWeek> src) throws FHIRException { 217 if (src == null || src.isEmpty()) 218 return null; 219 Enumeration<Enumerations.DaysOfWeek> tgt = new Enumeration<>(new Enumerations.DaysOfWeekEnumFactory()); 220 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 221 if (src.getValue() == null) { 222 tgt.setValue(null); 223 } else { 224 switch (src.getValue()) { 225 case MON: 226 tgt.setValue(Enumerations.DaysOfWeek.MON); 227 break; 228 case TUE: 229 tgt.setValue(Enumerations.DaysOfWeek.TUE); 230 break; 231 case WED: 232 tgt.setValue(Enumerations.DaysOfWeek.WED); 233 break; 234 case THU: 235 tgt.setValue(Enumerations.DaysOfWeek.THU); 236 break; 237 case FRI: 238 tgt.setValue(Enumerations.DaysOfWeek.FRI); 239 break; 240 case SAT: 241 tgt.setValue(Enumerations.DaysOfWeek.SAT); 242 break; 243 case SUN: 244 tgt.setValue(Enumerations.DaysOfWeek.SUN); 245 break; 246 default: 247 tgt.setValue(Enumerations.DaysOfWeek.NULL); 248 break; 249 } 250 } 251 return tgt; 252 } 253 254 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.HealthcareService.DaysOfWeek> convertDaysOfWeek(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> src) throws FHIRException { 255 if (src == null || src.isEmpty()) 256 return null; 257 org.hl7.fhir.r4.model.Enumeration<HealthcareService.DaysOfWeek> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new HealthcareService.DaysOfWeekEnumFactory()); 258 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 259 if (src.getValue() == null) { 260 tgt.setValue(null); 261 } else { 262 switch (src.getValue()) { 263 case MON: 264 tgt.setValue(HealthcareService.DaysOfWeek.MON); 265 break; 266 case TUE: 267 tgt.setValue(HealthcareService.DaysOfWeek.TUE); 268 break; 269 case WED: 270 tgt.setValue(HealthcareService.DaysOfWeek.WED); 271 break; 272 case THU: 273 tgt.setValue(HealthcareService.DaysOfWeek.THU); 274 break; 275 case FRI: 276 tgt.setValue(HealthcareService.DaysOfWeek.FRI); 277 break; 278 case SAT: 279 tgt.setValue(HealthcareService.DaysOfWeek.SAT); 280 break; 281 case SUN: 282 tgt.setValue(HealthcareService.DaysOfWeek.SUN); 283 break; 284 default: 285 tgt.setValue(HealthcareService.DaysOfWeek.NULL); 286 break; 287 } 288 } 289 return tgt; 290 } 291// 292// public static org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent convertHealthcareServiceNotAvailableComponent(org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent src) throws FHIRException { 293// if (src == null) 294// return null; 295// org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent tgt = new org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent(); 296// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 297// if (src.hasDescription()) 298// tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 299// if (src.hasDuring()) 300// tgt.setDuring(Period40_50.convertPeriod(src.getDuring())); 301// return tgt; 302// } 303// 304// public static org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent convertHealthcareServiceNotAvailableComponent(org.hl7.fhir.r5.model.HealthcareService.HealthcareServiceNotAvailableComponent src) throws FHIRException { 305// if (src == null) 306// return null; 307// org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent tgt = new org.hl7.fhir.r4.model.HealthcareService.HealthcareServiceNotAvailableComponent(); 308// ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 309// if (src.hasDescription()) 310// tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 311// if (src.hasDuring()) 312// tgt.setDuring(Period40_50.convertPeriod(src.getDuring())); 313// return tgt; 314// } 315}