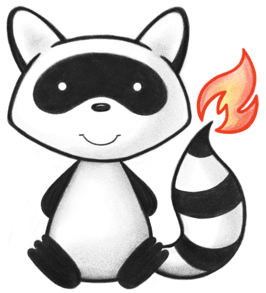
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Address40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.ContactPoint40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.HumanName40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Money40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Quantity40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.PositiveInt40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 014import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 015import org.hl7.fhir.exceptions.FHIRException; 016import org.hl7.fhir.r5.model.ExtendedContactDetail; 017 018/* 019 Copyright (c) 2011+, HL7, Inc. 020 All rights reserved. 021 022 Redistribution and use in source and binary forms, with or without modification, 023 are permitted provided that the following conditions are met: 024 025 * Redistributions of source code must retain the above copyright notice, this 026 list of conditions and the following disclaimer. 027 * Redistributions in binary form must reproduce the above copyright notice, 028 this list of conditions and the following disclaimer in the documentation 029 and/or other materials provided with the distribution. 030 * Neither the name of HL7 nor the names of its contributors may be used to 031 endorse or promote products derived from this software without specific 032 prior written permission. 033 034 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 035 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 036 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 037 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 038 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 039 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 040 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 041 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 042 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 043 POSSIBILITY OF SUCH DAMAGE. 044 045*/ 046// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 047public class InsurancePlan40_50 { 048 049 public static org.hl7.fhir.r5.model.InsurancePlan convertInsurancePlan(org.hl7.fhir.r4.model.InsurancePlan src) throws FHIRException { 050 if (src == null) 051 return null; 052 org.hl7.fhir.r5.model.InsurancePlan tgt = new org.hl7.fhir.r5.model.InsurancePlan(); 053 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 056 if (src.hasStatus()) 057 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 058 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 059 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 060 if (src.hasName()) 061 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 062 for (org.hl7.fhir.r4.model.StringType t : src.getAlias()) tgt.getAlias().add(String40_50.convertString(t)); 063 if (src.hasPeriod()) 064 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 065 if (src.hasOwnedBy()) 066 tgt.setOwnedBy(Reference40_50.convertReference(src.getOwnedBy())); 067 if (src.hasAdministeredBy()) 068 tgt.setAdministeredBy(Reference40_50.convertReference(src.getAdministeredBy())); 069 for (org.hl7.fhir.r4.model.Reference t : src.getCoverageArea()) 070 tgt.addCoverageArea(Reference40_50.convertReference(t)); 071 for (org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanContactComponent t : src.getContact()) 072 tgt.addContact(convertInsurancePlanContactComponent(t)); 073 for (org.hl7.fhir.r4.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference40_50.convertReference(t)); 074 for (org.hl7.fhir.r4.model.Reference t : src.getNetwork()) tgt.addNetwork(Reference40_50.convertReference(t)); 075 for (org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanCoverageComponent t : src.getCoverage()) 076 tgt.addCoverage(convertInsurancePlanCoverageComponent(t)); 077 for (org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanComponent t : src.getPlan()) 078 tgt.addPlan(convertInsurancePlanPlanComponent(t)); 079 return tgt; 080 } 081 082 public static org.hl7.fhir.r4.model.InsurancePlan convertInsurancePlan(org.hl7.fhir.r5.model.InsurancePlan src) throws FHIRException { 083 if (src == null) 084 return null; 085 org.hl7.fhir.r4.model.InsurancePlan tgt = new org.hl7.fhir.r4.model.InsurancePlan(); 086 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 087 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 088 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 089 if (src.hasStatus()) 090 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 091 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 092 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 093 if (src.hasName()) 094 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 095 for (org.hl7.fhir.r5.model.StringType t : src.getAlias()) tgt.getAlias().add(String40_50.convertString(t)); 096 if (src.hasPeriod()) 097 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 098 if (src.hasOwnedBy()) 099 tgt.setOwnedBy(Reference40_50.convertReference(src.getOwnedBy())); 100 if (src.hasAdministeredBy()) 101 tgt.setAdministeredBy(Reference40_50.convertReference(src.getAdministeredBy())); 102 for (org.hl7.fhir.r5.model.Reference t : src.getCoverageArea()) 103 tgt.addCoverageArea(Reference40_50.convertReference(t)); 104 for (ExtendedContactDetail t : src.getContact()) 105 tgt.addContact(convertInsurancePlanContactComponent(t)); 106 for (org.hl7.fhir.r5.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference40_50.convertReference(t)); 107 for (org.hl7.fhir.r5.model.Reference t : src.getNetwork()) tgt.addNetwork(Reference40_50.convertReference(t)); 108 for (org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanCoverageComponent t : src.getCoverage()) 109 tgt.addCoverage(convertInsurancePlanCoverageComponent(t)); 110 for (org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanComponent t : src.getPlan()) 111 tgt.addPlan(convertInsurancePlanPlanComponent(t)); 112 return tgt; 113 } 114 115 public static org.hl7.fhir.r5.model.ExtendedContactDetail convertInsurancePlanContactComponent(org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanContactComponent src) throws FHIRException { 116 if (src == null) 117 return null; 118 org.hl7.fhir.r5.model.ExtendedContactDetail tgt = new org.hl7.fhir.r5.model.ExtendedContactDetail(); 119 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 120 if (src.hasPurpose()) 121 tgt.setPurpose(CodeableConcept40_50.convertCodeableConcept(src.getPurpose())); 122 if (src.hasName()) 123 tgt.addName(HumanName40_50.convertHumanName(src.getName())); 124 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 125 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 126 if (src.hasAddress()) 127 tgt.setAddress(Address40_50.convertAddress(src.getAddress())); 128 return tgt; 129 } 130 131 public static org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanContactComponent convertInsurancePlanContactComponent(org.hl7.fhir.r5.model.ExtendedContactDetail src) throws FHIRException { 132 if (src == null) 133 return null; 134 org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanContactComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanContactComponent(); 135 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 136 if (src.hasPurpose()) 137 tgt.setPurpose(CodeableConcept40_50.convertCodeableConcept(src.getPurpose())); 138 if (src.hasName()) 139 tgt.setName(HumanName40_50.convertHumanName(src.getNameFirstRep())); 140 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 141 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 142 if (src.hasAddress()) 143 tgt.setAddress(Address40_50.convertAddress(src.getAddress())); 144 return tgt; 145 } 146 147 public static org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanCoverageComponent convertInsurancePlanCoverageComponent(org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanCoverageComponent src) throws FHIRException { 148 if (src == null) 149 return null; 150 org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanCoverageComponent tgt = new org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanCoverageComponent(); 151 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 152 if (src.hasType()) 153 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 154 for (org.hl7.fhir.r4.model.Reference t : src.getNetwork()) tgt.addNetwork(Reference40_50.convertReference(t)); 155 for (org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitComponent t : src.getBenefit()) 156 tgt.addBenefit(convertCoverageBenefitComponent(t)); 157 return tgt; 158 } 159 160 public static org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanCoverageComponent convertInsurancePlanCoverageComponent(org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanCoverageComponent src) throws FHIRException { 161 if (src == null) 162 return null; 163 org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanCoverageComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanCoverageComponent(); 164 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 165 if (src.hasType()) 166 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 167 for (org.hl7.fhir.r5.model.Reference t : src.getNetwork()) tgt.addNetwork(Reference40_50.convertReference(t)); 168 for (org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitComponent t : src.getBenefit()) 169 tgt.addBenefit(convertCoverageBenefitComponent(t)); 170 return tgt; 171 } 172 173 public static org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitComponent convertCoverageBenefitComponent(org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitComponent src) throws FHIRException { 174 if (src == null) 175 return null; 176 org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitComponent tgt = new org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitComponent(); 177 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 178 if (src.hasType()) 179 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 180 if (src.hasRequirement()) 181 tgt.setRequirementElement(String40_50.convertString(src.getRequirementElement())); 182 for (org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitLimitComponent t : src.getLimit()) 183 tgt.addLimit(convertCoverageBenefitLimitComponent(t)); 184 return tgt; 185 } 186 187 public static org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitComponent convertCoverageBenefitComponent(org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitComponent src) throws FHIRException { 188 if (src == null) 189 return null; 190 org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitComponent(); 191 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 192 if (src.hasType()) 193 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 194 if (src.hasRequirement()) 195 tgt.setRequirementElement(String40_50.convertString(src.getRequirementElement())); 196 for (org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitLimitComponent t : src.getLimit()) 197 tgt.addLimit(convertCoverageBenefitLimitComponent(t)); 198 return tgt; 199 } 200 201 public static org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitLimitComponent convertCoverageBenefitLimitComponent(org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitLimitComponent src) throws FHIRException { 202 if (src == null) 203 return null; 204 org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitLimitComponent tgt = new org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitLimitComponent(); 205 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 206 if (src.hasValue()) 207 tgt.setValue(Quantity40_50.convertQuantity(src.getValue())); 208 if (src.hasCode()) 209 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 210 return tgt; 211 } 212 213 public static org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitLimitComponent convertCoverageBenefitLimitComponent(org.hl7.fhir.r5.model.InsurancePlan.CoverageBenefitLimitComponent src) throws FHIRException { 214 if (src == null) 215 return null; 216 org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitLimitComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.CoverageBenefitLimitComponent(); 217 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 218 if (src.hasValue()) 219 tgt.setValue(Quantity40_50.convertQuantity(src.getValue())); 220 if (src.hasCode()) 221 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 222 return tgt; 223 } 224 225 public static org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanComponent convertInsurancePlanPlanComponent(org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanComponent src) throws FHIRException { 226 if (src == null) 227 return null; 228 org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanComponent tgt = new org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanComponent(); 229 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 230 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 231 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 232 if (src.hasType()) 233 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 234 for (org.hl7.fhir.r4.model.Reference t : src.getCoverageArea()) 235 tgt.addCoverageArea(Reference40_50.convertReference(t)); 236 for (org.hl7.fhir.r4.model.Reference t : src.getNetwork()) tgt.addNetwork(Reference40_50.convertReference(t)); 237 for (org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent t : src.getGeneralCost()) 238 tgt.addGeneralCost(convertInsurancePlanPlanGeneralCostComponent(t)); 239 for (org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent t : src.getSpecificCost()) 240 tgt.addSpecificCost(convertInsurancePlanPlanSpecificCostComponent(t)); 241 return tgt; 242 } 243 244 public static org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanComponent convertInsurancePlanPlanComponent(org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanComponent src) throws FHIRException { 245 if (src == null) 246 return null; 247 org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanComponent(); 248 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 249 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 250 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 251 if (src.hasType()) 252 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 253 for (org.hl7.fhir.r5.model.Reference t : src.getCoverageArea()) 254 tgt.addCoverageArea(Reference40_50.convertReference(t)); 255 for (org.hl7.fhir.r5.model.Reference t : src.getNetwork()) tgt.addNetwork(Reference40_50.convertReference(t)); 256 for (org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent t : src.getGeneralCost()) 257 tgt.addGeneralCost(convertInsurancePlanPlanGeneralCostComponent(t)); 258 for (org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent t : src.getSpecificCost()) 259 tgt.addSpecificCost(convertInsurancePlanPlanSpecificCostComponent(t)); 260 return tgt; 261 } 262 263 public static org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent convertInsurancePlanPlanGeneralCostComponent(org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent src) throws FHIRException { 264 if (src == null) 265 return null; 266 org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent tgt = new org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent(); 267 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 268 if (src.hasType()) 269 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 270 if (src.hasGroupSize()) 271 tgt.setGroupSizeElement(PositiveInt40_50.convertPositiveInt(src.getGroupSizeElement())); 272 if (src.hasCost()) 273 tgt.setCost(Money40_50.convertMoney(src.getCost())); 274 if (src.hasComment()) 275 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 276 return tgt; 277 } 278 279 public static org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent convertInsurancePlanPlanGeneralCostComponent(org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent src) throws FHIRException { 280 if (src == null) 281 return null; 282 org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanGeneralCostComponent(); 283 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 284 if (src.hasType()) 285 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 286 if (src.hasGroupSize()) 287 tgt.setGroupSizeElement(PositiveInt40_50.convertPositiveInt(src.getGroupSizeElement())); 288 if (src.hasCost()) 289 tgt.setCost(Money40_50.convertMoney(src.getCost())); 290 if (src.hasComment()) 291 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 292 return tgt; 293 } 294 295 public static org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent convertInsurancePlanPlanSpecificCostComponent(org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent src) throws FHIRException { 296 if (src == null) 297 return null; 298 org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent tgt = new org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent(); 299 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 300 if (src.hasCategory()) 301 tgt.setCategory(CodeableConcept40_50.convertCodeableConcept(src.getCategory())); 302 for (org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitComponent t : src.getBenefit()) 303 tgt.addBenefit(convertPlanBenefitComponent(t)); 304 return tgt; 305 } 306 307 public static org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent convertInsurancePlanPlanSpecificCostComponent(org.hl7.fhir.r5.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent src) throws FHIRException { 308 if (src == null) 309 return null; 310 org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.InsurancePlanPlanSpecificCostComponent(); 311 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 312 if (src.hasCategory()) 313 tgt.setCategory(CodeableConcept40_50.convertCodeableConcept(src.getCategory())); 314 for (org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitComponent t : src.getBenefit()) 315 tgt.addBenefit(convertPlanBenefitComponent(t)); 316 return tgt; 317 } 318 319 public static org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitComponent convertPlanBenefitComponent(org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitComponent src) throws FHIRException { 320 if (src == null) 321 return null; 322 org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitComponent tgt = new org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitComponent(); 323 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 324 if (src.hasType()) 325 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 326 for (org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitCostComponent t : src.getCost()) 327 tgt.addCost(convertPlanBenefitCostComponent(t)); 328 return tgt; 329 } 330 331 public static org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitComponent convertPlanBenefitComponent(org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitComponent src) throws FHIRException { 332 if (src == null) 333 return null; 334 org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitComponent(); 335 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 336 if (src.hasType()) 337 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 338 for (org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitCostComponent t : src.getCost()) 339 tgt.addCost(convertPlanBenefitCostComponent(t)); 340 return tgt; 341 } 342 343 public static org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitCostComponent convertPlanBenefitCostComponent(org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitCostComponent src) throws FHIRException { 344 if (src == null) 345 return null; 346 org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitCostComponent tgt = new org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitCostComponent(); 347 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 348 if (src.hasType()) 349 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 350 if (src.hasApplicability()) 351 tgt.setApplicability(CodeableConcept40_50.convertCodeableConcept(src.getApplicability())); 352 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getQualifiers()) 353 tgt.addQualifiers(CodeableConcept40_50.convertCodeableConcept(t)); 354 if (src.hasValue()) 355 tgt.setValue(Quantity40_50.convertQuantity(src.getValue())); 356 return tgt; 357 } 358 359 public static org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitCostComponent convertPlanBenefitCostComponent(org.hl7.fhir.r5.model.InsurancePlan.PlanBenefitCostComponent src) throws FHIRException { 360 if (src == null) 361 return null; 362 org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitCostComponent tgt = new org.hl7.fhir.r4.model.InsurancePlan.PlanBenefitCostComponent(); 363 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 364 if (src.hasType()) 365 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 366 if (src.hasApplicability()) 367 tgt.setApplicability(CodeableConcept40_50.convertCodeableConcept(src.getApplicability())); 368 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getQualifiers()) 369 tgt.addQualifiers(CodeableConcept40_50.convertCodeableConcept(t)); 370 if (src.hasValue()) 371 tgt.setValue(Quantity40_50.convertQuantity(src.getValue())); 372 return tgt; 373 } 374}