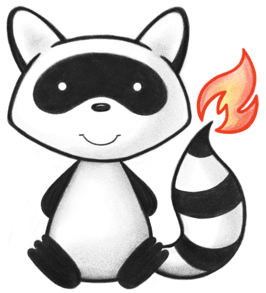
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Quantity40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Integer40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 012import org.hl7.fhir.exceptions.FHIRException; 013 014/* 015 Copyright (c) 2011+, HL7, Inc. 016 All rights reserved. 017 018 Redistribution and use in source and binary forms, with or without modification, 019 are permitted provided that the following conditions are met: 020 021 * Redistributions of source code must retain the above copyright notice, this 022 list of conditions and the following disclaimer. 023 * Redistributions in binary form must reproduce the above copyright notice, 024 this list of conditions and the following disclaimer in the documentation 025 and/or other materials provided with the distribution. 026 * Neither the name of HL7 nor the names of its contributors may be used to 027 endorse or promote products derived from this software without specific 028 prior written permission. 029 030 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 031 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 032 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 033 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 034 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 035 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 036 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 037 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 038 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 039 POSSIBILITY OF SUCH DAMAGE. 040 041*/ 042// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 043public class MeasureReport40_50 { 044 045 public static org.hl7.fhir.r5.model.MeasureReport convertMeasureReport(org.hl7.fhir.r4.model.MeasureReport src) throws FHIRException { 046 if (src == null) 047 return null; 048 org.hl7.fhir.r5.model.MeasureReport tgt = new org.hl7.fhir.r5.model.MeasureReport(); 049 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 050 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 051 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 052 if (src.hasStatus()) 053 tgt.setStatusElement(convertMeasureReportStatus(src.getStatusElement())); 054 if (src.hasType()) 055 tgt.setTypeElement(convertMeasureReportType(src.getTypeElement())); 056 if (src.hasMeasure()) 057 tgt.setMeasureElement(Canonical40_50.convertCanonical(src.getMeasureElement())); 058 if (src.hasSubject()) 059 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 060 if (src.hasDate()) 061 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 062 if (src.hasReporter()) 063 tgt.setReporter(Reference40_50.convertReference(src.getReporter())); 064 if (src.hasPeriod()) 065 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 066 if (src.hasImprovementNotation()) 067 tgt.setImprovementNotation(CodeableConcept40_50.convertCodeableConcept(src.getImprovementNotation())); 068 for (org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupComponent t : src.getGroup()) 069 tgt.addGroup(convertMeasureReportGroupComponent(t)); 070 for (org.hl7.fhir.r4.model.Reference t : src.getEvaluatedResource()) 071 tgt.addEvaluatedResource(Reference40_50.convertReference(t)); 072 return tgt; 073 } 074 075 public static org.hl7.fhir.r4.model.MeasureReport convertMeasureReport(org.hl7.fhir.r5.model.MeasureReport src) throws FHIRException { 076 if (src == null) 077 return null; 078 org.hl7.fhir.r4.model.MeasureReport tgt = new org.hl7.fhir.r4.model.MeasureReport(); 079 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 080 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 081 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 082 if (src.hasStatus()) 083 tgt.setStatusElement(convertMeasureReportStatus(src.getStatusElement())); 084 if (src.hasType()) 085 tgt.setTypeElement(convertMeasureReportType(src.getTypeElement())); 086 if (src.hasMeasure()) 087 tgt.setMeasureElement(Canonical40_50.convertCanonical(src.getMeasureElement())); 088 if (src.hasSubject()) 089 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 090 if (src.hasDate()) 091 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 092 if (src.hasReporter()) 093 tgt.setReporter(Reference40_50.convertReference(src.getReporter())); 094 if (src.hasPeriod()) 095 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 096 if (src.hasImprovementNotation()) 097 tgt.setImprovementNotation(CodeableConcept40_50.convertCodeableConcept(src.getImprovementNotation())); 098 for (org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupComponent t : src.getGroup()) 099 tgt.addGroup(convertMeasureReportGroupComponent(t)); 100 for (org.hl7.fhir.r5.model.Reference t : src.getEvaluatedResource()) 101 tgt.addEvaluatedResource(Reference40_50.convertReference(t)); 102 return tgt; 103 } 104 105 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MeasureReport.MeasureReportStatus> convertMeasureReportStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MeasureReport.MeasureReportStatus> src) throws FHIRException { 106 if (src == null || src.isEmpty()) 107 return null; 108 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MeasureReport.MeasureReportStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.MeasureReport.MeasureReportStatusEnumFactory()); 109 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 110 switch (src.getValue()) { 111 case COMPLETE: 112 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportStatus.COMPLETE); 113 break; 114 case PENDING: 115 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportStatus.PENDING); 116 break; 117 case ERROR: 118 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportStatus.ERROR); 119 break; 120 default: 121 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportStatus.NULL); 122 break; 123 } 124 return tgt; 125 } 126 127 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MeasureReport.MeasureReportStatus> convertMeasureReportStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MeasureReport.MeasureReportStatus> src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MeasureReport.MeasureReportStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.MeasureReport.MeasureReportStatusEnumFactory()); 131 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 132 switch (src.getValue()) { 133 case COMPLETE: 134 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportStatus.COMPLETE); 135 break; 136 case PENDING: 137 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportStatus.PENDING); 138 break; 139 case ERROR: 140 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportStatus.ERROR); 141 break; 142 default: 143 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportStatus.NULL); 144 break; 145 } 146 return tgt; 147 } 148 149 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MeasureReport.MeasureReportType> convertMeasureReportType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MeasureReport.MeasureReportType> src) throws FHIRException { 150 if (src == null || src.isEmpty()) 151 return null; 152 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MeasureReport.MeasureReportType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.MeasureReport.MeasureReportTypeEnumFactory()); 153 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 154 switch (src.getValue()) { 155 case INDIVIDUAL: 156 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportType.INDIVIDUAL); 157 break; 158 case SUBJECTLIST: 159 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportType.SUBJECTLIST); 160 break; 161 case SUMMARY: 162 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportType.SUMMARY); 163 break; 164 case DATACOLLECTION: 165 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportType.DATAEXCHANGE); 166 break; 167 default: 168 tgt.setValue(org.hl7.fhir.r5.model.MeasureReport.MeasureReportType.NULL); 169 break; 170 } 171 return tgt; 172 } 173 174 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MeasureReport.MeasureReportType> convertMeasureReportType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MeasureReport.MeasureReportType> src) throws FHIRException { 175 if (src == null || src.isEmpty()) 176 return null; 177 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MeasureReport.MeasureReportType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.MeasureReport.MeasureReportTypeEnumFactory()); 178 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 179 switch (src.getValue()) { 180 case INDIVIDUAL: 181 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportType.INDIVIDUAL); 182 break; 183 case SUBJECTLIST: 184 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportType.SUBJECTLIST); 185 break; 186 case SUMMARY: 187 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportType.SUMMARY); 188 break; 189 case DATAEXCHANGE: 190 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportType.DATACOLLECTION); 191 break; 192 default: 193 tgt.setValue(org.hl7.fhir.r4.model.MeasureReport.MeasureReportType.NULL); 194 break; 195 } 196 return tgt; 197 } 198 199 public static org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupComponent convertMeasureReportGroupComponent(org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupComponent src) throws FHIRException { 200 if (src == null) 201 return null; 202 org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupComponent tgt = new org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupComponent(); 203 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 204 if (src.hasCode()) 205 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 206 for (org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupPopulationComponent t : src.getPopulation()) 207 tgt.addPopulation(convertMeasureReportGroupPopulationComponent(t)); 208 if (src.hasMeasureScore()) 209 tgt.setMeasureScore(Quantity40_50.convertQuantity(src.getMeasureScore())); 210 for (org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupStratifierComponent t : src.getStratifier()) 211 tgt.addStratifier(convertMeasureReportGroupStratifierComponent(t)); 212 return tgt; 213 } 214 215 public static org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupComponent convertMeasureReportGroupComponent(org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupComponent src) throws FHIRException { 216 if (src == null) 217 return null; 218 org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupComponent tgt = new org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupComponent(); 219 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 220 if (src.hasCode()) 221 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 222 for (org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupPopulationComponent t : src.getPopulation()) 223 tgt.addPopulation(convertMeasureReportGroupPopulationComponent(t)); 224 if (src.hasMeasureScoreQuantity()) 225 tgt.setMeasureScore(Quantity40_50.convertQuantity(src.getMeasureScoreQuantity())); 226 for (org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupStratifierComponent t : src.getStratifier()) 227 tgt.addStratifier(convertMeasureReportGroupStratifierComponent(t)); 228 return tgt; 229 } 230 231 public static org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupPopulationComponent convertMeasureReportGroupPopulationComponent(org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupPopulationComponent src) throws FHIRException { 232 if (src == null) 233 return null; 234 org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupPopulationComponent tgt = new org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupPopulationComponent(); 235 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 236 if (src.hasCode()) 237 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 238 if (src.hasCount()) 239 tgt.setCountElement(Integer40_50.convertInteger(src.getCountElement())); 240 if (src.hasSubjectResults()) 241 tgt.setSubjectResults(Reference40_50.convertReference(src.getSubjectResults())); 242 return tgt; 243 } 244 245 public static org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupPopulationComponent convertMeasureReportGroupPopulationComponent(org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupPopulationComponent src) throws FHIRException { 246 if (src == null) 247 return null; 248 org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupPopulationComponent tgt = new org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupPopulationComponent(); 249 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 250 if (src.hasCode()) 251 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 252 if (src.hasCount()) 253 tgt.setCountElement(Integer40_50.convertInteger(src.getCountElement())); 254 if (src.hasSubjectResults()) 255 tgt.setSubjectResults(Reference40_50.convertReference(src.getSubjectResults())); 256 return tgt; 257 } 258 259 public static org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupStratifierComponent convertMeasureReportGroupStratifierComponent(org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupStratifierComponent src) throws FHIRException { 260 if (src == null) 261 return null; 262 org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupStratifierComponent tgt = new org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupStratifierComponent(); 263 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 264 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCode()) 265 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(t)); 266 for (org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponent t : src.getStratum()) 267 tgt.addStratum(convertStratifierGroupComponent(t)); 268 return tgt; 269 } 270 271 public static org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupStratifierComponent convertMeasureReportGroupStratifierComponent(org.hl7.fhir.r5.model.MeasureReport.MeasureReportGroupStratifierComponent src) throws FHIRException { 272 if (src == null) 273 return null; 274 org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupStratifierComponent tgt = new org.hl7.fhir.r4.model.MeasureReport.MeasureReportGroupStratifierComponent(); 275 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 276 tgt.addCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 277 for (org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponent t : src.getStratum()) 278 tgt.addStratum(convertStratifierGroupComponent(t)); 279 return tgt; 280 } 281 282 public static org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponent convertStratifierGroupComponent(org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponent src) throws FHIRException { 283 if (src == null) 284 return null; 285 org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponent tgt = new org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponent(); 286 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 287 if (src.hasValue()) 288 tgt.setValue(CodeableConcept40_50.convertCodeableConcept(src.getValue())); 289 for (org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponentComponent t : src.getComponent()) 290 tgt.addComponent(convertStratifierGroupComponentComponent(t)); 291 for (org.hl7.fhir.r4.model.MeasureReport.StratifierGroupPopulationComponent t : src.getPopulation()) 292 tgt.addPopulation(convertStratifierGroupPopulationComponent(t)); 293 if (src.hasMeasureScore()) 294 tgt.setMeasureScore(Quantity40_50.convertQuantity(src.getMeasureScore())); 295 return tgt; 296 } 297 298 public static org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponent convertStratifierGroupComponent(org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponent src) throws FHIRException { 299 if (src == null) 300 return null; 301 org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponent tgt = new org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponent(); 302 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 303 if (src.hasValueCodeableConcept()) 304 tgt.setValue(CodeableConcept40_50.convertCodeableConcept(src.getValueCodeableConcept())); 305 for (org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponentComponent t : src.getComponent()) 306 tgt.addComponent(convertStratifierGroupComponentComponent(t)); 307 for (org.hl7.fhir.r5.model.MeasureReport.StratifierGroupPopulationComponent t : src.getPopulation()) 308 tgt.addPopulation(convertStratifierGroupPopulationComponent(t)); 309 if (src.hasMeasureScoreQuantity()) 310 tgt.setMeasureScore(Quantity40_50.convertQuantity(src.getMeasureScoreQuantity())); 311 return tgt; 312 } 313 314 public static org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponentComponent convertStratifierGroupComponentComponent(org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponentComponent src) throws FHIRException { 315 if (src == null) 316 return null; 317 org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponentComponent tgt = new org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponentComponent(); 318 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 319 if (src.hasCode()) 320 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 321 if (src.hasValue()) 322 tgt.setValue(CodeableConcept40_50.convertCodeableConcept(src.getValue())); 323 return tgt; 324 } 325 326 public static org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponentComponent convertStratifierGroupComponentComponent(org.hl7.fhir.r5.model.MeasureReport.StratifierGroupComponentComponent src) throws FHIRException { 327 if (src == null) 328 return null; 329 org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponentComponent tgt = new org.hl7.fhir.r4.model.MeasureReport.StratifierGroupComponentComponent(); 330 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 331 if (src.hasCode()) 332 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 333 if (src.hasValueCodeableConcept()) 334 tgt.setValue(CodeableConcept40_50.convertCodeableConcept(src.getValueCodeableConcept())); 335 return tgt; 336 } 337 338 public static org.hl7.fhir.r5.model.MeasureReport.StratifierGroupPopulationComponent convertStratifierGroupPopulationComponent(org.hl7.fhir.r4.model.MeasureReport.StratifierGroupPopulationComponent src) throws FHIRException { 339 if (src == null) 340 return null; 341 org.hl7.fhir.r5.model.MeasureReport.StratifierGroupPopulationComponent tgt = new org.hl7.fhir.r5.model.MeasureReport.StratifierGroupPopulationComponent(); 342 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 343 if (src.hasCode()) 344 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 345 if (src.hasCount()) 346 tgt.setCountElement(Integer40_50.convertInteger(src.getCountElement())); 347 if (src.hasSubjectResults()) 348 tgt.setSubjectResults(Reference40_50.convertReference(src.getSubjectResults())); 349 return tgt; 350 } 351 352 public static org.hl7.fhir.r4.model.MeasureReport.StratifierGroupPopulationComponent convertStratifierGroupPopulationComponent(org.hl7.fhir.r5.model.MeasureReport.StratifierGroupPopulationComponent src) throws FHIRException { 353 if (src == null) 354 return null; 355 org.hl7.fhir.r4.model.MeasureReport.StratifierGroupPopulationComponent tgt = new org.hl7.fhir.r4.model.MeasureReport.StratifierGroupPopulationComponent(); 356 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 357 if (src.hasCode()) 358 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 359 if (src.hasCount()) 360 tgt.setCountElement(Integer40_50.convertInteger(src.getCountElement())); 361 if (src.hasSubjectResults()) 362 tgt.setSubjectResults(Reference40_50.convertReference(src.getSubjectResults())); 363 return tgt; 364 } 365}