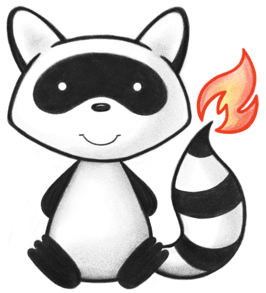
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Annotation40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Dosage40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.CodeableReference; 012import org.hl7.fhir.r5.model.Enumeration; 013import org.hl7.fhir.r5.model.MedicationStatement; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class MedicationStatement40_50 { 045 046 public static org.hl7.fhir.r5.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.r4.model.MedicationStatement src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.MedicationStatement tgt = new org.hl7.fhir.r5.model.MedicationStatement(); 050 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 053// for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 054// for (org.hl7.fhir.r4.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference40_50.convertReference(t)); 055 if (src.hasStatus()) 056 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 057// for (org.hl7.fhir.r4.model.CodeableConcept t : src.getStatusReason()) 058// tgt.addStatusReason(CodeableConcept40_50.convertCodeableConcept(t)); 059 if (src.hasCategory()) 060 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(src.getCategory())); 061 if (src.hasMedicationCodeableConcept()) { 062 tgt.getMedication().setConcept(CodeableConcept40_50.convertCodeableConcept(src.getMedicationCodeableConcept())); 063 } 064 if (src.hasMedicationReference()) { 065 tgt.getMedication().setReference(Reference40_50.convertReference(src.getMedicationReference())); 066 } 067 if (src.hasSubject()) 068 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 069 if (src.hasContext()) 070 tgt.setEncounter(Reference40_50.convertReference(src.getContext())); 071 if (src.hasEffective()) 072 tgt.setEffective(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getEffective())); 073 if (src.hasDateAsserted()) 074 tgt.setDateAssertedElement(DateTime40_50.convertDateTime(src.getDateAssertedElement())); 075 if (src.hasInformationSource()) 076 tgt.addInformationSource(Reference40_50.convertReference(src.getInformationSource())); 077 for (org.hl7.fhir.r4.model.Reference t : src.getDerivedFrom()) 078 tgt.addDerivedFrom(Reference40_50.convertReference(t)); 079 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 080 tgt.addReason(CodeableConcept40_50.convertCodeableConceptToCodeableReference(t)); 081 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 082 tgt.addReason(Reference40_50.convertReferenceToCodeableReference(t)); 083 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 084 for (org.hl7.fhir.r4.model.Dosage t : src.getDosage()) tgt.addDosage(Dosage40_50.convertDosage(t)); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.r4.model.MedicationStatement convertMedicationStatement(org.hl7.fhir.r5.model.MedicationStatement src) throws FHIRException { 089 if (src == null) 090 return null; 091 org.hl7.fhir.r4.model.MedicationStatement tgt = new org.hl7.fhir.r4.model.MedicationStatement(); 092 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 093 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 094 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 095// for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 096// for (org.hl7.fhir.r5.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference40_50.convertReference(t)); 097 if (src.hasStatus()) 098 tgt.setStatusElement(convertMedicationStatementStatus(src.getStatusElement())); 099// for (org.hl7.fhir.r5.model.CodeableConcept t : src.getStatusReason()) 100// tgt.addStatusReason(CodeableConcept40_50.convertCodeableConcept(t)); 101 if (src.hasCategory()) 102 tgt.setCategory(CodeableConcept40_50.convertCodeableConcept(src.getCategoryFirstRep())); 103 if (src.getMedication().hasConcept()) { 104 tgt.setMedication(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getMedication().getConcept())); 105 } 106 if (src.getMedication().hasReference()) { 107 tgt.setMedication(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getMedication().getReference())); 108 } 109 if (src.hasSubject()) 110 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 111 if (src.hasEncounter()) 112 tgt.setContext(Reference40_50.convertReference(src.getEncounter())); 113 if (src.hasEffective()) 114 tgt.setEffective(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getEffective())); 115 if (src.hasDateAsserted()) 116 tgt.setDateAssertedElement(DateTime40_50.convertDateTime(src.getDateAssertedElement())); 117 if (src.hasInformationSource()) 118 tgt.setInformationSource(Reference40_50.convertReference(src.getInformationSourceFirstRep())); 119 for (org.hl7.fhir.r5.model.Reference t : src.getDerivedFrom()) 120 tgt.addDerivedFrom(Reference40_50.convertReference(t)); 121 for (CodeableReference t : src.getReason()) 122 if (t.hasConcept()) 123 tgt.addReasonCode(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 124 for (CodeableReference t : src.getReason()) 125 if (t.hasReference()) 126 tgt.addReasonReference(Reference40_50.convertReference(t.getReference())); 127 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 128 for (org.hl7.fhir.r5.model.Dosage t : src.getDosage()) tgt.addDosage(Dosage40_50.convertDosage(t)); 129 return tgt; 130 } 131 132 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes> convertMedicationStatementStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus> src) throws FHIRException { 133 if (src == null || src.isEmpty()) 134 return null; 135 Enumeration<MedicationStatement.MedicationStatementStatusCodes> tgt = new Enumeration<>(new MedicationStatement.MedicationStatementStatusCodesEnumFactory()); 136 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 137 if (src.getValue() == null) { 138 tgt.setValue(null); 139 } else { 140 switch (src.getValue()) { 141 case ACTIVE: 142 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 143 break; 144 case COMPLETED: 145 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 146 break; 147 case ENTEREDINERROR: 148 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.ENTEREDINERROR); 149 break; 150 case INTENDED: 151 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 152 break; 153 case STOPPED: 154 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 155 break; 156 case ONHOLD: 157 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 158 break; 159 case UNKNOWN: 160 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 161 break; 162 case NOTTAKEN: 163 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.RECORDED); 164 break; 165 default: 166 tgt.setValue(MedicationStatement.MedicationStatementStatusCodes.NULL); 167 break; 168 } 169 } 170 return tgt; 171 } 172 173 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus> convertMedicationStatementStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.MedicationStatement.MedicationStatementStatusCodes> src) throws FHIRException { 174 if (src == null || src.isEmpty()) 175 return null; 176 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatusEnumFactory()); 177 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 178 if (src.getValue() == null) { 179 tgt.setValue(null); 180 } else { 181 switch (src.getValue()) { 182 // case ACTIVE: 183// tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.ACTIVE); 184// break; 185 case RECORDED: 186 tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.COMPLETED); 187 break; 188 case ENTEREDINERROR: 189 tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.ENTEREDINERROR); 190 break; 191// case UNKNOWN: 192// tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.INTENDED); 193// break; 194// case STOPPED: 195// tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.STOPPED); 196// break; 197// case ONHOLD: 198// tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.ONHOLD); 199// break; 200 case DRAFT: 201 tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.UNKNOWN); 202 break; 203// case NOTTAKEN: 204// tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.NOTTAKEN); 205// break; 206 default: 207 tgt.setValue(org.hl7.fhir.r4.model.MedicationStatement.MedicationStatementStatus.NULL); 208 break; 209 } 210 } 211 return tgt; 212 } 213}