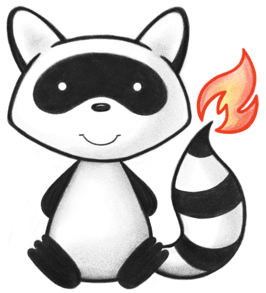
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.ContactDetail40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.UsageContext40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r4.model.Extension; 016import org.hl7.fhir.r4.model.StringType; 017import org.hl7.fhir.r4.model.UriType; 018import org.hl7.fhir.r5.model.Enumeration; 019import org.hl7.fhir.r5.model.NamingSystem; 020 021/* 022 Copyright (c) 2011+, HL7, Inc. 023 All rights reserved. 024 025 Redistribution and use in source and binary forms, with or without modification, 026 are permitted provided that the following conditions are met: 027 028 * Redistributions of source code must retain the above copyright notice, this 029 list of conditions and the following disclaimer. 030 * Redistributions in binary form must reproduce the above copyright notice, 031 this list of conditions and the following disclaimer in the documentation 032 and/or other materials provided with the distribution. 033 * Neither the name of HL7 nor the names of its contributors may be used to 034 endorse or promote products derived from this software without specific 035 prior written permission. 036 037 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 038 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 039 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 040 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 041 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 042 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 043 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 044 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 045 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 046 POSSIBILITY OF SUCH DAMAGE. 047 048*/ 049// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 050public class NamingSystem40_50 { 051 052 public static org.hl7.fhir.r5.model.NamingSystem convertNamingSystem(org.hl7.fhir.r4.model.NamingSystem src) throws FHIRException { 053 if (src == null) 054 return null; 055 056 org.hl7.fhir.r5.model.NamingSystem tgt = new org.hl7.fhir.r5.model.NamingSystem(); 057 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt, VersionConvertorConstants.EXT_NAMINGSYSTEM_URL, VersionConvertorConstants.EXT_NAMINGSYSTEM_VERSION, VersionConvertorConstants.EXT_NAMINGSYSTEM_TITLE); 058 059 if (src.getExtensionsByUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_URL).size() == 1) { 060 tgt.setUrlElement(Uri40_50.convertUri((UriType) src.getExtensionByUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_URL).getValue())); 061 } 062 if (src.hasExtension(VersionConvertorConstants.EXT_NAMINGSYSTEM_VERSION)) { 063 tgt.setVersionElement(String40_50.convertString((StringType) src.getExtensionByUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_VERSION).getValue())); 064 } 065 if (src.hasName()) 066 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 067 if (src.hasExtension(VersionConvertorConstants.EXT_NAMINGSYSTEM_TITLE)) { 068 tgt.setTitleElement(String40_50.convertString((StringType) src.getExtensionByUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_TITLE).getValue())); 069 } 070 071 if (src.hasStatus()) 072 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 073 if (src.hasKind()) 074 tgt.setKindElement(convertNamingSystemType(src.getKindElement())); 075 if (src.hasDate()) 076 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 077 if (src.hasPublisher()) 078 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 079 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 080 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 081 if (src.hasResponsible()) 082 tgt.setResponsibleElement(String40_50.convertString(src.getResponsibleElement())); 083 if (src.hasType()) 084 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 085 if (src.hasDescription()) 086 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 087 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 088 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 089 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 090 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 091 if (src.hasUsage()) 092 tgt.setUsageElement(String40_50.convertString(src.getUsageElement())); 093 for (org.hl7.fhir.r4.model.NamingSystem.NamingSystemUniqueIdComponent t : src.getUniqueId()) 094 tgt.addUniqueId(convertNamingSystemUniqueIdComponent(t)); 095 return tgt; 096 } 097 098 public static org.hl7.fhir.r4.model.NamingSystem convertNamingSystem(org.hl7.fhir.r5.model.NamingSystem src) throws FHIRException { 099 if (src == null) 100 return null; 101 org.hl7.fhir.r4.model.NamingSystem tgt = new org.hl7.fhir.r4.model.NamingSystem(); 102 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt, VersionConvertorConstants.EXT_NAMINGSYSTEM_URL); 103 if (src.hasUrlElement()) { 104 tgt.getExtension().add(new Extension().setUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_URL).setValue(Uri40_50.convertUri(src.getUrlElement()))); 105 } 106 if (src.hasVersionElement()) 107 tgt.getExtension().add(new Extension().setUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_VERSION).setValue(String40_50.convertString(src.getVersionElement()))); 108 if (src.hasName()) 109 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 110 if (src.hasTitleElement()) 111 tgt.getExtension().add(new Extension().setUrl(VersionConvertorConstants.EXT_NAMINGSYSTEM_TITLE).setValue(String40_50.convertString(src.getTitleElement()))); 112 113 if (src.hasStatus()) 114 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 115 if (src.hasKind()) 116 tgt.setKindElement(convertNamingSystemType(src.getKindElement())); 117 if (src.hasDate()) 118 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 119 if (src.hasPublisher()) 120 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 121 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 122 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 123 if (src.hasResponsible()) 124 tgt.setResponsibleElement(String40_50.convertString(src.getResponsibleElement())); 125 if (src.hasType()) 126 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 127 if (src.hasDescription()) 128 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 129 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 130 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 131 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 132 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 133 if (src.hasUsage()) 134 tgt.setUsageElement(String40_50.convertString(src.getUsageElement())); 135 for (org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent t : src.getUniqueId()) 136 tgt.addUniqueId(convertNamingSystemUniqueIdComponent(t)); 137 return tgt; 138 } 139 140 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.NamingSystem.NamingSystemType> convertNamingSystemType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.NamingSystem.NamingSystemType> src) throws FHIRException { 141 if (src == null || src.isEmpty()) 142 return null; 143 Enumeration<NamingSystem.NamingSystemType> tgt = new Enumeration<>(new NamingSystem.NamingSystemTypeEnumFactory()); 144 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 145 if (src.getValue() == null) { 146 tgt.setValue(null); 147 } else { 148 switch (src.getValue()) { 149 case CODESYSTEM: 150 tgt.setValue(NamingSystem.NamingSystemType.CODESYSTEM); 151 break; 152 case IDENTIFIER: 153 tgt.setValue(NamingSystem.NamingSystemType.IDENTIFIER); 154 break; 155 case ROOT: 156 tgt.setValue(NamingSystem.NamingSystemType.ROOT); 157 break; 158 default: 159 tgt.setValue(NamingSystem.NamingSystemType.NULL); 160 break; 161 } 162 } 163 return tgt; 164 } 165 166 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.NamingSystem.NamingSystemType> convertNamingSystemType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.NamingSystem.NamingSystemType> src) throws FHIRException { 167 if (src == null || src.isEmpty()) 168 return null; 169 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.NamingSystem.NamingSystemType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.NamingSystem.NamingSystemTypeEnumFactory()); 170 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 171 if (src.getValue() == null) { 172 tgt.setValue(null); 173 } else { 174 switch (src.getValue()) { 175 case CODESYSTEM: 176 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemType.CODESYSTEM); 177 break; 178 case IDENTIFIER: 179 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemType.IDENTIFIER); 180 break; 181 case ROOT: 182 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemType.ROOT); 183 break; 184 default: 185 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemType.NULL); 186 break; 187 } 188 } 189 return tgt; 190 } 191 192 public static org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent convertNamingSystemUniqueIdComponent(org.hl7.fhir.r4.model.NamingSystem.NamingSystemUniqueIdComponent src) throws FHIRException { 193 if (src == null) 194 return null; 195 org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent tgt = new org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent(); 196 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 197 if (src.hasType()) 198 tgt.setTypeElement(convertNamingSystemIdentifierType(src.getTypeElement())); 199 if (src.hasValue()) 200 tgt.setValueElement(String40_50.convertString(src.getValueElement())); 201 if (src.hasPreferred()) 202 tgt.setPreferredElement(Boolean40_50.convertBoolean(src.getPreferredElement())); 203 if (src.hasComment()) 204 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 205 if (src.hasPeriod()) 206 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 207 return tgt; 208 } 209 210 public static org.hl7.fhir.r4.model.NamingSystem.NamingSystemUniqueIdComponent convertNamingSystemUniqueIdComponent(org.hl7.fhir.r5.model.NamingSystem.NamingSystemUniqueIdComponent src) throws FHIRException { 211 if (src == null) 212 return null; 213 org.hl7.fhir.r4.model.NamingSystem.NamingSystemUniqueIdComponent tgt = new org.hl7.fhir.r4.model.NamingSystem.NamingSystemUniqueIdComponent(); 214 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 215 if (src.hasType()) 216 tgt.setTypeElement(convertNamingSystemIdentifierType(src.getTypeElement())); 217 if (src.hasValue()) 218 tgt.setValueElement(String40_50.convertString(src.getValueElement())); 219 if (src.hasPreferred()) 220 tgt.setPreferredElement(Boolean40_50.convertBoolean(src.getPreferredElement())); 221 if (src.hasComment()) 222 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 223 if (src.hasPeriod()) 224 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 225 return tgt; 226 } 227 228 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.NamingSystem.NamingSystemIdentifierType> convertNamingSystemIdentifierType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType> src) throws FHIRException { 229 if (src == null || src.isEmpty()) 230 return null; 231 Enumeration<NamingSystem.NamingSystemIdentifierType> tgt = new Enumeration<>(new NamingSystem.NamingSystemIdentifierTypeEnumFactory()); 232 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 233 if (src.getValue() == null) { 234 tgt.setValue(null); 235 } else { 236 switch (src.getValue()) { 237 case OID: 238 tgt.setValue(NamingSystem.NamingSystemIdentifierType.OID); 239 break; 240 case UUID: 241 tgt.setValue(NamingSystem.NamingSystemIdentifierType.UUID); 242 break; 243 case URI: 244 tgt.setValue(NamingSystem.NamingSystemIdentifierType.URI); 245 break; 246 case OTHER: 247 tgt.setValue(NamingSystem.NamingSystemIdentifierType.OTHER); 248 break; 249 default: 250 tgt.setValue(NamingSystem.NamingSystemIdentifierType.NULL); 251 break; 252 } 253 } 254 return tgt; 255 } 256 257 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType> convertNamingSystemIdentifierType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.NamingSystem.NamingSystemIdentifierType> src) throws FHIRException { 258 if (src == null || src.isEmpty()) 259 return null; 260 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierTypeEnumFactory()); 261 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 262 if (src.getValue() == null) { 263 tgt.setValue(null); 264 } else { 265 switch (src.getValue()) { 266 case OID: 267 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType.OID); 268 break; 269 case UUID: 270 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType.UUID); 271 break; 272 case URI: 273 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType.URI); 274 break; 275 case OTHER: 276 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType.OTHER); 277 break; 278 default: 279 tgt.setValue(org.hl7.fhir.r4.model.NamingSystem.NamingSystemIdentifierType.NULL); 280 break; 281 } 282 } 283 return tgt; 284 } 285}