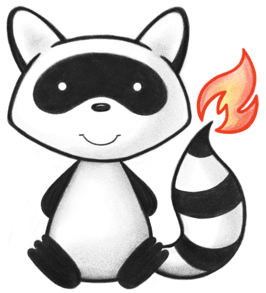
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Address40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.ContactPoint40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.HumanName40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.ExtendedContactDetail; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class Organization40_50 { 045 046 public static org.hl7.fhir.r5.model.Organization convertOrganization(org.hl7.fhir.r4.model.Organization src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.Organization tgt = new org.hl7.fhir.r5.model.Organization(); 050 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 053 if (src.hasActive()) 054 tgt.setActiveElement(Boolean40_50.convertBoolean(src.getActiveElement())); 055 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 056 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 057 if (src.hasName()) 058 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 059 for (org.hl7.fhir.r4.model.StringType t : src.getAlias()) tgt.getAlias().add(String40_50.convertString(t)); 060 for (org.hl7.fhir.r4.model.Address t : src.getAddress()) tgt.addContact().setAddress(Address40_50.convertAddress(t)); 061 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 062 tgt.getContactFirstRep().addTelecom(ContactPoint40_50.convertContactPoint(t)); 063 if (src.hasPartOf()) 064 tgt.setPartOf(Reference40_50.convertReference(src.getPartOf())); 065 for (org.hl7.fhir.r4.model.Organization.OrganizationContactComponent t : src.getContact()) 066 tgt.addContact(convertOrganizationContactComponent(t)); 067 for (org.hl7.fhir.r4.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference40_50.convertReference(t)); 068 return tgt; 069 } 070 071 public static org.hl7.fhir.r4.model.Organization convertOrganization(org.hl7.fhir.r5.model.Organization src) throws FHIRException { 072 if (src == null) 073 return null; 074 org.hl7.fhir.r4.model.Organization tgt = new org.hl7.fhir.r4.model.Organization(); 075 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 076 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 077 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 078 if (src.hasActive()) 079 tgt.setActiveElement(Boolean40_50.convertBoolean(src.getActiveElement())); 080 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 081 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 082 if (src.hasName()) 083 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 084 for (org.hl7.fhir.r5.model.StringType t : src.getAlias()) tgt.getAlias().add(String40_50.convertString(t)); 085 for (ExtendedContactDetail t1 : src.getContact()) 086 for (org.hl7.fhir.r5.model.ContactPoint t : t1.getTelecom()) 087 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 088 for (ExtendedContactDetail t : src.getContact()) 089 if (t.hasAddress()) 090 tgt.addAddress(Address40_50.convertAddress(t.getAddress())); 091 if (src.hasPartOf()) 092 tgt.setPartOf(Reference40_50.convertReference(src.getPartOf())); 093 for (org.hl7.fhir.r5.model.ExtendedContactDetail t : src.getContact()) 094 tgt.addContact(convertOrganizationContactComponent(t)); 095 for (org.hl7.fhir.r5.model.Reference t : src.getEndpoint()) tgt.addEndpoint(Reference40_50.convertReference(t)); 096 return tgt; 097 } 098 099 public static org.hl7.fhir.r5.model.ExtendedContactDetail convertOrganizationContactComponent(org.hl7.fhir.r4.model.Organization.OrganizationContactComponent src) throws FHIRException { 100 if (src == null) 101 return null; 102 org.hl7.fhir.r5.model.ExtendedContactDetail tgt = new org.hl7.fhir.r5.model.ExtendedContactDetail(); 103 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 104 if (src.hasPurpose()) 105 tgt.setPurpose(CodeableConcept40_50.convertCodeableConcept(src.getPurpose())); 106 if (src.hasName()) 107 tgt.addName(HumanName40_50.convertHumanName(src.getName())); 108 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 109 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 110 if (src.hasAddress()) 111 tgt.setAddress(Address40_50.convertAddress(src.getAddress())); 112 return tgt; 113 } 114 115 public static org.hl7.fhir.r4.model.Organization.OrganizationContactComponent convertOrganizationContactComponent(org.hl7.fhir.r5.model.ExtendedContactDetail src) throws FHIRException { 116 if (src == null) 117 return null; 118 org.hl7.fhir.r4.model.Organization.OrganizationContactComponent tgt = new org.hl7.fhir.r4.model.Organization.OrganizationContactComponent(); 119 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 120 if (src.hasPurpose()) 121 tgt.setPurpose(CodeableConcept40_50.convertCodeableConcept(src.getPurpose())); 122 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) 123 tgt.setName(HumanName40_50.convertHumanName(t)); 124 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 125 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 126 if (src.hasAddress()) 127 tgt.setAddress(Address40_50.convertAddress(src.getAddress())); 128 return tgt; 129 } 130}