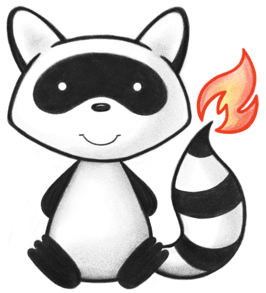
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Address40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Attachment40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.ContactPoint40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.HumanName40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Date40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 014import org.hl7.fhir.exceptions.FHIRException; 015import org.hl7.fhir.r5.model.Enumeration; 016import org.hl7.fhir.r5.model.Patient; 017 018/* 019 Copyright (c) 2011+, HL7, Inc. 020 All rights reserved. 021 022 Redistribution and use in source and binary forms, with or without modification, 023 are permitted provided that the following conditions are met: 024 025 * Redistributions of source code must retain the above copyright notice, this 026 list of conditions and the following disclaimer. 027 * Redistributions in binary form must reproduce the above copyright notice, 028 this list of conditions and the following disclaimer in the documentation 029 and/or other materials provided with the distribution. 030 * Neither the name of HL7 nor the names of its contributors may be used to 031 endorse or promote products derived from this software without specific 032 prior written permission. 033 034 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 035 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 036 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 037 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 038 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 039 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 040 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 041 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 042 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 043 POSSIBILITY OF SUCH DAMAGE. 044 045*/ 046// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 047public class Patient40_50 { 048 049 public static org.hl7.fhir.r5.model.Patient convertPatient(org.hl7.fhir.r4.model.Patient src) throws FHIRException { 050 if (src == null) 051 return null; 052 org.hl7.fhir.r5.model.Patient tgt = new org.hl7.fhir.r5.model.Patient(); 053 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 056 if (src.hasActive()) 057 tgt.setActiveElement(Boolean40_50.convertBoolean(src.getActiveElement())); 058 for (org.hl7.fhir.r4.model.HumanName t : src.getName()) tgt.addName(HumanName40_50.convertHumanName(t)); 059 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 060 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 061 if (src.hasGender()) 062 tgt.setGenderElement(Enumerations40_50.convertAdministrativeGender(src.getGenderElement())); 063 if (src.hasBirthDate()) 064 tgt.setBirthDateElement(Date40_50.convertDate(src.getBirthDateElement())); 065 if (src.hasDeceased()) 066 tgt.setDeceased(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getDeceased())); 067 for (org.hl7.fhir.r4.model.Address t : src.getAddress()) tgt.addAddress(Address40_50.convertAddress(t)); 068 if (src.hasMaritalStatus()) 069 tgt.setMaritalStatus(CodeableConcept40_50.convertCodeableConcept(src.getMaritalStatus())); 070 if (src.hasMultipleBirth()) 071 tgt.setMultipleBirth(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getMultipleBirth())); 072 for (org.hl7.fhir.r4.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment40_50.convertAttachment(t)); 073 for (org.hl7.fhir.r4.model.Patient.ContactComponent t : src.getContact()) 074 tgt.addContact(convertContactComponent(t)); 075 for (org.hl7.fhir.r4.model.Patient.PatientCommunicationComponent t : src.getCommunication()) 076 tgt.addCommunication(convertPatientCommunicationComponent(t)); 077 for (org.hl7.fhir.r4.model.Reference t : src.getGeneralPractitioner()) 078 tgt.addGeneralPractitioner(Reference40_50.convertReference(t)); 079 if (src.hasManagingOrganization()) 080 tgt.setManagingOrganization(Reference40_50.convertReference(src.getManagingOrganization())); 081 for (org.hl7.fhir.r4.model.Patient.PatientLinkComponent t : src.getLink()) 082 tgt.addLink(convertPatientLinkComponent(t)); 083 return tgt; 084 } 085 086 public static org.hl7.fhir.r4.model.Patient convertPatient(org.hl7.fhir.r5.model.Patient src) throws FHIRException { 087 if (src == null) 088 return null; 089 org.hl7.fhir.r4.model.Patient tgt = new org.hl7.fhir.r4.model.Patient(); 090 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 091 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 092 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 093 if (src.hasActive()) 094 tgt.setActiveElement(Boolean40_50.convertBoolean(src.getActiveElement())); 095 for (org.hl7.fhir.r5.model.HumanName t : src.getName()) tgt.addName(HumanName40_50.convertHumanName(t)); 096 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 097 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 098 if (src.hasGender()) 099 tgt.setGenderElement(Enumerations40_50.convertAdministrativeGender(src.getGenderElement())); 100 if (src.hasBirthDate()) 101 tgt.setBirthDateElement(Date40_50.convertDate(src.getBirthDateElement())); 102 if (src.hasDeceased()) 103 tgt.setDeceased(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getDeceased())); 104 for (org.hl7.fhir.r5.model.Address t : src.getAddress()) tgt.addAddress(Address40_50.convertAddress(t)); 105 if (src.hasMaritalStatus()) 106 tgt.setMaritalStatus(CodeableConcept40_50.convertCodeableConcept(src.getMaritalStatus())); 107 if (src.hasMultipleBirth()) 108 tgt.setMultipleBirth(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getMultipleBirth())); 109 for (org.hl7.fhir.r5.model.Attachment t : src.getPhoto()) tgt.addPhoto(Attachment40_50.convertAttachment(t)); 110 for (org.hl7.fhir.r5.model.Patient.ContactComponent t : src.getContact()) 111 tgt.addContact(convertContactComponent(t)); 112 for (org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent t : src.getCommunication()) 113 tgt.addCommunication(convertPatientCommunicationComponent(t)); 114 for (org.hl7.fhir.r5.model.Reference t : src.getGeneralPractitioner()) 115 tgt.addGeneralPractitioner(Reference40_50.convertReference(t)); 116 if (src.hasManagingOrganization()) 117 tgt.setManagingOrganization(Reference40_50.convertReference(src.getManagingOrganization())); 118 for (org.hl7.fhir.r5.model.Patient.PatientLinkComponent t : src.getLink()) 119 tgt.addLink(convertPatientLinkComponent(t)); 120 return tgt; 121 } 122 123 public static org.hl7.fhir.r5.model.Patient.ContactComponent convertContactComponent(org.hl7.fhir.r4.model.Patient.ContactComponent src) throws FHIRException { 124 if (src == null) 125 return null; 126 org.hl7.fhir.r5.model.Patient.ContactComponent tgt = new org.hl7.fhir.r5.model.Patient.ContactComponent(); 127 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 128 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getRelationship()) 129 tgt.addRelationship(CodeableConcept40_50.convertCodeableConcept(t)); 130 if (src.hasName()) 131 tgt.setName(HumanName40_50.convertHumanName(src.getName())); 132 for (org.hl7.fhir.r4.model.ContactPoint t : src.getTelecom()) 133 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 134 if (src.hasAddress()) 135 tgt.setAddress(Address40_50.convertAddress(src.getAddress())); 136 if (src.hasGender()) 137 tgt.setGenderElement(Enumerations40_50.convertAdministrativeGender(src.getGenderElement())); 138 if (src.hasOrganization()) 139 tgt.setOrganization(Reference40_50.convertReference(src.getOrganization())); 140 if (src.hasPeriod()) 141 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 142 return tgt; 143 } 144 145 public static org.hl7.fhir.r4.model.Patient.ContactComponent convertContactComponent(org.hl7.fhir.r5.model.Patient.ContactComponent src) throws FHIRException { 146 if (src == null) 147 return null; 148 org.hl7.fhir.r4.model.Patient.ContactComponent tgt = new org.hl7.fhir.r4.model.Patient.ContactComponent(); 149 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 150 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getRelationship()) 151 tgt.addRelationship(CodeableConcept40_50.convertCodeableConcept(t)); 152 if (src.hasName()) 153 tgt.setName(HumanName40_50.convertHumanName(src.getName())); 154 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 155 tgt.addTelecom(ContactPoint40_50.convertContactPoint(t)); 156 if (src.hasAddress()) 157 tgt.setAddress(Address40_50.convertAddress(src.getAddress())); 158 if (src.hasGender()) 159 tgt.setGenderElement(Enumerations40_50.convertAdministrativeGender(src.getGenderElement())); 160 if (src.hasOrganization()) 161 tgt.setOrganization(Reference40_50.convertReference(src.getOrganization())); 162 if (src.hasPeriod()) 163 tgt.setPeriod(Period40_50.convertPeriod(src.getPeriod())); 164 return tgt; 165 } 166 167 public static org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent convertPatientCommunicationComponent(org.hl7.fhir.r4.model.Patient.PatientCommunicationComponent src) throws FHIRException { 168 if (src == null) 169 return null; 170 org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent tgt = new org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent(); 171 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 172 if (src.hasLanguage()) 173 tgt.setLanguage(CodeableConcept40_50.convertCodeableConcept(src.getLanguage())); 174 if (src.hasPreferred()) 175 tgt.setPreferredElement(Boolean40_50.convertBoolean(src.getPreferredElement())); 176 return tgt; 177 } 178 179 public static org.hl7.fhir.r4.model.Patient.PatientCommunicationComponent convertPatientCommunicationComponent(org.hl7.fhir.r5.model.Patient.PatientCommunicationComponent src) throws FHIRException { 180 if (src == null) 181 return null; 182 org.hl7.fhir.r4.model.Patient.PatientCommunicationComponent tgt = new org.hl7.fhir.r4.model.Patient.PatientCommunicationComponent(); 183 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 184 if (src.hasLanguage()) 185 tgt.setLanguage(CodeableConcept40_50.convertCodeableConcept(src.getLanguage())); 186 if (src.hasPreferred()) 187 tgt.setPreferredElement(Boolean40_50.convertBoolean(src.getPreferredElement())); 188 return tgt; 189 } 190 191 public static org.hl7.fhir.r5.model.Patient.PatientLinkComponent convertPatientLinkComponent(org.hl7.fhir.r4.model.Patient.PatientLinkComponent src) throws FHIRException { 192 if (src == null) 193 return null; 194 org.hl7.fhir.r5.model.Patient.PatientLinkComponent tgt = new org.hl7.fhir.r5.model.Patient.PatientLinkComponent(); 195 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 196 if (src.hasOther()) 197 tgt.setOther(Reference40_50.convertReference(src.getOther())); 198 if (src.hasType()) 199 tgt.setTypeElement(convertLinkType(src.getTypeElement())); 200 return tgt; 201 } 202 203 public static org.hl7.fhir.r4.model.Patient.PatientLinkComponent convertPatientLinkComponent(org.hl7.fhir.r5.model.Patient.PatientLinkComponent src) throws FHIRException { 204 if (src == null) 205 return null; 206 org.hl7.fhir.r4.model.Patient.PatientLinkComponent tgt = new org.hl7.fhir.r4.model.Patient.PatientLinkComponent(); 207 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 208 if (src.hasOther()) 209 tgt.setOther(Reference40_50.convertReference(src.getOther())); 210 if (src.hasType()) 211 tgt.setTypeElement(convertLinkType(src.getTypeElement())); 212 return tgt; 213 } 214 215 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Patient.LinkType> convertLinkType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Patient.LinkType> src) throws FHIRException { 216 if (src == null || src.isEmpty()) 217 return null; 218 Enumeration<Patient.LinkType> tgt = new Enumeration<>(new Patient.LinkTypeEnumFactory()); 219 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 220 if (src.getValue() == null) { 221 tgt.setValue(null); 222 } else { 223 switch (src.getValue()) { 224 case REPLACEDBY: 225 tgt.setValue(Patient.LinkType.REPLACEDBY); 226 break; 227 case REPLACES: 228 tgt.setValue(Patient.LinkType.REPLACES); 229 break; 230 case REFER: 231 tgt.setValue(Patient.LinkType.REFER); 232 break; 233 case SEEALSO: 234 tgt.setValue(Patient.LinkType.SEEALSO); 235 break; 236 default: 237 tgt.setValue(Patient.LinkType.NULL); 238 break; 239 } 240 } 241 return tgt; 242 } 243 244 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Patient.LinkType> convertLinkType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Patient.LinkType> src) throws FHIRException { 245 if (src == null || src.isEmpty()) 246 return null; 247 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Patient.LinkType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Patient.LinkTypeEnumFactory()); 248 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 249 if (src.getValue() == null) { 250 tgt.setValue(null); 251 } else { 252 switch (src.getValue()) { 253 case REPLACEDBY: 254 tgt.setValue(org.hl7.fhir.r4.model.Patient.LinkType.REPLACEDBY); 255 break; 256 case REPLACES: 257 tgt.setValue(org.hl7.fhir.r4.model.Patient.LinkType.REPLACES); 258 break; 259 case REFER: 260 tgt.setValue(org.hl7.fhir.r4.model.Patient.LinkType.REFER); 261 break; 262 case SEEALSO: 263 tgt.setValue(org.hl7.fhir.r4.model.Patient.LinkType.SEEALSO); 264 break; 265 default: 266 tgt.setValue(org.hl7.fhir.r4.model.Patient.LinkType.NULL); 267 break; 268 } 269 } 270 return tgt; 271 } 272}