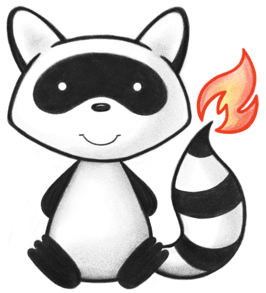
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Money40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Date40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r4.model.PaymentNotice; 012import org.hl7.fhir.r5.model.Enumeration; 013import org.hl7.fhir.r5.model.Enumerations; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class PaymentNotice40_50 { 045 046 public static org.hl7.fhir.r5.model.PaymentNotice convertPaymentNotice(org.hl7.fhir.r4.model.PaymentNotice src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.PaymentNotice tgt = new org.hl7.fhir.r5.model.PaymentNotice(); 050 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertPaymentNoticeStatus(src.getStatusElement())); 055 if (src.hasRequest()) 056 tgt.setRequest(Reference40_50.convertReference(src.getRequest())); 057 if (src.hasResponse()) 058 tgt.setResponse(Reference40_50.convertReference(src.getResponse())); 059 if (src.hasCreated()) 060 tgt.setCreatedElement(DateTime40_50.convertDateTime(src.getCreatedElement())); 061 if (src.hasProvider()) 062 tgt.setReporter(Reference40_50.convertReference(src.getProvider())); 063 if (src.hasPayment()) 064 tgt.setPayment(Reference40_50.convertReference(src.getPayment())); 065 if (src.hasPaymentDate()) 066 tgt.setPaymentDateElement(Date40_50.convertDate(src.getPaymentDateElement())); 067 if (src.hasPayee()) 068 tgt.setPayee(Reference40_50.convertReference(src.getPayee())); 069 if (src.hasRecipient()) 070 tgt.setRecipient(Reference40_50.convertReference(src.getRecipient())); 071 if (src.hasAmount()) 072 tgt.setAmount(Money40_50.convertMoney(src.getAmount())); 073 if (src.hasPaymentStatus()) 074 tgt.setPaymentStatus(CodeableConcept40_50.convertCodeableConcept(src.getPaymentStatus())); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.r4.model.PaymentNotice convertPaymentNotice(org.hl7.fhir.r5.model.PaymentNotice src) throws FHIRException { 079 if (src == null) 080 return null; 081 org.hl7.fhir.r4.model.PaymentNotice tgt = new org.hl7.fhir.r4.model.PaymentNotice(); 082 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 083 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 084 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 085 if (src.hasStatus()) 086 tgt.setStatusElement(convertPaymentNoticeStatus(src.getStatusElement())); 087 if (src.hasRequest()) 088 tgt.setRequest(Reference40_50.convertReference(src.getRequest())); 089 if (src.hasResponse()) 090 tgt.setResponse(Reference40_50.convertReference(src.getResponse())); 091 if (src.hasCreated()) 092 tgt.setCreatedElement(DateTime40_50.convertDateTime(src.getCreatedElement())); 093 if (src.hasReporter()) 094 tgt.setProvider(Reference40_50.convertReference(src.getReporter())); 095 if (src.hasPayment()) 096 tgt.setPayment(Reference40_50.convertReference(src.getPayment())); 097 if (src.hasPaymentDate()) 098 tgt.setPaymentDateElement(Date40_50.convertDate(src.getPaymentDateElement())); 099 if (src.hasPayee()) 100 tgt.setPayee(Reference40_50.convertReference(src.getPayee())); 101 if (src.hasRecipient()) 102 tgt.setRecipient(Reference40_50.convertReference(src.getRecipient())); 103 if (src.hasAmount()) 104 tgt.setAmount(Money40_50.convertMoney(src.getAmount())); 105 if (src.hasPaymentStatus()) 106 tgt.setPaymentStatus(CodeableConcept40_50.convertCodeableConcept(src.getPaymentStatus())); 107 return tgt; 108 } 109 110 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FinancialResourceStatusCodes> convertPaymentNoticeStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.PaymentNotice.PaymentNoticeStatus> src) throws FHIRException { 111 if (src == null || src.isEmpty()) 112 return null; 113 Enumeration<Enumerations.FinancialResourceStatusCodes> tgt = new Enumeration<>(new Enumerations.FinancialResourceStatusCodesEnumFactory()); 114 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 115 if (src.getValue() == null) { 116 tgt.setValue(null); 117 } else { 118 switch (src.getValue()) { 119 case ACTIVE: 120 tgt.setValue(Enumerations.FinancialResourceStatusCodes.ACTIVE); 121 break; 122 case CANCELLED: 123 tgt.setValue(Enumerations.FinancialResourceStatusCodes.CANCELLED); 124 break; 125 case DRAFT: 126 tgt.setValue(Enumerations.FinancialResourceStatusCodes.DRAFT); 127 break; 128 case ENTEREDINERROR: 129 tgt.setValue(Enumerations.FinancialResourceStatusCodes.ENTEREDINERROR); 130 break; 131 default: 132 tgt.setValue(Enumerations.FinancialResourceStatusCodes.NULL); 133 break; 134 } 135 } 136 return tgt; 137 } 138 139 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.PaymentNotice.PaymentNoticeStatus> convertPaymentNoticeStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FinancialResourceStatusCodes> src) throws FHIRException { 140 if (src == null || src.isEmpty()) 141 return null; 142 org.hl7.fhir.r4.model.Enumeration<PaymentNotice.PaymentNoticeStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new PaymentNotice.PaymentNoticeStatusEnumFactory()); 143 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 144 if (src.getValue() == null) { 145 tgt.setValue(null); 146 } else { 147 switch (src.getValue()) { 148 case ACTIVE: 149 tgt.setValue(PaymentNotice.PaymentNoticeStatus.ACTIVE); 150 break; 151 case CANCELLED: 152 tgt.setValue(PaymentNotice.PaymentNoticeStatus.CANCELLED); 153 break; 154 case DRAFT: 155 tgt.setValue(PaymentNotice.PaymentNoticeStatus.DRAFT); 156 break; 157 case ENTEREDINERROR: 158 tgt.setValue(PaymentNotice.PaymentNoticeStatus.ENTEREDINERROR); 159 break; 160 default: 161 tgt.setValue(PaymentNotice.PaymentNoticeStatus.NULL); 162 break; 163 } 164 } 165 return tgt; 166 } 167}