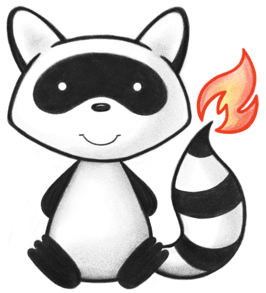
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Coding40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Period40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.ContactDetail40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.UsageContext40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Code40_50; 014import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Date40_50; 015import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 016import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Integer40_50; 017import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 018import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 019import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 020import org.hl7.fhir.exceptions.FHIRException; 021import org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType; 022import org.hl7.fhir.r5.model.CodeType; 023import org.hl7.fhir.r5.model.Enumeration; 024import org.hl7.fhir.r5.model.Questionnaire; 025import org.hl7.fhir.r5.model.Questionnaire.QuestionnaireAnswerConstraint; 026 027/* 028 Copyright (c) 2011+, HL7, Inc. 029 All rights reserved. 030 031 Redistribution and use in source and binary forms, with or without modification, 032 are permitted provided that the following conditions are met: 033 034 * Redistributions of source code must retain the above copyright notice, this 035 list of conditions and the following disclaimer. 036 * Redistributions in binary form must reproduce the above copyright notice, 037 this list of conditions and the following disclaimer in the documentation 038 and/or other materials provided with the distribution. 039 * Neither the name of HL7 nor the names of its contributors may be used to 040 endorse or promote products derived from this software without specific 041 prior written permission. 042 043 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 044 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 045 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 046 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 047 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 048 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 049 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 050 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 051 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 052 POSSIBILITY OF SUCH DAMAGE. 053 054*/ 055// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 056public class Questionnaire40_50 { 057 058 public static org.hl7.fhir.r5.model.Questionnaire convertQuestionnaire(org.hl7.fhir.r4.model.Questionnaire src) throws FHIRException { 059 if (src == null) 060 return null; 061 org.hl7.fhir.r5.model.Questionnaire tgt = new org.hl7.fhir.r5.model.Questionnaire(); 062 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 063 if (src.hasUrl()) 064 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 065 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 066 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 067 if (src.hasVersion()) 068 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 069 if (src.hasName()) 070 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 071 if (src.hasTitle()) 072 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 073 for (org.hl7.fhir.r4.model.CanonicalType t : src.getDerivedFrom()) 074 tgt.getDerivedFrom().add(Canonical40_50.convertCanonical(t)); 075 if (src.hasStatus()) 076 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 077 if (src.hasExperimental()) 078 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 079 for (org.hl7.fhir.r4.model.CodeType t : src.getSubjectType()) 080 tgt.getSubjectType().add(Code40_50.convertCode(t)); 081 if (src.hasDate()) 082 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 083 if (src.hasPublisher()) 084 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 085 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 086 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 087 if (src.hasDescription()) 088 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 089 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 090 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 091 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 092 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 093 if (src.hasPurpose()) 094 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 095 if (src.hasCopyright()) 096 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 097 if (src.hasApprovalDate()) 098 tgt.setApprovalDateElement(Date40_50.convertDate(src.getApprovalDateElement())); 099 if (src.hasLastReviewDate()) 100 tgt.setLastReviewDateElement(Date40_50.convertDate(src.getLastReviewDateElement())); 101 if (src.hasEffectivePeriod()) 102 tgt.setEffectivePeriod(Period40_50.convertPeriod(src.getEffectivePeriod())); 103 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addCode(Coding40_50.convertCoding(t)); 104 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 105 tgt.addItem(convertQuestionnaireItemComponent(t)); 106 return tgt; 107 } 108 109 public static org.hl7.fhir.r4.model.Questionnaire convertQuestionnaire(org.hl7.fhir.r5.model.Questionnaire src) throws FHIRException { 110 if (src == null) 111 return null; 112 org.hl7.fhir.r4.model.Questionnaire tgt = new org.hl7.fhir.r4.model.Questionnaire(); 113 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 114 if (src.hasUrl()) 115 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 116 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 117 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 118 if (src.hasVersion()) 119 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 120 if (src.hasName()) 121 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 122 if (src.hasTitle()) 123 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 124 for (org.hl7.fhir.r5.model.CanonicalType t : src.getDerivedFrom()) 125 tgt.getDerivedFrom().add(Canonical40_50.convertCanonical(t)); 126 if (src.hasStatus()) 127 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 128 if (src.hasExperimental()) 129 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 130 for (CodeType t : src.getSubjectType()) tgt.getSubjectType().add(Code40_50.convertCode(t)); 131 if (src.hasDate()) 132 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 133 if (src.hasPublisher()) 134 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 135 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 136 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 137 if (src.hasDescription()) 138 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 139 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 140 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 141 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 142 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 143 if (src.hasPurpose()) 144 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 145 if (src.hasCopyright()) 146 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 147 if (src.hasApprovalDate()) 148 tgt.setApprovalDateElement(Date40_50.convertDate(src.getApprovalDateElement())); 149 if (src.hasLastReviewDate()) 150 tgt.setLastReviewDateElement(Date40_50.convertDate(src.getLastReviewDateElement())); 151 if (src.hasEffectivePeriod()) 152 tgt.setEffectivePeriod(Period40_50.convertPeriod(src.getEffectivePeriod())); 153 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addCode(Coding40_50.convertCoding(t)); 154 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 155 tgt.addItem(convertQuestionnaireItemComponent(t)); 156 return tgt; 157 } 158 159 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 160 if (src == null) 161 return null; 162 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent(); 163 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 164 if (src.hasLinkId()) 165 tgt.setLinkIdElement(String40_50.convertString(src.getLinkIdElement())); 166 if (src.hasDefinition()) 167 tgt.setDefinitionElement(Uri40_50.convertUri(src.getDefinitionElement())); 168 for (org.hl7.fhir.r4.model.Coding t : src.getCode()) tgt.addCode(Coding40_50.convertCoding(t)); 169 if (src.hasPrefix()) 170 tgt.setPrefixElement(String40_50.convertString(src.getPrefixElement())); 171 if (src.hasText()) 172 tgt.setTextElement(MarkDown40_50.convertStringToMarkdown(src.getTextElement())); 173 if (src.hasType()) { 174 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement())); 175 if (src.getType() == QuestionnaireItemType.CHOICE) { 176 tgt.setAnswerConstraint(QuestionnaireAnswerConstraint.OPTIONSONLY); 177 } else if (src.getType() == QuestionnaireItemType.OPENCHOICE) { 178 tgt.setAnswerConstraint(QuestionnaireAnswerConstraint.OPTIONSORSTRING); 179 } 180 } 181 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 182 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 183 if (src.hasEnableBehavior()) 184 tgt.setEnableBehaviorElement(convertEnableWhenBehavior(src.getEnableBehaviorElement())); 185 if (src.hasRequired()) 186 tgt.setRequiredElement(Boolean40_50.convertBoolean(src.getRequiredElement())); 187 if (src.hasRepeats()) 188 tgt.setRepeatsElement(Boolean40_50.convertBoolean(src.getRepeatsElement())); 189 if (src.hasReadOnly()) 190 tgt.setReadOnlyElement(Boolean40_50.convertBoolean(src.getReadOnlyElement())); 191 if (src.hasMaxLength()) 192 tgt.setMaxLengthElement(Integer40_50.convertInteger(src.getMaxLengthElement())); 193 if (src.hasAnswerValueSet()) 194 tgt.setAnswerValueSetElement(Canonical40_50.convertCanonical(src.getAnswerValueSetElement())); 195 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent t : src.getAnswerOption()) 196 tgt.addAnswerOption(convertQuestionnaireItemAnswerOptionComponent(t)); 197 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemInitialComponent t : src.getInitial()) 198 tgt.addInitial(convertQuestionnaireItemInitialComponent(t)); 199 for (org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 200 tgt.addItem(convertQuestionnaireItemComponent(t)); 201 return tgt; 202 } 203 204 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent convertQuestionnaireItemComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent src) throws FHIRException { 205 if (src == null) 206 return null; 207 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemComponent(); 208 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 209 if (src.hasLinkId()) 210 tgt.setLinkIdElement(String40_50.convertString(src.getLinkIdElement())); 211 if (src.hasDefinition()) 212 tgt.setDefinitionElement(Uri40_50.convertUri(src.getDefinitionElement())); 213 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addCode(Coding40_50.convertCoding(t)); 214 if (src.hasPrefix()) 215 tgt.setPrefixElement(String40_50.convertString(src.getPrefixElement())); 216 if (src.hasText()) 217 tgt.setTextElement(String40_50.convertString(src.getTextElement())); 218 if (src.hasType()) 219 tgt.setTypeElement(convertQuestionnaireItemType(src.getTypeElement(), src.getAnswerConstraint())); 220 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent t : src.getEnableWhen()) 221 tgt.addEnableWhen(convertQuestionnaireItemEnableWhenComponent(t)); 222 if (src.hasEnableBehavior()) 223 tgt.setEnableBehaviorElement(convertEnableWhenBehavior(src.getEnableBehaviorElement())); 224 if (src.hasRequired()) 225 tgt.setRequiredElement(Boolean40_50.convertBoolean(src.getRequiredElement())); 226 if (src.hasRepeats()) 227 tgt.setRepeatsElement(Boolean40_50.convertBoolean(src.getRepeatsElement())); 228 if (src.hasReadOnly()) 229 tgt.setReadOnlyElement(Boolean40_50.convertBoolean(src.getReadOnlyElement())); 230 if (src.hasMaxLength()) 231 tgt.setMaxLengthElement(Integer40_50.convertInteger(src.getMaxLengthElement())); 232 if (src.hasAnswerValueSet()) 233 tgt.setAnswerValueSetElement(Canonical40_50.convertCanonical(src.getAnswerValueSetElement())); 234 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent t : src.getAnswerOption()) 235 tgt.addAnswerOption(convertQuestionnaireItemAnswerOptionComponent(t)); 236 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemInitialComponent t : src.getInitial()) 237 tgt.addInitial(convertQuestionnaireItemInitialComponent(t)); 238 for (org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemComponent t : src.getItem()) 239 tgt.addItem(convertQuestionnaireItemComponent(t)); 240 return tgt; 241 } 242 243 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> src) throws FHIRException { 244 if (src == null || src.isEmpty()) 245 return null; 246 Enumeration<Questionnaire.QuestionnaireItemType> tgt = new Enumeration<>(new Questionnaire.QuestionnaireItemTypeEnumFactory()); 247 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 248 tgt.addExtension(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL, new CodeType(src.getValueAsString())); 249 if (src.getValue() == null) { 250 tgt.setValue(null); 251 } else { 252 switch (src.getValue()) { 253 case GROUP: 254 tgt.setValue(Questionnaire.QuestionnaireItemType.GROUP); 255 break; 256 case DISPLAY: 257 tgt.setValue(Questionnaire.QuestionnaireItemType.DISPLAY); 258 break; 259 case QUESTION: 260 tgt.setValue(Questionnaire.QuestionnaireItemType.GROUP); 261 break; 262 case BOOLEAN: 263 tgt.setValue(Questionnaire.QuestionnaireItemType.BOOLEAN); 264 break; 265 case DECIMAL: 266 tgt.setValue(Questionnaire.QuestionnaireItemType.DECIMAL); 267 break; 268 case INTEGER: 269 tgt.setValue(Questionnaire.QuestionnaireItemType.INTEGER); 270 break; 271 case DATE: 272 tgt.setValue(Questionnaire.QuestionnaireItemType.DATE); 273 break; 274 case DATETIME: 275 tgt.setValue(Questionnaire.QuestionnaireItemType.DATETIME); 276 break; 277 case TIME: 278 tgt.setValue(Questionnaire.QuestionnaireItemType.TIME); 279 break; 280 case STRING: 281 tgt.setValue(Questionnaire.QuestionnaireItemType.STRING); 282 break; 283 case TEXT: 284 tgt.setValue(Questionnaire.QuestionnaireItemType.TEXT); 285 break; 286 case URL: 287 tgt.setValue(Questionnaire.QuestionnaireItemType.URL); 288 break; 289 case CHOICE: 290 tgt.setValue(Questionnaire.QuestionnaireItemType.CODING); 291 break; 292 case OPENCHOICE: 293 tgt.setValue(Questionnaire.QuestionnaireItemType.CODING); 294 break; 295 case ATTACHMENT: 296 tgt.setValue(Questionnaire.QuestionnaireItemType.ATTACHMENT); 297 break; 298 case REFERENCE: 299 tgt.setValue(Questionnaire.QuestionnaireItemType.REFERENCE); 300 break; 301 case QUANTITY: 302 tgt.setValue(Questionnaire.QuestionnaireItemType.QUANTITY); 303 break; 304 default: 305 tgt.setValue(Questionnaire.QuestionnaireItemType.NULL); 306 break; 307 } 308 } 309 return tgt; 310 } 311 312 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> convertQuestionnaireItemType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemType> src, QuestionnaireAnswerConstraint constraint) throws FHIRException { 313 if (src == null || src.isEmpty()) 314 return null; 315 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemTypeEnumFactory()); 316 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt, VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL); 317 if (src.hasExtension(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL)) { 318 tgt.setValueAsString(src.getExtensionString(VersionConvertorConstants.EXT_QUESTIONNAIRE_ITEM_TYPE_ORIGINAL)); 319 } else { 320 if (src.getValue() == null) { 321 tgt.setValue(null); 322 } else { 323 switch (src.getValue()) { 324 case GROUP: 325 tgt.setValue(QuestionnaireItemType.GROUP); 326 break; 327 case DISPLAY: 328 tgt.setValue(QuestionnaireItemType.DISPLAY); 329 break; 330 // case QUESTION: return org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemType.QUESTION; 331 case BOOLEAN: 332 tgt.setValue(QuestionnaireItemType.BOOLEAN); 333 break; 334 case DECIMAL: 335 tgt.setValue(QuestionnaireItemType.DECIMAL); 336 break; 337 case INTEGER: 338 tgt.setValue(QuestionnaireItemType.INTEGER); 339 break; 340 case DATE: 341 tgt.setValue(QuestionnaireItemType.DATE); 342 break; 343 case DATETIME: 344 tgt.setValue(QuestionnaireItemType.DATETIME); 345 break; 346 case TIME: 347 tgt.setValue(QuestionnaireItemType.TIME); 348 break; 349 case STRING: 350 tgt.setValue(QuestionnaireItemType.STRING); 351 break; 352 case TEXT: 353 tgt.setValue(QuestionnaireItemType.TEXT); 354 break; 355 case URL: 356 tgt.setValue(QuestionnaireItemType.URL); 357 break; 358 case CODING: 359 if (constraint == QuestionnaireAnswerConstraint.OPTIONSORSTRING) 360 tgt.setValue(QuestionnaireItemType.OPENCHOICE); 361 else 362 tgt.setValue(QuestionnaireItemType.CHOICE); 363 break; 364 case ATTACHMENT: 365 tgt.setValue(QuestionnaireItemType.ATTACHMENT); 366 break; 367 case REFERENCE: 368 tgt.setValue(QuestionnaireItemType.REFERENCE); 369 break; 370 case QUANTITY: 371 tgt.setValue(QuestionnaireItemType.QUANTITY); 372 break; 373 default: 374 tgt.setValue(QuestionnaireItemType.NULL); 375 break; 376 } 377 } 378 } 379 return tgt; 380 } 381 382 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.EnableWhenBehavior> convertEnableWhenBehavior(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.EnableWhenBehavior> src) throws FHIRException { 383 if (src == null || src.isEmpty()) 384 return null; 385 Enumeration<Questionnaire.EnableWhenBehavior> tgt = new Enumeration<>(new Questionnaire.EnableWhenBehaviorEnumFactory()); 386 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 387 if (src.getValue() == null) { 388 tgt.setValue(null); 389 } else { 390 switch (src.getValue()) { 391 case ALL: 392 tgt.setValue(Questionnaire.EnableWhenBehavior.ALL); 393 break; 394 case ANY: 395 tgt.setValue(Questionnaire.EnableWhenBehavior.ANY); 396 break; 397 default: 398 tgt.setValue(Questionnaire.EnableWhenBehavior.NULL); 399 break; 400 } 401 } 402 return tgt; 403 } 404 405 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.EnableWhenBehavior> convertEnableWhenBehavior(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.EnableWhenBehavior> src) throws FHIRException { 406 if (src == null || src.isEmpty()) 407 return null; 408 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.EnableWhenBehavior> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Questionnaire.EnableWhenBehaviorEnumFactory()); 409 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 410 if (src.getValue() == null) { 411 tgt.setValue(null); 412 } else { 413 switch (src.getValue()) { 414 case ALL: 415 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.EnableWhenBehavior.ALL); 416 break; 417 case ANY: 418 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.EnableWhenBehavior.ANY); 419 break; 420 default: 421 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.EnableWhenBehavior.NULL); 422 break; 423 } 424 } 425 return tgt; 426 } 427 428 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 429 if (src == null) 430 return null; 431 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 432 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 433 if (src.hasQuestion()) 434 tgt.setQuestionElement(String40_50.convertString(src.getQuestionElement())); 435 if (src.hasOperator()) 436 tgt.setOperatorElement(convertQuestionnaireItemOperator(src.getOperatorElement())); 437 if (src.hasAnswer()) 438 tgt.setAnswer(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getAnswer())); 439 return tgt; 440 } 441 442 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent convertQuestionnaireItemEnableWhenComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemEnableWhenComponent src) throws FHIRException { 443 if (src == null) 444 return null; 445 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemEnableWhenComponent(); 446 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 447 if (src.hasQuestion()) 448 tgt.setQuestionElement(String40_50.convertString(src.getQuestionElement())); 449 if (src.hasOperator()) 450 tgt.setOperatorElement(convertQuestionnaireItemOperator(src.getOperatorElement())); 451 if (src.hasAnswer()) 452 tgt.setAnswer(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getAnswer())); 453 return tgt; 454 } 455 456 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemOperator> convertQuestionnaireItemOperator(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator> src) throws FHIRException { 457 if (src == null || src.isEmpty()) 458 return null; 459 Enumeration<Questionnaire.QuestionnaireItemOperator> tgt = new Enumeration<>(new Questionnaire.QuestionnaireItemOperatorEnumFactory()); 460 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 461 if (src.getValue() == null) { 462 tgt.setValue(null); 463 } else { 464 switch (src.getValue()) { 465 case EXISTS: 466 tgt.setValue(Questionnaire.QuestionnaireItemOperator.EXISTS); 467 break; 468 case EQUAL: 469 tgt.setValue(Questionnaire.QuestionnaireItemOperator.EQUAL); 470 break; 471 case NOT_EQUAL: 472 tgt.setValue(Questionnaire.QuestionnaireItemOperator.NOT_EQUAL); 473 break; 474 case GREATER_THAN: 475 tgt.setValue(Questionnaire.QuestionnaireItemOperator.GREATER_THAN); 476 break; 477 case LESS_THAN: 478 tgt.setValue(Questionnaire.QuestionnaireItemOperator.LESS_THAN); 479 break; 480 case GREATER_OR_EQUAL: 481 tgt.setValue(Questionnaire.QuestionnaireItemOperator.GREATER_OR_EQUAL); 482 break; 483 case LESS_OR_EQUAL: 484 tgt.setValue(Questionnaire.QuestionnaireItemOperator.LESS_OR_EQUAL); 485 break; 486 default: 487 tgt.setValue(Questionnaire.QuestionnaireItemOperator.NULL); 488 break; 489 } 490 } 491 return tgt; 492 } 493 494 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator> convertQuestionnaireItemOperator(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemOperator> src) throws FHIRException { 495 if (src == null || src.isEmpty()) 496 return null; 497 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperatorEnumFactory()); 498 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 499 if (src.getValue() == null) { 500 tgt.setValue(null); 501 } else { 502 switch (src.getValue()) { 503 case EXISTS: 504 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.EXISTS); 505 break; 506 case EQUAL: 507 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.EQUAL); 508 break; 509 case NOT_EQUAL: 510 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.NOT_EQUAL); 511 break; 512 case GREATER_THAN: 513 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.GREATER_THAN); 514 break; 515 case LESS_THAN: 516 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.LESS_THAN); 517 break; 518 case GREATER_OR_EQUAL: 519 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.GREATER_OR_EQUAL); 520 break; 521 case LESS_OR_EQUAL: 522 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.LESS_OR_EQUAL); 523 break; 524 default: 525 tgt.setValue(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemOperator.NULL); 526 break; 527 } 528 } 529 return tgt; 530 } 531 532 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent convertQuestionnaireItemAnswerOptionComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent src) throws FHIRException { 533 if (src == null) 534 return null; 535 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent(); 536 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 537 if (src.hasValue()) 538 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 539 if (src.hasInitialSelected()) 540 tgt.setInitialSelectedElement(Boolean40_50.convertBoolean(src.getInitialSelectedElement())); 541 return tgt; 542 } 543 544 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent convertQuestionnaireItemAnswerOptionComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemAnswerOptionComponent src) throws FHIRException { 545 if (src == null) 546 return null; 547 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemAnswerOptionComponent(); 548 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 549 if (src.hasValue()) 550 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 551 if (src.hasInitialSelected()) 552 tgt.setInitialSelectedElement(Boolean40_50.convertBoolean(src.getInitialSelectedElement())); 553 return tgt; 554 } 555 556 public static org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemInitialComponent convertQuestionnaireItemInitialComponent(org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemInitialComponent src) throws FHIRException { 557 if (src == null) 558 return null; 559 org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemInitialComponent tgt = new org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemInitialComponent(); 560 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 561 if (src.hasValue()) 562 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 563 return tgt; 564 } 565 566 public static org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemInitialComponent convertQuestionnaireItemInitialComponent(org.hl7.fhir.r5.model.Questionnaire.QuestionnaireItemInitialComponent src) throws FHIRException { 567 if (src == null) 568 return null; 569 org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemInitialComponent tgt = new org.hl7.fhir.r4.model.Questionnaire.QuestionnaireItemInitialComponent(); 570 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 571 if (src.hasValue()) 572 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 573 return tgt; 574 } 575}