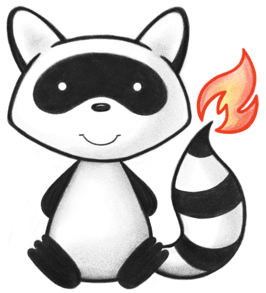
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.ContactDetail40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.UsageContext40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Code40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 014import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 015import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 016import org.hl7.fhir.exceptions.FHIRException; 017import org.hl7.fhir.r5.model.Enumeration; 018import org.hl7.fhir.r5.model.Enumerations; 019import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAll; 020import org.hl7.fhir.r5.model.Enumerations.VersionIndependentResourceTypesAllEnumFactory; 021import org.hl7.fhir.r5.model.SearchParameter; 022 023/* 024 Copyright (c) 2011+, HL7, Inc. 025 All rights reserved. 026 027 Redistribution and use in source and binary forms, with or without modification, 028 are permitted provided that the following conditions are met: 029 030 * Redistributions of source code must retain the above copyright notice, this 031 list of conditions and the following disclaimer. 032 * Redistributions in binary form must reproduce the above copyright notice, 033 this list of conditions and the following disclaimer in the documentation 034 and/or other materials provided with the distribution. 035 * Neither the name of HL7 nor the names of its contributors may be used to 036 endorse or promote products derived from this software without specific 037 prior written permission. 038 039 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 040 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 041 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 042 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 043 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 044 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 045 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 046 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 047 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 048 POSSIBILITY OF SUCH DAMAGE. 049 050*/ 051// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 052public class SearchParameter40_50 { 053 054 public static org.hl7.fhir.r5.model.SearchParameter convertSearchParameter(org.hl7.fhir.r4.model.SearchParameter src) throws FHIRException { 055 if (src == null) 056 return null; 057 org.hl7.fhir.r5.model.SearchParameter tgt = new org.hl7.fhir.r5.model.SearchParameter(); 058 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 059 if (src.hasUrl()) 060 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 061 if (src.hasVersion()) 062 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 063 if (src.hasName()) 064 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 065 if (src.hasDerivedFrom()) 066 tgt.setDerivedFromElement(Canonical40_50.convertCanonical(src.getDerivedFromElement())); 067 if (src.hasStatus()) 068 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 069 if (src.hasExperimental()) 070 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 071 if (src.hasDate()) 072 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 073 if (src.hasPublisher()) 074 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 075 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 076 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 077 if (src.hasDescription()) 078 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 079 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 080 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 081 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 082 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 083 if (src.hasPurpose()) 084 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 085 if (src.hasCode()) 086 tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 087 for (org.hl7.fhir.r4.model.CodeType t : src.getBase()) tgt.getBase().add(new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory(), Code40_50.convertCode(t))); 088 if (src.hasType()) 089 tgt.setTypeElement(Enumerations40_50.convertSearchParamType(src.getTypeElement())); 090 if (src.hasExpression()) 091 tgt.setExpressionElement(String40_50.convertString(src.getExpressionElement())); 092// if (src.hasXpath()) 093// tgt.setXpathElement(String40_50.convertString(src.getXpathElement())); 094 if (src.hasXpathUsage()) 095 tgt.setProcessingModeElement(convertXPathUsageType(src.getXpathUsageElement())); 096 for (org.hl7.fhir.r4.model.CodeType t : src.getTarget()) tgt.getTarget().add(new Enumeration<VersionIndependentResourceTypesAll>(new VersionIndependentResourceTypesAllEnumFactory(), Code40_50.convertCode(t))); 097 if (src.hasMultipleOr()) 098 tgt.setMultipleOrElement(Boolean40_50.convertBoolean(src.getMultipleOrElement())); 099 if (src.hasMultipleAnd()) 100 tgt.setMultipleAndElement(Boolean40_50.convertBoolean(src.getMultipleAndElement())); 101 tgt.setComparator(src.getComparator().stream() 102 .map(SearchParameter40_50::convertSearchComparator) 103 .collect(Collectors.toList())); 104 tgt.setModifier(src.getModifier().stream() 105 .map(SearchParameter40_50::convertSearchModifierCode) 106 .collect(Collectors.toList())); 107 for (org.hl7.fhir.r4.model.StringType t : src.getChain()) tgt.getChain().add(String40_50.convertString(t)); 108 for (org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent t : src.getComponent()) 109 tgt.addComponent(convertSearchParameterComponentComponent(t)); 110 return tgt; 111 } 112 113 public static org.hl7.fhir.r4.model.SearchParameter convertSearchParameter(org.hl7.fhir.r5.model.SearchParameter src) throws FHIRException { 114 if (src == null) 115 return null; 116 org.hl7.fhir.r4.model.SearchParameter tgt = new org.hl7.fhir.r4.model.SearchParameter(); 117 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 118 if (src.hasUrl()) 119 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 120 if (src.hasVersion()) 121 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 122 if (src.hasName()) 123 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 124 if (src.hasDerivedFrom()) 125 tgt.setDerivedFromElement(Canonical40_50.convertCanonical(src.getDerivedFromElement())); 126 if (src.hasStatus()) 127 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 128 if (src.hasExperimental()) 129 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 130 if (src.hasDate()) 131 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 132 if (src.hasPublisher()) 133 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 134 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 135 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 136 if (src.hasDescription()) 137 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 138 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 139 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 140 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 141 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 142 if (src.hasPurpose()) 143 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 144 if (src.hasCode()) 145 tgt.setCodeElement(Code40_50.convertCode(src.getCodeElement())); 146 for (Enumeration<VersionIndependentResourceTypesAll> t : src.getBase()) tgt.getBase().add(Code40_50.convertCode(t.getCodeType())); 147 if (src.hasType()) 148 tgt.setTypeElement(Enumerations40_50.convertSearchParamType(src.getTypeElement())); 149 if (src.hasExpression()) 150 tgt.setExpressionElement(String40_50.convertString(src.getExpressionElement())); 151// if (src.hasXpath()) 152// tgt.setXpathElement(String40_50.convertString(src.getXpathElement())); 153 if (src.hasProcessingMode()) 154 tgt.setXpathUsageElement(convertXPathUsageType(src.getProcessingModeElement())); 155 for (Enumeration<VersionIndependentResourceTypesAll> t : src.getTarget()) tgt.getTarget().add(Code40_50.convertCode(t.getCodeType())); 156 if (src.hasMultipleOr()) 157 tgt.setMultipleOrElement(Boolean40_50.convertBoolean(src.getMultipleOrElement())); 158 if (src.hasMultipleAnd()) 159 tgt.setMultipleAndElement(Boolean40_50.convertBoolean(src.getMultipleAndElement())); 160 tgt.setComparator(src.getComparator().stream() 161 .map(SearchParameter40_50::convertSearchComparator) 162 .collect(Collectors.toList())); 163 tgt.setModifier(src.getModifier().stream() 164 .map(SearchParameter40_50::convertSearchModifierCode) 165 .collect(Collectors.toList())); 166 for (org.hl7.fhir.r5.model.StringType t : src.getChain()) tgt.getChain().add(String40_50.convertString(t)); 167 for (org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent t : src.getComponent()) 168 tgt.addComponent(convertSearchParameterComponentComponent(t)); 169 return tgt; 170 } 171 172 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> convertXPathUsageType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> src) throws FHIRException { 173 if (src == null || src.isEmpty()) 174 return null; 175 Enumeration<SearchParameter.SearchProcessingModeType> tgt = new Enumeration<>(new SearchParameter.SearchProcessingModeTypeEnumFactory()); 176 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 177 if (src.getValue() == null) { 178 tgt.setValue(null); 179 } else { 180 switch (src.getValue()) { 181 case NORMAL: 182 tgt.setValue(SearchParameter.SearchProcessingModeType.NORMAL); 183 break; 184 case PHONETIC: 185 tgt.setValue(SearchParameter.SearchProcessingModeType.PHONETIC); 186 break; 187 case NEARBY: 188 tgt.setValue(SearchParameter.SearchProcessingModeType.OTHER); 189 break; 190 case DISTANCE: 191 tgt.setValue(SearchParameter.SearchProcessingModeType.OTHER); 192 break; 193 case OTHER: 194 tgt.setValue(SearchParameter.SearchProcessingModeType.OTHER); 195 break; 196 default: 197 tgt.setValue(SearchParameter.SearchProcessingModeType.NULL); 198 break; 199 } 200 } 201 return tgt; 202 } 203 204 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> convertXPathUsageType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SearchParameter.SearchProcessingModeType> src) throws FHIRException { 205 if (src == null || src.isEmpty()) 206 return null; 207 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.XPathUsageType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SearchParameter.XPathUsageTypeEnumFactory()); 208 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 209 if (src.getValue() == null) { 210 tgt.setValue(null); 211 } else { 212 switch (src.getValue()) { 213 case NORMAL: 214 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.NORMAL); 215 break; 216 case PHONETIC: 217 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.PHONETIC); 218 break; 219 case OTHER: 220 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.OTHER); 221 break; 222 default: 223 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.XPathUsageType.NULL); 224 break; 225 } 226 } 227 return tgt; 228 } 229 230 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator> convertSearchComparator(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchComparator> src) throws FHIRException { 231 if (src == null || src.isEmpty()) 232 return null; 233 Enumeration<Enumerations.SearchComparator> tgt = new Enumeration<>(new Enumerations.SearchComparatorEnumFactory()); 234 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 235 if (src.getValue() == null) { 236 tgt.setValue(null); 237 } else { 238 switch (src.getValue()) { 239 case EQ: 240 tgt.setValue(Enumerations.SearchComparator.EQ); 241 break; 242 case NE: 243 tgt.setValue(Enumerations.SearchComparator.NE); 244 break; 245 case GT: 246 tgt.setValue(Enumerations.SearchComparator.GT); 247 break; 248 case LT: 249 tgt.setValue(Enumerations.SearchComparator.LT); 250 break; 251 case GE: 252 tgt.setValue(Enumerations.SearchComparator.GE); 253 break; 254 case LE: 255 tgt.setValue(Enumerations.SearchComparator.LE); 256 break; 257 case SA: 258 tgt.setValue(Enumerations.SearchComparator.SA); 259 break; 260 case EB: 261 tgt.setValue(Enumerations.SearchComparator.EB); 262 break; 263 case AP: 264 tgt.setValue(Enumerations.SearchComparator.AP); 265 break; 266 default: 267 tgt.setValue(Enumerations.SearchComparator.NULL); 268 break; 269 } 270 } 271 return tgt; 272 } 273 274 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchComparator> convertSearchComparator(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchComparator> src) throws FHIRException { 275 if (src == null || src.isEmpty()) 276 return null; 277 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchComparator> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SearchParameter.SearchComparatorEnumFactory()); 278 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 279 if (src.getValue() == null) { 280 tgt.setValue(null); 281 } else { 282 switch (src.getValue()) { 283 case EQ: 284 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.EQ); 285 break; 286 case NE: 287 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.NE); 288 break; 289 case GT: 290 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.GT); 291 break; 292 case LT: 293 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.LT); 294 break; 295 case GE: 296 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.GE); 297 break; 298 case LE: 299 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.LE); 300 break; 301 case SA: 302 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.SA); 303 break; 304 case EB: 305 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.EB); 306 break; 307 case AP: 308 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.AP); 309 break; 310 default: 311 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchComparator.NULL); 312 break; 313 } 314 } 315 return tgt; 316 } 317 318 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode> convertSearchModifierCode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode> src) throws FHIRException { 319 if (src == null || src.isEmpty()) 320 return null; 321 Enumeration<Enumerations.SearchModifierCode> tgt = new Enumeration<>(new Enumerations.SearchModifierCodeEnumFactory()); 322 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 323 if (src.getValue() == null) { 324 tgt.setValue(null); 325 } else { 326 switch (src.getValue()) { 327 case MISSING: 328 tgt.setValue(Enumerations.SearchModifierCode.MISSING); 329 break; 330 case EXACT: 331 tgt.setValue(Enumerations.SearchModifierCode.EXACT); 332 break; 333 case CONTAINS: 334 tgt.setValue(Enumerations.SearchModifierCode.CONTAINS); 335 break; 336 case NOT: 337 tgt.setValue(Enumerations.SearchModifierCode.NOT); 338 break; 339 case TEXT: 340 tgt.setValue(Enumerations.SearchModifierCode.TEXT); 341 break; 342 case IN: 343 tgt.setValue(Enumerations.SearchModifierCode.IN); 344 break; 345 case NOTIN: 346 tgt.setValue(Enumerations.SearchModifierCode.NOTIN); 347 break; 348 case BELOW: 349 tgt.setValue(Enumerations.SearchModifierCode.BELOW); 350 break; 351 case ABOVE: 352 tgt.setValue(Enumerations.SearchModifierCode.ABOVE); 353 break; 354 case TYPE: 355 tgt.setValue(Enumerations.SearchModifierCode.TYPE); 356 break; 357 case IDENTIFIER: 358 tgt.setValue(Enumerations.SearchModifierCode.IDENTIFIER); 359 break; 360 case OFTYPE: 361 tgt.setValue(Enumerations.SearchModifierCode.OFTYPE); 362 break; 363 default: 364 tgt.setValue(Enumerations.SearchModifierCode.NULL); 365 break; 366 } 367 } 368 return tgt; 369 } 370 371 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode> convertSearchModifierCode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchModifierCode> src) throws FHIRException { 372 if (src == null || src.isEmpty()) 373 return null; 374 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SearchParameter.SearchModifierCodeEnumFactory()); 375 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 376 if (src.getValue() == null) { 377 tgt.setValue(null); 378 } else { 379 switch (src.getValue()) { 380 case MISSING: 381 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.MISSING); 382 break; 383 case EXACT: 384 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.EXACT); 385 break; 386 case CONTAINS: 387 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.CONTAINS); 388 break; 389 case NOT: 390 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.NOT); 391 break; 392 case TEXT: 393 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.TEXT); 394 break; 395 case IN: 396 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.IN); 397 break; 398 case NOTIN: 399 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.NOTIN); 400 break; 401 case BELOW: 402 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.BELOW); 403 break; 404 case ABOVE: 405 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.ABOVE); 406 break; 407 case TYPE: 408 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.TYPE); 409 break; 410 case IDENTIFIER: 411 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.IDENTIFIER); 412 break; 413 case OFTYPE: 414 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.OFTYPE); 415 break; 416 default: 417 tgt.setValue(org.hl7.fhir.r4.model.SearchParameter.SearchModifierCode.NULL); 418 break; 419 } 420 } 421 return tgt; 422 } 423 424 public static org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent convertSearchParameterComponentComponent(org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent src) throws FHIRException { 425 if (src == null) 426 return null; 427 org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent tgt = new org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent(); 428 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 429 if (src.hasDefinition()) 430 tgt.setDefinitionElement(Canonical40_50.convertCanonical(src.getDefinitionElement())); 431 if (src.hasExpression()) 432 tgt.setExpressionElement(String40_50.convertString(src.getExpressionElement())); 433 return tgt; 434 } 435 436 public static org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent convertSearchParameterComponentComponent(org.hl7.fhir.r5.model.SearchParameter.SearchParameterComponentComponent src) throws FHIRException { 437 if (src == null) 438 return null; 439 org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent tgt = new org.hl7.fhir.r4.model.SearchParameter.SearchParameterComponentComponent(); 440 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 441 if (src.hasDefinition()) 442 tgt.setDefinitionElement(Canonical40_50.convertCanonical(src.getDefinitionElement())); 443 if (src.hasExpression()) 444 tgt.setExpressionElement(String40_50.convertString(src.getExpressionElement())); 445 return tgt; 446 } 447}