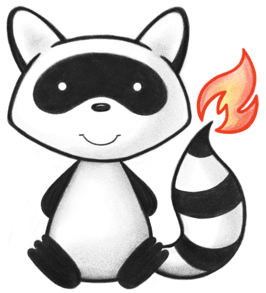
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Annotation40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r4.model.ServiceRequest; 015import org.hl7.fhir.r5.model.CodeableReference; 016import org.hl7.fhir.r5.model.Enumeration; 017import org.hl7.fhir.r5.model.Enumerations; 018import org.hl7.fhir.r5.model.ServiceRequest.ServiceRequestOrderDetailComponent; 019 020/* 021 Copyright (c) 2011+, HL7, Inc. 022 All rights reserved. 023 024 Redistribution and use in source and binary forms, with or without modification, 025 are permitted provided that the following conditions are met: 026 027 * Redistributions of source code must retain the above copyright notice, this 028 list of conditions and the following disclaimer. 029 * Redistributions in binary form must reproduce the above copyright notice, 030 this list of conditions and the following disclaimer in the documentation 031 and/or other materials provided with the distribution. 032 * Neither the name of HL7 nor the names of its contributors may be used to 033 endorse or promote products derived from this software without specific 034 prior written permission. 035 036 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 037 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 038 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 039 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 040 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 041 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 042 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 043 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 044 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 045 POSSIBILITY OF SUCH DAMAGE. 046 047*/ 048// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 049public class ServiceRequest40_50 { 050 051 public static org.hl7.fhir.r5.model.ServiceRequest convertServiceRequest(org.hl7.fhir.r4.model.ServiceRequest src) throws FHIRException { 052 if (src == null) 053 return null; 054 org.hl7.fhir.r5.model.ServiceRequest tgt = new org.hl7.fhir.r5.model.ServiceRequest(); 055 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 056 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 057 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 058 for (org.hl7.fhir.r4.model.CanonicalType t : src.getInstantiatesCanonical()) 059 tgt.getInstantiatesCanonical().add(Canonical40_50.convertCanonical(t)); 060 for (org.hl7.fhir.r4.model.UriType t : src.getInstantiatesUri()) 061 tgt.getInstantiatesUri().add(Uri40_50.convertUri(t)); 062 for (org.hl7.fhir.r4.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 063 for (org.hl7.fhir.r4.model.Reference t : src.getReplaces()) tgt.addReplaces(Reference40_50.convertReference(t)); 064 if (src.hasRequisition()) 065 tgt.setRequisition(Identifier40_50.convertIdentifier(src.getRequisition())); 066 if (src.hasStatus()) 067 tgt.setStatusElement(convertServiceRequestStatus(src.getStatusElement())); 068 if (src.hasIntent()) 069 tgt.setIntentElement(convertServiceRequestIntent(src.getIntentElement())); 070 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 071 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 072 if (src.hasPriority()) 073 tgt.setPriorityElement(convertServiceRequestPriority(src.getPriorityElement())); 074 if (src.hasDoNotPerform()) 075 tgt.setDoNotPerformElement(Boolean40_50.convertBoolean(src.getDoNotPerformElement())); 076 if (src.hasCode()) 077 tgt.setCode(CodeableConcept40_50.convertCodeableConceptToCodeableReference(src.getCode())); 078 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getOrderDetail()) 079 tgt.addOrderDetail().addParameter().setValue(CodeableConcept40_50.convertCodeableConcept(t)); 080 if (src.hasQuantity()) 081 tgt.setQuantity(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getQuantity())); 082 if (src.hasSubject()) 083 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 084 if (src.hasEncounter()) 085 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 086 if (src.hasOccurrence()) 087 tgt.setOccurrence(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOccurrence())); 088 if (src.hasAsNeeded()) 089 tgt.setAsNeeded(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getAsNeeded())); 090 if (src.hasAuthoredOn()) 091 tgt.setAuthoredOnElement(DateTime40_50.convertDateTime(src.getAuthoredOnElement())); 092 if (src.hasRequester()) 093 tgt.setRequester(Reference40_50.convertReference(src.getRequester())); 094 if (src.hasPerformerType()) 095 tgt.setPerformerType(CodeableConcept40_50.convertCodeableConcept(src.getPerformerType())); 096 for (org.hl7.fhir.r4.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference40_50.convertReference(t)); 097 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getLocationCode()) 098 tgt.addLocation(CodeableConcept40_50.convertCodeableConceptToCodeableReference(t)); 099 for (org.hl7.fhir.r4.model.Reference t : src.getLocationReference()) 100 tgt.addLocation(Reference40_50.convertReferenceToCodeableReference(t)); 101 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 102 tgt.addReason(CodeableConcept40_50.convertCodeableConceptToCodeableReference(t)); 103 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 104 tgt.addReason(Reference40_50.convertReferenceToCodeableReference(t)); 105 for (org.hl7.fhir.r4.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference40_50.convertReference(t)); 106 for (org.hl7.fhir.r4.model.Reference t : src.getSupportingInfo()) 107 tgt.addSupportingInfo(Reference40_50.convertReferenceToCodeableReference(t)); 108 for (org.hl7.fhir.r4.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference40_50.convertReference(t)); 109 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getBodySite()) 110 tgt.addBodySite(CodeableConcept40_50.convertCodeableConcept(t)); 111 for (org.hl7.fhir.r4.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 112 if (src.hasPatientInstruction()) 113 tgt.addPatientInstruction().setInstruction(String40_50.convertStringToMarkdown(src.getPatientInstructionElement())); 114 for (org.hl7.fhir.r4.model.Reference t : src.getRelevantHistory()) 115 tgt.addRelevantHistory(Reference40_50.convertReference(t)); 116 return tgt; 117 } 118 119 public static org.hl7.fhir.r4.model.ServiceRequest convertServiceRequest(org.hl7.fhir.r5.model.ServiceRequest src) throws FHIRException { 120 if (src == null) 121 return null; 122 org.hl7.fhir.r4.model.ServiceRequest tgt = new org.hl7.fhir.r4.model.ServiceRequest(); 123 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 124 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 125 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 126 for (org.hl7.fhir.r5.model.CanonicalType t : src.getInstantiatesCanonical()) 127 tgt.getInstantiatesCanonical().add(Canonical40_50.convertCanonical(t)); 128 for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesUri()) 129 tgt.getInstantiatesUri().add(Uri40_50.convertUri(t)); 130 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference40_50.convertReference(t)); 131 for (org.hl7.fhir.r5.model.Reference t : src.getReplaces()) tgt.addReplaces(Reference40_50.convertReference(t)); 132 if (src.hasRequisition()) 133 tgt.setRequisition(Identifier40_50.convertIdentifier(src.getRequisition())); 134 if (src.hasStatus()) 135 tgt.setStatusElement(convertServiceRequestStatus(src.getStatusElement())); 136 if (src.hasIntent()) 137 tgt.setIntentElement(convertServiceRequestIntent(src.getIntentElement())); 138 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 139 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 140 if (src.hasPriority()) 141 tgt.setPriorityElement(convertServiceRequestPriority(src.getPriorityElement())); 142 if (src.hasDoNotPerform()) 143 tgt.setDoNotPerformElement(Boolean40_50.convertBoolean(src.getDoNotPerformElement())); 144 if (src.hasCode()) 145 tgt.setCode(CodeableConcept40_50.convertCodeableReferenceToCodeableConcept(src.getCode())); 146 for (ServiceRequestOrderDetailComponent t : src.getOrderDetail()) { 147 if (t.getParameterFirstRep().hasValueCodeableConcept()) { 148 tgt.addOrderDetail(CodeableConcept40_50.convertCodeableConcept(t.getParameterFirstRep().getValueCodeableConcept())); 149 } 150 } 151 if (src.hasQuantity()) 152 tgt.setQuantity(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getQuantity())); 153 if (src.hasSubject()) 154 tgt.setSubject(Reference40_50.convertReference(src.getSubject())); 155 if (src.hasEncounter()) 156 tgt.setEncounter(Reference40_50.convertReference(src.getEncounter())); 157 if (src.hasOccurrence()) 158 tgt.setOccurrence(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOccurrence())); 159 if (src.hasAsNeeded()) 160 tgt.setAsNeeded(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getAsNeeded())); 161 if (src.hasAuthoredOn()) 162 tgt.setAuthoredOnElement(DateTime40_50.convertDateTime(src.getAuthoredOnElement())); 163 if (src.hasRequester()) 164 tgt.setRequester(Reference40_50.convertReference(src.getRequester())); 165 if (src.hasPerformerType()) 166 tgt.setPerformerType(CodeableConcept40_50.convertCodeableConcept(src.getPerformerType())); 167 for (org.hl7.fhir.r5.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference40_50.convertReference(t)); 168 for (CodeableReference t : src.getLocation()) 169 if (t.hasConcept()) 170 tgt.addLocationCode(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 171 for (CodeableReference t : src.getLocation()) 172 if (t.hasReference()) 173 tgt.addLocationReference(Reference40_50.convertReference(t.getReference())); 174 for (CodeableReference t : src.getReason()) 175 if (t.hasConcept()) 176 tgt.addReasonCode(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 177 for (CodeableReference t : src.getReason()) 178 if (t.hasReference()) 179 tgt.addReasonReference(Reference40_50.convertReference(t.getReference())); 180 for (org.hl7.fhir.r5.model.Reference t : src.getInsurance()) tgt.addInsurance(Reference40_50.convertReference(t)); 181 for (CodeableReference t : src.getSupportingInfo()) 182 if (t.hasReference()) 183 tgt.addSupportingInfo(Reference40_50.convertReference(t.getReference())); 184 for (org.hl7.fhir.r5.model.Reference t : src.getSpecimen()) tgt.addSpecimen(Reference40_50.convertReference(t)); 185 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getBodySite()) 186 tgt.addBodySite(CodeableConcept40_50.convertCodeableConcept(t)); 187 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation40_50.convertAnnotation(t)); 188 if (src.getPatientInstructionFirstRep().hasInstructionMarkdownType()) 189 tgt.setPatientInstructionElement(String40_50.convertString(src.getPatientInstructionFirstRep().getInstructionMarkdownType())); 190 for (org.hl7.fhir.r5.model.Reference t : src.getRelevantHistory()) 191 tgt.addRelevantHistory(Reference40_50.convertReference(t)); 192 return tgt; 193 } 194 195 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> convertServiceRequestStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ServiceRequest.ServiceRequestStatus> src) throws FHIRException { 196 if (src == null || src.isEmpty()) 197 return null; 198 Enumeration<Enumerations.RequestStatus> tgt = new Enumeration<>(new Enumerations.RequestStatusEnumFactory()); 199 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 200 if (src.getValue() == null) { 201 tgt.setValue(null); 202 } else { 203 switch (src.getValue()) { 204 case DRAFT: 205 tgt.setValue(Enumerations.RequestStatus.DRAFT); 206 break; 207 case ACTIVE: 208 tgt.setValue(Enumerations.RequestStatus.ACTIVE); 209 break; 210 case ONHOLD: 211 tgt.setValue(Enumerations.RequestStatus.ONHOLD); 212 break; 213 case REVOKED: 214 tgt.setValue(Enumerations.RequestStatus.REVOKED); 215 break; 216 case COMPLETED: 217 tgt.setValue(Enumerations.RequestStatus.COMPLETED); 218 break; 219 case ENTEREDINERROR: 220 tgt.setValue(Enumerations.RequestStatus.ENTEREDINERROR); 221 break; 222 case UNKNOWN: 223 tgt.setValue(Enumerations.RequestStatus.UNKNOWN); 224 break; 225 default: 226 tgt.setValue(Enumerations.RequestStatus.NULL); 227 break; 228 } 229 } 230 return tgt; 231 } 232 233 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ServiceRequest.ServiceRequestStatus> convertServiceRequestStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> src) throws FHIRException { 234 if (src == null || src.isEmpty()) 235 return null; 236 org.hl7.fhir.r4.model.Enumeration<ServiceRequest.ServiceRequestStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ServiceRequest.ServiceRequestStatusEnumFactory()); 237 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 238 if (src.getValue() == null) { 239 tgt.setValue(null); 240 } else { 241 switch (src.getValue()) { 242 case DRAFT: 243 tgt.setValue(ServiceRequest.ServiceRequestStatus.DRAFT); 244 break; 245 case ACTIVE: 246 tgt.setValue(ServiceRequest.ServiceRequestStatus.ACTIVE); 247 break; 248 case ONHOLD: 249 tgt.setValue(ServiceRequest.ServiceRequestStatus.ONHOLD); 250 break; 251 case REVOKED: 252 tgt.setValue(ServiceRequest.ServiceRequestStatus.REVOKED); 253 break; 254 case COMPLETED: 255 tgt.setValue(ServiceRequest.ServiceRequestStatus.COMPLETED); 256 break; 257 case ENTEREDINERROR: 258 tgt.setValue(ServiceRequest.ServiceRequestStatus.ENTEREDINERROR); 259 break; 260 case UNKNOWN: 261 tgt.setValue(ServiceRequest.ServiceRequestStatus.UNKNOWN); 262 break; 263 default: 264 tgt.setValue(ServiceRequest.ServiceRequestStatus.NULL); 265 break; 266 } 267 } 268 return tgt; 269 } 270 271 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestIntent> convertServiceRequestIntent(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ServiceRequest.ServiceRequestIntent> src) throws FHIRException { 272 if (src == null || src.isEmpty()) 273 return null; 274 Enumeration<Enumerations.RequestIntent> tgt = new Enumeration<>(new Enumerations.RequestIntentEnumFactory()); 275 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 276 if (src.getValue() == null) { 277 tgt.setValue(null); 278 } else { 279 switch (src.getValue()) { 280 case PROPOSAL: 281 tgt.setValue(Enumerations.RequestIntent.PROPOSAL); 282 break; 283 case PLAN: 284 tgt.setValue(Enumerations.RequestIntent.PLAN); 285 break; 286 case DIRECTIVE: 287 tgt.setValue(Enumerations.RequestIntent.DIRECTIVE); 288 break; 289 case ORDER: 290 tgt.setValue(Enumerations.RequestIntent.ORDER); 291 break; 292 case ORIGINALORDER: 293 tgt.setValue(Enumerations.RequestIntent.ORIGINALORDER); 294 break; 295 case REFLEXORDER: 296 tgt.setValue(Enumerations.RequestIntent.REFLEXORDER); 297 break; 298 case FILLERORDER: 299 tgt.setValue(Enumerations.RequestIntent.FILLERORDER); 300 break; 301 case INSTANCEORDER: 302 tgt.setValue(Enumerations.RequestIntent.INSTANCEORDER); 303 break; 304 case OPTION: 305 tgt.setValue(Enumerations.RequestIntent.OPTION); 306 break; 307 default: 308 tgt.setValue(Enumerations.RequestIntent.NULL); 309 break; 310 } 311 } 312 return tgt; 313 } 314 315 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ServiceRequest.ServiceRequestIntent> convertServiceRequestIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestIntent> src) throws FHIRException { 316 if (src == null || src.isEmpty()) 317 return null; 318 org.hl7.fhir.r4.model.Enumeration<ServiceRequest.ServiceRequestIntent> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ServiceRequest.ServiceRequestIntentEnumFactory()); 319 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 320 if (src.getValue() == null) { 321 tgt.setValue(null); 322 } else { 323 switch (src.getValue()) { 324 case PROPOSAL: 325 tgt.setValue(ServiceRequest.ServiceRequestIntent.PROPOSAL); 326 break; 327 case PLAN: 328 tgt.setValue(ServiceRequest.ServiceRequestIntent.PLAN); 329 break; 330 case DIRECTIVE: 331 tgt.setValue(ServiceRequest.ServiceRequestIntent.DIRECTIVE); 332 break; 333 case ORDER: 334 tgt.setValue(ServiceRequest.ServiceRequestIntent.ORDER); 335 break; 336 case ORIGINALORDER: 337 tgt.setValue(ServiceRequest.ServiceRequestIntent.ORIGINALORDER); 338 break; 339 case REFLEXORDER: 340 tgt.setValue(ServiceRequest.ServiceRequestIntent.REFLEXORDER); 341 break; 342 case FILLERORDER: 343 tgt.setValue(ServiceRequest.ServiceRequestIntent.FILLERORDER); 344 break; 345 case INSTANCEORDER: 346 tgt.setValue(ServiceRequest.ServiceRequestIntent.INSTANCEORDER); 347 break; 348 case OPTION: 349 tgt.setValue(ServiceRequest.ServiceRequestIntent.OPTION); 350 break; 351 default: 352 tgt.setValue(ServiceRequest.ServiceRequestIntent.NULL); 353 break; 354 } 355 } 356 return tgt; 357 } 358 359 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertServiceRequestPriority(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ServiceRequest.ServiceRequestPriority> src) throws FHIRException { 360 if (src == null || src.isEmpty()) 361 return null; 362 Enumeration<Enumerations.RequestPriority> tgt = new Enumeration<>(new Enumerations.RequestPriorityEnumFactory()); 363 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 364 if (src.getValue() == null) { 365 tgt.setValue(null); 366 } else { 367 switch (src.getValue()) { 368 case ROUTINE: 369 tgt.setValue(Enumerations.RequestPriority.ROUTINE); 370 break; 371 case URGENT: 372 tgt.setValue(Enumerations.RequestPriority.URGENT); 373 break; 374 case ASAP: 375 tgt.setValue(Enumerations.RequestPriority.ASAP); 376 break; 377 case STAT: 378 tgt.setValue(Enumerations.RequestPriority.STAT); 379 break; 380 default: 381 tgt.setValue(Enumerations.RequestPriority.NULL); 382 break; 383 } 384 } 385 return tgt; 386 } 387 388 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.ServiceRequest.ServiceRequestPriority> convertServiceRequestPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 389 if (src == null || src.isEmpty()) 390 return null; 391 org.hl7.fhir.r4.model.Enumeration<ServiceRequest.ServiceRequestPriority> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new ServiceRequest.ServiceRequestPriorityEnumFactory()); 392 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 393 if (src.getValue() == null) { 394 tgt.setValue(null); 395 } else { 396 switch (src.getValue()) { 397 case ROUTINE: 398 tgt.setValue(ServiceRequest.ServiceRequestPriority.ROUTINE); 399 break; 400 case URGENT: 401 tgt.setValue(ServiceRequest.ServiceRequestPriority.URGENT); 402 break; 403 case ASAP: 404 tgt.setValue(ServiceRequest.ServiceRequestPriority.ASAP); 405 break; 406 case STAT: 407 tgt.setValue(ServiceRequest.ServiceRequestPriority.STAT); 408 break; 409 default: 410 tgt.setValue(ServiceRequest.ServiceRequestPriority.NULL); 411 break; 412 } 413 } 414 return tgt; 415 } 416}