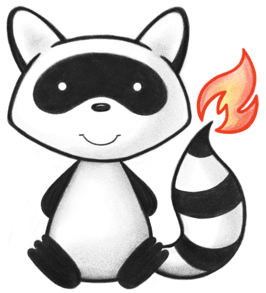
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Coding40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.ContactDetail40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.UsageContext40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Id40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 014import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 015import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 016import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.ElementDefinition40_50; 017import org.hl7.fhir.exceptions.FHIRException; 018 019/* 020 Copyright (c) 2011+, HL7, Inc. 021 All rights reserved. 022 023 Redistribution and use in source and binary forms, with or without modification, 024 are permitted provided that the following conditions are met: 025 026 * Redistributions of source code must retain the above copyright notice, this 027 list of conditions and the following disclaimer. 028 * Redistributions in binary form must reproduce the above copyright notice, 029 this list of conditions and the following disclaimer in the documentation 030 and/or other materials provided with the distribution. 031 * Neither the name of HL7 nor the names of its contributors may be used to 032 endorse or promote products derived from this software without specific 033 prior written permission. 034 035 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 036 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 037 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 038 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 039 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 040 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 041 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 042 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 043 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 044 POSSIBILITY OF SUCH DAMAGE. 045 046*/ 047// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 048public class StructureDefinition40_50 { 049 050 public static org.hl7.fhir.r5.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.r4.model.StructureDefinition src) throws FHIRException { 051 if (src == null) 052 return null; 053 org.hl7.fhir.r5.model.StructureDefinition tgt = new org.hl7.fhir.r5.model.StructureDefinition(); 054 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 055 if (src.hasUrl()) 056 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 057 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 058 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 059 if (src.hasVersion()) 060 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 061 if (src.hasName()) 062 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 063 if (src.hasTitle()) 064 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 065 if (src.hasStatus()) 066 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 067 if (src.hasExperimental()) 068 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 069 if (src.hasDate()) 070 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 071 if (src.hasPublisher()) 072 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 073 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 074 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 075 if (src.hasDescription()) 076 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 077 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 078 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 079 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 080 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 081 if (src.hasPurpose()) 082 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 083 if (src.hasCopyright()) 084 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 085 for (org.hl7.fhir.r4.model.Coding t : src.getKeyword()) tgt.addKeyword(Coding40_50.convertCoding(t)); 086 if (src.hasFhirVersion()) 087 tgt.setFhirVersionElement(Enumerations40_50.convertFHIRVersion(src.getFhirVersionElement())); 088 for (org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 089 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 090 if (src.hasKind()) 091 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement())); 092 if (src.hasAbstract()) 093 tgt.setAbstractElement(Boolean40_50.convertBoolean(src.getAbstractElement())); 094 for (org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent t : src.getContext()) 095 tgt.addContext(convertStructureDefinitionContextComponent(t)); 096 for (org.hl7.fhir.r4.model.StringType t : src.getContextInvariant()) 097 tgt.getContextInvariant().add(String40_50.convertString(t)); 098 if (src.hasType()) 099 tgt.setTypeElement(Uri40_50.convertUri(src.getTypeElement())); 100 if (src.hasBaseDefinition()) 101 tgt.setBaseDefinitionElement(Canonical40_50.convertCanonical(src.getBaseDefinitionElement())); 102 if (src.hasDerivation()) 103 tgt.setDerivationElement(convertTypeDerivationRule(src.getDerivationElement())); 104 if (src.hasSnapshot()) 105 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 106 if (src.hasDifferential()) 107 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 108 return tgt; 109 } 110 111 public static org.hl7.fhir.r4.model.StructureDefinition convertStructureDefinition(org.hl7.fhir.r5.model.StructureDefinition src) throws FHIRException { 112 if (src == null) 113 return null; 114 org.hl7.fhir.r4.model.StructureDefinition tgt = new org.hl7.fhir.r4.model.StructureDefinition(); 115 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 116 if (src.hasUrl()) 117 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 118 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 119 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 120 if (src.hasVersion()) 121 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 122 if (src.hasName()) 123 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 124 if (src.hasTitle()) 125 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 126 if (src.hasStatus()) 127 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 128 if (src.hasExperimental()) 129 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 130 if (src.hasDate()) 131 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 132 if (src.hasPublisher()) 133 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 134 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 135 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 136 if (src.hasDescription()) 137 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 138 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 139 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 140 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 141 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 142 if (src.hasPurpose()) 143 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 144 if (src.hasCopyright()) 145 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 146 for (org.hl7.fhir.r5.model.Coding t : src.getKeyword()) tgt.addKeyword(Coding40_50.convertCoding(t)); 147 if (src.hasFhirVersion()) 148 tgt.setFhirVersionElement(Enumerations40_50.convertFHIRVersion(src.getFhirVersionElement())); 149 for (org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent t : src.getMapping()) 150 tgt.addMapping(convertStructureDefinitionMappingComponent(t)); 151 if (src.hasKind()) 152 tgt.setKindElement(convertStructureDefinitionKind(src.getKindElement())); 153 if (src.hasAbstract()) 154 tgt.setAbstractElement(Boolean40_50.convertBoolean(src.getAbstractElement())); 155 for (org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent t : src.getContext()) 156 tgt.addContext(convertStructureDefinitionContextComponent(t)); 157 for (org.hl7.fhir.r5.model.StringType t : src.getContextInvariant()) 158 tgt.getContextInvariant().add(String40_50.convertString(t)); 159 if (src.hasType()) 160 tgt.setTypeElement(Uri40_50.convertUri(src.getTypeElement())); 161 if (src.hasBaseDefinition()) 162 tgt.setBaseDefinitionElement(Canonical40_50.convertCanonical(src.getBaseDefinitionElement())); 163 if (src.hasDerivation()) 164 tgt.setDerivationElement(convertTypeDerivationRule(src.getDerivationElement())); 165 if (src.hasSnapshot()) 166 tgt.setSnapshot(convertStructureDefinitionSnapshotComponent(src.getSnapshot())); 167 if (src.hasDifferential()) 168 tgt.setDifferential(convertStructureDefinitionDifferentialComponent(src.getDifferential())); 169 return tgt; 170 } 171 172 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind> src) throws FHIRException { 173 if (src == null || src.isEmpty()) 174 return null; 175 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 176 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 177 switch (src.getValue()) { 178 case PRIMITIVETYPE: 179 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.PRIMITIVETYPE); 180 break; 181 case COMPLEXTYPE: 182 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.COMPLEXTYPE); 183 break; 184 case RESOURCE: 185 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 186 break; 187 case LOGICAL: 188 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 189 break; 190 default: 191 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind.NULL); 192 break; 193 } 194 return tgt; 195 } 196 197 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind> convertStructureDefinitionKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionKind> src) throws FHIRException { 198 if (src == null || src.isEmpty()) 199 return null; 200 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKindEnumFactory()); 201 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 202 switch (src.getValue()) { 203 case PRIMITIVETYPE: 204 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.PRIMITIVETYPE); 205 break; 206 case COMPLEXTYPE: 207 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.COMPLEXTYPE); 208 break; 209 case RESOURCE: 210 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.RESOURCE); 211 break; 212 case LOGICAL: 213 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.LOGICAL); 214 break; 215 default: 216 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionKind.NULL); 217 break; 218 } 219 return tgt; 220 } 221 222 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule> convertTypeDerivationRule(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule> src) throws FHIRException { 223 if (src == null || src.isEmpty()) 224 return null; 225 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRuleEnumFactory()); 226 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 227 switch (src.getValue()) { 228 case SPECIALIZATION: 229 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 230 break; 231 case CONSTRAINT: 232 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule.CONSTRAINT); 233 break; 234 default: 235 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule.NULL); 236 break; 237 } 238 return tgt; 239 } 240 241 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule> convertTypeDerivationRule(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.TypeDerivationRule> src) throws FHIRException { 242 if (src == null || src.isEmpty()) 243 return null; 244 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRuleEnumFactory()); 245 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 246 switch (src.getValue()) { 247 case SPECIALIZATION: 248 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.SPECIALIZATION); 249 break; 250 case CONSTRAINT: 251 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.CONSTRAINT); 252 break; 253 default: 254 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.TypeDerivationRule.NULL); 255 break; 256 } 257 return tgt; 258 } 259 260 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 261 if (src == null) 262 return null; 263 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent(); 264 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 265 if (src.hasIdentity()) 266 tgt.setIdentityElement(Id40_50.convertId(src.getIdentityElement())); 267 if (src.hasUri()) 268 tgt.setUriElement(Uri40_50.convertUri(src.getUriElement())); 269 if (src.hasName()) 270 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 271 if (src.hasComment()) 272 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 273 return tgt; 274 } 275 276 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent convertStructureDefinitionMappingComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionMappingComponent src) throws FHIRException { 277 if (src == null) 278 return null; 279 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionMappingComponent(); 280 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 281 if (src.hasIdentity()) 282 tgt.setIdentityElement(Id40_50.convertId(src.getIdentityElement())); 283 if (src.hasUri()) 284 tgt.setUriElement(Uri40_50.convertUri(src.getUriElement())); 285 if (src.hasName()) 286 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 287 if (src.hasComment()) 288 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 289 return tgt; 290 } 291 292 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent convertStructureDefinitionContextComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent src) throws FHIRException { 293 if (src == null) 294 return null; 295 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent(); 296 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 297 if (src.hasType()) 298 tgt.setTypeElement(convertExtensionContextType(src.getTypeElement())); 299 if (src.hasExpression()) 300 tgt.setExpressionElement(String40_50.convertString(src.getExpressionElement())); 301 return tgt; 302 } 303 304 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent convertStructureDefinitionContextComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionContextComponent src) throws FHIRException { 305 if (src == null) 306 return null; 307 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionContextComponent(); 308 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 309 if (src.hasType()) 310 tgt.setTypeElement(convertExtensionContextType(src.getTypeElement())); 311 if (src.hasExpression()) 312 tgt.setExpressionElement(String40_50.convertString(src.getExpressionElement())); 313 return tgt; 314 } 315 316 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType> convertExtensionContextType(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType> src) throws FHIRException { 317 if (src == null || src.isEmpty()) 318 return null; 319 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextTypeEnumFactory()); 320 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 321 switch (src.getValue()) { 322 case FHIRPATH: 323 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType.FHIRPATH); 324 break; 325 case ELEMENT: 326 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType.ELEMENT); 327 break; 328 case EXTENSION: 329 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType.EXTENSION); 330 break; 331 default: 332 tgt.setValue(org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType.NULL); 333 break; 334 } 335 return tgt; 336 } 337 338 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType> convertExtensionContextType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureDefinition.ExtensionContextType> src) throws FHIRException { 339 if (src == null || src.isEmpty()) 340 return null; 341 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextTypeEnumFactory()); 342 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 343 switch (src.getValue()) { 344 case FHIRPATH: 345 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType.FHIRPATH); 346 break; 347 case ELEMENT: 348 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType.ELEMENT); 349 break; 350 case EXTENSION: 351 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType.EXTENSION); 352 break; 353 default: 354 tgt.setValue(org.hl7.fhir.r4.model.StructureDefinition.ExtensionContextType.NULL); 355 break; 356 } 357 return tgt; 358 } 359 360 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 361 if (src == null) 362 return null; 363 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 364 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 365 for (org.hl7.fhir.r4.model.ElementDefinition t : src.getElement()) 366 tgt.addElement(ElementDefinition40_50.convertElementDefinition(t)); 367 return tgt; 368 } 369 370 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent convertStructureDefinitionSnapshotComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionSnapshotComponent src) throws FHIRException { 371 if (src == null) 372 return null; 373 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionSnapshotComponent(); 374 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 375 for (org.hl7.fhir.r5.model.ElementDefinition t : src.getElement()) 376 tgt.addElement(ElementDefinition40_50.convertElementDefinition(t)); 377 return tgt; 378 } 379 380 public static org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 381 if (src == null) 382 return null; 383 org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 384 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 385 for (org.hl7.fhir.r4.model.ElementDefinition t : src.getElement()) 386 tgt.addElement(ElementDefinition40_50.convertElementDefinition(t)); 387 return tgt; 388 } 389 390 public static org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent convertStructureDefinitionDifferentialComponent(org.hl7.fhir.r5.model.StructureDefinition.StructureDefinitionDifferentialComponent src) throws FHIRException { 391 if (src == null) 392 return null; 393 org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent tgt = new org.hl7.fhir.r4.model.StructureDefinition.StructureDefinitionDifferentialComponent(); 394 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 395 for (org.hl7.fhir.r5.model.ElementDefinition t : src.getElement()) 396 tgt.addElement(ElementDefinition40_50.convertElementDefinition(t)); 397 return tgt; 398 } 399}