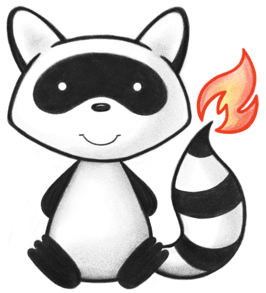
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.ContactDetail40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.metadata40_50.UsageContext40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 011import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Canonical40_50; 012import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 013import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Id40_50; 014import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Integer40_50; 015import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.MarkDown40_50; 016import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 017import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Uri40_50; 018import org.hl7.fhir.exceptions.FHIRException; 019import org.hl7.fhir.r4.model.StructureMap.StructureMapGroupTypeMode; 020import org.hl7.fhir.r4.utils.ToolingExtensions; 021import org.hl7.fhir.r5.fhirpath.FHIRPathConstant; 022import org.hl7.fhir.r5.model.*; 023import org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent; 024import org.hl7.fhir.utilities.Utilities; 025 026/* 027 Copyright (c) 2011+, HL7, Inc. 028 All rights reserved. 029 030 Redistribution and use in source and binary forms, with or without modification, 031 are permitted provided that the following conditions are met: 032 033 * Redistributions of source code must retain the above copyright notice, this 034 list of conditions and the following disclaimer. 035 * Redistributions in binary form must reproduce the above copyright notice, 036 this list of conditions and the following disclaimer in the documentation 037 and/or other materials provided with the distribution. 038 * Neither the name of HL7 nor the names of its contributors may be used to 039 endorse or promote products derived from this software without specific 040 prior written permission. 041 042 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 043 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 044 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 045 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 046 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 047 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 048 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 049 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 050 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 051 POSSIBILITY OF SUCH DAMAGE. 052 053*/ 054// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 055public class StructureMap40_50 { 056 057 public static org.hl7.fhir.r5.model.StructureMap convertStructureMap(org.hl7.fhir.r4.model.StructureMap src) throws FHIRException { 058 if (src == null) 059 return null; 060 org.hl7.fhir.r5.model.StructureMap tgt = new org.hl7.fhir.r5.model.StructureMap(); 061 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 062 if (src.hasUrl()) 063 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 064 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 065 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 066 if (src.hasVersion()) 067 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 068 if (src.hasName()) 069 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 070 if (src.hasTitle()) 071 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 072 if (src.hasStatus()) 073 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 074 if (src.hasExperimental()) 075 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 076 if (src.hasDate()) 077 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 078 if (src.hasPublisher()) 079 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 080 for (org.hl7.fhir.r4.model.ContactDetail t : src.getContact()) 081 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 082 if (src.hasDescription()) 083 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 084 for (org.hl7.fhir.r4.model.UsageContext t : src.getUseContext()) 085 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 086 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getJurisdiction()) 087 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 088 if (src.hasPurpose()) 089 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 090 if (src.hasCopyright()) 091 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 092 for (org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent t : src.getStructure()) 093 tgt.addStructure(convertStructureMapStructureComponent(t)); 094 for (org.hl7.fhir.r4.model.CanonicalType t : src.getImport()) 095 tgt.getImport().add(Canonical40_50.convertCanonical(t)); 096 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent t : src.getGroup()) 097 tgt.addGroup(convertStructureMapGroupComponent(t)); 098 return tgt; 099 } 100 101 public static org.hl7.fhir.r4.model.StructureMap convertStructureMap(org.hl7.fhir.r5.model.StructureMap src) throws FHIRException { 102 if (src == null) 103 return null; 104 org.hl7.fhir.r4.model.StructureMap tgt = new org.hl7.fhir.r4.model.StructureMap(); 105 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 106 if (src.hasUrl()) 107 tgt.setUrlElement(Uri40_50.convertUri(src.getUrlElement())); 108 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 109 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 110 if (src.hasVersion()) 111 tgt.setVersionElement(String40_50.convertString(src.getVersionElement())); 112 if (src.hasName()) 113 tgt.setNameElement(String40_50.convertString(src.getNameElement())); 114 if (src.hasTitle()) 115 tgt.setTitleElement(String40_50.convertString(src.getTitleElement())); 116 if (src.hasStatus()) 117 tgt.setStatusElement(Enumerations40_50.convertPublicationStatus(src.getStatusElement())); 118 if (src.hasExperimental()) 119 tgt.setExperimentalElement(Boolean40_50.convertBoolean(src.getExperimentalElement())); 120 if (src.hasDate()) 121 tgt.setDateElement(DateTime40_50.convertDateTime(src.getDateElement())); 122 if (src.hasPublisher()) 123 tgt.setPublisherElement(String40_50.convertString(src.getPublisherElement())); 124 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 125 tgt.addContact(ContactDetail40_50.convertContactDetail(t)); 126 if (src.hasDescription()) 127 tgt.setDescriptionElement(MarkDown40_50.convertMarkdown(src.getDescriptionElement())); 128 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 129 tgt.addUseContext(UsageContext40_50.convertUsageContext(t)); 130 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 131 tgt.addJurisdiction(CodeableConcept40_50.convertCodeableConcept(t)); 132 if (src.hasPurpose()) 133 tgt.setPurposeElement(MarkDown40_50.convertMarkdown(src.getPurposeElement())); 134 if (src.hasCopyright()) 135 tgt.setCopyrightElement(MarkDown40_50.convertMarkdown(src.getCopyrightElement())); 136 for (org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent t : src.getStructure()) 137 tgt.addStructure(convertStructureMapStructureComponent(t)); 138 for (org.hl7.fhir.r5.model.CanonicalType t : src.getImport()) 139 tgt.getImport().add(Canonical40_50.convertCanonical(t)); 140 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent t : src.getGroup()) 141 tgt.addGroup(convertStructureMapGroupComponent(t)); 142 return tgt; 143 } 144 145 public static org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent convertStructureMapStructureComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent src) throws FHIRException { 146 if (src == null) 147 return null; 148 org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent(); 149 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 150 if (src.hasUrl()) 151 tgt.setUrlElement(Canonical40_50.convertCanonical(src.getUrlElement())); 152 if (src.hasMode()) 153 tgt.setModeElement(convertStructureMapModelMode(src.getModeElement())); 154 if (src.hasAlias()) 155 tgt.setAliasElement(String40_50.convertString(src.getAliasElement())); 156 if (src.hasDocumentation()) 157 tgt.setDocumentationElement(String40_50.convertString(src.getDocumentationElement())); 158 return tgt; 159 } 160 161 public static org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent convertStructureMapStructureComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapStructureComponent src) throws FHIRException { 162 if (src == null) 163 return null; 164 org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapStructureComponent(); 165 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 166 if (src.hasUrl()) 167 tgt.setUrlElement(Canonical40_50.convertCanonical(src.getUrlElement())); 168 if (src.hasMode()) 169 tgt.setModeElement(convertStructureMapModelMode(src.getModeElement())); 170 if (src.hasAlias()) 171 tgt.setAliasElement(String40_50.convertString(src.getAliasElement())); 172 if (src.hasDocumentation()) 173 tgt.setDocumentationElement(String40_50.convertString(src.getDocumentationElement())); 174 return tgt; 175 } 176 177 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode> convertStructureMapModelMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode> src) throws FHIRException { 178 if (src == null || src.isEmpty()) 179 return null; 180 Enumeration<StructureMap.StructureMapModelMode> tgt = new Enumeration<>(new StructureMap.StructureMapModelModeEnumFactory()); 181 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 182 if (src.getValue() == null) { 183 tgt.setValue(null); 184 } else { 185 switch (src.getValue()) { 186 case SOURCE: 187 tgt.setValue(StructureMap.StructureMapModelMode.SOURCE); 188 break; 189 case QUERIED: 190 tgt.setValue(StructureMap.StructureMapModelMode.QUERIED); 191 break; 192 case TARGET: 193 tgt.setValue(StructureMap.StructureMapModelMode.TARGET); 194 break; 195 case PRODUCED: 196 tgt.setValue(StructureMap.StructureMapModelMode.PRODUCED); 197 break; 198 default: 199 tgt.setValue(StructureMap.StructureMapModelMode.NULL); 200 break; 201 } 202 } 203 return tgt; 204 } 205 206 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode> convertStructureMapModelMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapModelMode> src) throws FHIRException { 207 if (src == null || src.isEmpty()) 208 return null; 209 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapModelModeEnumFactory()); 210 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 211 if (src.getValue() == null) { 212 tgt.setValue(null); 213 } else { 214 switch (src.getValue()) { 215 case SOURCE: 216 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.SOURCE); 217 break; 218 case QUERIED: 219 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.QUERIED); 220 break; 221 case TARGET: 222 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.TARGET); 223 break; 224 case PRODUCED: 225 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.PRODUCED); 226 break; 227 default: 228 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapModelMode.NULL); 229 break; 230 } 231 } 232 return tgt; 233 } 234 235 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent convertStructureMapGroupComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent src) throws FHIRException { 236 if (src == null) 237 return null; 238 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent(); 239 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 240 if (src.hasName()) 241 tgt.setNameElement(Id40_50.convertId(src.getNameElement())); 242 if (src.hasExtends()) 243 tgt.setExtendsElement(Id40_50.convertId(src.getExtendsElement())); 244 if (src.hasTypeMode()) 245 tgt.setTypeModeElement(convertStructureMapGroupTypeMode(src.getTypeModeElement())); 246 if (src.hasDocumentation()) 247 tgt.setDocumentationElement(String40_50.convertString(src.getDocumentationElement())); 248 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent t : src.getInput()) 249 tgt.addInput(convertStructureMapGroupInputComponent(t)); 250 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 251 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 252 return tgt; 253 } 254 255 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent convertStructureMapGroupComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupComponent src) throws FHIRException { 256 if (src == null) 257 return null; 258 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupComponent(); 259 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 260 if (src.hasName()) 261 tgt.setNameElement(Id40_50.convertId(src.getNameElement())); 262 if (src.hasExtends()) 263 tgt.setExtendsElement(Id40_50.convertId(src.getExtendsElement())); 264 if (src.hasTypeMode()) { 265 tgt.setTypeModeElement(convertStructureMapGroupTypeMode(src.getTypeModeElement())); 266 } else { 267 tgt.setTypeMode(StructureMapGroupTypeMode.NONE); 268 } 269 if (src.hasDocumentation()) 270 tgt.setDocumentationElement(String40_50.convertString(src.getDocumentationElement())); 271 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent t : src.getInput()) 272 tgt.addInput(convertStructureMapGroupInputComponent(t)); 273 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 274 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 275 return tgt; 276 } 277 278 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeMode> convertStructureMapGroupTypeMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapGroupTypeMode> src) throws FHIRException { 279 if (src == null || src.isEmpty()) 280 return null; 281 Enumeration<StructureMap.StructureMapGroupTypeMode> tgt = new Enumeration<>(new StructureMap.StructureMapGroupTypeModeEnumFactory()); 282 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 283 if (src.getValue() == null) { 284 tgt.setValue(null); 285 } else { 286 switch (src.getValue()) { 287 case NONE: 288 return null; 289 case TYPES: 290 tgt.setValue(StructureMap.StructureMapGroupTypeMode.TYPES); 291 break; 292 case TYPEANDTYPES: 293 tgt.setValue(StructureMap.StructureMapGroupTypeMode.TYPEANDTYPES); 294 break; 295 default: 296 tgt.setValue(StructureMap.StructureMapGroupTypeMode.NULL); 297 break; 298 } 299 } 300 return tgt; 301 } 302 303 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapGroupTypeMode> convertStructureMapGroupTypeMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapGroupTypeMode> src) throws FHIRException { 304 org.hl7.fhir.r4.model.Enumeration<StructureMapGroupTypeMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupTypeModeEnumFactory()); 305 if (src == null || src.isEmpty()) { 306 tgt.setValue(StructureMapGroupTypeMode.NONE); 307 return tgt; 308 } 309 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 310 if (src.getValue() == null) { 311 tgt.setValue(null); 312 } else { 313 switch (src.getValue()) { 314 case TYPES: 315 tgt.setValue(StructureMapGroupTypeMode.TYPES); 316 break; 317 case TYPEANDTYPES: 318 tgt.setValue(StructureMapGroupTypeMode.TYPEANDTYPES); 319 break; 320 default: 321 tgt.setValue(StructureMapGroupTypeMode.NULL); 322 break; 323 } 324 } 325 return tgt; 326 } 327 328 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent convertStructureMapGroupInputComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent src) throws FHIRException { 329 if (src == null) 330 return null; 331 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent(); 332 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 333 if (src.hasName()) 334 tgt.setNameElement(Id40_50.convertId(src.getNameElement())); 335 if (src.hasType()) 336 tgt.setTypeElement(String40_50.convertString(src.getTypeElement())); 337 if (src.hasMode()) 338 tgt.setModeElement(convertStructureMapInputMode(src.getModeElement())); 339 if (src.hasDocumentation()) 340 tgt.setDocumentationElement(String40_50.convertString(src.getDocumentationElement())); 341 return tgt; 342 } 343 344 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent convertStructureMapGroupInputComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupInputComponent src) throws FHIRException { 345 if (src == null) 346 return null; 347 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupInputComponent(); 348 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 349 if (src.hasName()) 350 tgt.setNameElement(Id40_50.convertId(src.getNameElement())); 351 if (src.hasType()) 352 tgt.setTypeElement(String40_50.convertString(src.getTypeElement())); 353 if (src.hasMode()) 354 tgt.setModeElement(convertStructureMapInputMode(src.getModeElement())); 355 if (src.hasDocumentation()) 356 tgt.setDocumentationElement(String40_50.convertString(src.getDocumentationElement())); 357 return tgt; 358 } 359 360 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapInputMode> convertStructureMapInputMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode> src) throws FHIRException { 361 if (src == null || src.isEmpty()) 362 return null; 363 Enumeration<StructureMap.StructureMapInputMode> tgt = new Enumeration<>(new StructureMap.StructureMapInputModeEnumFactory()); 364 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 365 if (src.getValue() == null) { 366 tgt.setValue(null); 367 } else { 368 switch (src.getValue()) { 369 case SOURCE: 370 tgt.setValue(StructureMap.StructureMapInputMode.SOURCE); 371 break; 372 case TARGET: 373 tgt.setValue(StructureMap.StructureMapInputMode.TARGET); 374 break; 375 default: 376 tgt.setValue(StructureMap.StructureMapInputMode.NULL); 377 break; 378 } 379 } 380 return tgt; 381 } 382 383 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode> convertStructureMapInputMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapInputMode> src) throws FHIRException { 384 if (src == null || src.isEmpty()) 385 return null; 386 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapInputModeEnumFactory()); 387 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 388 if (src.getValue() == null) { 389 tgt.setValue(null); 390 } else { 391 switch (src.getValue()) { 392 case SOURCE: 393 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode.SOURCE); 394 break; 395 case TARGET: 396 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode.TARGET); 397 break; 398 default: 399 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapInputMode.NULL); 400 break; 401 } 402 } 403 return tgt; 404 } 405 406 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent convertStructureMapGroupRuleComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent src) throws FHIRException { 407 if (src == null) 408 return null; 409 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent(); 410 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 411 if (src.hasName()) 412 tgt.setNameElement(Id40_50.convertId(src.getNameElement())); 413 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent t : src.getSource()) 414 tgt.addSource(convertStructureMapGroupRuleSourceComponent(t)); 415 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent t : src.getTarget()) 416 tgt.addTarget(convertStructureMapGroupRuleTargetComponent(t)); 417 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 418 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 419 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent t : src.getDependent()) 420 tgt.addDependent(convertStructureMapGroupRuleDependentComponent(t)); 421 if (src.hasDocumentation()) 422 tgt.setDocumentationElement(String40_50.convertString(src.getDocumentationElement())); 423 return tgt; 424 } 425 426 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent convertStructureMapGroupRuleComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent src) throws FHIRException { 427 if (src == null) 428 return null; 429 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleComponent(); 430 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 431 if (src.hasName()) 432 tgt.setNameElement(Id40_50.convertId(src.getNameElement())); 433 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent t : src.getSource()) 434 tgt.addSource(convertStructureMapGroupRuleSourceComponent(t)); 435 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent t : src.getTarget()) 436 tgt.addTarget(convertStructureMapGroupRuleTargetComponent(t)); 437 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleComponent t : src.getRule()) 438 tgt.addRule(convertStructureMapGroupRuleComponent(t)); 439 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent t : src.getDependent()) 440 tgt.addDependent(convertStructureMapGroupRuleDependentComponent(t)); 441 if (src.hasDocumentation()) 442 tgt.setDocumentationElement(String40_50.convertString(src.getDocumentationElement())); 443 return tgt; 444 } 445 446 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent convertStructureMapGroupRuleSourceComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent src) throws FHIRException { 447 if (src == null) 448 return null; 449 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent(); 450 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 451 if (src.hasContext()) 452 tgt.setContextElement(Id40_50.convertId(src.getContextElement())); 453 if (src.hasMin()) 454 tgt.setMinElement(Integer40_50.convertInteger(src.getMinElement())); 455 if (src.hasMax()) 456 tgt.setMaxElement(String40_50.convertString(src.getMaxElement())); 457 if (src.hasType()) 458 tgt.setTypeElement(String40_50.convertString(src.getTypeElement())); 459 if (src.hasDefaultValue()) 460 tgt.setDefaultValueElement((StringType) ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getDefaultValue())); 461 if (src.hasElement()) 462 tgt.setElementElement(String40_50.convertString(src.getElementElement())); 463 if (src.hasListMode()) 464 tgt.setListModeElement(convertStructureMapSourceListMode(src.getListModeElement())); 465 if (src.hasVariable()) 466 tgt.setVariableElement(Id40_50.convertId(src.getVariableElement())); 467 if (src.hasCondition()) 468 tgt.setConditionElement(String40_50.convertString(src.getConditionElement())); 469 if (src.hasCheck()) 470 tgt.setCheckElement(String40_50.convertString(src.getCheckElement())); 471 if (src.hasLogMessage()) 472 tgt.setLogMessageElement(String40_50.convertString(src.getLogMessageElement())); 473 return tgt; 474 } 475 476 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent convertStructureMapGroupRuleSourceComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleSourceComponent src) throws FHIRException { 477 if (src == null) 478 return null; 479 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleSourceComponent(); 480 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 481 if (src.hasContext()) 482 tgt.setContextElement(Id40_50.convertId(src.getContextElement())); 483 if (src.hasMin()) 484 tgt.setMinElement(Integer40_50.convertInteger(src.getMinElement())); 485 if (src.hasMax()) 486 tgt.setMaxElement(String40_50.convertString(src.getMaxElement())); 487 if (src.hasType()) 488 tgt.setTypeElement(String40_50.convertString(src.getTypeElement())); 489 if (src.hasDefaultValue()) 490 tgt.setDefaultValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getDefaultValueElement())); 491 if (src.hasElement()) 492 tgt.setElementElement(String40_50.convertString(src.getElementElement())); 493 if (src.hasListMode()) 494 tgt.setListModeElement(convertStructureMapSourceListMode(src.getListModeElement())); 495 if (src.hasVariable()) 496 tgt.setVariableElement(Id40_50.convertId(src.getVariableElement())); 497 if (src.hasCondition()) 498 tgt.setConditionElement(String40_50.convertString(src.getConditionElement())); 499 if (src.hasCheck()) 500 tgt.setCheckElement(String40_50.convertString(src.getCheckElement())); 501 if (src.hasLogMessage()) 502 tgt.setLogMessageElement(String40_50.convertString(src.getLogMessageElement())); 503 return tgt; 504 } 505 506 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode> convertStructureMapSourceListMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode> src) throws FHIRException { 507 if (src == null || src.isEmpty()) 508 return null; 509 Enumeration<StructureMap.StructureMapSourceListMode> tgt = new Enumeration<>(new StructureMap.StructureMapSourceListModeEnumFactory()); 510 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 511 if (src.getValue() == null) { 512 tgt.setValue(null); 513 } else { 514 switch (src.getValue()) { 515 case FIRST: 516 tgt.setValue(StructureMap.StructureMapSourceListMode.FIRST); 517 break; 518 case NOTFIRST: 519 tgt.setValue(StructureMap.StructureMapSourceListMode.NOTFIRST); 520 break; 521 case LAST: 522 tgt.setValue(StructureMap.StructureMapSourceListMode.LAST); 523 break; 524 case NOTLAST: 525 tgt.setValue(StructureMap.StructureMapSourceListMode.NOTLAST); 526 break; 527 case ONLYONE: 528 tgt.setValue(StructureMap.StructureMapSourceListMode.ONLYONE); 529 break; 530 default: 531 tgt.setValue(StructureMap.StructureMapSourceListMode.NULL); 532 break; 533 } 534 } 535 return tgt; 536 } 537 538 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode> convertStructureMapSourceListMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapSourceListMode> src) throws FHIRException { 539 if (src == null || src.isEmpty()) 540 return null; 541 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListModeEnumFactory()); 542 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 543 if (src.getValue() == null) { 544 tgt.setValue(null); 545 } else { 546 switch (src.getValue()) { 547 case FIRST: 548 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.FIRST); 549 break; 550 case NOTFIRST: 551 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.NOTFIRST); 552 break; 553 case LAST: 554 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.LAST); 555 break; 556 case NOTLAST: 557 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.NOTLAST); 558 break; 559 case ONLYONE: 560 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.ONLYONE); 561 break; 562 default: 563 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapSourceListMode.NULL); 564 break; 565 } 566 } 567 return tgt; 568 } 569 570 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent convertStructureMapGroupRuleTargetComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent src) throws FHIRException { 571 if (src == null) 572 return null; 573 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent(); 574 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 575 if (src.hasContext()) 576 tgt.setContextElement(Id40_50.convertIdToString(src.getContextElement())); 577 if (src.hasContextType() && src.getContextType() != org.hl7.fhir.r4.model.StructureMap.StructureMapContextType.VARIABLE) 578 throw new Error("This conversion is not supported. Consult code maintainers"); // this should never happens - no one knows what the intent was here. 579 if (src.hasElement()) 580 tgt.setElementElement(String40_50.convertString(src.getElementElement())); 581 if (src.hasVariable()) 582 tgt.setVariableElement(Id40_50.convertId(src.getVariableElement())); 583 tgt.setListMode(src.getListMode().stream() 584 .map(StructureMap40_50::convertStructureMapTargetListMode) 585 .collect(Collectors.toList())); 586 if (src.hasListRuleId()) 587 tgt.setListRuleIdElement(Id40_50.convertId(src.getListRuleIdElement())); 588 if (src.hasTransform()) 589 tgt.setTransformElement(convertStructureMapTransform(src.getTransformElement())); 590 for (org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) 591 tgt.addParameter(convertStructureMapGroupRuleTargetParameterComponent(t)); 592 return tgt; 593 } 594 595 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent convertStructureMapGroupRuleTargetComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetComponent src) throws FHIRException { 596 if (src == null) 597 return null; 598 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetComponent(); 599 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 600 if (src.hasContext()) 601 tgt.setContextElement(Id40_50.convertId(src.getContextElement())); 602 tgt.setContextType(org.hl7.fhir.r4.model.StructureMap.StructureMapContextType.VARIABLE); 603 if (src.hasElement()) 604 tgt.setElementElement(String40_50.convertString(src.getElementElement())); 605 if (src.hasVariable()) 606 tgt.setVariableElement(Id40_50.convertId(src.getVariableElement())); 607 tgt.setListMode(src.getListMode().stream() 608 .map(StructureMap40_50::convertStructureMapTargetListMode) 609 .collect(Collectors.toList())); 610 if (src.hasListRuleId()) 611 tgt.setListRuleIdElement(Id40_50.convertId(src.getListRuleIdElement())); 612 if (src.hasTransform()) 613 tgt.setTransformElement(convertStructureMapTransform(src.getTransformElement())); 614 for (org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) 615 tgt.addParameter(convertStructureMapGroupRuleTargetParameterComponent(t)); 616 return tgt; 617 } 618 619 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode> convertStructureMapTargetListMode(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode> src) throws FHIRException { 620 if (src == null || src.isEmpty()) 621 return null; 622 Enumeration<StructureMap.StructureMapTargetListMode> tgt = new Enumeration<>(new StructureMap.StructureMapTargetListModeEnumFactory()); 623 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 624 if (src.getValue() == null) { 625 tgt.setValue(null); 626 } else { 627 switch (src.getValue()) { 628 case FIRST: 629 tgt.setValue(StructureMap.StructureMapTargetListMode.FIRST); 630 break; 631 case SHARE: 632 tgt.setValue(StructureMap.StructureMapTargetListMode.SHARE); 633 break; 634 case LAST: 635 tgt.setValue(StructureMap.StructureMapTargetListMode.LAST); 636 break; 637 case COLLATE: 638 tgt.setValue(StructureMap.StructureMapTargetListMode.SINGLE); 639 break; 640 default: 641 tgt.setValue(StructureMap.StructureMapTargetListMode.NULL); 642 break; 643 } 644 } 645 return tgt; 646 } 647 648 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode> convertStructureMapTargetListMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTargetListMode> src) throws FHIRException { 649 if (src == null || src.isEmpty()) 650 return null; 651 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListModeEnumFactory()); 652 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 653 if (src.getValue() == null) { 654 tgt.setValue(null); 655 } else { 656 switch (src.getValue()) { 657 case FIRST: 658 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.FIRST); 659 break; 660 case SHARE: 661 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.SHARE); 662 break; 663 case LAST: 664 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.LAST); 665 break; 666 case SINGLE: 667 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.COLLATE); 668 break; 669 default: 670 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTargetListMode.NULL); 671 break; 672 } 673 } 674 return tgt; 675 } 676 677 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTransform> convertStructureMapTransform(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTransform> src) throws FHIRException { 678 if (src == null || src.isEmpty()) 679 return null; 680 Enumeration<StructureMap.StructureMapTransform> tgt = new Enumeration<>(new StructureMap.StructureMapTransformEnumFactory()); 681 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 682 if (src.getValue() == null) { 683 tgt.setValue(null); 684 } else { 685 switch (src.getValue()) { 686 case CREATE: 687 tgt.setValue(StructureMap.StructureMapTransform.CREATE); 688 break; 689 case COPY: 690 tgt.setValue(StructureMap.StructureMapTransform.COPY); 691 break; 692 case TRUNCATE: 693 tgt.setValue(StructureMap.StructureMapTransform.TRUNCATE); 694 break; 695 case ESCAPE: 696 tgt.setValue(StructureMap.StructureMapTransform.ESCAPE); 697 break; 698 case CAST: 699 tgt.setValue(StructureMap.StructureMapTransform.CAST); 700 break; 701 case APPEND: 702 tgt.setValue(StructureMap.StructureMapTransform.APPEND); 703 break; 704 case TRANSLATE: 705 tgt.setValue(StructureMap.StructureMapTransform.TRANSLATE); 706 break; 707 case REFERENCE: 708 tgt.setValue(StructureMap.StructureMapTransform.REFERENCE); 709 break; 710 case DATEOP: 711 tgt.setValue(StructureMap.StructureMapTransform.DATEOP); 712 break; 713 case UUID: 714 tgt.setValue(StructureMap.StructureMapTransform.UUID); 715 break; 716 case POINTER: 717 tgt.setValue(StructureMap.StructureMapTransform.POINTER); 718 break; 719 case EVALUATE: 720 tgt.setValue(StructureMap.StructureMapTransform.EVALUATE); 721 break; 722 case CC: 723 tgt.setValue(StructureMap.StructureMapTransform.CC); 724 break; 725 case C: 726 tgt.setValue(StructureMap.StructureMapTransform.C); 727 break; 728 case QTY: 729 tgt.setValue(StructureMap.StructureMapTransform.QTY); 730 break; 731 case ID: 732 tgt.setValue(StructureMap.StructureMapTransform.ID); 733 break; 734 case CP: 735 tgt.setValue(StructureMap.StructureMapTransform.CP); 736 break; 737 default: 738 tgt.setValue(StructureMap.StructureMapTransform.NULL); 739 break; 740 } 741 } 742 return tgt; 743 } 744 745 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTransform> convertStructureMapTransform(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.StructureMap.StructureMapTransform> src) throws FHIRException { 746 if (src == null || src.isEmpty()) 747 return null; 748 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.StructureMap.StructureMapTransform> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.StructureMap.StructureMapTransformEnumFactory()); 749 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 750 if (src.getValue() == null) { 751 tgt.setValue(null); 752 } else { 753 switch (src.getValue()) { 754 case CREATE: 755 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.CREATE); 756 break; 757 case COPY: 758 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.COPY); 759 break; 760 case TRUNCATE: 761 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.TRUNCATE); 762 break; 763 case ESCAPE: 764 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.ESCAPE); 765 break; 766 case CAST: 767 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.CAST); 768 break; 769 case APPEND: 770 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.APPEND); 771 break; 772 case TRANSLATE: 773 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.TRANSLATE); 774 break; 775 case REFERENCE: 776 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.REFERENCE); 777 break; 778 case DATEOP: 779 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.DATEOP); 780 break; 781 case UUID: 782 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.UUID); 783 break; 784 case POINTER: 785 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.POINTER); 786 break; 787 case EVALUATE: 788 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.EVALUATE); 789 break; 790 case CC: 791 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.CC); 792 break; 793 case C: 794 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.C); 795 break; 796 case QTY: 797 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.QTY); 798 break; 799 case ID: 800 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.ID); 801 break; 802 case CP: 803 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.CP); 804 break; 805 default: 806 tgt.setValue(org.hl7.fhir.r4.model.StructureMap.StructureMapTransform.NULL); 807 break; 808 } 809 } 810 return tgt; 811 } 812 813 //DIRTY 814 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent convertStructureMapGroupRuleTargetParameterComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent src) throws FHIRException { 815 if (src == null) 816 return null; 817 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent(); 818 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 819 if (src.hasValue()) 820 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 821 return tgt; 822 } 823 824 825 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent convertStructureMapGroupRuleTargetParameterComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleTargetParameterComponent src) throws FHIRException { 826 if (src == null) 827 return null; 828 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleTargetParameterComponent(); 829 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 830 if (src.hasValue()) 831 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 832 return tgt; 833 } 834 835 //DIRTY 836 public static org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent convertStructureMapGroupRuleDependentComponent(org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent src) throws FHIRException { 837 if (src == null) 838 return null; 839 org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent tgt = new org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent(); 840 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 841 if (src.hasName()) 842 tgt.setNameElement(Id40_50.convertId(src.getNameElement())); 843 for (org.hl7.fhir.r4.model.StringType t : src.getVariable()) tgt.addParameter().setValue(convertVariableStringToParameterDataType(t)); 844 return tgt; 845 } 846 847 public static org.hl7.fhir.r5.model.DataType convertVariableStringToParameterDataType(org.hl7.fhir.r4.model.StringType src) { 848 if (src.hasExtension(ToolingExtensions.EXT_ORIGINAL_VARIABLE_TYPE)) { 849 return ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getExtensionByUrl(ToolingExtensions.EXT_ORIGINAL_VARIABLE_TYPE).getValue()); 850 } else { 851 org.hl7.fhir.r5.model.IdType tgt = new org.hl7.fhir.r5.model.IdType(); 852 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 853 if (src.hasValue()) { 854 tgt.setValueAsString(src.getValueAsString()); 855 } 856 return tgt; 857 } 858 } 859 860 public static org.hl7.fhir.r5.model.DataType convertVariableStringToGuessedParameterDataType(org.hl7.fhir.r4.model.StringType it) { 861 final String stringValue = it.asStringValue(); 862 if (!FHIRPathConstant.isFHIRPathConstant(stringValue)) { 863 return new IdType(stringValue); 864 } else if (FHIRPathConstant.isFHIRPathStringConstant(stringValue)) 865 return new StringType(stringValue); 866 else { 867 return convertVariableStringToGuessedParameterConstantType(stringValue); 868 } 869 } 870 871 public static DataType convertVariableStringToGuessedParameterConstantType(String stringValue) { 872 if (Utilities.isInteger(stringValue)) 873 return new IntegerType(stringValue); 874 else if (Utilities.isDecimal(stringValue, false)) 875 return new DecimalType(stringValue); 876 else if (Utilities.existsInList(stringValue, "true", "false")) 877 return new BooleanType(stringValue.equals("true")); 878 else 879 return new StringType(stringValue); 880 } 881 882 //DIRTY 883 public static org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent convertStructureMapGroupRuleDependentComponent(org.hl7.fhir.r5.model.StructureMap.StructureMapGroupRuleDependentComponent src) throws FHIRException { 884 if (src == null) 885 return null; 886 org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent tgt = new org.hl7.fhir.r4.model.StructureMap.StructureMapGroupRuleDependentComponent(); 887 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 888 if (src.hasName()) 889 tgt.setNameElement(Id40_50.convertId(src.getNameElement())); 890 for (StructureMapGroupRuleTargetParameterComponent t : src.getParameter()) { 891 tgt.getVariable().add(convertStructureMapGroupRuleTargetParameterComponentToString(t)); 892 } 893 return tgt; 894 } 895 896 public static org.hl7.fhir.r4.model.StringType convertStructureMapGroupRuleTargetParameterComponentToString(StructureMapGroupRuleTargetParameterComponent src) { 897 org.hl7.fhir.r4.model.StringType tgt = new org.hl7.fhir.r4.model.StringType(); 898 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 899 if (src.hasValueIdType()) { 900 tgt.setValueAsString(src.getValueIdType().getValueAsString()); 901 } else if (src.hasValue()) { 902 tgt.addExtension(ToolingExtensions.EXT_ORIGINAL_VARIABLE_TYPE,ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 903 } 904 return tgt; 905 } 906}