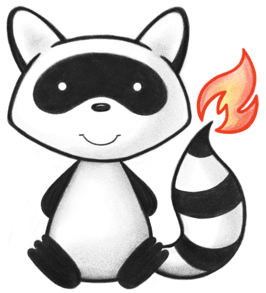
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Ratio40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.SimpleQuantity40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 010import org.hl7.fhir.exceptions.FHIRException; 011import org.hl7.fhir.r5.model.Identifier; 012 013/* 014 Copyright (c) 2011+, HL7, Inc. 015 All rights reserved. 016 017 Redistribution and use in source and binary forms, with or without modification, 018 are permitted provided that the following conditions are met: 019 020 * Redistributions of source code must retain the above copyright notice, this 021 list of conditions and the following disclaimer. 022 * Redistributions in binary form must reproduce the above copyright notice, 023 this list of conditions and the following disclaimer in the documentation 024 and/or other materials provided with the distribution. 025 * Neither the name of HL7 nor the names of its contributors may be used to 026 endorse or promote products derived from this software without specific 027 prior written permission. 028 029 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 030 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 031 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 032 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 033 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 034 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 035 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 036 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 037 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 038 POSSIBILITY OF SUCH DAMAGE. 039 040*/ 041// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 042public class Substance40_50 { 043 044 public static org.hl7.fhir.r5.model.Substance convertSubstance(org.hl7.fhir.r4.model.Substance src) throws FHIRException { 045 if (src == null) 046 return null; 047 org.hl7.fhir.r5.model.Substance tgt = new org.hl7.fhir.r5.model.Substance(); 048 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 049 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 050 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 051 if (src.hasStatus()) 052 tgt.setStatusElement(convertFHIRSubstanceStatus(src.getStatusElement())); 053 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCategory()) 054 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 055 if (src.hasCode()) 056 tgt.getCode().setConcept(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 057 if (src.hasDescription()) 058 tgt.setDescriptionElement(String40_50.convertStringToMarkdown(src.getDescriptionElement())); 059 for (org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent t : src.getInstance()) 060 convertSubstanceInstanceComponent(t, tgt); 061 for (org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 062 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 063 return tgt; 064 } 065 066 public static org.hl7.fhir.r4.model.Substance convertSubstance(org.hl7.fhir.r5.model.Substance src) throws FHIRException { 067 if (src == null) 068 return null; 069 org.hl7.fhir.r4.model.Substance tgt = new org.hl7.fhir.r4.model.Substance(); 070 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 071 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 072 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 073 if (src.hasStatus()) 074 tgt.setStatusElement(convertFHIRSubstanceStatus(src.getStatusElement())); 075 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 076 tgt.addCategory(CodeableConcept40_50.convertCodeableConcept(t)); 077 if (src.getCode().hasConcept()) 078 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode().getConcept())); 079 if (src.hasDescription()) 080 tgt.setDescriptionElement(String40_50.convertString(src.getDescriptionElement())); 081 if (src.getInstance()) { 082 tgt.addInstance(convertSubstanceInstanceComponent(src)); 083 } 084 for (org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent t : src.getIngredient()) 085 tgt.addIngredient(convertSubstanceIngredientComponent(t)); 086 return tgt; 087 } 088 089 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus> convertFHIRSubstanceStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus> src) throws FHIRException { 090 if (src == null || src.isEmpty()) 091 return null; 092 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatusEnumFactory()); 093 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 094 switch (src.getValue()) { 095 case ACTIVE: 096 tgt.setValue(org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus.ACTIVE); 097 break; 098 case INACTIVE: 099 tgt.setValue(org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus.INACTIVE); 100 break; 101 case ENTEREDINERROR: 102 tgt.setValue(org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus.ENTEREDINERROR); 103 break; 104 default: 105 tgt.setValue(org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus.NULL); 106 break; 107 } 108 return tgt; 109 } 110 111 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus> convertFHIRSubstanceStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Substance.FHIRSubstanceStatus> src) throws FHIRException { 112 if (src == null || src.isEmpty()) 113 return null; 114 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatusEnumFactory()); 115 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 116 switch (src.getValue()) { 117 case ACTIVE: 118 tgt.setValue(org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus.ACTIVE); 119 break; 120 case INACTIVE: 121 tgt.setValue(org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus.INACTIVE); 122 break; 123 case ENTEREDINERROR: 124 tgt.setValue(org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus.ENTEREDINERROR); 125 break; 126 default: 127 tgt.setValue(org.hl7.fhir.r4.model.Substance.FHIRSubstanceStatus.NULL); 128 break; 129 } 130 return tgt; 131 } 132 133 public static void convertSubstanceInstanceComponent(org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent src, org.hl7.fhir.r5.model.Substance tgt) throws FHIRException { 134 tgt.setInstance(true); 135 if (src.hasIdentifier()) 136 tgt.addIdentifier(Identifier40_50.convertIdentifier(src.getIdentifier())); 137 if (src.hasExpiry()) 138 tgt.setExpiryElement(DateTime40_50.convertDateTime(src.getExpiryElement())); 139 if (src.hasQuantity()) 140 tgt.setQuantity(SimpleQuantity40_50.convertSimpleQuantity(src.getQuantity())); 141 } 142 143 public static org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent convertSubstanceInstanceComponent(org.hl7.fhir.r5.model.Substance src) throws FHIRException { 144 org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent tgt = new org.hl7.fhir.r4.model.Substance.SubstanceInstanceComponent(); 145 for (Identifier t : src.getIdentifier()) { 146 tgt.setIdentifier(Identifier40_50.convertIdentifier(t)); 147 } 148 if (src.hasExpiry()) 149 tgt.setExpiryElement(DateTime40_50.convertDateTime(src.getExpiryElement())); 150 if (src.hasQuantity()) 151 tgt.setQuantity(SimpleQuantity40_50.convertSimpleQuantity(src.getQuantity())); 152 return tgt; 153 } 154 155 public static org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 156 if (src == null) 157 return null; 158 org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent(); 159 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 160 if (src.hasQuantity()) 161 tgt.setQuantity(Ratio40_50.convertRatio(src.getQuantity())); 162 if (src.hasSubstance()) 163 tgt.setSubstance(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getSubstance())); 164 return tgt; 165 } 166 167 public static org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent convertSubstanceIngredientComponent(org.hl7.fhir.r5.model.Substance.SubstanceIngredientComponent src) throws FHIRException { 168 if (src == null) 169 return null; 170 org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent tgt = new org.hl7.fhir.r4.model.Substance.SubstanceIngredientComponent(); 171 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 172 if (src.hasQuantity()) 173 tgt.setQuantity(Ratio40_50.convertRatio(src.getQuantity())); 174 if (src.hasSubstance()) 175 tgt.setSubstance(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getSubstance())); 176 return tgt; 177 } 178}