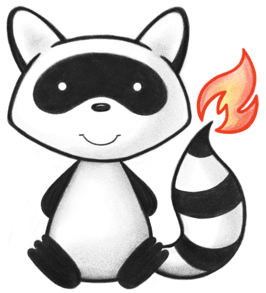
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Attachment40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Boolean40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010/* 011 Copyright (c) 2011+, HL7, Inc. 012 All rights reserved. 013 014 Redistribution and use in source and binary forms, with or without modification, 015 are permitted provided that the following conditions are met: 016 017 * Redistributions of source code must retain the above copyright notice, this 018 list of conditions and the following disclaimer. 019 * Redistributions in binary form must reproduce the above copyright notice, 020 this list of conditions and the following disclaimer in the documentation 021 and/or other materials provided with the distribution. 022 * Neither the name of HL7 nor the names of its contributors may be used to 023 endorse or promote products derived from this software without specific 024 prior written permission. 025 026 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 027 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 028 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 029 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 030 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 031 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 032 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 033 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 034 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 035 POSSIBILITY OF SUCH DAMAGE. 036 037*/ 038// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 039public class SubstancePolymer40_50 { 040 041 public static org.hl7.fhir.r5.model.SubstancePolymer convertSubstancePolymer(org.hl7.fhir.r4.model.SubstancePolymer src) throws FHIRException { 042 if (src == null) 043 return null; 044 org.hl7.fhir.r5.model.SubstancePolymer tgt = new org.hl7.fhir.r5.model.SubstancePolymer(); 045 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 046 if (src.hasClass_()) 047 tgt.setClass_(CodeableConcept40_50.convertCodeableConcept(src.getClass_())); 048 if (src.hasGeometry()) 049 tgt.setGeometry(CodeableConcept40_50.convertCodeableConcept(src.getGeometry())); 050 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCopolymerConnectivity()) 051 tgt.addCopolymerConnectivity(CodeableConcept40_50.convertCodeableConcept(t)); 052 for (org.hl7.fhir.r4.model.StringType t : src.getModification()) 053 tgt.setModificationElement(String40_50.convertString(t)); 054 for (org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetComponent t : src.getMonomerSet()) 055 tgt.addMonomerSet(convertSubstancePolymerMonomerSetComponent(t)); 056 for (org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatComponent t : src.getRepeat()) 057 tgt.addRepeat(convertSubstancePolymerRepeatComponent(t)); 058 return tgt; 059 } 060 061 public static org.hl7.fhir.r4.model.SubstancePolymer convertSubstancePolymer(org.hl7.fhir.r5.model.SubstancePolymer src) throws FHIRException { 062 if (src == null) 063 return null; 064 org.hl7.fhir.r4.model.SubstancePolymer tgt = new org.hl7.fhir.r4.model.SubstancePolymer(); 065 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 066 if (src.hasClass_()) 067 tgt.setClass_(CodeableConcept40_50.convertCodeableConcept(src.getClass_())); 068 if (src.hasGeometry()) 069 tgt.setGeometry(CodeableConcept40_50.convertCodeableConcept(src.getGeometry())); 070 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCopolymerConnectivity()) 071 tgt.addCopolymerConnectivity(CodeableConcept40_50.convertCodeableConcept(t)); 072 if (src.hasModification()) tgt.getModification().add(String40_50.convertString(src.getModificationElement())); 073 for (org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetComponent t : src.getMonomerSet()) 074 tgt.addMonomerSet(convertSubstancePolymerMonomerSetComponent(t)); 075 for (org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatComponent t : src.getRepeat()) 076 tgt.addRepeat(convertSubstancePolymerRepeatComponent(t)); 077 return tgt; 078 } 079 080 public static org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetComponent convertSubstancePolymerMonomerSetComponent(org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetComponent src) throws FHIRException { 081 if (src == null) 082 return null; 083 org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetComponent tgt = new org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetComponent(); 084 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 085 if (src.hasRatioType()) 086 tgt.setRatioType(CodeableConcept40_50.convertCodeableConcept(src.getRatioType())); 087 for (org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent t : src.getStartingMaterial()) 088 tgt.addStartingMaterial(convertSubstancePolymerMonomerSetStartingMaterialComponent(t)); 089 return tgt; 090 } 091 092 public static org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetComponent convertSubstancePolymerMonomerSetComponent(org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetComponent src) throws FHIRException { 093 if (src == null) 094 return null; 095 org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetComponent tgt = new org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetComponent(); 096 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 097 if (src.hasRatioType()) 098 tgt.setRatioType(CodeableConcept40_50.convertCodeableConcept(src.getRatioType())); 099 for (org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent t : src.getStartingMaterial()) 100 tgt.addStartingMaterial(convertSubstancePolymerMonomerSetStartingMaterialComponent(t)); 101 return tgt; 102 } 103 104 public static org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent convertSubstancePolymerMonomerSetStartingMaterialComponent(org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent src) throws FHIRException { 105 if (src == null) 106 return null; 107 org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent tgt = new org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent(); 108 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 109 if (src.hasMaterial()) 110 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getMaterial())); 111 if (src.hasType()) 112 tgt.setCategory(CodeableConcept40_50.convertCodeableConcept(src.getType())); 113 if (src.hasIsDefining()) 114 tgt.setIsDefiningElement(Boolean40_50.convertBoolean(src.getIsDefiningElement())); 115// todo 116// if (src.hasAmount()) 117// tgt.setAmount(convertSubstanceAmount(src.getAmount())); 118 return tgt; 119 } 120 121 public static org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent convertSubstancePolymerMonomerSetStartingMaterialComponent(org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent src) throws FHIRException { 122 if (src == null) 123 return null; 124 org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent tgt = new org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerMonomerSetStartingMaterialComponent(); 125 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 126 if (src.hasCode()) 127 tgt.setMaterial(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 128 if (src.hasType()) 129 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getCategory())); 130 if (src.hasIsDefining()) 131 tgt.setIsDefiningElement(Boolean40_50.convertBoolean(src.getIsDefiningElement())); 132 // todo 133// if (src.hasAmount()) 134// tgt.setAmount(convertSubstanceAmount(src.getAmount())); 135 return tgt; 136 } 137 138 public static org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatComponent convertSubstancePolymerRepeatComponent(org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatComponent src) throws FHIRException { 139 if (src == null) 140 return null; 141 org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatComponent tgt = new org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatComponent(); 142 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 143 // todo 144// if (src.hasNumberOfUnits()) 145// tgt.setNumberOfUnitsElement(convertInteger(src.getNumberOfUnitsElement())); 146 if (src.hasAverageMolecularFormula()) 147 tgt.setAverageMolecularFormulaElement(String40_50.convertString(src.getAverageMolecularFormulaElement())); 148 if (src.hasRepeatUnitAmountType()) 149 tgt.setRepeatUnitAmountType(CodeableConcept40_50.convertCodeableConcept(src.getRepeatUnitAmountType())); 150 for (org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent t : src.getRepeatUnit()) 151 tgt.addRepeatUnit(convertSubstancePolymerRepeatRepeatUnitComponent(t)); 152 return tgt; 153 } 154 155 public static org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatComponent convertSubstancePolymerRepeatComponent(org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatComponent src) throws FHIRException { 156 if (src == null) 157 return null; 158 org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatComponent tgt = new org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatComponent(); 159 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 160 // todo 161// if (src.hasNumberOfUnits()) 162// tgt.setNumberOfUnitsElement(convertInteger(src.getNumberOfUnitsElement())); 163 if (src.hasAverageMolecularFormula()) 164 tgt.setAverageMolecularFormulaElement(String40_50.convertString(src.getAverageMolecularFormulaElement())); 165 if (src.hasRepeatUnitAmountType()) 166 tgt.setRepeatUnitAmountType(CodeableConcept40_50.convertCodeableConcept(src.getRepeatUnitAmountType())); 167 for (org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent t : src.getRepeatUnit()) 168 tgt.addRepeatUnit(convertSubstancePolymerRepeatRepeatUnitComponent(t)); 169 return tgt; 170 } 171 172 public static org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent convertSubstancePolymerRepeatRepeatUnitComponent(org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent src) throws FHIRException { 173 if (src == null) 174 return null; 175 org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent tgt = new org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent(); 176 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 177 if (src.hasOrientationOfPolymerisation()) 178 tgt.setOrientation(CodeableConcept40_50.convertCodeableConcept(src.getOrientationOfPolymerisation())); 179 if (src.hasRepeatUnit()) 180 tgt.setUnitElement(String40_50.convertString(src.getRepeatUnitElement())); 181 // todo 182// if (src.hasAmount()) 183// tgt.setAmount(convertSubstanceAmount(src.getAmount())); 184 for (org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent t : src.getDegreeOfPolymerisation()) 185 tgt.addDegreeOfPolymerisation(convertSubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(t)); 186 for (org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent t : src.getStructuralRepresentation()) 187 tgt.addStructuralRepresentation(convertSubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(t)); 188 return tgt; 189 } 190 191 public static org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent convertSubstancePolymerRepeatRepeatUnitComponent(org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent src) throws FHIRException { 192 if (src == null) 193 return null; 194 org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent tgt = new org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitComponent(); 195 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 196 if (src.hasOrientation()) 197 tgt.setOrientationOfPolymerisation(CodeableConcept40_50.convertCodeableConcept(src.getOrientation())); 198 if (src.hasUnit()) 199 tgt.setRepeatUnitElement(String40_50.convertString(src.getUnitElement())); 200 // todo 201// if (src.hasAmount()) 202// tgt.setAmount(convertSubstanceAmount(src.getAmount())); 203 for (org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent t : src.getDegreeOfPolymerisation()) 204 tgt.addDegreeOfPolymerisation(convertSubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(t)); 205 for (org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent t : src.getStructuralRepresentation()) 206 tgt.addStructuralRepresentation(convertSubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(t)); 207 return tgt; 208 } 209 210 public static org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent convertSubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent src) throws FHIRException { 211 if (src == null) 212 return null; 213 org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent tgt = new org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(); 214 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 215 // todo 216// if (src.hasDegree()) 217// tgt.setDegree(convertCodeableConcept(src.getDegree())); 218// if (src.hasAmount()) 219// tgt.setAmount(convertSubstanceAmount(src.getAmount())); 220 return tgt; 221 } 222 223 public static org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent convertSubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent src) throws FHIRException { 224 if (src == null) 225 return null; 226 org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent tgt = new org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitDegreeOfPolymerisationComponent(); 227 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 228 // todo 229// if (src.hasDegree()) 230// tgt.setDegree(convertCodeableConcept(src.getDegree())); 231// if (src.hasAmount()) 232// tgt.setAmount(convertSubstanceAmount(src.getAmount())); 233 return tgt; 234 } 235 236 public static org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent convertSubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent src) throws FHIRException { 237 if (src == null) 238 return null; 239 org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent tgt = new org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(); 240 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 241 if (src.hasType()) 242 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 243 if (src.hasRepresentation()) 244 tgt.setRepresentationElement(String40_50.convertString(src.getRepresentationElement())); 245 if (src.hasAttachment()) 246 tgt.setAttachment(Attachment40_50.convertAttachment(src.getAttachment())); 247 return tgt; 248 } 249 250 public static org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent convertSubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(org.hl7.fhir.r5.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent src) throws FHIRException { 251 if (src == null) 252 return null; 253 org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent tgt = new org.hl7.fhir.r4.model.SubstancePolymer.SubstancePolymerRepeatRepeatUnitStructuralRepresentationComponent(); 254 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 255 if (src.hasType()) 256 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 257 if (src.hasRepresentation()) 258 tgt.setRepresentationElement(String40_50.convertString(src.getRepresentationElement())); 259 if (src.hasAttachment()) 260 tgt.setAttachment(Attachment40_50.convertAttachment(src.getAttachment())); 261 return tgt; 262 } 263}