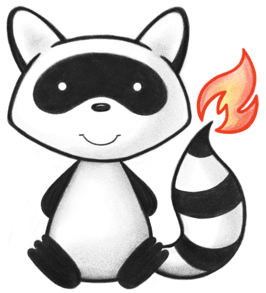
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010/* 011 Copyright (c) 2011+, HL7, Inc. 012 All rights reserved. 013 014 Redistribution and use in source and binary forms, with or without modification, 015 are permitted provided that the following conditions are met: 016 017 * Redistributions of source code must retain the above copyright notice, this 018 list of conditions and the following disclaimer. 019 * Redistributions in binary form must reproduce the above copyright notice, 020 this list of conditions and the following disclaimer in the documentation 021 and/or other materials provided with the distribution. 022 * Neither the name of HL7 nor the names of its contributors may be used to 023 endorse or promote products derived from this software without specific 024 prior written permission. 025 026 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 027 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 028 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 029 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 030 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 031 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 032 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 033 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 034 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 035 POSSIBILITY OF SUCH DAMAGE. 036 037*/ 038// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 039public class SubstanceReferenceInformation40_50 { 040 041 public static org.hl7.fhir.r5.model.SubstanceReferenceInformation convertSubstanceReferenceInformation(org.hl7.fhir.r4.model.SubstanceReferenceInformation src) throws FHIRException { 042 if (src == null) 043 return null; 044 org.hl7.fhir.r5.model.SubstanceReferenceInformation tgt = new org.hl7.fhir.r5.model.SubstanceReferenceInformation(); 045 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 046 if (src.hasComment()) 047 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 048 for (org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent t : src.getGene()) 049 tgt.addGene(convertSubstanceReferenceInformationGeneComponent(t)); 050 for (org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent t : src.getGeneElement()) 051 tgt.addGeneElement(convertSubstanceReferenceInformationGeneElementComponent(t)); 052// for (org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent t : src.getClassification()) tgt.addClassification(convertSubstanceReferenceInformationClassificationComponent(t)); 053 for (org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent t : src.getTarget()) 054 tgt.addTarget(convertSubstanceReferenceInformationTargetComponent(t)); 055 return tgt; 056 } 057 058 public static org.hl7.fhir.r4.model.SubstanceReferenceInformation convertSubstanceReferenceInformation(org.hl7.fhir.r5.model.SubstanceReferenceInformation src) throws FHIRException { 059 if (src == null) 060 return null; 061 org.hl7.fhir.r4.model.SubstanceReferenceInformation tgt = new org.hl7.fhir.r4.model.SubstanceReferenceInformation(); 062 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 063 if (src.hasComment()) 064 tgt.setCommentElement(String40_50.convertString(src.getCommentElement())); 065 for (org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent t : src.getGene()) 066 tgt.addGene(convertSubstanceReferenceInformationGeneComponent(t)); 067 for (org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent t : src.getGeneElement()) 068 tgt.addGeneElement(convertSubstanceReferenceInformationGeneElementComponent(t)); 069// for (org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent t : src.getClassification()) tgt.addClassification(convertSubstanceReferenceInformationClassificationComponent(t)); 070 for (org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent t : src.getTarget()) 071 tgt.addTarget(convertSubstanceReferenceInformationTargetComponent(t)); 072 return tgt; 073 } 074 075 public static org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent convertSubstanceReferenceInformationGeneComponent(org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent src) throws FHIRException { 076 if (src == null) 077 return null; 078 org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent tgt = new org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent(); 079 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 080 if (src.hasGeneSequenceOrigin()) 081 tgt.setGeneSequenceOrigin(CodeableConcept40_50.convertCodeableConcept(src.getGeneSequenceOrigin())); 082 if (src.hasGene()) 083 tgt.setGene(CodeableConcept40_50.convertCodeableConcept(src.getGene())); 084 for (org.hl7.fhir.r4.model.Reference t : src.getSource()) tgt.addSource(Reference40_50.convertReference(t)); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent convertSubstanceReferenceInformationGeneComponent(org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent src) throws FHIRException { 089 if (src == null) 090 return null; 091 org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent tgt = new org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneComponent(); 092 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 093 if (src.hasGeneSequenceOrigin()) 094 tgt.setGeneSequenceOrigin(CodeableConcept40_50.convertCodeableConcept(src.getGeneSequenceOrigin())); 095 if (src.hasGene()) 096 tgt.setGene(CodeableConcept40_50.convertCodeableConcept(src.getGene())); 097 for (org.hl7.fhir.r5.model.Reference t : src.getSource()) tgt.addSource(Reference40_50.convertReference(t)); 098 return tgt; 099 } 100 101 public static org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent convertSubstanceReferenceInformationGeneElementComponent(org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent src) throws FHIRException { 102 if (src == null) 103 return null; 104 org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent tgt = new org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent(); 105 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 106 if (src.hasType()) 107 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 108 if (src.hasElement()) 109 tgt.setElement(Identifier40_50.convertIdentifier(src.getElement())); 110 for (org.hl7.fhir.r4.model.Reference t : src.getSource()) tgt.addSource(Reference40_50.convertReference(t)); 111 return tgt; 112 } 113 114 public static org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent convertSubstanceReferenceInformationGeneElementComponent(org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent src) throws FHIRException { 115 if (src == null) 116 return null; 117 org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent tgt = new org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationGeneElementComponent(); 118 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 119 if (src.hasType()) 120 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 121 if (src.hasElement()) 122 tgt.setElement(Identifier40_50.convertIdentifier(src.getElement())); 123 for (org.hl7.fhir.r5.model.Reference t : src.getSource()) tgt.addSource(Reference40_50.convertReference(t)); 124 return tgt; 125 } 126 127 // public static org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent convertSubstanceReferenceInformationClassificationComponent(org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent src) throws FHIRException { 128// if (src == null) 129// return null; 130// org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent tgt = new org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent(); 131// copyElement(src, tgt); 132// if (src.hasDomain()) 133// tgt.setDomain(convertCodeableConcept(src.getDomain())); 134// if (src.hasClassification()) 135// tgt.setClassification(convertCodeableConcept(src.getClassification())); 136// for (org.hl7.fhir.r4.model.CodeableConcept t : src.getSubtype()) tgt.addSubtype(convertCodeableConcept(t)); 137// for (org.hl7.fhir.r4.model.Reference t : src.getSource()) tgt.addSource(convertReference(t)); 138// return tgt; 139// } 140// 141// public static org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent convertSubstanceReferenceInformationClassificationComponent(org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent src) throws FHIRException { 142// if (src == null) 143// return null; 144// org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent tgt = new org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationClassificationComponent(); 145// copyElement(src, tgt); 146// if (src.hasDomain()) 147// tgt.setDomain(convertCodeableConcept(src.getDomain())); 148// if (src.hasClassification()) 149// tgt.setClassification(convertCodeableConcept(src.getClassification())); 150// for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSubtype()) tgt.addSubtype(convertCodeableConcept(t)); 151// for (org.hl7.fhir.r5.model.Reference t : src.getSource()) tgt.addSource(convertReference(t)); 152// return tgt; 153// } 154// 155 public static org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent convertSubstanceReferenceInformationTargetComponent(org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent src) throws FHIRException { 156 if (src == null) 157 return null; 158 org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent tgt = new org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent(); 159 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 160 if (src.hasTarget()) 161 tgt.setTarget(Identifier40_50.convertIdentifier(src.getTarget())); 162 if (src.hasType()) 163 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 164 if (src.hasInteraction()) 165 tgt.setInteraction(CodeableConcept40_50.convertCodeableConcept(src.getInteraction())); 166 if (src.hasOrganism()) 167 tgt.setOrganism(CodeableConcept40_50.convertCodeableConcept(src.getOrganism())); 168 if (src.hasOrganismType()) 169 tgt.setOrganismType(CodeableConcept40_50.convertCodeableConcept(src.getOrganismType())); 170 if (src.hasAmount()) 171 tgt.setAmount(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getAmount())); 172 if (src.hasAmountType()) 173 tgt.setAmountType(CodeableConcept40_50.convertCodeableConcept(src.getAmountType())); 174 for (org.hl7.fhir.r4.model.Reference t : src.getSource()) tgt.addSource(Reference40_50.convertReference(t)); 175 return tgt; 176 } 177 178 public static org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent convertSubstanceReferenceInformationTargetComponent(org.hl7.fhir.r5.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent src) throws FHIRException { 179 if (src == null) 180 return null; 181 org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent tgt = new org.hl7.fhir.r4.model.SubstanceReferenceInformation.SubstanceReferenceInformationTargetComponent(); 182 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 183 if (src.hasTarget()) 184 tgt.setTarget(Identifier40_50.convertIdentifier(src.getTarget())); 185 if (src.hasType()) 186 tgt.setType(CodeableConcept40_50.convertCodeableConcept(src.getType())); 187 if (src.hasInteraction()) 188 tgt.setInteraction(CodeableConcept40_50.convertCodeableConcept(src.getInteraction())); 189 if (src.hasOrganism()) 190 tgt.setOrganism(CodeableConcept40_50.convertCodeableConcept(src.getOrganism())); 191 if (src.hasOrganismType()) 192 tgt.setOrganismType(CodeableConcept40_50.convertCodeableConcept(src.getOrganismType())); 193 if (src.hasAmount()) 194 tgt.setAmount(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getAmount())); 195 if (src.hasAmountType()) 196 tgt.setAmountType(CodeableConcept40_50.convertCodeableConcept(src.getAmountType())); 197 for (org.hl7.fhir.r5.model.Reference t : src.getSource()) tgt.addSource(Reference40_50.convertReference(t)); 198 return tgt; 199 } 200}