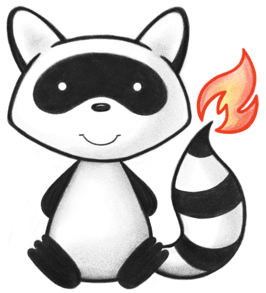
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Identifier40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Quantity40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 009import org.hl7.fhir.exceptions.FHIRException; 010import org.hl7.fhir.r5.model.CodeableReference; 011import org.hl7.fhir.r5.model.Enumeration; 012import org.hl7.fhir.r5.model.Enumerations; 013import org.hl7.fhir.r5.model.SupplyRequest; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class SupplyRequest40_50 { 045 046 public static org.hl7.fhir.r5.model.SupplyRequest convertSupplyRequest(org.hl7.fhir.r4.model.SupplyRequest src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.SupplyRequest tgt = new org.hl7.fhir.r5.model.SupplyRequest(); 050 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertSupplyRequestStatus(src.getStatusElement())); 055 if (src.hasCategory()) 056 tgt.setCategory(CodeableConcept40_50.convertCodeableConcept(src.getCategory())); 057 if (src.hasPriority()) 058 tgt.setPriorityElement(convertRequestPriority(src.getPriorityElement())); 059 if (src.hasItem()) { 060 if (src.hasItemCodeableConcept()) { 061 tgt.getItem().setConcept(CodeableConcept40_50.convertCodeableConcept(src.getItemCodeableConcept())); 062 } else { 063 tgt.getItem().setReference(Reference40_50.convertReference(src.getItemReference())); 064 } 065 } 066 if (src.hasQuantity()) 067 tgt.setQuantity(Quantity40_50.convertQuantity(src.getQuantity())); 068 for (org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestParameterComponent t : src.getParameter()) 069 tgt.addParameter(convertSupplyRequestParameterComponent(t)); 070 if (src.hasOccurrence()) 071 tgt.setOccurrence(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOccurrence())); 072 if (src.hasAuthoredOn()) 073 tgt.setAuthoredOnElement(DateTime40_50.convertDateTime(src.getAuthoredOnElement())); 074 if (src.hasRequester()) 075 tgt.setRequester(Reference40_50.convertReference(src.getRequester())); 076 for (org.hl7.fhir.r4.model.Reference t : src.getSupplier()) tgt.addSupplier(Reference40_50.convertReference(t)); 077 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getReasonCode()) 078 tgt.addReason(CodeableConcept40_50.convertCodeableConceptToCodeableReference(t)); 079 for (org.hl7.fhir.r4.model.Reference t : src.getReasonReference()) 080 tgt.addReason(Reference40_50.convertReferenceToCodeableReference(t)); 081 if (src.hasDeliverFrom()) 082 tgt.setDeliverFrom(Reference40_50.convertReference(src.getDeliverFrom())); 083 if (src.hasDeliverTo()) 084 tgt.setDeliverTo(Reference40_50.convertReference(src.getDeliverTo())); 085 return tgt; 086 } 087 088 public static org.hl7.fhir.r4.model.SupplyRequest convertSupplyRequest(org.hl7.fhir.r5.model.SupplyRequest src) throws FHIRException { 089 if (src == null) 090 return null; 091 org.hl7.fhir.r4.model.SupplyRequest tgt = new org.hl7.fhir.r4.model.SupplyRequest(); 092 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 093 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 094 tgt.addIdentifier(Identifier40_50.convertIdentifier(t)); 095 if (src.hasStatus()) 096 tgt.setStatusElement(convertSupplyRequestStatus(src.getStatusElement())); 097 if (src.hasCategory()) 098 tgt.setCategory(CodeableConcept40_50.convertCodeableConcept(src.getCategory())); 099 if (src.hasPriority()) 100 tgt.setPriorityElement(convertRequestPriority(src.getPriorityElement())); 101 if (src.hasItem()) { 102 if (src.getItem().hasReference()) { 103 tgt.setItem(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getItem().getReference())); 104 } else { 105 tgt.setItem(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getItem().getConcept())); 106 } 107 } 108 if (src.hasQuantity()) 109 tgt.setQuantity(Quantity40_50.convertQuantity(src.getQuantity())); 110 for (org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestParameterComponent t : src.getParameter()) 111 tgt.addParameter(convertSupplyRequestParameterComponent(t)); 112 if (src.hasOccurrence()) 113 tgt.setOccurrence(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getOccurrence())); 114 if (src.hasAuthoredOn()) 115 tgt.setAuthoredOnElement(DateTime40_50.convertDateTime(src.getAuthoredOnElement())); 116 if (src.hasRequester()) 117 tgt.setRequester(Reference40_50.convertReference(src.getRequester())); 118 for (org.hl7.fhir.r5.model.Reference t : src.getSupplier()) tgt.addSupplier(Reference40_50.convertReference(t)); 119 for (CodeableReference t : src.getReason()) 120 if (t.hasConcept()) 121 tgt.addReasonCode(CodeableConcept40_50.convertCodeableConcept(t.getConcept())); 122 for (CodeableReference t : src.getReason()) 123 if (t.hasReference()) 124 tgt.addReasonReference(Reference40_50.convertReference(t.getReference())); 125 if (src.hasDeliverFrom()) 126 tgt.setDeliverFrom(Reference40_50.convertReference(src.getDeliverFrom())); 127 if (src.hasDeliverTo()) 128 tgt.setDeliverTo(Reference40_50.convertReference(src.getDeliverTo())); 129 return tgt; 130 } 131 132 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestStatus> convertSupplyRequestStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus> src) throws FHIRException { 133 if (src == null || src.isEmpty()) 134 return null; 135 Enumeration<SupplyRequest.SupplyRequestStatus> tgt = new Enumeration<>(new SupplyRequest.SupplyRequestStatusEnumFactory()); 136 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 137 if (src.getValue() == null) { 138 tgt.setValue(null); 139 } else { 140 switch (src.getValue()) { 141 case DRAFT: 142 tgt.setValue(SupplyRequest.SupplyRequestStatus.DRAFT); 143 break; 144 case ACTIVE: 145 tgt.setValue(SupplyRequest.SupplyRequestStatus.ACTIVE); 146 break; 147 case SUSPENDED: 148 tgt.setValue(SupplyRequest.SupplyRequestStatus.SUSPENDED); 149 break; 150 case CANCELLED: 151 tgt.setValue(SupplyRequest.SupplyRequestStatus.CANCELLED); 152 break; 153 case COMPLETED: 154 tgt.setValue(SupplyRequest.SupplyRequestStatus.COMPLETED); 155 break; 156 case ENTEREDINERROR: 157 tgt.setValue(SupplyRequest.SupplyRequestStatus.ENTEREDINERROR); 158 break; 159 case UNKNOWN: 160 tgt.setValue(SupplyRequest.SupplyRequestStatus.UNKNOWN); 161 break; 162 default: 163 tgt.setValue(SupplyRequest.SupplyRequestStatus.NULL); 164 break; 165 } 166 } 167 return tgt; 168 } 169 170 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus> convertSupplyRequestStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestStatus> src) throws FHIRException { 171 if (src == null || src.isEmpty()) 172 return null; 173 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatusEnumFactory()); 174 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 175 if (src.getValue() == null) { 176 tgt.setValue(null); 177 } else { 178 switch (src.getValue()) { 179 case DRAFT: 180 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus.DRAFT); 181 break; 182 case ACTIVE: 183 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus.ACTIVE); 184 break; 185 case SUSPENDED: 186 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus.SUSPENDED); 187 break; 188 case CANCELLED: 189 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus.CANCELLED); 190 break; 191 case COMPLETED: 192 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus.COMPLETED); 193 break; 194 case ENTEREDINERROR: 195 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus.ENTEREDINERROR); 196 break; 197 case UNKNOWN: 198 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus.UNKNOWN); 199 break; 200 default: 201 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestStatus.NULL); 202 break; 203 } 204 } 205 return tgt; 206 } 207 208 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertRequestPriority(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.RequestPriority> src) throws FHIRException { 209 if (src == null || src.isEmpty()) 210 return null; 211 Enumeration<Enumerations.RequestPriority> tgt = new Enumeration<>(new Enumerations.RequestPriorityEnumFactory()); 212 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 213 if (src.getValue() == null) { 214 tgt.setValue(null); 215 } else { 216 switch (src.getValue()) { 217 case ROUTINE: 218 tgt.setValue(Enumerations.RequestPriority.ROUTINE); 219 break; 220 case URGENT: 221 tgt.setValue(Enumerations.RequestPriority.URGENT); 222 break; 223 case ASAP: 224 tgt.setValue(Enumerations.RequestPriority.ASAP); 225 break; 226 case STAT: 227 tgt.setValue(Enumerations.RequestPriority.STAT); 228 break; 229 default: 230 tgt.setValue(Enumerations.RequestPriority.NULL); 231 break; 232 } 233 } 234 return tgt; 235 } 236 237 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.RequestPriority> convertRequestPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 238 if (src == null || src.isEmpty()) 239 return null; 240 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.SupplyRequest.RequestPriority> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.SupplyRequest.RequestPriorityEnumFactory()); 241 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 242 if (src.getValue() == null) { 243 tgt.setValue(null); 244 } else { 245 switch (src.getValue()) { 246 case ROUTINE: 247 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.RequestPriority.ROUTINE); 248 break; 249 case URGENT: 250 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.RequestPriority.URGENT); 251 break; 252 case ASAP: 253 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.RequestPriority.ASAP); 254 break; 255 case STAT: 256 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.RequestPriority.STAT); 257 break; 258 default: 259 tgt.setValue(org.hl7.fhir.r4.model.SupplyRequest.RequestPriority.NULL); 260 break; 261 } 262 } 263 return tgt; 264 } 265 266 public static org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestParameterComponent convertSupplyRequestParameterComponent(org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestParameterComponent src) throws FHIRException { 267 if (src == null) 268 return null; 269 org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestParameterComponent tgt = new org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestParameterComponent(); 270 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 271 if (src.hasCode()) 272 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 273 if (src.hasValue()) 274 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 275 return tgt; 276 } 277 278 public static org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestParameterComponent convertSupplyRequestParameterComponent(org.hl7.fhir.r5.model.SupplyRequest.SupplyRequestParameterComponent src) throws FHIRException { 279 if (src == null) 280 return null; 281 org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestParameterComponent tgt = new org.hl7.fhir.r4.model.SupplyRequest.SupplyRequestParameterComponent(); 282 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 283 if (src.hasCode()) 284 tgt.setCode(CodeableConcept40_50.convertCodeableConcept(src.getCode())); 285 if (src.hasValue()) 286 tgt.setValue(ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().convertType(src.getValue())); 287 return tgt; 288 } 289}