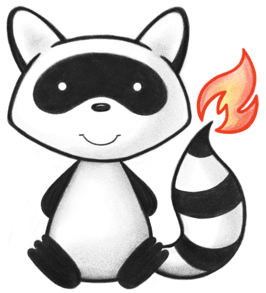
001package org.hl7.fhir.convertors.conv40_50.resources40_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext40_50; 004import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.CodeableConcept40_50; 005import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Signature40_50; 006import org.hl7.fhir.convertors.conv40_50.datatypes40_50.general40_50.Timing40_50; 007import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.Date40_50; 008import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.DateTime40_50; 009import org.hl7.fhir.convertors.conv40_50.datatypes40_50.primitive40_50.String40_50; 010import org.hl7.fhir.convertors.conv40_50.datatypes40_50.special40_50.Reference40_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.Enumeration; 013import org.hl7.fhir.r5.model.VerificationResult; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class VerificationResult40_50 { 045 046 public static org.hl7.fhir.r5.model.VerificationResult convertVerificationResult(org.hl7.fhir.r4.model.VerificationResult src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.VerificationResult tgt = new org.hl7.fhir.r5.model.VerificationResult(); 050 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4.model.Reference t : src.getTarget()) tgt.addTarget(Reference40_50.convertReference(t)); 052 for (org.hl7.fhir.r4.model.StringType t : src.getTargetLocation()) 053 tgt.getTargetLocation().add(String40_50.convertString(t)); 054 if (src.hasNeed()) 055 tgt.setNeed(CodeableConcept40_50.convertCodeableConcept(src.getNeed())); 056 if (src.hasStatus()) 057 tgt.setStatusElement(convertStatus(src.getStatusElement())); 058 if (src.hasStatusDate()) 059 tgt.setStatusDateElement(DateTime40_50.convertDateTime(src.getStatusDateElement())); 060 if (src.hasValidationType()) 061 tgt.setValidationType(CodeableConcept40_50.convertCodeableConcept(src.getValidationType())); 062 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getValidationProcess()) 063 tgt.addValidationProcess(CodeableConcept40_50.convertCodeableConcept(t)); 064 if (src.hasFrequency()) 065 tgt.setFrequency(Timing40_50.convertTiming(src.getFrequency())); 066 if (src.hasLastPerformed()) 067 tgt.setLastPerformedElement(DateTime40_50.convertDateTime(src.getLastPerformedElement())); 068 if (src.hasNextScheduled()) 069 tgt.setNextScheduledElement(Date40_50.convertDate(src.getNextScheduledElement())); 070 if (src.hasFailureAction()) 071 tgt.setFailureAction(CodeableConcept40_50.convertCodeableConcept(src.getFailureAction())); 072 for (org.hl7.fhir.r4.model.VerificationResult.VerificationResultPrimarySourceComponent t : src.getPrimarySource()) 073 tgt.addPrimarySource(convertVerificationResultPrimarySourceComponent(t)); 074 if (src.hasAttestation()) 075 tgt.setAttestation(convertVerificationResultAttestationComponent(src.getAttestation())); 076 for (org.hl7.fhir.r4.model.VerificationResult.VerificationResultValidatorComponent t : src.getValidator()) 077 tgt.addValidator(convertVerificationResultValidatorComponent(t)); 078 return tgt; 079 } 080 081 public static org.hl7.fhir.r4.model.VerificationResult convertVerificationResult(org.hl7.fhir.r5.model.VerificationResult src) throws FHIRException { 082 if (src == null) 083 return null; 084 org.hl7.fhir.r4.model.VerificationResult tgt = new org.hl7.fhir.r4.model.VerificationResult(); 085 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyDomainResource(src, tgt); 086 for (org.hl7.fhir.r5.model.Reference t : src.getTarget()) tgt.addTarget(Reference40_50.convertReference(t)); 087 for (org.hl7.fhir.r5.model.StringType t : src.getTargetLocation()) 088 tgt.getTargetLocation().add(String40_50.convertString(t)); 089 if (src.hasNeed()) 090 tgt.setNeed(CodeableConcept40_50.convertCodeableConcept(src.getNeed())); 091 if (src.hasStatus()) 092 tgt.setStatusElement(convertStatus(src.getStatusElement())); 093 if (src.hasStatusDate()) 094 tgt.setStatusDateElement(DateTime40_50.convertDateTime(src.getStatusDateElement())); 095 if (src.hasValidationType()) 096 tgt.setValidationType(CodeableConcept40_50.convertCodeableConcept(src.getValidationType())); 097 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getValidationProcess()) 098 tgt.addValidationProcess(CodeableConcept40_50.convertCodeableConcept(t)); 099 if (src.hasFrequency()) 100 tgt.setFrequency(Timing40_50.convertTiming(src.getFrequency())); 101 if (src.hasLastPerformed()) 102 tgt.setLastPerformedElement(DateTime40_50.convertDateTime(src.getLastPerformedElement())); 103 if (src.hasNextScheduled()) 104 tgt.setNextScheduledElement(Date40_50.convertDate(src.getNextScheduledElement())); 105 if (src.hasFailureAction()) 106 tgt.setFailureAction(CodeableConcept40_50.convertCodeableConcept(src.getFailureAction())); 107 for (org.hl7.fhir.r5.model.VerificationResult.VerificationResultPrimarySourceComponent t : src.getPrimarySource()) 108 tgt.addPrimarySource(convertVerificationResultPrimarySourceComponent(t)); 109 if (src.hasAttestation()) 110 tgt.setAttestation(convertVerificationResultAttestationComponent(src.getAttestation())); 111 for (org.hl7.fhir.r5.model.VerificationResult.VerificationResultValidatorComponent t : src.getValidator()) 112 tgt.addValidator(convertVerificationResultValidatorComponent(t)); 113 return tgt; 114 } 115 116 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.VerificationResult.VerificationResultStatus> convertStatus(org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.VerificationResult.Status> src) throws FHIRException { 117 if (src == null || src.isEmpty()) 118 return null; 119 Enumeration<VerificationResult.VerificationResultStatus> tgt = new Enumeration<>(new VerificationResult.VerificationResultStatusEnumFactory()); 120 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 121 if (src.getValue() == null) { 122 tgt.setValue(null); 123 } else { 124 switch (src.getValue()) { 125 case ATTESTED: 126 tgt.setValue(VerificationResult.VerificationResultStatus.ATTESTED); 127 break; 128 case VALIDATED: 129 tgt.setValue(VerificationResult.VerificationResultStatus.VALIDATED); 130 break; 131 case INPROCESS: 132 tgt.setValue(VerificationResult.VerificationResultStatus.INPROCESS); 133 break; 134 case REQREVALID: 135 tgt.setValue(VerificationResult.VerificationResultStatus.REQREVALID); 136 break; 137 case VALFAIL: 138 tgt.setValue(VerificationResult.VerificationResultStatus.VALFAIL); 139 break; 140 case REVALFAIL: 141 tgt.setValue(VerificationResult.VerificationResultStatus.REVALFAIL); 142 break; 143 default: 144 tgt.setValue(VerificationResult.VerificationResultStatus.NULL); 145 break; 146 } 147 } 148 return tgt; 149 } 150 151 static public org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.VerificationResult.Status> convertStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.VerificationResult.VerificationResultStatus> src) throws FHIRException { 152 if (src == null || src.isEmpty()) 153 return null; 154 org.hl7.fhir.r4.model.Enumeration<org.hl7.fhir.r4.model.VerificationResult.Status> tgt = new org.hl7.fhir.r4.model.Enumeration<>(new org.hl7.fhir.r4.model.VerificationResult.StatusEnumFactory()); 155 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyElement(src, tgt); 156 if (src.getValue() == null) { 157 tgt.setValue(null); 158 } else { 159 switch (src.getValue()) { 160 case ATTESTED: 161 tgt.setValue(org.hl7.fhir.r4.model.VerificationResult.Status.ATTESTED); 162 break; 163 case VALIDATED: 164 tgt.setValue(org.hl7.fhir.r4.model.VerificationResult.Status.VALIDATED); 165 break; 166 case INPROCESS: 167 tgt.setValue(org.hl7.fhir.r4.model.VerificationResult.Status.INPROCESS); 168 break; 169 case REQREVALID: 170 tgt.setValue(org.hl7.fhir.r4.model.VerificationResult.Status.REQREVALID); 171 break; 172 case VALFAIL: 173 tgt.setValue(org.hl7.fhir.r4.model.VerificationResult.Status.VALFAIL); 174 break; 175 case REVALFAIL: 176 tgt.setValue(org.hl7.fhir.r4.model.VerificationResult.Status.REVALFAIL); 177 break; 178 default: 179 tgt.setValue(org.hl7.fhir.r4.model.VerificationResult.Status.NULL); 180 break; 181 } 182 } 183 return tgt; 184 } 185 186 public static org.hl7.fhir.r5.model.VerificationResult.VerificationResultPrimarySourceComponent convertVerificationResultPrimarySourceComponent(org.hl7.fhir.r4.model.VerificationResult.VerificationResultPrimarySourceComponent src) throws FHIRException { 187 if (src == null) 188 return null; 189 org.hl7.fhir.r5.model.VerificationResult.VerificationResultPrimarySourceComponent tgt = new org.hl7.fhir.r5.model.VerificationResult.VerificationResultPrimarySourceComponent(); 190 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 191 if (src.hasWho()) 192 tgt.setWho(Reference40_50.convertReference(src.getWho())); 193 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getType()) 194 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 195 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getCommunicationMethod()) 196 tgt.addCommunicationMethod(CodeableConcept40_50.convertCodeableConcept(t)); 197 if (src.hasValidationStatus()) 198 tgt.setValidationStatus(CodeableConcept40_50.convertCodeableConcept(src.getValidationStatus())); 199 if (src.hasValidationDate()) 200 tgt.setValidationDateElement(DateTime40_50.convertDateTime(src.getValidationDateElement())); 201 if (src.hasCanPushUpdates()) 202 tgt.setCanPushUpdates(CodeableConcept40_50.convertCodeableConcept(src.getCanPushUpdates())); 203 for (org.hl7.fhir.r4.model.CodeableConcept t : src.getPushTypeAvailable()) 204 tgt.addPushTypeAvailable(CodeableConcept40_50.convertCodeableConcept(t)); 205 return tgt; 206 } 207 208 public static org.hl7.fhir.r4.model.VerificationResult.VerificationResultPrimarySourceComponent convertVerificationResultPrimarySourceComponent(org.hl7.fhir.r5.model.VerificationResult.VerificationResultPrimarySourceComponent src) throws FHIRException { 209 if (src == null) 210 return null; 211 org.hl7.fhir.r4.model.VerificationResult.VerificationResultPrimarySourceComponent tgt = new org.hl7.fhir.r4.model.VerificationResult.VerificationResultPrimarySourceComponent(); 212 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 213 if (src.hasWho()) 214 tgt.setWho(Reference40_50.convertReference(src.getWho())); 215 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 216 tgt.addType(CodeableConcept40_50.convertCodeableConcept(t)); 217 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCommunicationMethod()) 218 tgt.addCommunicationMethod(CodeableConcept40_50.convertCodeableConcept(t)); 219 if (src.hasValidationStatus()) 220 tgt.setValidationStatus(CodeableConcept40_50.convertCodeableConcept(src.getValidationStatus())); 221 if (src.hasValidationDate()) 222 tgt.setValidationDateElement(DateTime40_50.convertDateTime(src.getValidationDateElement())); 223 if (src.hasCanPushUpdates()) 224 tgt.setCanPushUpdates(CodeableConcept40_50.convertCodeableConcept(src.getCanPushUpdates())); 225 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getPushTypeAvailable()) 226 tgt.addPushTypeAvailable(CodeableConcept40_50.convertCodeableConcept(t)); 227 return tgt; 228 } 229 230 public static org.hl7.fhir.r5.model.VerificationResult.VerificationResultAttestationComponent convertVerificationResultAttestationComponent(org.hl7.fhir.r4.model.VerificationResult.VerificationResultAttestationComponent src) throws FHIRException { 231 if (src == null) 232 return null; 233 org.hl7.fhir.r5.model.VerificationResult.VerificationResultAttestationComponent tgt = new org.hl7.fhir.r5.model.VerificationResult.VerificationResultAttestationComponent(); 234 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 235 if (src.hasWho()) 236 tgt.setWho(Reference40_50.convertReference(src.getWho())); 237 if (src.hasOnBehalfOf()) 238 tgt.setOnBehalfOf(Reference40_50.convertReference(src.getOnBehalfOf())); 239 if (src.hasCommunicationMethod()) 240 tgt.setCommunicationMethod(CodeableConcept40_50.convertCodeableConcept(src.getCommunicationMethod())); 241 if (src.hasDate()) 242 tgt.setDateElement(Date40_50.convertDate(src.getDateElement())); 243 if (src.hasSourceIdentityCertificate()) 244 tgt.setSourceIdentityCertificateElement(String40_50.convertString(src.getSourceIdentityCertificateElement())); 245 if (src.hasProxyIdentityCertificate()) 246 tgt.setProxyIdentityCertificateElement(String40_50.convertString(src.getProxyIdentityCertificateElement())); 247 if (src.hasProxySignature()) 248 tgt.setProxySignature(Signature40_50.convertSignature(src.getProxySignature())); 249 if (src.hasSourceSignature()) 250 tgt.setSourceSignature(Signature40_50.convertSignature(src.getSourceSignature())); 251 return tgt; 252 } 253 254 public static org.hl7.fhir.r4.model.VerificationResult.VerificationResultAttestationComponent convertVerificationResultAttestationComponent(org.hl7.fhir.r5.model.VerificationResult.VerificationResultAttestationComponent src) throws FHIRException { 255 if (src == null) 256 return null; 257 org.hl7.fhir.r4.model.VerificationResult.VerificationResultAttestationComponent tgt = new org.hl7.fhir.r4.model.VerificationResult.VerificationResultAttestationComponent(); 258 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 259 if (src.hasWho()) 260 tgt.setWho(Reference40_50.convertReference(src.getWho())); 261 if (src.hasOnBehalfOf()) 262 tgt.setOnBehalfOf(Reference40_50.convertReference(src.getOnBehalfOf())); 263 if (src.hasCommunicationMethod()) 264 tgt.setCommunicationMethod(CodeableConcept40_50.convertCodeableConcept(src.getCommunicationMethod())); 265 if (src.hasDate()) 266 tgt.setDateElement(Date40_50.convertDate(src.getDateElement())); 267 if (src.hasSourceIdentityCertificate()) 268 tgt.setSourceIdentityCertificateElement(String40_50.convertString(src.getSourceIdentityCertificateElement())); 269 if (src.hasProxyIdentityCertificate()) 270 tgt.setProxyIdentityCertificateElement(String40_50.convertString(src.getProxyIdentityCertificateElement())); 271 if (src.hasProxySignature()) 272 tgt.setProxySignature(Signature40_50.convertSignature(src.getProxySignature())); 273 if (src.hasSourceSignature()) 274 tgt.setSourceSignature(Signature40_50.convertSignature(src.getSourceSignature())); 275 return tgt; 276 } 277 278 public static org.hl7.fhir.r5.model.VerificationResult.VerificationResultValidatorComponent convertVerificationResultValidatorComponent(org.hl7.fhir.r4.model.VerificationResult.VerificationResultValidatorComponent src) throws FHIRException { 279 if (src == null) 280 return null; 281 org.hl7.fhir.r5.model.VerificationResult.VerificationResultValidatorComponent tgt = new org.hl7.fhir.r5.model.VerificationResult.VerificationResultValidatorComponent(); 282 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 283 if (src.hasOrganization()) 284 tgt.setOrganization(Reference40_50.convertReference(src.getOrganization())); 285 if (src.hasIdentityCertificate()) 286 tgt.setIdentityCertificateElement(String40_50.convertString(src.getIdentityCertificateElement())); 287 if (src.hasAttestationSignature()) 288 tgt.setAttestationSignature(Signature40_50.convertSignature(src.getAttestationSignature())); 289 return tgt; 290 } 291 292 public static org.hl7.fhir.r4.model.VerificationResult.VerificationResultValidatorComponent convertVerificationResultValidatorComponent(org.hl7.fhir.r5.model.VerificationResult.VerificationResultValidatorComponent src) throws FHIRException { 293 if (src == null) 294 return null; 295 org.hl7.fhir.r4.model.VerificationResult.VerificationResultValidatorComponent tgt = new org.hl7.fhir.r4.model.VerificationResult.VerificationResultValidatorComponent(); 296 ConversionContext40_50.INSTANCE.getVersionConvertor_40_50().copyBackboneElement(src, tgt); 297 if (src.hasOrganization()) 298 tgt.setOrganization(Reference40_50.convertReference(src.getOrganization())); 299 if (src.hasIdentityCertificate()) 300 tgt.setIdentityCertificateElement(String40_50.convertString(src.getIdentityCertificateElement())); 301 if (src.hasAttestationSignature()) 302 tgt.setAttestationSignature(Signature40_50.convertSignature(src.getAttestationSignature())); 303 return tgt; 304 } 305}