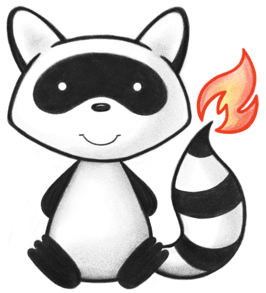
001package org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Code43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010public class Coding43_50 { 011 public static org.hl7.fhir.r5.model.Coding convertCoding(org.hl7.fhir.r4b.model.Coding src) throws FHIRException { 012 if (src == null) return null; 013 org.hl7.fhir.r5.model.Coding tgt = new org.hl7.fhir.r5.model.Coding(); 014 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 015 if (src.hasSystem()) tgt.setSystemElement(Uri43_50.convertUri(src.getSystemElement())); 016 if (src.hasVersion()) tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 017 if (src.hasCode()) tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 018 if (src.hasDisplay()) tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 019 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean43_50.convertBoolean(src.getUserSelectedElement())); 020 return tgt; 021 } 022 023 public static org.hl7.fhir.r4b.model.Coding convertCoding(org.hl7.fhir.r5.model.Coding src) throws FHIRException { 024 if (src == null) return null; 025 org.hl7.fhir.r4b.model.Coding tgt = new org.hl7.fhir.r4b.model.Coding(); 026 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 027 if (src.hasSystem()) tgt.setSystemElement(Uri43_50.convertUri(src.getSystemElement())); 028 if (src.hasVersion()) tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 029 if (src.hasCode()) tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 030 if (src.hasDisplay()) tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 031 if (src.hasUserSelected()) tgt.setUserSelectedElement(Boolean43_50.convertBoolean(src.getUserSelectedElement())); 032 return tgt; 033 } 034 035 036 public static org.hl7.fhir.r5.model.CodeableConcept convertCodingToCodeableConcept(org.hl7.fhir.r4b.model.Coding src) throws FHIRException { 037 if (src == null) return null; 038 org.hl7.fhir.r5.model.CodeableConcept tgt = new org.hl7.fhir.r5.model.CodeableConcept(); 039 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 040 if (src.hasSystem()) tgt.getCodingFirstRep().setSystem(src.getSystem()); 041 if (src.hasVersion()) tgt.getCodingFirstRep().setVersion(src.getVersion()); 042 if (src.hasCode()) tgt.getCodingFirstRep().setCode(src.getCode()); 043 if (src.hasDisplay()) tgt.getCodingFirstRep().setDisplay(src.getDisplay()); 044 if (src.hasUserSelected()) tgt.getCodingFirstRep().setUserSelected(src.getUserSelected()); 045 return tgt; 046 } 047 048 public static org.hl7.fhir.r5.model.Coding convertCoding(org.hl7.fhir.r4b.model.CodeableConcept src) throws FHIRException { 049 if (src == null) return null; 050 org.hl7.fhir.r5.model.Coding tgt = new org.hl7.fhir.r5.model.Coding(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 052 if (src.hasCoding()) { 053 if (src.getCodingFirstRep().hasSystem()) tgt.setSystem(src.getCodingFirstRep().getSystem()); 054 if (src.getCodingFirstRep().hasVersion()) tgt.setVersion(src.getCodingFirstRep().getVersion()); 055 if (src.getCodingFirstRep().hasCode()) tgt.setCode(src.getCodingFirstRep().getCode()); 056 if (src.getCodingFirstRep().hasDisplay()) tgt.setDisplay(src.getCodingFirstRep().getDisplay()); 057 if (src.getCodingFirstRep().hasUserSelected()) tgt.setUserSelected(src.getCodingFirstRep().getUserSelected()); 058 } 059 return tgt; 060 } 061 062 063 public static org.hl7.fhir.r4b.model.Coding convertCoding(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 064 if (src == null) return null; 065 org.hl7.fhir.r4b.model.Coding tgt = new org.hl7.fhir.r4b.model.Coding(); 066 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 067 if (src.hasCoding()) { 068 if (src.getCodingFirstRep().hasSystem()) tgt.setSystem(src.getCodingFirstRep().getSystem()); 069 if (src.getCodingFirstRep().hasVersion()) tgt.setVersion(src.getCodingFirstRep().getVersion()); 070 if (src.getCodingFirstRep().hasCode()) tgt.setCode(src.getCodingFirstRep().getCode()); 071 if (src.getCodingFirstRep().hasDisplay()) tgt.setDisplay(src.getCodingFirstRep().getDisplay()); 072 if (src.getCodingFirstRep().hasUserSelected()) tgt.setUserSelected(src.getCodingFirstRep().getUserSelected()); 073 } 074 return tgt; 075 } 076 077 078}