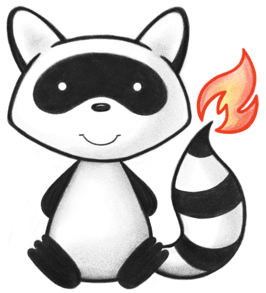
001package org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 007import org.hl7.fhir.exceptions.FHIRException; 008import org.hl7.fhir.r5.model.Identifier; 009 010public class Identifier43_50 { 011 public static org.hl7.fhir.r5.model.Identifier convertIdentifier(org.hl7.fhir.r4b.model.Identifier src) throws FHIRException { 012 if (src == null) return null; 013 org.hl7.fhir.r5.model.Identifier tgt = new org.hl7.fhir.r5.model.Identifier(); 014 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 015 if (src.hasUse()) tgt.setUseElement(convertIdentifierUse(src.getUseElement())); 016 if (src.hasType()) tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 017 if (src.hasSystem()) tgt.setSystemElement(Uri43_50.convertUri(src.getSystemElement())); 018 if (src.hasValue()) tgt.setValueElement(String43_50.convertString(src.getValueElement())); 019 if (src.hasPeriod()) tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 020 if (src.hasAssigner()) tgt.setAssigner(Reference43_50.convertReference(src.getAssigner())); 021 return tgt; 022 } 023 024 public static org.hl7.fhir.r4b.model.Identifier convertIdentifier(org.hl7.fhir.r5.model.Identifier src) throws FHIRException { 025 if (src == null) return null; 026 org.hl7.fhir.r4b.model.Identifier tgt = new org.hl7.fhir.r4b.model.Identifier(); 027 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 028 if (src.hasUse()) tgt.setUseElement(convertIdentifierUse(src.getUseElement())); 029 if (src.hasType()) tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 030 if (src.hasSystem()) tgt.setSystemElement(Uri43_50.convertUri(src.getSystemElement())); 031 if (src.hasValue()) tgt.setValueElement(String43_50.convertString(src.getValueElement())); 032 if (src.hasPeriod()) tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 033 if (src.hasAssigner()) tgt.setAssigner(Reference43_50.convertReference(src.getAssigner())); 034 return tgt; 035 } 036 037 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Identifier.IdentifierUse> convertIdentifierUse(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Identifier.IdentifierUse> src) throws FHIRException { 038 if (src == null || src.isEmpty()) return null; 039 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Identifier.IdentifierUse> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Identifier.IdentifierUseEnumFactory()); 040 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 041 if (src.getValue() == null) { 042 tgt.setValue(null); 043} else { 044 switch(src.getValue()) { 045 case USUAL: 046 tgt.setValue(Identifier.IdentifierUse.USUAL); 047 break; 048 case OFFICIAL: 049 tgt.setValue(Identifier.IdentifierUse.OFFICIAL); 050 break; 051 case TEMP: 052 tgt.setValue(Identifier.IdentifierUse.TEMP); 053 break; 054 case SECONDARY: 055 tgt.setValue(Identifier.IdentifierUse.SECONDARY); 056 break; 057 case OLD: 058 tgt.setValue(Identifier.IdentifierUse.OLD); 059 break; 060 default: 061 tgt.setValue(Identifier.IdentifierUse.NULL); 062 break; 063 } 064} 065 return tgt; 066 } 067 068 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Identifier.IdentifierUse> convertIdentifierUse(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Identifier.IdentifierUse> src) throws FHIRException { 069 if (src == null || src.isEmpty()) return null; 070 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Identifier.IdentifierUse> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Identifier.IdentifierUseEnumFactory()); 071 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 072 if (src.getValue() == null) { 073 tgt.setValue(null); 074} else { 075 switch(src.getValue()) { 076 case USUAL: 077 tgt.setValue(org.hl7.fhir.r4b.model.Identifier.IdentifierUse.USUAL); 078 break; 079 case OFFICIAL: 080 tgt.setValue(org.hl7.fhir.r4b.model.Identifier.IdentifierUse.OFFICIAL); 081 break; 082 case TEMP: 083 tgt.setValue(org.hl7.fhir.r4b.model.Identifier.IdentifierUse.TEMP); 084 break; 085 case SECONDARY: 086 tgt.setValue(org.hl7.fhir.r4b.model.Identifier.IdentifierUse.SECONDARY); 087 break; 088 case OLD: 089 tgt.setValue(org.hl7.fhir.r4b.model.Identifier.IdentifierUse.OLD); 090 break; 091 default: 092 tgt.setValue(org.hl7.fhir.r4b.model.Identifier.IdentifierUse.NULL); 093 break; 094 } 095} 096 return tgt; 097 } 098}