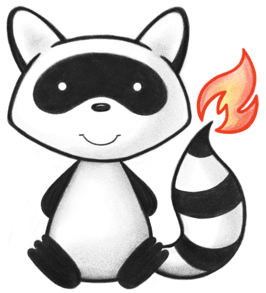
001package org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.BackboneElement43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.BackboneType43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Decimal43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.PositiveInt43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Time43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.UnsignedInt43_50; 013import org.hl7.fhir.exceptions.FHIRException; 014 015public class Timing43_50 { 016 public static org.hl7.fhir.r4b.model.Timing convertTiming(org.hl7.fhir.r5.model.Timing src) throws FHIRException { 017 if (src == null) return null; 018 org.hl7.fhir.r4b.model.Timing tgt = new org.hl7.fhir.r4b.model.Timing(); 019 BackboneType43_50.copyBackboneType(src, tgt); 020 for (org.hl7.fhir.r5.model.DateTimeType t : src.getEvent()) tgt.getEvent().add(DateTime43_50.convertDateTime(t)); 021 if (src.hasRepeat()) tgt.setRepeat(convertTimingRepeatComponent(src.getRepeat())); 022 if (src.hasCode()) tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 023 return tgt; 024 } 025 026 public static org.hl7.fhir.r5.model.Timing convertTiming(org.hl7.fhir.r4b.model.Timing src) throws FHIRException { 027 if (src == null) return null; 028 org.hl7.fhir.r5.model.Timing tgt = new org.hl7.fhir.r5.model.Timing(); 029 BackboneElement43_50.copyBackboneElement(src, tgt); 030 for (org.hl7.fhir.r4b.model.DateTimeType t : src.getEvent()) tgt.getEvent().add(DateTime43_50.convertDateTime(t)); 031 if (src.hasRepeat()) tgt.setRepeat(convertTimingRepeatComponent(src.getRepeat())); 032 if (src.hasCode()) tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 033 return tgt; 034 } 035 036 public static org.hl7.fhir.r5.model.Timing.TimingRepeatComponent convertTimingRepeatComponent(org.hl7.fhir.r4b.model.Timing.TimingRepeatComponent src) throws FHIRException { 037 if (src == null) return null; 038 org.hl7.fhir.r5.model.Timing.TimingRepeatComponent tgt = new org.hl7.fhir.r5.model.Timing.TimingRepeatComponent(); 039 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 040 if (src.hasBounds()) 041 tgt.setBounds(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getBounds())); 042 if (src.hasCount()) tgt.setCountElement(PositiveInt43_50.convertPositiveInt(src.getCountElement())); 043 if (src.hasCountMax()) tgt.setCountMaxElement(PositiveInt43_50.convertPositiveInt(src.getCountMaxElement())); 044 if (src.hasDuration()) tgt.setDurationElement(Decimal43_50.convertDecimal(src.getDurationElement())); 045 if (src.hasDurationMax()) tgt.setDurationMaxElement(Decimal43_50.convertDecimal(src.getDurationMaxElement())); 046 if (src.hasDurationUnit()) tgt.setDurationUnitElement(convertUnitsOfTime(src.getDurationUnitElement())); 047 if (src.hasFrequency()) tgt.setFrequencyElement(PositiveInt43_50.convertPositiveInt(src.getFrequencyElement())); 048 if (src.hasFrequencyMax()) 049 tgt.setFrequencyMaxElement(PositiveInt43_50.convertPositiveInt(src.getFrequencyMaxElement())); 050 if (src.hasPeriod()) tgt.setPeriodElement(Decimal43_50.convertDecimal(src.getPeriodElement())); 051 if (src.hasPeriodMax()) tgt.setPeriodMaxElement(Decimal43_50.convertDecimal(src.getPeriodMaxElement())); 052 if (src.hasPeriodUnit()) tgt.setPeriodUnitElement(convertUnitsOfTime(src.getPeriodUnitElement())); 053 tgt.setDayOfWeek(src.getDayOfWeek().stream().map(Timing43_50::convertDayOfWeek).collect(Collectors.toList())); 054 if (src.hasWhen()) 055 tgt.setWhen(src.getWhen().stream().map(Timing43_50::convertEventTiming).collect(Collectors.toList())); 056 for (org.hl7.fhir.r4b.model.TimeType t : src.getTimeOfDay()) tgt.getTimeOfDay().add(Time43_50.convertTime(t)); 057 if (src.hasOffset()) tgt.setOffsetElement(UnsignedInt43_50.convertUnsignedInt(src.getOffsetElement())); 058 return tgt; 059 } 060 061 public static org.hl7.fhir.r4b.model.Timing.TimingRepeatComponent convertTimingRepeatComponent(org.hl7.fhir.r5.model.Timing.TimingRepeatComponent src) throws FHIRException { 062 if (src == null) return null; 063 org.hl7.fhir.r4b.model.Timing.TimingRepeatComponent tgt = new org.hl7.fhir.r4b.model.Timing.TimingRepeatComponent(); 064 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 065 if (src.hasBounds()) 066 tgt.setBounds(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getBounds())); 067 if (src.hasCount()) tgt.setCountElement(PositiveInt43_50.convertPositiveInt(src.getCountElement())); 068 if (src.hasCountMax()) tgt.setCountMaxElement(PositiveInt43_50.convertPositiveInt(src.getCountMaxElement())); 069 if (src.hasDuration()) tgt.setDurationElement(Decimal43_50.convertDecimal(src.getDurationElement())); 070 if (src.hasDurationMax()) tgt.setDurationMaxElement(Decimal43_50.convertDecimal(src.getDurationMaxElement())); 071 if (src.hasDurationUnit()) tgt.setDurationUnitElement(convertUnitsOfTime(src.getDurationUnitElement())); 072 if (src.hasFrequency()) tgt.setFrequencyElement(PositiveInt43_50.convertPositiveInt(src.getFrequencyElement())); 073 if (src.hasFrequencyMax()) 074 tgt.setFrequencyMaxElement(PositiveInt43_50.convertPositiveInt(src.getFrequencyMaxElement())); 075 if (src.hasPeriod()) tgt.setPeriodElement(Decimal43_50.convertDecimal(src.getPeriodElement())); 076 if (src.hasPeriodMax()) tgt.setPeriodMaxElement(Decimal43_50.convertDecimal(src.getPeriodMaxElement())); 077 if (src.hasPeriodUnit()) tgt.setPeriodUnitElement(convertUnitsOfTime(src.getPeriodUnitElement())); 078 tgt.setDayOfWeek(src.getDayOfWeek().stream().map(Timing43_50::convertDayOfWeek).collect(Collectors.toList())); 079 if (src.hasWhen()) 080 tgt.setWhen(src.getWhen().stream().map(Timing43_50::convertEventTiming).collect(Collectors.toList())); 081 for (org.hl7.fhir.r5.model.TimeType t : src.getTimeOfDay()) tgt.getTimeOfDay().add(Time43_50.convertTime(t)); 082 if (src.hasOffset()) tgt.setOffsetElement(UnsignedInt43_50.convertUnsignedInt(src.getOffsetElement())); 083 return tgt; 084 } 085 086 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Timing.UnitsOfTime> convertUnitsOfTime(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Timing.UnitsOfTime> src) throws FHIRException { 087 if (src == null || src.isEmpty()) return null; 088 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Timing.UnitsOfTime> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Timing.UnitsOfTimeEnumFactory()); 089 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 090 if (src.getValue() == null) { 091 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.NULL); 092 } else { 093 switch (src.getValue()) { 094 case S: 095 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.S); 096 break; 097 case MIN: 098 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.MIN); 099 break; 100 case H: 101 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.H); 102 break; 103 case D: 104 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.D); 105 break; 106 case WK: 107 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.WK); 108 break; 109 case MO: 110 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.MO); 111 break; 112 case A: 113 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.A); 114 break; 115 default: 116 tgt.setValue(org.hl7.fhir.r5.model.Timing.UnitsOfTime.NULL); 117 break; 118 } 119 } 120 return tgt; 121 } 122 123 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Timing.UnitsOfTime> convertUnitsOfTime(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Timing.UnitsOfTime> src) throws FHIRException { 124 if (src == null || src.isEmpty()) return null; 125 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Timing.UnitsOfTime> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Timing.UnitsOfTimeEnumFactory()); 126 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 127 if (src.getValue() == null) { 128 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.NULL); 129 } else { 130 switch (src.getValue()) { 131 case S: 132 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.S); 133 break; 134 case MIN: 135 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.MIN); 136 break; 137 case H: 138 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.H); 139 break; 140 case D: 141 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.D); 142 break; 143 case WK: 144 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.WK); 145 break; 146 case MO: 147 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.MO); 148 break; 149 case A: 150 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.A); 151 break; 152 default: 153 tgt.setValue(org.hl7.fhir.r4b.model.Timing.UnitsOfTime.NULL); 154 break; 155 } 156 } 157 return tgt; 158 } 159 160 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> convertDayOfWeek(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek> src) throws FHIRException { 161 if (src == null || src.isEmpty()) return null; 162 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.DaysOfWeekEnumFactory()); 163 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 164 if (src.getValue() == null) { 165 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.NULL); 166 } else { 167 switch (src.getValue()) { 168 case MON: 169 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.MON); 170 break; 171 case TUE: 172 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.TUE); 173 break; 174 case WED: 175 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.WED); 176 break; 177 case THU: 178 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.THU); 179 break; 180 case FRI: 181 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.FRI); 182 break; 183 case SAT: 184 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.SAT); 185 break; 186 case SUN: 187 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.SUN); 188 break; 189 default: 190 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.DaysOfWeek.NULL); 191 break; 192 } 193 } 194 return tgt; 195 } 196 197 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek> convertDayOfWeek(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.DaysOfWeek> src) throws FHIRException { 198 if (src == null || src.isEmpty()) return null; 199 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.DaysOfWeekEnumFactory()); 200 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 201 if (src.getValue() == null) { 202 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.NULL); 203 } else { 204 switch (src.getValue()) { 205 case MON: 206 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.MON); 207 break; 208 case TUE: 209 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.TUE); 210 break; 211 case WED: 212 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.WED); 213 break; 214 case THU: 215 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.THU); 216 break; 217 case FRI: 218 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.FRI); 219 break; 220 case SAT: 221 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.SAT); 222 break; 223 case SUN: 224 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.SUN); 225 break; 226 default: 227 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DaysOfWeek.NULL); 228 break; 229 } 230 } 231 return tgt; 232 } 233 234 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Timing.EventTiming> convertEventTiming(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Timing.EventTiming> src) throws FHIRException { 235 if (src == null || src.isEmpty()) return null; 236 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Timing.EventTiming> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Timing.EventTimingEnumFactory()); 237 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 238 if (src.getValue() == null) { 239 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.NULL); 240 } else { 241 switch (src.getValue()) { 242 case MORN: 243 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.MORN); 244 break; 245 case MORN_EARLY: 246 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.MORN_EARLY); 247 break; 248 case MORN_LATE: 249 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.MORN_LATE); 250 break; 251 case NOON: 252 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.NOON); 253 break; 254 case AFT: 255 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.AFT); 256 break; 257 case AFT_EARLY: 258 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.AFT_EARLY); 259 break; 260 case AFT_LATE: 261 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.AFT_LATE); 262 break; 263 case EVE: 264 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.EVE); 265 break; 266 case EVE_EARLY: 267 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.EVE_EARLY); 268 break; 269 case EVE_LATE: 270 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.EVE_LATE); 271 break; 272 case NIGHT: 273 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.NIGHT); 274 break; 275 case PHS: 276 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.PHS); 277 break; 278 case HS: 279 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.HS); 280 break; 281 case WAKE: 282 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.WAKE); 283 break; 284 case C: 285 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.C); 286 break; 287 case CM: 288 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.CM); 289 break; 290 case CD: 291 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.CD); 292 break; 293 case CV: 294 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.CV); 295 break; 296 case AC: 297 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.AC); 298 break; 299 case ACM: 300 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.ACM); 301 break; 302 case ACD: 303 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.ACD); 304 break; 305 case ACV: 306 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.ACV); 307 break; 308 case PC: 309 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.PC); 310 break; 311 case PCM: 312 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.PCM); 313 break; 314 case PCD: 315 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.PCD); 316 break; 317 case PCV: 318 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.PCV); 319 break; 320 default: 321 tgt.setValue(org.hl7.fhir.r5.model.Timing.EventTiming.NULL); 322 break; 323 } 324 } 325 return tgt; 326 } 327 328 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Timing.EventTiming> convertEventTiming(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Timing.EventTiming> src) throws FHIRException { 329 if (src == null || src.isEmpty()) return null; 330 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Timing.EventTiming> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Timing.EventTimingEnumFactory()); 331 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 332 if (src.getValue() == null) { 333 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.NULL); 334 } else { 335 switch (src.getValue()) { 336 case MORN: 337 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.MORN); 338 break; 339 case MORN_EARLY: 340 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.MORN_EARLY); 341 break; 342 case MORN_LATE: 343 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.MORN_LATE); 344 break; 345 case NOON: 346 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.NOON); 347 break; 348 case AFT: 349 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.AFT); 350 break; 351 case AFT_EARLY: 352 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.AFT_EARLY); 353 break; 354 case AFT_LATE: 355 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.AFT_LATE); 356 break; 357 case EVE: 358 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.EVE); 359 break; 360 case EVE_EARLY: 361 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.EVE_EARLY); 362 break; 363 case EVE_LATE: 364 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.EVE_LATE); 365 break; 366 case NIGHT: 367 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.NIGHT); 368 break; 369 case PHS: 370 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.PHS); 371 break; 372 case HS: 373 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.HS); 374 break; 375 case WAKE: 376 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.WAKE); 377 break; 378 case C: 379 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.C); 380 break; 381 case CM: 382 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.CM); 383 break; 384 case CD: 385 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.CD); 386 break; 387 case CV: 388 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.CV); 389 break; 390 case AC: 391 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.AC); 392 break; 393 case ACM: 394 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.ACM); 395 break; 396 case ACD: 397 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.ACD); 398 break; 399 case ACV: 400 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.ACV); 401 break; 402 case PC: 403 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.PC); 404 break; 405 case PCM: 406 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.PCM); 407 break; 408 case PCD: 409 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.PCD); 410 break; 411 case PCV: 412 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.PCV); 413 break; 414 default: 415 tgt.setValue(org.hl7.fhir.r4b.model.Timing.EventTiming.NULL); 416 break; 417 } 418 } 419 return tgt; 420 } 421}