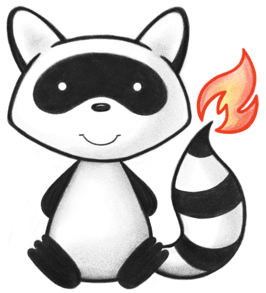
001package org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.Utilities43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Coding43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.PositiveInt43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 009import org.hl7.fhir.exceptions.FHIRException; 010 011public class DataRequirement43_50 { 012 public static org.hl7.fhir.r5.model.DataRequirement convertDataRequirement(org.hl7.fhir.r4b.model.DataRequirement src) throws FHIRException { 013 if (src == null) return null; 014 org.hl7.fhir.r5.model.DataRequirement tgt = new org.hl7.fhir.r5.model.DataRequirement(); 015 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 016 if (src.hasType()) { 017 Utilities43_50.convertType(src.getTypeElement(), tgt.getTypeElement()); 018 } 019 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getProfile()) 020 tgt.getProfile().add(Canonical43_50.convertCanonical(t)); 021 if (src.hasSubject()) 022 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 023 for (org.hl7.fhir.r4b.model.StringType t : src.getMustSupport()) 024 tgt.getMustSupport().add(String43_50.convertString(t)); 025 for (org.hl7.fhir.r4b.model.DataRequirement.DataRequirementCodeFilterComponent t : src.getCodeFilter()) 026 tgt.addCodeFilter(convertDataRequirementCodeFilterComponent(t)); 027 for (org.hl7.fhir.r4b.model.DataRequirement.DataRequirementDateFilterComponent t : src.getDateFilter()) 028 tgt.addDateFilter(convertDataRequirementDateFilterComponent(t)); 029 if (src.hasLimit()) tgt.setLimitElement(PositiveInt43_50.convertPositiveInt(src.getLimitElement())); 030 for (org.hl7.fhir.r4b.model.DataRequirement.DataRequirementSortComponent t : src.getSort()) 031 tgt.addSort(convertDataRequirementSortComponent(t)); 032 return tgt; 033 } 034 035 public static org.hl7.fhir.r4b.model.DataRequirement convertDataRequirement(org.hl7.fhir.r5.model.DataRequirement src) throws FHIRException { 036 if (src == null) return null; 037 org.hl7.fhir.r4b.model.DataRequirement tgt = new org.hl7.fhir.r4b.model.DataRequirement(); 038 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 039 if (src.hasType()) { 040 Utilities43_50.convertType(src.getTypeElement(), tgt.getTypeElement()); 041 } 042 for (org.hl7.fhir.r5.model.CanonicalType t : src.getProfile()) 043 tgt.getProfile().add(Canonical43_50.convertCanonical(t)); 044 if (src.hasSubject()) 045 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 046 for (org.hl7.fhir.r5.model.StringType t : src.getMustSupport()) 047 tgt.getMustSupport().add(String43_50.convertString(t)); 048 for (org.hl7.fhir.r5.model.DataRequirement.DataRequirementCodeFilterComponent t : src.getCodeFilter()) 049 tgt.addCodeFilter(convertDataRequirementCodeFilterComponent(t)); 050 for (org.hl7.fhir.r5.model.DataRequirement.DataRequirementDateFilterComponent t : src.getDateFilter()) 051 tgt.addDateFilter(convertDataRequirementDateFilterComponent(t)); 052 if (src.hasLimit()) tgt.setLimitElement(PositiveInt43_50.convertPositiveInt(src.getLimitElement())); 053 for (org.hl7.fhir.r5.model.DataRequirement.DataRequirementSortComponent t : src.getSort()) 054 tgt.addSort(convertDataRequirementSortComponent(t)); 055 return tgt; 056 } 057 058 public static org.hl7.fhir.r5.model.DataRequirement.DataRequirementCodeFilterComponent convertDataRequirementCodeFilterComponent(org.hl7.fhir.r4b.model.DataRequirement.DataRequirementCodeFilterComponent src) throws FHIRException { 059 if (src == null) return null; 060 org.hl7.fhir.r5.model.DataRequirement.DataRequirementCodeFilterComponent tgt = new org.hl7.fhir.r5.model.DataRequirement.DataRequirementCodeFilterComponent(); 061 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 062 if (src.hasPath()) tgt.setPathElement(String43_50.convertString(src.getPathElement())); 063 if (src.hasSearchParam()) tgt.setSearchParamElement(String43_50.convertString(src.getSearchParamElement())); 064 if (src.hasValueSet()) tgt.setValueSetElement(Canonical43_50.convertCanonical(src.getValueSetElement())); 065 for (org.hl7.fhir.r4b.model.Coding t : src.getCode()) tgt.addCode(Coding43_50.convertCoding(t)); 066 return tgt; 067 } 068 069 public static org.hl7.fhir.r4b.model.DataRequirement.DataRequirementCodeFilterComponent convertDataRequirementCodeFilterComponent(org.hl7.fhir.r5.model.DataRequirement.DataRequirementCodeFilterComponent src) throws FHIRException { 070 if (src == null) return null; 071 org.hl7.fhir.r4b.model.DataRequirement.DataRequirementCodeFilterComponent tgt = new org.hl7.fhir.r4b.model.DataRequirement.DataRequirementCodeFilterComponent(); 072 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 073 if (src.hasPath()) tgt.setPathElement(String43_50.convertString(src.getPathElement())); 074 if (src.hasSearchParam()) tgt.setSearchParamElement(String43_50.convertString(src.getSearchParamElement())); 075 if (src.hasValueSet()) tgt.setValueSetElement(Canonical43_50.convertCanonical(src.getValueSetElement())); 076 for (org.hl7.fhir.r5.model.Coding t : src.getCode()) tgt.addCode(Coding43_50.convertCoding(t)); 077 return tgt; 078 } 079 080 public static org.hl7.fhir.r5.model.DataRequirement.DataRequirementDateFilterComponent convertDataRequirementDateFilterComponent(org.hl7.fhir.r4b.model.DataRequirement.DataRequirementDateFilterComponent src) throws FHIRException { 081 if (src == null) return null; 082 org.hl7.fhir.r5.model.DataRequirement.DataRequirementDateFilterComponent tgt = new org.hl7.fhir.r5.model.DataRequirement.DataRequirementDateFilterComponent(); 083 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 084 if (src.hasPath()) tgt.setPathElement(String43_50.convertString(src.getPathElement())); 085 if (src.hasSearchParam()) tgt.setSearchParamElement(String43_50.convertString(src.getSearchParamElement())); 086 if (src.hasValue()) 087 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 088 return tgt; 089 } 090 091 public static org.hl7.fhir.r4b.model.DataRequirement.DataRequirementDateFilterComponent convertDataRequirementDateFilterComponent(org.hl7.fhir.r5.model.DataRequirement.DataRequirementDateFilterComponent src) throws FHIRException { 092 if (src == null) return null; 093 org.hl7.fhir.r4b.model.DataRequirement.DataRequirementDateFilterComponent tgt = new org.hl7.fhir.r4b.model.DataRequirement.DataRequirementDateFilterComponent(); 094 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 095 if (src.hasPath()) tgt.setPathElement(String43_50.convertString(src.getPathElement())); 096 if (src.hasSearchParam()) tgt.setSearchParamElement(String43_50.convertString(src.getSearchParamElement())); 097 if (src.hasValue()) 098 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 099 return tgt; 100 } 101 102 public static org.hl7.fhir.r5.model.DataRequirement.DataRequirementSortComponent convertDataRequirementSortComponent(org.hl7.fhir.r4b.model.DataRequirement.DataRequirementSortComponent src) throws FHIRException { 103 if (src == null) return null; 104 org.hl7.fhir.r5.model.DataRequirement.DataRequirementSortComponent tgt = new org.hl7.fhir.r5.model.DataRequirement.DataRequirementSortComponent(); 105 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 106 if (src.hasPath()) tgt.setPathElement(String43_50.convertString(src.getPathElement())); 107 if (src.hasDirection()) tgt.setDirectionElement(convertSortDirection(src.getDirectionElement())); 108 return tgt; 109 } 110 111 public static org.hl7.fhir.r4b.model.DataRequirement.DataRequirementSortComponent convertDataRequirementSortComponent(org.hl7.fhir.r5.model.DataRequirement.DataRequirementSortComponent src) throws FHIRException { 112 if (src == null) return null; 113 org.hl7.fhir.r4b.model.DataRequirement.DataRequirementSortComponent tgt = new org.hl7.fhir.r4b.model.DataRequirement.DataRequirementSortComponent(); 114 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 115 if (src.hasPath()) tgt.setPathElement(String43_50.convertString(src.getPathElement())); 116 if (src.hasDirection()) tgt.setDirectionElement(convertSortDirection(src.getDirectionElement())); 117 return tgt; 118 } 119 120 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DataRequirement.SortDirection> convertSortDirection(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.DataRequirement.SortDirection> src) throws FHIRException { 121 if (src == null || src.isEmpty()) return null; 122 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DataRequirement.SortDirection> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.DataRequirement.SortDirectionEnumFactory()); 123 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 124 if (src.getValue() == null) { 125 tgt.setValue(org.hl7.fhir.r5.model.DataRequirement.SortDirection.NULL); 126 } else { 127 switch (src.getValue()) { 128 case ASCENDING: 129 tgt.setValue(org.hl7.fhir.r5.model.DataRequirement.SortDirection.ASCENDING); 130 break; 131 case DESCENDING: 132 tgt.setValue(org.hl7.fhir.r5.model.DataRequirement.SortDirection.DESCENDING); 133 break; 134 default: 135 tgt.setValue(org.hl7.fhir.r5.model.DataRequirement.SortDirection.NULL); 136 break; 137 } 138 } 139 return tgt; 140 } 141 142 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.DataRequirement.SortDirection> convertSortDirection(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DataRequirement.SortDirection> src) throws FHIRException { 143 if (src == null || src.isEmpty()) return null; 144 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.DataRequirement.SortDirection> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.DataRequirement.SortDirectionEnumFactory()); 145 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 146 if (src.getValue() == null) { 147 tgt.setValue(org.hl7.fhir.r4b.model.DataRequirement.SortDirection.NULL); 148 } else { 149 switch (src.getValue()) { 150 case ASCENDING: 151 tgt.setValue(org.hl7.fhir.r4b.model.DataRequirement.SortDirection.ASCENDING); 152 break; 153 case DESCENDING: 154 tgt.setValue(org.hl7.fhir.r4b.model.DataRequirement.SortDirection.DESCENDING); 155 break; 156 default: 157 tgt.setValue(org.hl7.fhir.r4b.model.DataRequirement.SortDirection.NULL); 158 break; 159 } 160 } 161 return tgt; 162 } 163}