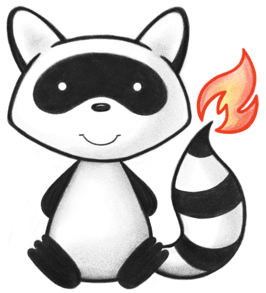
001package org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Attachment43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Url43_50; 009import org.hl7.fhir.exceptions.FHIRException; 010 011public class RelatedArtifact43_50 { 012 public static org.hl7.fhir.r5.model.RelatedArtifact convertRelatedArtifact(org.hl7.fhir.r4b.model.RelatedArtifact src) throws FHIRException { 013 if (src == null) return null; 014 org.hl7.fhir.r5.model.RelatedArtifact tgt = new org.hl7.fhir.r5.model.RelatedArtifact(); 015 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 016 if (src.hasType()) tgt.setTypeElement(convertRelatedArtifactType(src.getTypeElement())); 017 if (src.hasLabel()) tgt.setLabelElement(String43_50.convertString(src.getLabelElement())); 018 if (src.hasDisplay()) tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 019 if (src.hasCitation()) tgt.setCitationElement(MarkDown43_50.convertMarkdown(src.getCitationElement())); 020 if (src.hasUrl()) tgt.getDocument().setUrlElement(Url43_50.convertUrl(src.getUrlElement())); 021 if (src.hasDocument()) tgt.setDocument(Attachment43_50.convertAttachment(src.getDocument())); 022 if (src.hasResource()) tgt.setResourceElement(Canonical43_50.convertCanonical(src.getResourceElement())); 023 return tgt; 024 } 025 026 public static org.hl7.fhir.r4b.model.RelatedArtifact convertRelatedArtifact(org.hl7.fhir.r5.model.RelatedArtifact src) throws FHIRException { 027 if (src == null) return null; 028 org.hl7.fhir.r4b.model.RelatedArtifact tgt = new org.hl7.fhir.r4b.model.RelatedArtifact(); 029 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 030 if (src.hasType()) tgt.setTypeElement(convertRelatedArtifactType(src.getTypeElement())); 031 if (src.hasLabel()) tgt.setLabelElement(String43_50.convertString(src.getLabelElement())); 032 if (src.hasDisplay()) tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 033 if (src.hasCitation()) tgt.setCitationElement(MarkDown43_50.convertMarkdown(src.getCitationElement())); 034 if (src.getDocument().hasUrl()) tgt.setUrlElement(Url43_50.convertUrl(src.getDocument().getUrlElement())); 035 if (src.hasDocument()) tgt.setDocument(Attachment43_50.convertAttachment(src.getDocument())); 036 if (src.hasResource()) tgt.setResourceElement(Canonical43_50.convertCanonical(src.getResourceElement())); 037 return tgt; 038 } 039 040 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> convertRelatedArtifactType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType> src) throws FHIRException { 041 if (src == null || src.isEmpty()) return null; 042 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactTypeEnumFactory()); 043 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 044 if (src.getValue() == null) { 045 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.NULL); 046 } else { 047 switch (src.getValue()) { 048 case DOCUMENTATION: 049 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.DOCUMENTATION); 050 break; 051 case JUSTIFICATION: 052 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.JUSTIFICATION); 053 break; 054 case CITATION: 055 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.CITATION); 056 break; 057 case PREDECESSOR: 058 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.PREDECESSOR); 059 break; 060 case SUCCESSOR: 061 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.SUCCESSOR); 062 break; 063 case DERIVEDFROM: 064 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.DERIVEDFROM); 065 break; 066 case DEPENDSON: 067 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.DEPENDSON); 068 break; 069 case COMPOSEDOF: 070 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.COMPOSEDOF); 071 break; 072 default: 073 tgt.setValue(org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType.NULL); 074 break; 075 } 076 } 077 return tgt; 078 } 079 080 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType> convertRelatedArtifactType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> src) throws FHIRException { 081 if (src == null || src.isEmpty()) return null; 082 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactTypeEnumFactory()); 083 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 084 if (src.getValue() == null) { 085 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.NULL); 086 } else { 087 switch (src.getValue()) { 088 case DOCUMENTATION: 089 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.DOCUMENTATION); 090 break; 091 case JUSTIFICATION: 092 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.JUSTIFICATION); 093 break; 094 case CITATION: 095 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.CITATION); 096 break; 097 case PREDECESSOR: 098 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.PREDECESSOR); 099 break; 100 case SUCCESSOR: 101 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.SUCCESSOR); 102 break; 103 case DERIVEDFROM: 104 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.DERIVEDFROM); 105 break; 106 case DEPENDSON: 107 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.DEPENDSON); 108 break; 109 case COMPOSEDOF: 110 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.COMPOSEDOF); 111 break; 112 default: 113 tgt.setValue(org.hl7.fhir.r4b.model.RelatedArtifact.RelatedArtifactType.NULL); 114 break; 115 } 116 } 117 return tgt; 118 } 119}