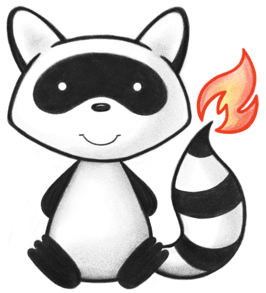
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.VersionConvertorConstants; 004import org.hl7.fhir.convertors.context.ConversionContext43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.SimpleQuantity43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.Expression43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.RelatedArtifact43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 015import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 016import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 017import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 018import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 019import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 020import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Dosage43_50; 021import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 022import org.hl7.fhir.exceptions.FHIRException; 023import org.hl7.fhir.r5.model.*; 024 025/* 026 Copyright (c) 2011+, HL7, Inc. 027 All rights reserved. 028 029 Redistribution and use in source and binary forms, with or without modification, 030 are permitted provided that the following conditions are met: 031 032 * Redistributions of source code must retain the above copyright notice, this 033 list of conditions and the following disclaimer. 034 * Redistributions in binary form must reproduce the above copyright notice, 035 this list of conditions and the following disclaimer in the documentation 036 and/or other materials provided with the distribution. 037 * Neither the name of HL7 nor the names of its contributors may be used to 038 endorse or promote products derived from this software without specific 039 prior written permission. 040 041 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 042 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 043 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 044 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 045 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 046 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 047 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 048 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 049 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 050 POSSIBILITY OF SUCH DAMAGE. 051 052*/ 053// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 054public class ActivityDefinition43_50 { 055 056 public static org.hl7.fhir.r5.model.ActivityDefinition convertActivityDefinition(org.hl7.fhir.r4b.model.ActivityDefinition src) throws FHIRException { 057 if (src == null) 058 return null; 059 org.hl7.fhir.r5.model.ActivityDefinition tgt = new org.hl7.fhir.r5.model.ActivityDefinition(); 060 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 061 if (src.hasUrl()) 062 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 063 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 064 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 065 if (src.hasVersion()) 066 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 067 if (src.hasName()) 068 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 069 if (src.hasTitle()) 070 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 071 if (src.hasSubtitle()) 072 tgt.setSubtitleElement(String43_50.convertString(src.getSubtitleElement())); 073 if (src.hasStatus()) 074 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 075 if (src.hasExperimental()) 076 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 077 if (src.hasSubject()) 078 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 079 if (src.hasDate()) 080 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 081 if (src.hasPublisher()) 082 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 083 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 084 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 085 if (src.hasDescription()) 086 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 087 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 088 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 089 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 090 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 091 if (src.hasPurpose()) 092 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 093 if (src.hasUsage()) 094 tgt.setUsageElement(String43_50.convertStringToMarkdown(src.getUsageElement())); 095 if (src.hasCopyright()) 096 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 097 if (src.hasApprovalDate()) 098 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 099 if (src.hasLastReviewDate()) 100 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 101 if (src.hasEffectivePeriod()) 102 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 103 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getTopic()) 104 tgt.addTopic(CodeableConcept43_50.convertCodeableConcept(t)); 105 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getAuthor()) 106 tgt.addAuthor(ContactDetail43_50.convertContactDetail(t)); 107 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getEditor()) 108 tgt.addEditor(ContactDetail43_50.convertContactDetail(t)); 109 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getReviewer()) 110 tgt.addReviewer(ContactDetail43_50.convertContactDetail(t)); 111 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getEndorser()) 112 tgt.addEndorser(ContactDetail43_50.convertContactDetail(t)); 113 for (org.hl7.fhir.r4b.model.RelatedArtifact t : src.getRelatedArtifact()) 114 tgt.addRelatedArtifact(RelatedArtifact43_50.convertRelatedArtifact(t)); 115 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getLibrary()) 116 tgt.getLibrary().add(Canonical43_50.convertCanonical(t)); 117 if (src.hasKind()) 118 tgt.setKindElement(convertActivityDefinitionKind(src.getKindElement())); 119 if (src.hasProfile()) 120 tgt.setProfileElement(Canonical43_50.convertCanonical(src.getProfileElement())); 121 if (src.hasCode()) 122 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 123 if (src.hasIntent()) 124 tgt.setIntentElement(convertRequestIntent(src.getIntentElement())); 125 if (src.hasPriority()) 126 tgt.setPriorityElement(convertRequestPriority(src.getPriorityElement())); 127 if (src.hasDoNotPerform()) 128 tgt.setDoNotPerformElement(Boolean43_50.convertBoolean(src.getDoNotPerformElement())); 129 if (src.hasTiming()) 130 tgt.setTiming(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getTiming())); 131 if (src.hasLocation()) 132 tgt.setLocation(new CodeableReference(Reference43_50.convertReference(src.getLocation()))); 133 for (org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionParticipantComponent t : src.getParticipant()) 134 tgt.addParticipant(convertActivityDefinitionParticipantComponent(t)); 135 if (src.hasProduct()) 136 tgt.setProduct(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getProduct())); 137 if (src.hasQuantity()) 138 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 139 for (org.hl7.fhir.r4b.model.Dosage t : src.getDosage()) tgt.addDosage(Dosage43_50.convertDosage(t)); 140 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getBodySite()) 141 tgt.addBodySite(CodeableConcept43_50.convertCodeableConcept(t)); 142 for (org.hl7.fhir.r4b.model.Reference t : src.getSpecimenRequirement()) 143 tgt.getSpecimenRequirement().add(Reference43_50.convertReferenceToCanonical(t)); 144 for (org.hl7.fhir.r4b.model.Reference t : src.getObservationRequirement()) 145 tgt.getObservationRequirement().add(Reference43_50.convertReferenceToCanonical(t)); 146 for (org.hl7.fhir.r4b.model.Reference t : src.getObservationResultRequirement()) 147 tgt.getObservationResultRequirement().add(Reference43_50.convertReferenceToCanonical(t)); 148 if (src.hasTransform()) 149 tgt.setTransformElement(Canonical43_50.convertCanonical(src.getTransformElement())); 150 for (org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent t : src.getDynamicValue()) 151 tgt.addDynamicValue(convertActivityDefinitionDynamicValueComponent(t)); 152 return tgt; 153 } 154 155 public static org.hl7.fhir.r4b.model.ActivityDefinition convertActivityDefinition(org.hl7.fhir.r5.model.ActivityDefinition src) throws FHIRException { 156 if (src == null) 157 return null; 158 org.hl7.fhir.r4b.model.ActivityDefinition tgt = new org.hl7.fhir.r4b.model.ActivityDefinition(); 159 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 160 if (src.hasUrl()) 161 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 162 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 163 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 164 if (src.hasVersion()) 165 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 166 if (src.hasName()) 167 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 168 if (src.hasTitle()) 169 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 170 if (src.hasSubtitle()) 171 tgt.setSubtitleElement(String43_50.convertString(src.getSubtitleElement())); 172 if (src.hasStatus()) 173 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 174 if (src.hasExperimental()) 175 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 176 if (src.hasSubject()) 177 tgt.setSubject(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getSubject())); 178 if (src.hasDate()) 179 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 180 if (src.hasPublisher()) 181 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 182 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 183 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 184 if (src.hasDescription()) 185 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 186 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 187 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 188 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 189 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 190 if (src.hasPurpose()) 191 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 192 if (src.hasUsage()) 193 tgt.setUsageElement(String43_50.convertString(src.getUsageElement())); 194 if (src.hasCopyright()) 195 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 196 if (src.hasApprovalDate()) 197 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 198 if (src.hasLastReviewDate()) 199 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 200 if (src.hasEffectivePeriod()) 201 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 202 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getTopic()) 203 tgt.addTopic(CodeableConcept43_50.convertCodeableConcept(t)); 204 for (org.hl7.fhir.r5.model.ContactDetail t : src.getAuthor()) 205 tgt.addAuthor(ContactDetail43_50.convertContactDetail(t)); 206 for (org.hl7.fhir.r5.model.ContactDetail t : src.getEditor()) 207 tgt.addEditor(ContactDetail43_50.convertContactDetail(t)); 208 for (org.hl7.fhir.r5.model.ContactDetail t : src.getReviewer()) 209 tgt.addReviewer(ContactDetail43_50.convertContactDetail(t)); 210 for (org.hl7.fhir.r5.model.ContactDetail t : src.getEndorser()) 211 tgt.addEndorser(ContactDetail43_50.convertContactDetail(t)); 212 for (org.hl7.fhir.r5.model.RelatedArtifact t : src.getRelatedArtifact()) 213 tgt.addRelatedArtifact(RelatedArtifact43_50.convertRelatedArtifact(t)); 214 for (org.hl7.fhir.r5.model.CanonicalType t : src.getLibrary()) 215 tgt.getLibrary().add(Canonical43_50.convertCanonical(t)); 216 if (src.hasKind()) 217 tgt.setKindElement(convertActivityDefinitionKind(src.getKindElement())); 218 if (src.hasProfile()) 219 tgt.setProfileElement(Canonical43_50.convertCanonical(src.getProfileElement())); 220 if (src.hasCode()) 221 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 222 if (src.hasIntent()) 223 tgt.setIntentElement(convertRequestIntent(src.getIntentElement())); 224 if (src.hasPriority()) 225 tgt.setPriorityElement(convertRequestPriority(src.getPriorityElement())); 226 if (src.hasDoNotPerform()) 227 tgt.setDoNotPerformElement(Boolean43_50.convertBoolean(src.getDoNotPerformElement())); 228 if (src.hasTiming()) 229 tgt.setTiming(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getTiming())); 230 if (src.getLocation().hasReference()) 231 tgt.setLocation(Reference43_50.convertReference(src.getLocation().getReference())); 232 for (org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionParticipantComponent t : src.getParticipant()) 233 tgt.addParticipant(convertActivityDefinitionParticipantComponent(t)); 234 if (src.hasProduct()) 235 tgt.setProduct(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getProduct())); 236 if (src.hasQuantity()) 237 tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 238 for (org.hl7.fhir.r5.model.Dosage t : src.getDosage()) tgt.addDosage(Dosage43_50.convertDosage(t)); 239 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getBodySite()) 240 tgt.addBodySite(CodeableConcept43_50.convertCodeableConcept(t)); 241 for (org.hl7.fhir.r5.model.CanonicalType t : src.getSpecimenRequirement()) 242 tgt.addSpecimenRequirement(Reference43_50.convertReferenceToCanonical(t)); 243 for (org.hl7.fhir.r5.model.CanonicalType t : src.getObservationRequirement()) 244 tgt.addObservationRequirement(Reference43_50.convertReferenceToCanonical(t)); 245 for (org.hl7.fhir.r5.model.CanonicalType t : src.getObservationResultRequirement()) 246 tgt.addObservationResultRequirement(Reference43_50.convertReferenceToCanonical(t)); 247 if (src.hasTransform()) 248 tgt.setTransformElement(Canonical43_50.convertCanonical(src.getTransformElement())); 249 for (org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent t : src.getDynamicValue()) 250 tgt.addDynamicValue(convertActivityDefinitionDynamicValueComponent(t)); 251 return tgt; 252 } 253 254 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ActivityDefinition.RequestResourceTypes> convertActivityDefinitionKind(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType> src) throws FHIRException { 255 if (src == null || src.isEmpty()) 256 return null; 257 Enumeration<ActivityDefinition.RequestResourceTypes> tgt = new Enumeration<>(new ActivityDefinition.RequestResourceTypesEnumFactory()); 258 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 259 if (src.getValue() == null) { 260 tgt.setValue(null); 261 } else { 262 switch (src.getValue()) { 263 case APPOINTMENT: 264 tgt.setValue(ActivityDefinition.RequestResourceTypes.APPOINTMENT); 265 break; 266 case APPOINTMENTRESPONSE: 267 tgt.setValue(ActivityDefinition.RequestResourceTypes.APPOINTMENTRESPONSE); 268 break; 269 case CAREPLAN: 270 tgt.setValue(ActivityDefinition.RequestResourceTypes.CAREPLAN); 271 break; 272 case CLAIM: 273 tgt.setValue(ActivityDefinition.RequestResourceTypes.CLAIM); 274 break; 275 case COMMUNICATIONREQUEST: 276 tgt.setValue(ActivityDefinition.RequestResourceTypes.COMMUNICATIONREQUEST); 277 break; 278 case DEVICEREQUEST: 279 tgt.setValue(ActivityDefinition.RequestResourceTypes.DEVICEREQUEST); 280 break; 281 case ENROLLMENTREQUEST: 282 tgt.setValue(ActivityDefinition.RequestResourceTypes.ENROLLMENTREQUEST); 283 break; 284 case IMMUNIZATIONRECOMMENDATION: 285 tgt.setValue(ActivityDefinition.RequestResourceTypes.IMMUNIZATIONRECOMMENDATION); 286 break; 287 case MEDICATIONREQUEST: 288 tgt.setValue(ActivityDefinition.RequestResourceTypes.MEDICATIONREQUEST); 289 break; 290 case NUTRITIONORDER: 291 tgt.setValue(ActivityDefinition.RequestResourceTypes.NUTRITIONORDER); 292 break; 293 case SERVICEREQUEST: 294 tgt.setValue(ActivityDefinition.RequestResourceTypes.SERVICEREQUEST); 295 break; 296 case SUPPLYREQUEST: 297 tgt.setValue(ActivityDefinition.RequestResourceTypes.SUPPLYREQUEST); 298 break; 299 case TASK: 300 tgt.setValue(null); 301 tgt.addExtension(VersionConvertorConstants.EXT_ACTUAL_RESOURCE_NAME, new CodeType("Task")); 302 break; 303 case VISIONPRESCRIPTION: 304 tgt.setValue(ActivityDefinition.RequestResourceTypes.VISIONPRESCRIPTION); 305 break; 306 default: 307 tgt.setValue(ActivityDefinition.RequestResourceTypes.NULL); 308 break; 309 } 310 } 311 return tgt; 312 } 313 314 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType> convertActivityDefinitionKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.ActivityDefinition.RequestResourceTypes> src) throws FHIRException { 315 if (src == null || src.isEmpty()) 316 return null; 317 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceTypeEnumFactory()); 318 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt, VersionConvertorConstants.EXT_ACTUAL_RESOURCE_NAME); 319 if (src.hasExtension(VersionConvertorConstants.EXT_ACTUAL_RESOURCE_NAME)) { 320 tgt.setValueAsString(src.getExtensionString(VersionConvertorConstants.EXT_ACTUAL_RESOURCE_NAME)); 321 } else { 322 if (src.getValue() == null) { 323 tgt.setValue(null); 324 } else { 325 switch (src.getValue()) { 326 case APPOINTMENT: 327 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.APPOINTMENT); 328 break; 329 case APPOINTMENTRESPONSE: 330 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.APPOINTMENTRESPONSE); 331 break; 332 case CAREPLAN: 333 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.CAREPLAN); 334 break; 335 case CLAIM: 336 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.CLAIM); 337 break; 338 case COMMUNICATIONREQUEST: 339 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.COMMUNICATIONREQUEST); 340 break; 341 case DEVICEREQUEST: 342 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.DEVICEREQUEST); 343 break; 344 case ENROLLMENTREQUEST: 345 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.ENROLLMENTREQUEST); 346 break; 347 case IMMUNIZATIONRECOMMENDATION: 348 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.IMMUNIZATIONRECOMMENDATION); 349 break; 350 case MEDICATIONREQUEST: 351 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.MEDICATIONREQUEST); 352 break; 353 case NUTRITIONORDER: 354 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.NUTRITIONORDER); 355 break; 356 case SERVICEREQUEST: 357 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.SERVICEREQUEST); 358 break; 359 case SUPPLYREQUEST: 360 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.SUPPLYREQUEST); 361 break; 362 case VISIONPRESCRIPTION: 363 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.VISIONPRESCRIPTION); 364 break; 365 default: 366 tgt.setValue(org.hl7.fhir.r4b.model.ActivityDefinition.RequestResourceType.NULL); 367 break; 368 } 369 } 370 } 371 return tgt; 372 } 373 374 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestIntent> convertRequestIntent(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestIntent> src) throws FHIRException { 375 if (src == null || src.isEmpty()) 376 return null; 377 Enumeration<Enumerations.RequestIntent> tgt = new Enumeration<>(new Enumerations.RequestIntentEnumFactory()); 378 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 379 if (src.getValue() == null) { 380 tgt.setValue(null); 381 } else { 382 switch (src.getValue()) { 383 case PROPOSAL: 384 tgt.setValue(Enumerations.RequestIntent.PROPOSAL); 385 break; 386 case PLAN: 387 tgt.setValue(Enumerations.RequestIntent.PLAN); 388 break; 389 case DIRECTIVE: 390 tgt.setValue(Enumerations.RequestIntent.DIRECTIVE); 391 break; 392 case ORDER: 393 tgt.setValue(Enumerations.RequestIntent.ORDER); 394 break; 395 case ORIGINALORDER: 396 tgt.setValue(Enumerations.RequestIntent.ORIGINALORDER); 397 break; 398 case REFLEXORDER: 399 tgt.setValue(Enumerations.RequestIntent.REFLEXORDER); 400 break; 401 case FILLERORDER: 402 tgt.setValue(Enumerations.RequestIntent.FILLERORDER); 403 break; 404 case INSTANCEORDER: 405 tgt.setValue(Enumerations.RequestIntent.INSTANCEORDER); 406 break; 407 case OPTION: 408 tgt.setValue(Enumerations.RequestIntent.OPTION); 409 break; 410 default: 411 tgt.setValue(Enumerations.RequestIntent.NULL); 412 break; 413 } 414 } 415 return tgt; 416 } 417 418 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestIntent> convertRequestIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestIntent> src) throws FHIRException { 419 if (src == null || src.isEmpty()) 420 return null; 421 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestIntent> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestIntentEnumFactory()); 422 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 423 if (src.getValue() == null) { 424 tgt.setValue(null); 425 } else { 426 switch (src.getValue()) { 427 case PROPOSAL: 428 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.PROPOSAL); 429 break; 430 case PLAN: 431 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.PLAN); 432 break; 433 case DIRECTIVE: 434 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.DIRECTIVE); 435 break; 436 case ORDER: 437 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.ORDER); 438 break; 439 case ORIGINALORDER: 440 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.ORIGINALORDER); 441 break; 442 case REFLEXORDER: 443 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.REFLEXORDER); 444 break; 445 case FILLERORDER: 446 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.FILLERORDER); 447 break; 448 case INSTANCEORDER: 449 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.INSTANCEORDER); 450 break; 451 case OPTION: 452 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.OPTION); 453 break; 454 default: 455 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestIntent.NULL); 456 break; 457 } 458 } 459 return tgt; 460 } 461 462 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertRequestPriority(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> src) throws FHIRException { 463 if (src == null || src.isEmpty()) 464 return null; 465 Enumeration<Enumerations.RequestPriority> tgt = new Enumeration<>(new Enumerations.RequestPriorityEnumFactory()); 466 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 467 if (src.getValue() == null) { 468 tgt.setValue(null); 469 } else { 470 switch (src.getValue()) { 471 case ROUTINE: 472 tgt.setValue(Enumerations.RequestPriority.ROUTINE); 473 break; 474 case URGENT: 475 tgt.setValue(Enumerations.RequestPriority.URGENT); 476 break; 477 case ASAP: 478 tgt.setValue(Enumerations.RequestPriority.ASAP); 479 break; 480 case STAT: 481 tgt.setValue(Enumerations.RequestPriority.STAT); 482 break; 483 default: 484 tgt.setValue(Enumerations.RequestPriority.NULL); 485 break; 486 } 487 } 488 return tgt; 489 } 490 491 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> convertRequestPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 492 if (src == null || src.isEmpty()) 493 return null; 494 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestPriorityEnumFactory()); 495 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 496 if (src.getValue() == null) { 497 tgt.setValue(null); 498 } else { 499 switch (src.getValue()) { 500 case ROUTINE: 501 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ROUTINE); 502 break; 503 case URGENT: 504 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.URGENT); 505 break; 506 case ASAP: 507 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ASAP); 508 break; 509 case STAT: 510 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.STAT); 511 break; 512 default: 513 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.NULL); 514 break; 515 } 516 } 517 return tgt; 518 } 519 520 public static org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionParticipantComponent convertActivityDefinitionParticipantComponent(org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionParticipantComponent src) throws FHIRException { 521 if (src == null) 522 return null; 523 org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionParticipantComponent tgt = new org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionParticipantComponent(); 524 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 525 if (src.hasType()) 526 tgt.setTypeElement(convertActivityParticipantType(src.getTypeElement())); 527 if (src.hasRole()) 528 tgt.setRole(CodeableConcept43_50.convertCodeableConcept(src.getRole())); 529 return tgt; 530 } 531 532 public static org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionParticipantComponent convertActivityDefinitionParticipantComponent(org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionParticipantComponent src) throws FHIRException { 533 if (src == null) 534 return null; 535 org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionParticipantComponent tgt = new org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionParticipantComponent(); 536 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 537 if (src.hasType()) 538 tgt.setTypeElement(convertActivityParticipantType(src.getTypeElement())); 539 if (src.hasRole()) 540 tgt.setRole(CodeableConcept43_50.convertCodeableConcept(src.getRole())); 541 return tgt; 542 } 543 544 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionParticipantType> convertActivityParticipantType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType> src) throws FHIRException { 545 if (src == null || src.isEmpty()) 546 return null; 547 Enumeration<Enumerations.ActionParticipantType> tgt = new Enumeration<>(new Enumerations.ActionParticipantTypeEnumFactory()); 548 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 549 if (src.getValue() == null) { 550 tgt.setValue(null); 551 } else { 552 switch (src.getValue()) { 553 case PATIENT: 554 tgt.setValue(Enumerations.ActionParticipantType.PATIENT); 555 break; 556 case PRACTITIONER: 557 tgt.setValue(Enumerations.ActionParticipantType.PRACTITIONER); 558 break; 559 case RELATEDPERSON: 560 tgt.setValue(Enumerations.ActionParticipantType.RELATEDPERSON); 561 break; 562 case DEVICE: 563 tgt.setValue(Enumerations.ActionParticipantType.DEVICE); 564 break; 565 default: 566 tgt.setValue(Enumerations.ActionParticipantType.NULL); 567 break; 568 } 569 } 570 return tgt; 571 } 572 573 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType> convertActivityParticipantType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ActionParticipantType> src) throws FHIRException { 574 if (src == null || src.isEmpty()) 575 return null; 576 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ActionParticipantTypeEnumFactory()); 577 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 578 if (src.getValue() == null) { 579 tgt.setValue(null); 580 } else { 581 switch (src.getValue()) { 582 case PATIENT: 583 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.PATIENT); 584 break; 585 case PRACTITIONER: 586 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.PRACTITIONER); 587 break; 588 case RELATEDPERSON: 589 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.RELATEDPERSON); 590 break; 591 case DEVICE: 592 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.DEVICE); 593 break; 594 default: 595 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ActionParticipantType.NULL); 596 break; 597 } 598 } 599 return tgt; 600 } 601 602 public static org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent convertActivityDefinitionDynamicValueComponent(org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent src) throws FHIRException { 603 if (src == null) 604 return null; 605 org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent tgt = new org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent(); 606 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 607 if (src.hasPath()) 608 tgt.setPathElement(String43_50.convertString(src.getPathElement())); 609 if (src.hasExpression()) 610 tgt.setExpression(Expression43_50.convertExpression(src.getExpression())); 611 return tgt; 612 } 613 614 public static org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent convertActivityDefinitionDynamicValueComponent(org.hl7.fhir.r5.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent src) throws FHIRException { 615 if (src == null) 616 return null; 617 org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent tgt = new org.hl7.fhir.r4b.model.ActivityDefinition.ActivityDefinitionDynamicValueComponent(); 618 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 619 if (src.hasPath()) 620 tgt.setPathElement(String43_50.convertString(src.getPathElement())); 621 if (src.hasExpression()) 622 tgt.setExpression(Expression43_50.convertExpression(src.getExpression())); 623 return tgt; 624 } 625}