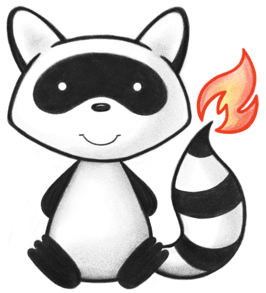
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Coding43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Base64Binary43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Instant43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.AuditEvent; 015import org.hl7.fhir.r5.model.CodeableConcept; 016import org.hl7.fhir.r5.model.Enumeration; 017 018/* 019 Copyright (c) 2011+, HL7, Inc. 020 All rights reserved. 021 022 Redistribution and use in source and binary forms, with or without modification, 023 are permitted provided that the following conditions are met: 024 025 * Redistributions of source code must retain the above copyright notice, this 026 list of conditions and the following disclaimer. 027 * Redistributions in binary form must reproduce the above copyright notice, 028 this list of conditions and the following disclaimer in the documentation 029 and/or other materials provided with the distribution. 030 * Neither the name of HL7 nor the names of its contributors may be used to 031 endorse or promote products derived from this software without specific 032 prior written permission. 033 034 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 035 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 036 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 037 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 038 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 039 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 040 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 041 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 042 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 043 POSSIBILITY OF SUCH DAMAGE. 044 045*/ 046// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 047public class AuditEvent43_50 { 048 049 public static org.hl7.fhir.r5.model.AuditEvent convertAuditEvent(org.hl7.fhir.r4b.model.AuditEvent src) throws FHIRException { 050 if (src == null) 051 return null; 052 org.hl7.fhir.r5.model.AuditEvent tgt = new org.hl7.fhir.r5.model.AuditEvent(); 053 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 054 if (src.hasType()) 055 tgt.getCategoryFirstRep().addCoding(Coding43_50.convertCoding(src.getType())); 056 for (org.hl7.fhir.r4b.model.Coding t : src.getSubtype()) tgt.getCode().addCoding(Coding43_50.convertCoding(t)); 057 if (src.hasAction()) 058 tgt.setActionElement(convertAuditEventAction(src.getActionElement())); 059 if (src.hasPeriod()) 060 tgt.setOccurred(Period43_50.convertPeriod(src.getPeriod())); 061 if (src.hasRecorded()) 062 tgt.setRecordedElement(Instant43_50.convertInstant(src.getRecordedElement())); 063 if (src.hasOutcome()) 064 tgt.getOutcome().getCode().setSystem("http://terminology.hl7.org/CodeSystem/audit-event-outcome").setCode(src.getOutcome().toCode()); 065 if (src.hasOutcomeDesc()) 066 tgt.getOutcome().getDetailFirstRep().setTextElement(String43_50.convertString(src.getOutcomeDescElement())); 067 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getPurposeOfEvent()) 068 tgt.addAuthorization(CodeableConcept43_50.convertCodeableConcept(t)); 069 for (org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentComponent t : src.getAgent()) 070 tgt.addAgent(convertAuditEventAgentComponent(t)); 071 if (src.hasSource()) 072 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 073 for (org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityComponent t : src.getEntity()) 074 tgt.addEntity(convertAuditEventEntityComponent(t)); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.r4b.model.AuditEvent convertAuditEvent(org.hl7.fhir.r5.model.AuditEvent src) throws FHIRException { 079 if (src == null) 080 return null; 081 org.hl7.fhir.r4b.model.AuditEvent tgt = new org.hl7.fhir.r4b.model.AuditEvent(); 082 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 083 if (src.getCategoryFirstRep().hasCoding()) { 084 tgt.setType(Coding43_50.convertCoding(src.getCategoryFirstRep().getCodingFirstRep())); 085 } 086 for (org.hl7.fhir.r5.model.Coding t : src.getCode().getCoding()) tgt.addSubtype(Coding43_50.convertCoding(t)); 087 if (src.hasAction()) 088 tgt.setActionElement(convertAuditEventAction(src.getActionElement())); 089 if (src.hasOccurredPeriod()) 090 tgt.setPeriod(Period43_50.convertPeriod(src.getOccurredPeriod())); 091 if (src.hasRecorded()) 092 tgt.setRecordedElement(Instant43_50.convertInstant(src.getRecordedElement())); 093 if (src.hasOutcome() && "http://terminology.hl7.org/CodeSystem/audit-event-outcome".equals(src.getOutcome().getCode().getSystem())) 094 tgt.getOutcomeElement().setValueAsString(src.getOutcome().getCode().getCode()); 095 if (src.getOutcome().getDetailFirstRep().hasText()) 096 tgt.setOutcomeDescElement(String43_50.convertString(src.getOutcome().getDetailFirstRep().getTextElement())); 097 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getAuthorization()) 098 tgt.addPurposeOfEvent(CodeableConcept43_50.convertCodeableConcept(t)); 099 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent t : src.getAgent()) 100 tgt.addAgent(convertAuditEventAgentComponent(t)); 101 if (src.hasSource()) 102 tgt.setSource(convertAuditEventSourceComponent(src.getSource())); 103 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent t : src.getEntity()) 104 tgt.addEntity(convertAuditEventEntityComponent(t)); 105 return tgt; 106 } 107 108 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction> src) throws FHIRException { 109 if (src == null || src.isEmpty()) 110 return null; 111 Enumeration<AuditEvent.AuditEventAction> tgt = new Enumeration<>(new AuditEvent.AuditEventActionEnumFactory()); 112 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 113 if (src.getValue() == null) { 114 tgt.setValue(null); 115 } else { 116 switch (src.getValue()) { 117 case C: 118 tgt.setValue(AuditEvent.AuditEventAction.C); 119 break; 120 case R: 121 tgt.setValue(AuditEvent.AuditEventAction.R); 122 break; 123 case U: 124 tgt.setValue(AuditEvent.AuditEventAction.U); 125 break; 126 case D: 127 tgt.setValue(AuditEvent.AuditEventAction.D); 128 break; 129 case E: 130 tgt.setValue(AuditEvent.AuditEventAction.E); 131 break; 132 default: 133 tgt.setValue(AuditEvent.AuditEventAction.NULL); 134 break; 135 } 136 } 137 return tgt; 138 } 139 140 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction> convertAuditEventAction(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAction> src) throws FHIRException { 141 if (src == null || src.isEmpty()) 142 return null; 143 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.AuditEvent.AuditEventActionEnumFactory()); 144 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 145 if (src.getValue() == null) { 146 tgt.setValue(null); 147 } else { 148 switch (src.getValue()) { 149 case C: 150 tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction.C); 151 break; 152 case R: 153 tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction.R); 154 break; 155 case U: 156 tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction.U); 157 break; 158 case D: 159 tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction.D); 160 break; 161 case E: 162 tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction.E); 163 break; 164 default: 165 tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAction.NULL); 166 break; 167 } 168 } 169 return tgt; 170 } 171 172 173 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent convertAuditEventAgentComponent(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentComponent src) throws FHIRException { 174 if (src == null) 175 return null; 176 org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent(); 177 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 178 if (src.hasType()) 179 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 180 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getRole()) 181 tgt.addRole(CodeableConcept43_50.convertCodeableConcept(t)); 182 if (src.hasWho()) 183 tgt.setWho(Reference43_50.convertReference(src.getWho())); 184// if (src.hasAltId()) 185// tgt.setAltIdElement(String43_50.convertString(src.getAltIdElement())); 186// if (src.hasName()) 187// tgt.setNameElement(String43_50.convertString(src.getNameElement())); 188 if (src.hasRequestor()) 189 tgt.setRequestorElement(Boolean43_50.convertBoolean(src.getRequestorElement())); 190 if (src.hasLocation()) 191 tgt.setLocation(Reference43_50.convertReference(src.getLocation())); 192 for (org.hl7.fhir.r4b.model.UriType t : src.getPolicy()) tgt.getPolicy().add(Uri43_50.convertUri(t)); 193// if (src.hasMedia()) 194// tgt.setMedia(Coding43_50.convertCoding(src.getMedia())); 195// if (src.hasNetwork()) 196// tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 197 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getPurposeOfUse()) 198 tgt.addAuthorization(CodeableConcept43_50.convertCodeableConcept(t)); 199 return tgt; 200 } 201 202 public static org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentComponent convertAuditEventAgentComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentComponent src) throws FHIRException { 203 if (src == null) 204 return null; 205 org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentComponent tgt = new org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentComponent(); 206 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 207 if (src.hasType()) 208 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 209 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getRole()) 210 tgt.addRole(CodeableConcept43_50.convertCodeableConcept(t)); 211 if (src.hasWho()) 212 tgt.setWho(Reference43_50.convertReference(src.getWho())); 213// if (src.hasAltId()) 214// tgt.setAltIdElement(String43_50.convertString(src.getAltIdElement())); 215// if (src.hasName()) 216// tgt.setNameElement(String43_50.convertString(src.getNameElement())); 217 if (src.hasRequestor()) 218 tgt.setRequestorElement(Boolean43_50.convertBoolean(src.getRequestorElement())); 219 if (src.hasLocation()) 220 tgt.setLocation(Reference43_50.convertReference(src.getLocation())); 221 for (org.hl7.fhir.r5.model.UriType t : src.getPolicy()) tgt.getPolicy().add(Uri43_50.convertUri(t)); 222// if (src.hasMedia()) 223// tgt.setMedia(Coding43_50.convertCoding(src.getMedia())); 224// if (src.hasNetwork()) 225// tgt.setNetwork(convertAuditEventAgentNetworkComponent(src.getNetwork())); 226 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getAuthorization()) 227 tgt.addPurposeOfUse(CodeableConcept43_50.convertCodeableConcept(t)); 228 return tgt; 229 } 230 231// public static org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkComponent src) throws FHIRException { 232// if (src == null) 233// return null; 234// org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent(); 235// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 236// if (src.hasAddress()) 237// tgt.setAddressElement(String43_50.convertString(src.getAddressElement())); 238// if (src.hasType()) 239// tgt.setTypeElement(convertAuditEventAgentNetworkType(src.getTypeElement())); 240// return tgt; 241// } 242// 243// public static org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkComponent convertAuditEventAgentNetworkComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkComponent src) throws FHIRException { 244// if (src == null) 245// return null; 246// org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkComponent tgt = new org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkComponent(); 247// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 248// if (src.hasAddress()) 249// tgt.setAddressElement(String43_50.convertString(src.getAddressElement())); 250// if (src.hasType()) 251// tgt.setTypeElement(convertAuditEventAgentNetworkType(src.getTypeElement())); 252// return tgt; 253// } 254// 255// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> convertAuditEventAgentNetworkType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType> src) throws FHIRException { 256// if (src == null || src.isEmpty()) 257// return null; 258// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkTypeEnumFactory()); 259// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 260// switch (src.getValue()) { 261// case _1: 262// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._1); 263// break; 264// case _2: 265// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._2); 266// break; 267// case _3: 268// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._3); 269// break; 270// case _4: 271// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._4); 272// break; 273// case _5: 274// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType._5); 275// break; 276// default: 277// tgt.setValue(org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType.NULL); 278// break; 279// } 280// return tgt; 281// } 282// 283// static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType> convertAuditEventAgentNetworkType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.AuditEvent.AuditEventAgentNetworkType> src) throws FHIRException { 284// if (src == null || src.isEmpty()) 285// return null; 286// org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkTypeEnumFactory()); 287// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 288// switch (src.getValue()) { 289// case _1: 290// tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType._1); 291// break; 292// case _2: 293// tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType._2); 294// break; 295// case _3: 296// tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType._3); 297// break; 298// case _4: 299// tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType._4); 300// break; 301// case _5: 302// tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType._5); 303// break; 304// default: 305// tgt.setValue(org.hl7.fhir.r4b.model.AuditEvent.AuditEventAgentNetworkType.NULL); 306// break; 307// } 308// return tgt; 309// } 310 311 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.r4b.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 312 if (src == null) 313 return null; 314 org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent(); 315 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 316// if (src.hasSite()) 317// tgt.setSiteElement(String43_50.convertString(src.getSiteElement())); 318 if (src.hasObserver()) 319 tgt.setObserver(Reference43_50.convertReference(src.getObserver())); 320 for (org.hl7.fhir.r4b.model.Coding t : src.getType()) tgt.addType().addCoding(Coding43_50.convertCoding(t)); 321 return tgt; 322 } 323 324 public static org.hl7.fhir.r4b.model.AuditEvent.AuditEventSourceComponent convertAuditEventSourceComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventSourceComponent src) throws FHIRException { 325 if (src == null) 326 return null; 327 org.hl7.fhir.r4b.model.AuditEvent.AuditEventSourceComponent tgt = new org.hl7.fhir.r4b.model.AuditEvent.AuditEventSourceComponent(); 328 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 329// if (src.hasSite()) 330// tgt.setSiteElement(String43_50.convertString(src.getSiteElement())); 331 if (src.hasObserver()) 332 tgt.setObserver(Reference43_50.convertReference(src.getObserver())); 333 for (CodeableConcept t : src.getType()) tgt.addType(Coding43_50.convertCoding(t.getCodingFirstRep())); 334 return tgt; 335 } 336 337 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent convertAuditEventEntityComponent(org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityComponent src) throws FHIRException { 338 if (src == null) 339 return null; 340 org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent(); 341 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 342 if (src.hasWhat()) 343 tgt.setWhat(Reference43_50.convertReference(src.getWhat())); 344// if (src.hasType()) 345// tgt.setType(Coding43_50.convertCoding(src.getType())); 346 if (src.hasRole()) 347 tgt.getRole().addCoding(Coding43_50.convertCoding(src.getRole())); 348// if (src.hasLifecycle()) 349// tgt.setLifecycle(Coding43_50.convertCoding(src.getLifecycle())); 350 for (org.hl7.fhir.r4b.model.Coding t : src.getSecurityLabel()) tgt.addSecurityLabel().addCoding(Coding43_50.convertCoding(t)); 351// if (src.hasName()) 352// tgt.setNameElement(String43_50.convertString(src.getNameElement())); 353 // if (src.hasDescription()) 354 // tgt.setDescriptionElement(convertString(src.getDescriptionElement())); 355 if (src.hasQuery()) 356 tgt.setQueryElement(Base64Binary43_50.convertBase64Binary(src.getQueryElement())); 357 for (org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityDetailComponent t : src.getDetail()) 358 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 359 return tgt; 360 } 361 362 public static org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityComponent convertAuditEventEntityComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityComponent src) throws FHIRException { 363 if (src == null) 364 return null; 365 org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityComponent tgt = new org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityComponent(); 366 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 367 if (src.hasWhat()) 368 tgt.setWhat(Reference43_50.convertReference(src.getWhat())); 369// if (src.hasType()) 370// tgt.setType(Coding43_50.convertCoding(src.getType())); 371 if (src.hasRole()) 372 tgt.setRole(Coding43_50.convertCoding(src.getRole().getCodingFirstRep())); 373// if (src.hasLifecycle()) 374// tgt.setLifecycle(Coding43_50.convertCoding(src.getLifecycle())); 375 for (CodeableConcept t : src.getSecurityLabel()) tgt.addSecurityLabel(Coding43_50.convertCoding(t.getCodingFirstRep())); 376// if (src.hasName()) 377// tgt.setNameElement(String43_50.convertString(src.getNameElement())); 378 // if (src.hasDescription()) 379 // tgt.setDescriptionElement(convertString(src.getDescriptionElement())); 380 if (src.hasQuery()) 381 tgt.setQueryElement(Base64Binary43_50.convertBase64Binary(src.getQueryElement())); 382 for (org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent t : src.getDetail()) 383 tgt.addDetail(convertAuditEventEntityDetailComponent(t)); 384 return tgt; 385 } 386 387 public static org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityDetailComponent src) throws FHIRException { 388 if (src == null) 389 return null; 390 org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent tgt = new org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent(); 391 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 392 if (src.hasType()) 393 tgt.getType().setTextElement(String43_50.convertString(src.getTypeElement())); 394 if (src.hasValue()) 395 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 396 return tgt; 397 } 398 399 public static org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityDetailComponent convertAuditEventEntityDetailComponent(org.hl7.fhir.r5.model.AuditEvent.AuditEventEntityDetailComponent src) throws FHIRException { 400 if (src == null) 401 return null; 402 org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityDetailComponent tgt = new org.hl7.fhir.r4b.model.AuditEvent.AuditEventEntityDetailComponent(); 403 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 404 if (src.getType().hasTextElement()) 405 tgt.setTypeElement(String43_50.convertString(src.getType().getTextElement())); 406 if (src.hasValue()) 407 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 408 return tgt; 409 } 410}