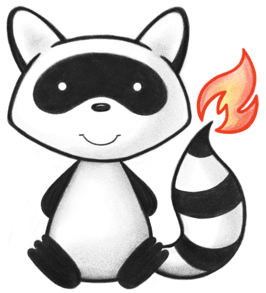
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Attachment43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 010import org.hl7.fhir.exceptions.FHIRException; 011 012/* 013 Copyright (c) 2011+, HL7, Inc. 014 All rights reserved. 015 016 Redistribution and use in source and binary forms, with or without modification, 017 are permitted provided that the following conditions are met: 018 019 * Redistributions of source code must retain the above copyright notice, this 020 list of conditions and the following disclaimer. 021 * Redistributions in binary form must reproduce the above copyright notice, 022 this list of conditions and the following disclaimer in the documentation 023 and/or other materials provided with the distribution. 024 * Neither the name of HL7 nor the names of its contributors may be used to 025 endorse or promote products derived from this software without specific 026 prior written permission. 027 028 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 029 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 030 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 031 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 032 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 033 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 034 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 035 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 036 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 037 POSSIBILITY OF SUCH DAMAGE. 038 039*/ 040// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 041public class BodyStructure43_50 { 042 043 public static org.hl7.fhir.r5.model.BodyStructure convertBodyStructure(org.hl7.fhir.r4b.model.BodyStructure src) throws FHIRException { 044 if (src == null) 045 return null; 046 org.hl7.fhir.r5.model.BodyStructure tgt = new org.hl7.fhir.r5.model.BodyStructure(); 047 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 048 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 049 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 050 if (src.hasActive()) 051 tgt.setActiveElement(Boolean43_50.convertBoolean(src.getActiveElement())); 052 if (src.hasMorphology()) 053 tgt.setMorphology(CodeableConcept43_50.convertCodeableConcept(src.getMorphology())); 054// if (src.hasLocation()) 055// tgt.setLocation(CodeableConcept43_50.convertCodeableConcept(src.getLocation())); 056// for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getLocationQualifier()) 057// tgt.addLocationQualifier(CodeableConcept43_50.convertCodeableConcept(t)); 058 if (src.hasDescription()) 059 tgt.setDescriptionElement(String43_50.convertStringToMarkdown(src.getDescriptionElement())); 060 for (org.hl7.fhir.r4b.model.Attachment t : src.getImage()) tgt.addImage(Attachment43_50.convertAttachment(t)); 061 if (src.hasPatient()) 062 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 063 return tgt; 064 } 065 066 public static org.hl7.fhir.r4b.model.BodyStructure convertBodyStructure(org.hl7.fhir.r5.model.BodyStructure src) throws FHIRException { 067 if (src == null) 068 return null; 069 org.hl7.fhir.r4b.model.BodyStructure tgt = new org.hl7.fhir.r4b.model.BodyStructure(); 070 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 071 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 072 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 073 if (src.hasActive()) 074 tgt.setActiveElement(Boolean43_50.convertBoolean(src.getActiveElement())); 075 if (src.hasMorphology()) 076 tgt.setMorphology(CodeableConcept43_50.convertCodeableConcept(src.getMorphology())); 077// if (src.hasLocation()) 078// tgt.setLocation(CodeableConcept43_50.convertCodeableConcept(src.getLocation())); 079// for (org.hl7.fhir.r5.model.CodeableConcept t : src.getLocationQualifier()) 080// tgt.addLocationQualifier(CodeableConcept43_50.convertCodeableConcept(t)); 081 if (src.hasDescription()) 082 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 083 for (org.hl7.fhir.r5.model.Attachment t : src.getImage()) tgt.addImage(Attachment43_50.convertAttachment(t)); 084 if (src.hasPatient()) 085 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 086 return tgt; 087 } 088}