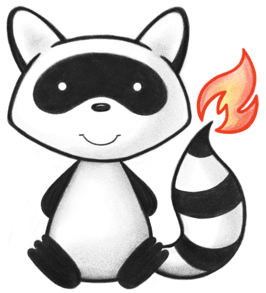
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Signature43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Decimal43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Instant43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.UnsignedInt43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.Bundle; 013import org.hl7.fhir.r5.model.Bundle.LinkRelationTypes; 014import org.hl7.fhir.r5.model.Enumeration; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class Bundle43_50 { 046 047 public static org.hl7.fhir.r5.model.Bundle convertBundle(org.hl7.fhir.r4b.model.Bundle src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.Bundle tgt = new org.hl7.fhir.r5.model.Bundle(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyResource(src, tgt); 052 if (src.hasIdentifier()) 053 tgt.setIdentifier(Identifier43_50.convertIdentifier(src.getIdentifier())); 054 if (src.hasType()) 055 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 056 if (src.hasTimestamp()) 057 tgt.setTimestampElement(Instant43_50.convertInstant(src.getTimestampElement())); 058 if (src.hasTotal()) 059 tgt.setTotalElement(UnsignedInt43_50.convertUnsignedInt(src.getTotalElement())); 060 for (org.hl7.fhir.r4b.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 061 for (org.hl7.fhir.r4b.model.Bundle.BundleEntryComponent t : src.getEntry()) 062 tgt.addEntry(convertBundleEntryComponent(t)); 063 if (src.hasSignature()) 064 tgt.setSignature(Signature43_50.convertSignature(src.getSignature())); 065 return tgt; 066 } 067 068 public static org.hl7.fhir.r4b.model.Bundle convertBundle(org.hl7.fhir.r5.model.Bundle src) throws FHIRException { 069 if (src == null) 070 return null; 071 org.hl7.fhir.r4b.model.Bundle tgt = new org.hl7.fhir.r4b.model.Bundle(); 072 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyResource(src, tgt); 073 if (src.hasIdentifier()) 074 tgt.setIdentifier(Identifier43_50.convertIdentifier(src.getIdentifier())); 075 if (src.hasType()) 076 tgt.setTypeElement(convertBundleType(src.getTypeElement())); 077 if (src.hasTimestamp()) 078 tgt.setTimestampElement(Instant43_50.convertInstant(src.getTimestampElement())); 079 if (src.hasTotal()) 080 tgt.setTotalElement(UnsignedInt43_50.convertUnsignedInt(src.getTotalElement())); 081 for (org.hl7.fhir.r5.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 082 for (org.hl7.fhir.r5.model.Bundle.BundleEntryComponent t : src.getEntry()) 083 tgt.addEntry(convertBundleEntryComponent(t)); 084 if (src.hasSignature()) 085 tgt.setSignature(Signature43_50.convertSignature(src.getSignature())); 086 return tgt; 087 } 088 089 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.BundleType> src) throws FHIRException { 090 if (src == null || src.isEmpty()) 091 return null; 092 Enumeration<Bundle.BundleType> tgt = new Enumeration<>(new Bundle.BundleTypeEnumFactory()); 093 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 094 if (src.getValue() == null) { 095 tgt.setValue(null); 096 } else { 097 switch (src.getValue()) { 098 case DOCUMENT: 099 tgt.setValue(Bundle.BundleType.DOCUMENT); 100 break; 101 case MESSAGE: 102 tgt.setValue(Bundle.BundleType.MESSAGE); 103 break; 104 case TRANSACTION: 105 tgt.setValue(Bundle.BundleType.TRANSACTION); 106 break; 107 case TRANSACTIONRESPONSE: 108 tgt.setValue(Bundle.BundleType.TRANSACTIONRESPONSE); 109 break; 110 case BATCH: 111 tgt.setValue(Bundle.BundleType.BATCH); 112 break; 113 case BATCHRESPONSE: 114 tgt.setValue(Bundle.BundleType.BATCHRESPONSE); 115 break; 116 case HISTORY: 117 tgt.setValue(Bundle.BundleType.HISTORY); 118 break; 119 case SEARCHSET: 120 tgt.setValue(Bundle.BundleType.SEARCHSET); 121 break; 122 case COLLECTION: 123 tgt.setValue(Bundle.BundleType.COLLECTION); 124 break; 125 default: 126 tgt.setValue(Bundle.BundleType.NULL); 127 break; 128 } 129 } 130 return tgt; 131 } 132 133 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.BundleType> convertBundleType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.BundleType> src) throws FHIRException { 134 if (src == null || src.isEmpty()) 135 return null; 136 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.BundleType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Bundle.BundleTypeEnumFactory()); 137 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 138 if (src.getValue() == null) { 139 tgt.setValue(null); 140 } else { 141 switch (src.getValue()) { 142 case DOCUMENT: 143 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.DOCUMENT); 144 break; 145 case MESSAGE: 146 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.MESSAGE); 147 break; 148 case TRANSACTION: 149 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.TRANSACTION); 150 break; 151 case TRANSACTIONRESPONSE: 152 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.TRANSACTIONRESPONSE); 153 break; 154 case BATCH: 155 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.BATCH); 156 break; 157 case BATCHRESPONSE: 158 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.BATCHRESPONSE); 159 break; 160 case HISTORY: 161 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.HISTORY); 162 break; 163 case SEARCHSET: 164 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.SEARCHSET); 165 break; 166 case COLLECTION: 167 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.COLLECTION); 168 break; 169 default: 170 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.BundleType.NULL); 171 break; 172 } 173 } 174 return tgt; 175 } 176 177 public static org.hl7.fhir.r5.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.r4b.model.Bundle.BundleLinkComponent src) throws FHIRException { 178 if (src == null) 179 return null; 180 org.hl7.fhir.r5.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleLinkComponent(); 181 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 182 if (src.hasRelation()) 183 tgt.setRelation(LinkRelationTypes.fromCode(src.getRelation())); 184 if (src.hasUrl()) 185 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 186 return tgt; 187 } 188 189 public static org.hl7.fhir.r4b.model.Bundle.BundleLinkComponent convertBundleLinkComponent(org.hl7.fhir.r5.model.Bundle.BundleLinkComponent src) throws FHIRException { 190 if (src == null) 191 return null; 192 org.hl7.fhir.r4b.model.Bundle.BundleLinkComponent tgt = new org.hl7.fhir.r4b.model.Bundle.BundleLinkComponent(); 193 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 194 if (src.hasRelation()) 195 tgt.setRelation((src.getRelation().toCode())); 196 if (src.hasUrl()) 197 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 198 return tgt; 199 } 200 201 public static org.hl7.fhir.r5.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r4b.model.Bundle.BundleEntryComponent src) throws FHIRException { 202 if (src == null) 203 return null; 204 org.hl7.fhir.r5.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryComponent(); 205 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 206 for (org.hl7.fhir.r4b.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 207 if (src.hasFullUrl()) 208 tgt.setFullUrlElement(Uri43_50.convertUri(src.getFullUrlElement())); 209 if (src.hasResource()) 210 tgt.setResource(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertResource(src.getResource())); 211 if (src.hasSearch()) 212 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 213 if (src.hasRequest()) 214 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 215 if (src.hasResponse()) 216 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 217 return tgt; 218 } 219 220 public static org.hl7.fhir.r4b.model.Bundle.BundleEntryComponent convertBundleEntryComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryComponent src) throws FHIRException { 221 if (src == null) 222 return null; 223 org.hl7.fhir.r4b.model.Bundle.BundleEntryComponent tgt = new org.hl7.fhir.r4b.model.Bundle.BundleEntryComponent(); 224 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 225 for (org.hl7.fhir.r5.model.Bundle.BundleLinkComponent t : src.getLink()) tgt.addLink(convertBundleLinkComponent(t)); 226 if (src.hasFullUrl()) 227 tgt.setFullUrlElement(Uri43_50.convertUri(src.getFullUrlElement())); 228 if (src.hasResource()) 229 tgt.setResource(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertResource(src.getResource())); 230 if (src.hasSearch()) 231 tgt.setSearch(convertBundleEntrySearchComponent(src.getSearch())); 232 if (src.hasRequest()) 233 tgt.setRequest(convertBundleEntryRequestComponent(src.getRequest())); 234 if (src.hasResponse()) 235 tgt.setResponse(convertBundleEntryResponseComponent(src.getResponse())); 236 return tgt; 237 } 238 239 public static org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.r4b.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 240 if (src == null) 241 return null; 242 org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent(); 243 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 244 if (src.hasMode()) 245 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 246 if (src.hasScore()) 247 tgt.setScoreElement(Decimal43_50.convertDecimal(src.getScoreElement())); 248 return tgt; 249 } 250 251 public static org.hl7.fhir.r4b.model.Bundle.BundleEntrySearchComponent convertBundleEntrySearchComponent(org.hl7.fhir.r5.model.Bundle.BundleEntrySearchComponent src) throws FHIRException { 252 if (src == null) 253 return null; 254 org.hl7.fhir.r4b.model.Bundle.BundleEntrySearchComponent tgt = new org.hl7.fhir.r4b.model.Bundle.BundleEntrySearchComponent(); 255 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 256 if (src.hasMode()) 257 tgt.setModeElement(convertSearchEntryMode(src.getModeElement())); 258 if (src.hasScore()) 259 tgt.setScoreElement(Decimal43_50.convertDecimal(src.getScoreElement())); 260 return tgt; 261 } 262 263 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.SearchEntryMode> src) throws FHIRException { 264 if (src == null || src.isEmpty()) 265 return null; 266 Enumeration<Bundle.SearchEntryMode> tgt = new Enumeration<>(new Bundle.SearchEntryModeEnumFactory()); 267 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 268 if (src.getValue() == null) { 269 tgt.setValue(null); 270 } else { 271 switch (src.getValue()) { 272 case MATCH: 273 tgt.setValue(Bundle.SearchEntryMode.MATCH); 274 break; 275 case INCLUDE: 276 tgt.setValue(Bundle.SearchEntryMode.INCLUDE); 277 break; 278 case OUTCOME: 279 tgt.setValue(Bundle.SearchEntryMode.OUTCOME); 280 break; 281 default: 282 tgt.setValue(Bundle.SearchEntryMode.NULL); 283 break; 284 } 285 } 286 return tgt; 287 } 288 289 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.SearchEntryMode> convertSearchEntryMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.SearchEntryMode> src) throws FHIRException { 290 if (src == null || src.isEmpty()) 291 return null; 292 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.SearchEntryMode> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Bundle.SearchEntryModeEnumFactory()); 293 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 294 if (src.getValue() == null) { 295 tgt.setValue(null); 296 } else { 297 switch (src.getValue()) { 298 case MATCH: 299 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.SearchEntryMode.MATCH); 300 break; 301 case INCLUDE: 302 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.SearchEntryMode.INCLUDE); 303 break; 304 case OUTCOME: 305 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.SearchEntryMode.OUTCOME); 306 break; 307 default: 308 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.SearchEntryMode.NULL); 309 break; 310 } 311 } 312 return tgt; 313 } 314 315 public static org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.r4b.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 316 if (src == null) 317 return null; 318 org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent(); 319 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 320 if (src.hasMethod()) 321 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 322 if (src.hasUrl()) 323 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 324 if (src.hasIfNoneMatch()) 325 tgt.setIfNoneMatchElement(String43_50.convertString(src.getIfNoneMatchElement())); 326 if (src.hasIfModifiedSince()) 327 tgt.setIfModifiedSinceElement(Instant43_50.convertInstant(src.getIfModifiedSinceElement())); 328 if (src.hasIfMatch()) 329 tgt.setIfMatchElement(String43_50.convertString(src.getIfMatchElement())); 330 if (src.hasIfNoneExist()) 331 tgt.setIfNoneExistElement(String43_50.convertString(src.getIfNoneExistElement())); 332 return tgt; 333 } 334 335 public static org.hl7.fhir.r4b.model.Bundle.BundleEntryRequestComponent convertBundleEntryRequestComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryRequestComponent src) throws FHIRException { 336 if (src == null) 337 return null; 338 org.hl7.fhir.r4b.model.Bundle.BundleEntryRequestComponent tgt = new org.hl7.fhir.r4b.model.Bundle.BundleEntryRequestComponent(); 339 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 340 if (src.hasMethod()) 341 tgt.setMethodElement(convertHTTPVerb(src.getMethodElement())); 342 if (src.hasUrl()) 343 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 344 if (src.hasIfNoneMatch()) 345 tgt.setIfNoneMatchElement(String43_50.convertString(src.getIfNoneMatchElement())); 346 if (src.hasIfModifiedSince()) 347 tgt.setIfModifiedSinceElement(Instant43_50.convertInstant(src.getIfModifiedSinceElement())); 348 if (src.hasIfMatch()) 349 tgt.setIfMatchElement(String43_50.convertString(src.getIfMatchElement())); 350 if (src.hasIfNoneExist()) 351 tgt.setIfNoneExistElement(String43_50.convertString(src.getIfNoneExistElement())); 352 return tgt; 353 } 354 355 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.HTTPVerb> src) throws FHIRException { 356 if (src == null || src.isEmpty()) 357 return null; 358 Enumeration<Bundle.HTTPVerb> tgt = new Enumeration<>(new Bundle.HTTPVerbEnumFactory()); 359 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 360 if (src.getValue() == null) { 361 tgt.setValue(null); 362 } else { 363 switch (src.getValue()) { 364 case GET: 365 tgt.setValue(Bundle.HTTPVerb.GET); 366 break; 367 case HEAD: 368 tgt.setValue(Bundle.HTTPVerb.HEAD); 369 break; 370 case POST: 371 tgt.setValue(Bundle.HTTPVerb.POST); 372 break; 373 case PUT: 374 tgt.setValue(Bundle.HTTPVerb.PUT); 375 break; 376 case DELETE: 377 tgt.setValue(Bundle.HTTPVerb.DELETE); 378 break; 379 case PATCH: 380 tgt.setValue(Bundle.HTTPVerb.PATCH); 381 break; 382 default: 383 tgt.setValue(Bundle.HTTPVerb.NULL); 384 break; 385 } 386 } 387 return tgt; 388 } 389 390 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.HTTPVerb> convertHTTPVerb(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Bundle.HTTPVerb> src) throws FHIRException { 391 if (src == null || src.isEmpty()) 392 return null; 393 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Bundle.HTTPVerb> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Bundle.HTTPVerbEnumFactory()); 394 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 395 if (src.getValue() == null) { 396 tgt.setValue(null); 397 } else { 398 switch (src.getValue()) { 399 case GET: 400 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.HTTPVerb.GET); 401 break; 402 case HEAD: 403 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.HTTPVerb.HEAD); 404 break; 405 case POST: 406 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.HTTPVerb.POST); 407 break; 408 case PUT: 409 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.HTTPVerb.PUT); 410 break; 411 case DELETE: 412 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.HTTPVerb.DELETE); 413 break; 414 case PATCH: 415 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.HTTPVerb.PATCH); 416 break; 417 default: 418 tgt.setValue(org.hl7.fhir.r4b.model.Bundle.HTTPVerb.NULL); 419 break; 420 } 421 } 422 return tgt; 423 } 424 425 public static org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.r4b.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 426 if (src == null) 427 return null; 428 org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent(); 429 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 430 if (src.hasStatus()) 431 tgt.setStatusElement(String43_50.convertString(src.getStatusElement())); 432 if (src.hasLocation()) 433 tgt.setLocationElement(Uri43_50.convertUri(src.getLocationElement())); 434 if (src.hasEtag()) 435 tgt.setEtagElement(String43_50.convertString(src.getEtagElement())); 436 if (src.hasLastModified()) 437 tgt.setLastModifiedElement(Instant43_50.convertInstant(src.getLastModifiedElement())); 438 if (src.hasOutcome()) 439 tgt.setOutcome(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertResource(src.getOutcome())); 440 return tgt; 441 } 442 443 public static org.hl7.fhir.r4b.model.Bundle.BundleEntryResponseComponent convertBundleEntryResponseComponent(org.hl7.fhir.r5.model.Bundle.BundleEntryResponseComponent src) throws FHIRException { 444 if (src == null) 445 return null; 446 org.hl7.fhir.r4b.model.Bundle.BundleEntryResponseComponent tgt = new org.hl7.fhir.r4b.model.Bundle.BundleEntryResponseComponent(); 447 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 448 if (src.hasStatus()) 449 tgt.setStatusElement(String43_50.convertString(src.getStatusElement())); 450 if (src.hasLocation()) 451 tgt.setLocationElement(Uri43_50.convertUri(src.getLocationElement())); 452 if (src.hasEtag()) 453 tgt.setEtagElement(String43_50.convertString(src.getEtagElement())); 454 if (src.hasLastModified()) 455 tgt.setLastModifiedElement(Instant43_50.convertInstant(src.getLastModifiedElement())); 456 if (src.hasOutcome()) 457 tgt.setOutcome(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertResource(src.getOutcome())); 458 return tgt; 459 } 460}