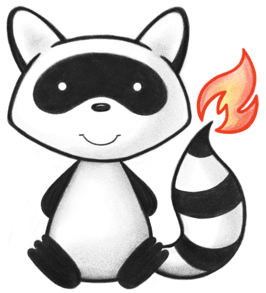
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.CodeableReference; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class CarePlan43_50 { 046 047 public static org.hl7.fhir.r5.model.CarePlan convertCarePlan(org.hl7.fhir.r4b.model.CarePlan src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.CarePlan tgt = new org.hl7.fhir.r5.model.CarePlan(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 054 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getInstantiatesCanonical()) 055 tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 056 for (org.hl7.fhir.r4b.model.UriType t : src.getInstantiatesUri()) 057 tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 058 for (org.hl7.fhir.r4b.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 059 for (org.hl7.fhir.r4b.model.Reference t : src.getReplaces()) tgt.addReplaces(Reference43_50.convertReference(t)); 060 for (org.hl7.fhir.r4b.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference43_50.convertReference(t)); 061 if (src.hasStatus()) 062 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 063 if (src.hasIntent()) 064 tgt.setIntentElement(convertCarePlanIntent(src.getIntentElement())); 065 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCategory()) 066 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 067 if (src.hasTitle()) 068 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 069 if (src.hasDescription()) 070 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 071 if (src.hasSubject()) 072 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 073 if (src.hasEncounter()) 074 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 075 if (src.hasPeriod()) 076 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 077 if (src.hasCreated()) 078 tgt.setCreatedElement(DateTime43_50.convertDateTime(src.getCreatedElement())); 079 if (src.hasAuthor()) 080 tgt.setCustodian(Reference43_50.convertReference(src.getAuthor())); 081 for (org.hl7.fhir.r4b.model.Reference t : src.getContributor()) 082 tgt.addContributor(Reference43_50.convertReference(t)); 083 for (org.hl7.fhir.r4b.model.Reference t : src.getCareTeam()) tgt.addCareTeam(Reference43_50.convertReference(t)); 084 for (org.hl7.fhir.r4b.model.Reference t : src.getAddresses()) 085 tgt.addAddresses(Reference43_50.convertReferenceToCodeableReference(t)); 086 for (org.hl7.fhir.r4b.model.Reference t : src.getSupportingInfo()) 087 tgt.addSupportingInfo(Reference43_50.convertReference(t)); 088 for (org.hl7.fhir.r4b.model.Reference t : src.getGoal()) tgt.addGoal(Reference43_50.convertReference(t)); 089 for (org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) 090 tgt.addActivity(convertCarePlanActivityComponent(t)); 091 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 092 return tgt; 093 } 094 095 public static org.hl7.fhir.r4b.model.CarePlan convertCarePlan(org.hl7.fhir.r5.model.CarePlan src) throws FHIRException { 096 if (src == null) 097 return null; 098 org.hl7.fhir.r4b.model.CarePlan tgt = new org.hl7.fhir.r4b.model.CarePlan(); 099 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 100 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 101 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 102 for (org.hl7.fhir.r5.model.CanonicalType t : src.getInstantiatesCanonical()) 103 tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 104 for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesUri()) 105 tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 106 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 107 for (org.hl7.fhir.r5.model.Reference t : src.getReplaces()) tgt.addReplaces(Reference43_50.convertReference(t)); 108 for (org.hl7.fhir.r5.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference43_50.convertReference(t)); 109 if (src.hasStatus()) 110 tgt.setStatusElement(convertCarePlanStatus(src.getStatusElement())); 111 if (src.hasIntent()) 112 tgt.setIntentElement(convertCarePlanIntent(src.getIntentElement())); 113 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 114 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 115 if (src.hasTitle()) 116 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 117 if (src.hasDescription()) 118 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 119 if (src.hasSubject()) 120 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 121 if (src.hasEncounter()) 122 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 123 if (src.hasPeriod()) 124 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 125 if (src.hasCreated()) 126 tgt.setCreatedElement(DateTime43_50.convertDateTime(src.getCreatedElement())); 127 if (src.hasCustodian()) 128 tgt.setAuthor(Reference43_50.convertReference(src.getCustodian())); 129 for (org.hl7.fhir.r5.model.Reference t : src.getContributor()) 130 tgt.addContributor(Reference43_50.convertReference(t)); 131 for (org.hl7.fhir.r5.model.Reference t : src.getCareTeam()) tgt.addCareTeam(Reference43_50.convertReference(t)); 132 for (CodeableReference t : src.getAddresses()) 133 if (t.hasReference()) 134 tgt.addAddresses(Reference43_50.convertReference(t.getReference())); 135 for (org.hl7.fhir.r5.model.Reference t : src.getSupportingInfo()) 136 tgt.addSupportingInfo(Reference43_50.convertReference(t)); 137 for (org.hl7.fhir.r5.model.Reference t : src.getGoal()) tgt.addGoal(Reference43_50.convertReference(t)); 138 for (org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent t : src.getActivity()) 139 tgt.addActivity(convertCarePlanActivityComponent(t)); 140 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 141 return tgt; 142 } 143 144 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> convertCarePlanStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestStatus> src) throws FHIRException { 145 if (src == null || src.isEmpty()) 146 return null; 147 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.RequestStatusEnumFactory()); 148 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 149 switch (src.getValue()) { 150 case DRAFT: 151 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.DRAFT); 152 break; 153 case ACTIVE: 154 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.ACTIVE); 155 break; 156 case ONHOLD: 157 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.ONHOLD); 158 break; 159 case REVOKED: 160 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.REVOKED); 161 break; 162 case COMPLETED: 163 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.COMPLETED); 164 break; 165 case ENTEREDINERROR: 166 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.ENTEREDINERROR); 167 break; 168 case UNKNOWN: 169 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.UNKNOWN); 170 break; 171 default: 172 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestStatus.NULL); 173 break; 174 } 175 return tgt; 176 } 177 178 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestStatus> convertCarePlanStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestStatus> src) throws FHIRException { 179 if (src == null || src.isEmpty()) 180 return null; 181 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestStatusEnumFactory()); 182 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 183 switch (src.getValue()) { 184 case DRAFT: 185 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.DRAFT); 186 break; 187 case ACTIVE: 188 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.ACTIVE); 189 break; 190 case ONHOLD: 191 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.ONHOLD); 192 break; 193 case REVOKED: 194 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.REVOKED); 195 break; 196 case COMPLETED: 197 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.COMPLETED); 198 break; 199 case ENTEREDINERROR: 200 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.ENTEREDINERROR); 201 break; 202 case UNKNOWN: 203 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.UNKNOWN); 204 break; 205 default: 206 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestStatus.NULL); 207 break; 208 } 209 return tgt; 210 } 211 212 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanIntent> convertCarePlanIntent(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanIntent> src) throws FHIRException { 213 if (src == null || src.isEmpty()) 214 return null; 215 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanIntent> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CarePlan.CarePlanIntentEnumFactory()); 216 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 217 switch (src.getValue()) { 218 case PROPOSAL: 219 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.PROPOSAL); 220 break; 221 case PLAN: 222 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.PLAN); 223 break; 224 case ORDER: 225 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.ORDER); 226 break; 227 case OPTION: 228 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.OPTION); 229 break; 230 default: 231 tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanIntent.NULL); 232 break; 233 } 234 return tgt; 235 } 236 237 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanIntent> convertCarePlanIntent(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanIntent> src) throws FHIRException { 238 if (src == null || src.isEmpty()) 239 return null; 240 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanIntent> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.CarePlan.CarePlanIntentEnumFactory()); 241 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 242 switch (src.getValue()) { 243 case PROPOSAL: 244 tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanIntent.PROPOSAL); 245 break; 246 case PLAN: 247 tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanIntent.PLAN); 248 break; 249 case ORDER: 250 tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanIntent.ORDER); 251 break; 252 case OPTION: 253 tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanIntent.OPTION); 254 break; 255 default: 256 tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanIntent.NULL); 257 break; 258 } 259 return tgt; 260 } 261 262 public static org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 263 if (src == null) 264 return null; 265 org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent(); 266 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 267 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getOutcomeCodeableConcept()) 268 tgt.addPerformedActivity(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 269 for (org.hl7.fhir.r4b.model.Reference t : src.getOutcomeReference()) 270 tgt.addPerformedActivity(Reference43_50.convertReferenceToCodeableReference(t)); 271 for (org.hl7.fhir.r4b.model.Annotation t : src.getProgress()) tgt.addProgress(Annotation43_50.convertAnnotation(t)); 272 if (src.hasReference()) 273 tgt.setPlannedActivityReference(Reference43_50.convertReference(src.getReference())); 274// if (src.hasDetail()) 275// tgt.setPlannedActivityDetail(convertCarePlanActivityDetailComponent(src.getDetail())); 276 return tgt; 277 } 278 279 public static org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityComponent convertCarePlanActivityComponent(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityComponent src) throws FHIRException { 280 if (src == null) 281 return null; 282 org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityComponent tgt = new org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityComponent(); 283 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 284 for (CodeableReference t : src.getPerformedActivity()) 285 if (t.hasConcept()) 286 tgt.addOutcomeCodeableConcept(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 287 for (CodeableReference t : src.getPerformedActivity()) 288 if (t.hasReference()) 289 tgt.addOutcomeReference(Reference43_50.convertReference(t.getReference())); 290 for (org.hl7.fhir.r5.model.Annotation t : src.getProgress()) tgt.addProgress(Annotation43_50.convertAnnotation(t)); 291 if (src.hasPlannedActivityReference()) 292 tgt.setReference(Reference43_50.convertReference(src.getPlannedActivityReference())); 293// if (src.hasPlannedActivityDetail()) 294// tgt.setDetail(convertCarePlanActivityDetailComponent(src.getPlannedActivityDetail())); 295 return tgt; 296 } 297 298// public static org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityDetailComponent src) throws FHIRException { 299// if (src == null) 300// return null; 301// org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent tgt = new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent(); 302// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 303// if (src.hasKind()) 304// tgt.setKindElement(convertCarePlanActivityKind(src.getKindElement())); 305// for (org.hl7.fhir.r4b.model.CanonicalType t : src.getInstantiatesCanonical()) 306// tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 307// for (org.hl7.fhir.r4b.model.UriType t : src.getInstantiatesUri()) 308// tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 309// if (src.hasCode()) 310// tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 311// for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 312// tgt.addReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 313// for (org.hl7.fhir.r4b.model.Reference t : src.getReasonReference()) 314// tgt.addReason(Reference43_50.convertReferenceToCodeableReference(t)); 315// for (org.hl7.fhir.r4b.model.Reference t : src.getGoal()) tgt.addGoal(Reference43_50.convertReference(t)); 316// if (src.hasStatus()) 317// tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 318// if (src.hasStatusReason()) 319// tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 320// if (src.hasDoNotPerform()) 321// tgt.setDoNotPerformElement(Boolean43_50.convertBoolean(src.getDoNotPerformElement())); 322// if (src.hasScheduled()) 323// tgt.setScheduled(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getScheduled())); 324// if (src.hasLocation()) 325// tgt.getLocation().setReference(Reference43_50.convertReference(src.getLocation())); 326// for (org.hl7.fhir.r4b.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference43_50.convertReference(t)); 327// if (src.hasProduct()) 328// tgt.setProduct(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getProduct())); 329// if (src.hasDailyAmount()) 330// tgt.setDailyAmount(SimpleQuantity43_50.convertSimpleQuantity(src.getDailyAmount())); 331// if (src.hasQuantity()) 332// tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 333// if (src.hasDescription()) 334// tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 335// return tgt; 336// } 337// 338// public static org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityDetailComponent convertCarePlanActivityDetailComponent(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityPlannedActivityDetailComponent src) throws FHIRException { 339// if (src == null) 340// return null; 341// org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityDetailComponent tgt = new org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityDetailComponent(); 342// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 343// if (src.hasKind()) 344// tgt.setKindElement(convertCarePlanActivityKind(src.getKindElement())); 345// for (org.hl7.fhir.r5.model.CanonicalType t : src.getInstantiatesCanonical()) 346// tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 347// for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesUri()) 348// tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 349// if (src.hasCode()) 350// tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 351// for (CodeableReference t : src.getReason()) 352// if (t.hasConcept()) 353// tgt.addReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 354// for (CodeableReference t : src.getReason()) 355// if (t.hasReference()) 356// tgt.addReasonReference(Reference43_50.convertReference(t.getReference())); 357// for (org.hl7.fhir.r5.model.Reference t : src.getGoal()) tgt.addGoal(Reference43_50.convertReference(t)); 358// if (src.hasStatus()) 359// tgt.setStatusElement(convertCarePlanActivityStatus(src.getStatusElement())); 360// if (src.hasStatusReason()) 361// tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 362// if (src.hasDoNotPerform()) 363// tgt.setDoNotPerformElement(Boolean43_50.convertBoolean(src.getDoNotPerformElement())); 364// if (src.hasScheduled()) 365// tgt.setScheduled(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getScheduled())); 366// if (src.getLocation().hasReference()) 367// tgt.setLocation(Reference43_50.convertReference(src.getLocation().getReference())); 368// for (org.hl7.fhir.r5.model.Reference t : src.getPerformer()) tgt.addPerformer(Reference43_50.convertReference(t)); 369// if (src.hasProduct()) 370// tgt.setProduct(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getProduct())); 371// if (src.hasDailyAmount()) 372// tgt.setDailyAmount(SimpleQuantity43_50.convertSimpleQuantity(src.getDailyAmount())); 373// if (src.hasQuantity()) 374// tgt.setQuantity(SimpleQuantity43_50.convertSimpleQuantity(src.getQuantity())); 375// if (src.hasDescription()) 376// tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 377// return tgt; 378// } 379// 380// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind> convertCarePlanActivityKind(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind> src) throws FHIRException { 381// if (src == null || src.isEmpty()) 382// return null; 383// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKindEnumFactory()); 384// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 385// switch (src.getValue()) { 386// case APPOINTMENT: 387// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.APPOINTMENT); 388// break; 389// case COMMUNICATIONREQUEST: 390// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.COMMUNICATIONREQUEST); 391// break; 392// case DEVICEREQUEST: 393// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.DEVICEREQUEST); 394// break; 395// case MEDICATIONREQUEST: 396// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.MEDICATIONREQUEST); 397// break; 398// case NUTRITIONORDER: 399// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.NUTRITIONORDER); 400// break; 401// case TASK: 402// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.TASK); 403// break; 404// case SERVICEREQUEST: 405// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.SERVICEREQUEST); 406// break; 407// case VISIONPRESCRIPTION: 408// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.VISIONPRESCRIPTION); 409// break; 410// default: 411// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind.NULL); 412// break; 413// } 414// return tgt; 415// } 416// 417// static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind> convertCarePlanActivityKind(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityKind> src) throws FHIRException { 418// if (src == null || src.isEmpty()) 419// return null; 420// org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKindEnumFactory()); 421// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 422// switch (src.getValue()) { 423// case APPOINTMENT: 424// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.APPOINTMENT); 425// break; 426// case COMMUNICATIONREQUEST: 427// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.COMMUNICATIONREQUEST); 428// break; 429// case DEVICEREQUEST: 430// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.DEVICEREQUEST); 431// break; 432// case MEDICATIONREQUEST: 433// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.MEDICATIONREQUEST); 434// break; 435// case NUTRITIONORDER: 436// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.NUTRITIONORDER); 437// break; 438// case TASK: 439// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.TASK); 440// break; 441// case SERVICEREQUEST: 442// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.SERVICEREQUEST); 443// break; 444// case VISIONPRESCRIPTION: 445// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.VISIONPRESCRIPTION); 446// break; 447// default: 448// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityKind.NULL); 449// break; 450// } 451// return tgt; 452// } 453// 454// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 455// if (src == null || src.isEmpty()) 456// return null; 457// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatusEnumFactory()); 458// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 459// switch (src.getValue()) { 460// case NOTSTARTED: 461// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 462// break; 463// case SCHEDULED: 464// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 465// break; 466// case INPROGRESS: 467// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 468// break; 469// case ONHOLD: 470// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.ONHOLD); 471// break; 472// case COMPLETED: 473// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.COMPLETED); 474// break; 475// case CANCELLED: 476// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.CANCELLED); 477// break; 478// case STOPPED: 479// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.STOPPED); 480// break; 481// case UNKNOWN: 482// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.UNKNOWN); 483// break; 484// case ENTEREDINERROR: 485// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.ENTEREDINERROR); 486// break; 487// default: 488// tgt.setValue(org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus.NULL); 489// break; 490// } 491// return tgt; 492// } 493// 494// static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus> convertCarePlanActivityStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CarePlan.CarePlanActivityStatus> src) throws FHIRException { 495// if (src == null || src.isEmpty()) 496// return null; 497// org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatusEnumFactory()); 498// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 499// switch (src.getValue()) { 500// case NOTSTARTED: 501// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.NOTSTARTED); 502// break; 503// case SCHEDULED: 504// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.SCHEDULED); 505// break; 506// case INPROGRESS: 507// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.INPROGRESS); 508// break; 509// case ONHOLD: 510// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.ONHOLD); 511// break; 512// case COMPLETED: 513// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.COMPLETED); 514// break; 515// case CANCELLED: 516// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.CANCELLED); 517// break; 518// case STOPPED: 519// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.STOPPED); 520// break; 521// case UNKNOWN: 522// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.UNKNOWN); 523// break; 524// case ENTEREDINERROR: 525// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.ENTEREDINERROR); 526// break; 527// default: 528// tgt.setValue(org.hl7.fhir.r4b.model.CarePlan.CarePlanActivityStatus.NULL); 529// break; 530// } 531// return tgt; 532// } 533}