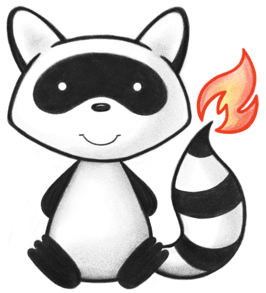
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.ContactPoint43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.CareTeam; 013import org.hl7.fhir.r5.model.CodeableReference; 014import org.hl7.fhir.r5.model.Enumeration; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class CareTeam43_50 { 046 047 public static org.hl7.fhir.r5.model.CareTeam convertCareTeam(org.hl7.fhir.r4b.model.CareTeam src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.CareTeam tgt = new org.hl7.fhir.r5.model.CareTeam(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 054 if (src.hasStatus()) 055 tgt.setStatusElement(convertCareTeamStatus(src.getStatusElement())); 056 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCategory()) 057 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 058 if (src.hasName()) 059 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 060 if (src.hasSubject()) 061 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 062 if (src.hasPeriod()) 063 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 064 for (org.hl7.fhir.r4b.model.CareTeam.CareTeamParticipantComponent t : src.getParticipant()) 065 tgt.addParticipant(convertCareTeamParticipantComponent(t)); 066 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 067 tgt.addReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 068 for (org.hl7.fhir.r4b.model.Reference t : src.getReasonReference()) 069 tgt.addReason(Reference43_50.convertReferenceToCodeableReference(t)); 070 for (org.hl7.fhir.r4b.model.Reference t : src.getManagingOrganization()) 071 tgt.addManagingOrganization(Reference43_50.convertReference(t)); 072 for (org.hl7.fhir.r4b.model.ContactPoint t : src.getTelecom()) 073 tgt.addTelecom(ContactPoint43_50.convertContactPoint(t)); 074 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.r4b.model.CareTeam convertCareTeam(org.hl7.fhir.r5.model.CareTeam src) throws FHIRException { 079 if (src == null) 080 return null; 081 org.hl7.fhir.r4b.model.CareTeam tgt = new org.hl7.fhir.r4b.model.CareTeam(); 082 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 083 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 084 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 085 if (src.hasStatus()) 086 tgt.setStatusElement(convertCareTeamStatus(src.getStatusElement())); 087 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 088 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 089 if (src.hasName()) 090 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 091 if (src.hasSubject()) 092 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 093 if (src.hasPeriod()) 094 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 095 for (org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent t : src.getParticipant()) 096 tgt.addParticipant(convertCareTeamParticipantComponent(t)); 097 for (CodeableReference t : src.getReason()) 098 if (t.hasConcept()) 099 tgt.addReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 100 for (CodeableReference t : src.getReason()) 101 if (t.hasReference()) 102 tgt.addReasonReference(Reference43_50.convertReference(t.getReference())); 103 for (org.hl7.fhir.r5.model.Reference t : src.getManagingOrganization()) 104 tgt.addManagingOrganization(Reference43_50.convertReference(t)); 105 for (org.hl7.fhir.r5.model.ContactPoint t : src.getTelecom()) 106 tgt.addTelecom(ContactPoint43_50.convertContactPoint(t)); 107 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 108 return tgt; 109 } 110 111 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CareTeam.CareTeamStatus> convertCareTeamStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus> src) throws FHIRException { 112 if (src == null || src.isEmpty()) 113 return null; 114 Enumeration<CareTeam.CareTeamStatus> tgt = new Enumeration<>(new CareTeam.CareTeamStatusEnumFactory()); 115 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 116 if (src.getValue() == null) { 117 tgt.setValue(null); 118 } else { 119 switch (src.getValue()) { 120 case PROPOSED: 121 tgt.setValue(CareTeam.CareTeamStatus.PROPOSED); 122 break; 123 case ACTIVE: 124 tgt.setValue(CareTeam.CareTeamStatus.ACTIVE); 125 break; 126 case SUSPENDED: 127 tgt.setValue(CareTeam.CareTeamStatus.SUSPENDED); 128 break; 129 case INACTIVE: 130 tgt.setValue(CareTeam.CareTeamStatus.INACTIVE); 131 break; 132 case ENTEREDINERROR: 133 tgt.setValue(CareTeam.CareTeamStatus.ENTEREDINERROR); 134 break; 135 default: 136 tgt.setValue(CareTeam.CareTeamStatus.NULL); 137 break; 138 } 139 } 140 return tgt; 141 } 142 143 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus> convertCareTeamStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CareTeam.CareTeamStatus> src) throws FHIRException { 144 if (src == null || src.isEmpty()) 145 return null; 146 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.CareTeam.CareTeamStatusEnumFactory()); 147 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 148 if (src.getValue() == null) { 149 tgt.setValue(null); 150 } else { 151 switch (src.getValue()) { 152 case PROPOSED: 153 tgt.setValue(org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus.PROPOSED); 154 break; 155 case ACTIVE: 156 tgt.setValue(org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus.ACTIVE); 157 break; 158 case SUSPENDED: 159 tgt.setValue(org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus.SUSPENDED); 160 break; 161 case INACTIVE: 162 tgt.setValue(org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus.INACTIVE); 163 break; 164 case ENTEREDINERROR: 165 tgt.setValue(org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus.ENTEREDINERROR); 166 break; 167 default: 168 tgt.setValue(org.hl7.fhir.r4b.model.CareTeam.CareTeamStatus.NULL); 169 break; 170 } 171 } 172 return tgt; 173 } 174 175 public static org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent convertCareTeamParticipantComponent(org.hl7.fhir.r4b.model.CareTeam.CareTeamParticipantComponent src) throws FHIRException { 176 if (src == null) 177 return null; 178 org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent tgt = new org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent(); 179 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 180 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getRole()) 181 tgt.setRole(CodeableConcept43_50.convertCodeableConcept(t)); 182 if (src.hasMember()) 183 tgt.setMember(Reference43_50.convertReference(src.getMember())); 184 if (src.hasOnBehalfOf()) 185 tgt.setOnBehalfOf(Reference43_50.convertReference(src.getOnBehalfOf())); 186 if (src.hasPeriod()) 187 tgt.setCoverage(Period43_50.convertPeriod(src.getPeriod())); 188 return tgt; 189 } 190 191 public static org.hl7.fhir.r4b.model.CareTeam.CareTeamParticipantComponent convertCareTeamParticipantComponent(org.hl7.fhir.r5.model.CareTeam.CareTeamParticipantComponent src) throws FHIRException { 192 if (src == null) 193 return null; 194 org.hl7.fhir.r4b.model.CareTeam.CareTeamParticipantComponent tgt = new org.hl7.fhir.r4b.model.CareTeam.CareTeamParticipantComponent(); 195 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 196 if (src.hasRole()) 197 tgt.addRole(CodeableConcept43_50.convertCodeableConcept(src.getRole())); 198 if (src.hasMember()) 199 tgt.setMember(Reference43_50.convertReference(src.getMember())); 200 if (src.hasOnBehalfOf()) 201 tgt.setOnBehalfOf(Reference43_50.convertReference(src.getOnBehalfOf())); 202 if (src.hasCoveragePeriod()) 203 tgt.setPeriod(Period43_50.convertPeriod(src.getCoveragePeriod())); 204 return tgt; 205 } 206}