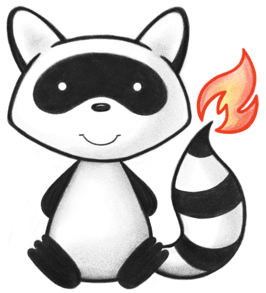
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Date43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 015import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 016import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 017import org.hl7.fhir.exceptions.FHIRException; 018 019/* 020 Copyright (c) 2011+, HL7, Inc. 021 All rights reserved. 022 023 Redistribution and use in source and binary forms, with or without modification, 024 are permitted provided that the following conditions are met: 025 026 * Redistributions of source code must retain the above copyright notice, this 027 list of conditions and the following disclaimer. 028 * Redistributions in binary form must reproduce the above copyright notice, 029 this list of conditions and the following disclaimer in the documentation 030 and/or other materials provided with the distribution. 031 * Neither the name of HL7 nor the names of its contributors may be used to 032 endorse or promote products derived from this software without specific 033 prior written permission. 034 035 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 036 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 037 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 038 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 039 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 040 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 041 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 042 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 043 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 044 POSSIBILITY OF SUCH DAMAGE. 045 046*/ 047// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 048public class ChargeItemDefinition43_50 { 049 050 public static org.hl7.fhir.r5.model.ChargeItemDefinition convertChargeItemDefinition(org.hl7.fhir.r4b.model.ChargeItemDefinition src) throws FHIRException { 051 if (src == null) 052 return null; 053 org.hl7.fhir.r5.model.ChargeItemDefinition tgt = new org.hl7.fhir.r5.model.ChargeItemDefinition(); 054 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 055 if (src.hasUrl()) 056 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 057 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 058 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 059 if (src.hasVersion()) 060 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 061 if (src.hasTitle()) 062 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 063 for (org.hl7.fhir.r4b.model.UriType t : src.getDerivedFromUri()) tgt.getDerivedFromUri().add(Uri43_50.convertUri(t)); 064 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getPartOf()) 065 tgt.getPartOf().add(Canonical43_50.convertCanonical(t)); 066 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getReplaces()) 067 tgt.getReplaces().add(Canonical43_50.convertCanonical(t)); 068 if (src.hasStatus()) 069 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 070 if (src.hasExperimental()) 071 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 072 if (src.hasDate()) 073 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 074 if (src.hasPublisher()) 075 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 076 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 077 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 078 if (src.hasDescription()) 079 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 080 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 081 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 082 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 083 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 084 if (src.hasCopyright()) 085 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 086 if (src.hasApprovalDate()) 087 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 088 if (src.hasLastReviewDate()) 089 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 090 if (src.hasEffectivePeriod()) 091 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 092 if (src.hasCode()) 093 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 094 for (org.hl7.fhir.r4b.model.Reference t : src.getInstance()) tgt.addInstance(Reference43_50.convertReference(t)); 095 for (org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent t : src.getApplicability()) 096 tgt.addApplicability(convertChargeItemDefinitionApplicabilityComponent(t)); 097 for (org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent t : src.getPropertyGroup()) 098 tgt.addPropertyGroup(convertChargeItemDefinitionPropertyGroupComponent(t)); 099 return tgt; 100 } 101 102 public static org.hl7.fhir.r4b.model.ChargeItemDefinition convertChargeItemDefinition(org.hl7.fhir.r5.model.ChargeItemDefinition src) throws FHIRException { 103 if (src == null) 104 return null; 105 org.hl7.fhir.r4b.model.ChargeItemDefinition tgt = new org.hl7.fhir.r4b.model.ChargeItemDefinition(); 106 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 107 if (src.hasUrl()) 108 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 109 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 110 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 111 if (src.hasVersion()) 112 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 113 if (src.hasTitle()) 114 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 115 for (org.hl7.fhir.r5.model.UriType t : src.getDerivedFromUri()) tgt.getDerivedFromUri().add(Uri43_50.convertUri(t)); 116 for (org.hl7.fhir.r5.model.CanonicalType t : src.getPartOf()) 117 tgt.getPartOf().add(Canonical43_50.convertCanonical(t)); 118 for (org.hl7.fhir.r5.model.CanonicalType t : src.getReplaces()) 119 tgt.getReplaces().add(Canonical43_50.convertCanonical(t)); 120 if (src.hasStatus()) 121 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 122 if (src.hasExperimental()) 123 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 124 if (src.hasDate()) 125 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 126 if (src.hasPublisher()) 127 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 128 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 129 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 130 if (src.hasDescription()) 131 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 132 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 133 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 134 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 135 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 136 if (src.hasCopyright()) 137 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 138 if (src.hasApprovalDate()) 139 tgt.setApprovalDateElement(Date43_50.convertDate(src.getApprovalDateElement())); 140 if (src.hasLastReviewDate()) 141 tgt.setLastReviewDateElement(Date43_50.convertDate(src.getLastReviewDateElement())); 142 if (src.hasEffectivePeriod()) 143 tgt.setEffectivePeriod(Period43_50.convertPeriod(src.getEffectivePeriod())); 144 if (src.hasCode()) 145 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 146 for (org.hl7.fhir.r5.model.Reference t : src.getInstance()) tgt.addInstance(Reference43_50.convertReference(t)); 147 for (org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent t : src.getApplicability()) 148 tgt.addApplicability(convertChargeItemDefinitionApplicabilityComponent(t)); 149 for (org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent t : src.getPropertyGroup()) 150 tgt.addPropertyGroup(convertChargeItemDefinitionPropertyGroupComponent(t)); 151 return tgt; 152 } 153 154 public static org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent convertChargeItemDefinitionApplicabilityComponent(org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent src) throws FHIRException { 155 if (src == null) 156 return null; 157 org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent tgt = new org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent(); 158 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 159 if (src.hasDescription()) 160 tgt.getCondition().setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 161 if (src.hasLanguage()) 162 tgt.getCondition().setLanguage(src.getLanguage()); 163 if (src.hasExpression()) 164 tgt.getCondition().setExpressionElement(String43_50.convertString(src.getExpressionElement())); 165 return tgt; 166 } 167 168 public static org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent convertChargeItemDefinitionApplicabilityComponent(org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent src) throws FHIRException { 169 if (src == null) 170 return null; 171 org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent tgt = new org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent(); 172 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 173 if (src.getCondition().hasDescription()) 174 tgt.setDescriptionElement(String43_50.convertString(src.getCondition().getDescriptionElement())); 175 if (src.getCondition().hasLanguage()) 176 tgt.setLanguageElement(String43_50.convertString(src.getCondition().getLanguageElement())); 177 if (src.getCondition().hasExpression()) 178 tgt.setExpressionElement(String43_50.convertString(src.getCondition().getExpressionElement())); 179 return tgt; 180 } 181 182 public static org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent convertChargeItemDefinitionPropertyGroupComponent(org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent src) throws FHIRException { 183 if (src == null) 184 return null; 185 org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent tgt = new org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent(); 186 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 187 for (org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent t : src.getApplicability()) 188 tgt.addApplicability(convertChargeItemDefinitionApplicabilityComponent(t)); 189// for (org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent t : src.getPriceComponent()) 190// tgt.addPriceComponent(convertChargeItemDefinitionPropertyGroupPriceComponentComponent(t)); 191 return tgt; 192 } 193 194 public static org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent convertChargeItemDefinitionPropertyGroupComponent(org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent src) throws FHIRException { 195 if (src == null) 196 return null; 197 org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent tgt = new org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupComponent(); 198 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 199 for (org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionApplicabilityComponent t : src.getApplicability()) 200 tgt.addApplicability(convertChargeItemDefinitionApplicabilityComponent(t)); 201// for (org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent t : src.getPriceComponent()) 202// tgt.addPriceComponent(convertChargeItemDefinitionPropertyGroupPriceComponentComponent(t)); 203 return tgt; 204 } 205 206// public static org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent convertChargeItemDefinitionPropertyGroupPriceComponentComponent(org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent src) throws FHIRException { 207// if (src == null) 208// return null; 209// org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent tgt = new org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent(); 210// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 211// if (src.hasType()) 212// tgt.setTypeElement(convertChargeItemDefinitionPriceComponentType(src.getTypeElement())); 213// if (src.hasCode()) 214// tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 215// if (src.hasFactor()) 216// tgt.setFactorElement(Decimal43_50.convertDecimal(src.getFactorElement())); 217// if (src.hasAmount()) 218// tgt.setAmount(Money43_50.convertMoney(src.getAmount())); 219// return tgt; 220// } 221// 222// public static org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent convertChargeItemDefinitionPropertyGroupPriceComponentComponent(org.hl7.fhir.r5.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent src) throws FHIRException { 223// if (src == null) 224// return null; 225// org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent tgt = new org.hl7.fhir.r4b.model.ChargeItemDefinition.ChargeItemDefinitionPropertyGroupPriceComponentComponent(); 226// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 227// if (src.hasType()) 228// tgt.setTypeElement(convertChargeItemDefinitionPriceComponentType(src.getTypeElement())); 229// if (src.hasCode()) 230// tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 231// if (src.hasFactor()) 232// tgt.setFactorElement(Decimal43_50.convertDecimal(src.getFactorElement())); 233// if (src.hasAmount()) 234// tgt.setAmount(Money43_50.convertMoney(src.getAmount())); 235// return tgt; 236// } 237// 238// static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType> convertChargeItemDefinitionPriceComponentType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType> src) throws FHIRException { 239// if (src == null || src.isEmpty()) 240// return null; 241// org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentTypeEnumFactory()); 242// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 243// switch (src.getValue()) { 244// case BASE: 245// tgt.setValue(org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType.BASE); 246// break; 247// case SURCHARGE: 248// tgt.setValue(org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType.SURCHARGE); 249// break; 250// case DEDUCTION: 251// tgt.setValue(org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType.DEDUCTION); 252// break; 253// case DISCOUNT: 254// tgt.setValue(org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType.DISCOUNT); 255// break; 256// case TAX: 257// tgt.setValue(org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType.TAX); 258// break; 259// case INFORMATIONAL: 260// tgt.setValue(org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType.INFORMATIONAL); 261// break; 262// default: 263// tgt.setValue(org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType.NULL); 264// break; 265// } 266// return tgt; 267// } 268// 269// static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType> convertChargeItemDefinitionPriceComponentType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.InvoicePriceComponentType> src) throws FHIRException { 270// if (src == null || src.isEmpty()) 271// return null; 272// org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentTypeEnumFactory()); 273// ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 274// switch (src.getValue()) { 275// case BASE: 276// tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType.BASE); 277// break; 278// case SURCHARGE: 279// tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType.SURCHARGE); 280// break; 281// case DEDUCTION: 282// tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType.DEDUCTION); 283// break; 284// case DISCOUNT: 285// tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType.DISCOUNT); 286// break; 287// case TAX: 288// tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType.TAX); 289// break; 290// case INFORMATIONAL: 291// tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType.INFORMATIONAL); 292// break; 293// default: 294// tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.InvoicePriceComponentType.NULL); 295// break; 296// } 297// return tgt; 298// } 299}