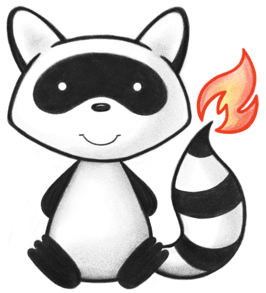
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r4b.model.ClinicalImpression; 013import org.hl7.fhir.r5.model.Enumeration; 014import org.hl7.fhir.r5.model.Enumerations; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class ClinicalImpression43_50 { 046 047 public static org.hl7.fhir.r5.model.ClinicalImpression convertClinicalImpression(org.hl7.fhir.r4b.model.ClinicalImpression src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.ClinicalImpression tgt = new org.hl7.fhir.r5.model.ClinicalImpression(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 054 if (src.hasStatus()) 055 tgt.setStatusElement(convertClinicalImpressionStatus(src.getStatusElement())); 056 if (src.hasStatusReason()) 057 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 058 // if (src.hasCode()) 059 // tgt.setCode(convertCodeableConcept(src.getCode())); 060 if (src.hasDescription()) 061 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 062 if (src.hasSubject()) 063 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 064 if (src.hasEncounter()) 065 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 066 if (src.hasEffective()) 067 tgt.setEffective(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getEffective())); 068 if (src.hasDate()) 069 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 070 if (src.hasAssessor()) 071 tgt.setPerformer(Reference43_50.convertReference(src.getAssessor())); 072 if (src.hasPrevious()) 073 tgt.setPrevious(Reference43_50.convertReference(src.getPrevious())); 074 for (org.hl7.fhir.r4b.model.Reference t : src.getProblem()) tgt.addProblem(Reference43_50.convertReference(t)); 075 for (org.hl7.fhir.r4b.model.UriType t : src.getProtocol()) tgt.getProtocol().add(Uri43_50.convertUri(t)); 076 if (src.hasSummary()) 077 tgt.setSummaryElement(String43_50.convertString(src.getSummaryElement())); 078 for (org.hl7.fhir.r4b.model.ClinicalImpression.ClinicalImpressionFindingComponent t : src.getFinding()) 079 tgt.addFinding(convertClinicalImpressionFindingComponent(t)); 080 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getPrognosisCodeableConcept()) 081 tgt.addPrognosisCodeableConcept(CodeableConcept43_50.convertCodeableConcept(t)); 082 for (org.hl7.fhir.r4b.model.Reference t : src.getPrognosisReference()) 083 tgt.addPrognosisReference(Reference43_50.convertReference(t)); 084 for (org.hl7.fhir.r4b.model.Reference t : src.getSupportingInfo()) 085 tgt.addSupportingInfo(Reference43_50.convertReference(t)); 086 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 087 return tgt; 088 } 089 090 public static org.hl7.fhir.r4b.model.ClinicalImpression convertClinicalImpression(org.hl7.fhir.r5.model.ClinicalImpression src) throws FHIRException { 091 if (src == null) 092 return null; 093 org.hl7.fhir.r4b.model.ClinicalImpression tgt = new org.hl7.fhir.r4b.model.ClinicalImpression(); 094 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 095 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 096 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 097 if (src.hasStatus()) 098 tgt.setStatusElement(convertClinicalImpressionStatus(src.getStatusElement())); 099 if (src.hasStatusReason()) 100 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 101 // if (src.hasCode()) 102 // tgt.setCode(convertCodeableConcept(src.getCode())); 103 if (src.hasDescription()) 104 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 105 if (src.hasSubject()) 106 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 107 if (src.hasEncounter()) 108 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 109 if (src.hasEffective()) 110 tgt.setEffective(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getEffective())); 111 if (src.hasDate()) 112 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 113 if (src.hasPerformer()) 114 tgt.setAssessor(Reference43_50.convertReference(src.getPerformer())); 115 if (src.hasPrevious()) 116 tgt.setPrevious(Reference43_50.convertReference(src.getPrevious())); 117 for (org.hl7.fhir.r5.model.Reference t : src.getProblem()) tgt.addProblem(Reference43_50.convertReference(t)); 118 for (org.hl7.fhir.r5.model.UriType t : src.getProtocol()) tgt.getProtocol().add(Uri43_50.convertUri(t)); 119 if (src.hasSummary()) 120 tgt.setSummaryElement(String43_50.convertString(src.getSummaryElement())); 121 for (org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent t : src.getFinding()) 122 tgt.addFinding(convertClinicalImpressionFindingComponent(t)); 123 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getPrognosisCodeableConcept()) 124 tgt.addPrognosisCodeableConcept(CodeableConcept43_50.convertCodeableConcept(t)); 125 for (org.hl7.fhir.r5.model.Reference t : src.getPrognosisReference()) 126 tgt.addPrognosisReference(Reference43_50.convertReference(t)); 127 for (org.hl7.fhir.r5.model.Reference t : src.getSupportingInfo()) 128 tgt.addSupportingInfo(Reference43_50.convertReference(t)); 129 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 130 return tgt; 131 } 132 133 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> convertClinicalImpressionStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.ClinicalImpression.ClinicalImpressionStatus> src) throws FHIRException { 134 if (src == null || src.isEmpty()) 135 return null; 136 Enumeration<Enumerations.EventStatus> tgt = new Enumeration<>(new Enumerations.EventStatusEnumFactory()); 137 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 138 if (src.getValue() == null) { 139 tgt.setValue(null); 140 } else { 141 switch (src.getValue()) { 142 case INPROGRESS: 143 tgt.setValue(Enumerations.EventStatus.INPROGRESS); 144 break; 145 case COMPLETED: 146 tgt.setValue(Enumerations.EventStatus.COMPLETED); 147 break; 148 case ENTEREDINERROR: 149 tgt.setValue(Enumerations.EventStatus.ENTEREDINERROR); 150 break; 151 default: 152 tgt.setValue(Enumerations.EventStatus.NULL); 153 break; 154 } 155 } 156 return tgt; 157 } 158 159 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.ClinicalImpression.ClinicalImpressionStatus> convertClinicalImpressionStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> src) throws FHIRException { 160 if (src == null || src.isEmpty()) 161 return null; 162 org.hl7.fhir.r4b.model.Enumeration<ClinicalImpression.ClinicalImpressionStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new ClinicalImpression.ClinicalImpressionStatusEnumFactory()); 163 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 164 if (src.getValue() == null) { 165 tgt.setValue(null); 166 } else { 167 switch (src.getValue()) { 168 case INPROGRESS: 169 tgt.setValue(ClinicalImpression.ClinicalImpressionStatus.INPROGRESS); 170 break; 171 case COMPLETED: 172 tgt.setValue(ClinicalImpression.ClinicalImpressionStatus.COMPLETED); 173 break; 174 case ENTEREDINERROR: 175 tgt.setValue(ClinicalImpression.ClinicalImpressionStatus.ENTEREDINERROR); 176 break; 177 default: 178 tgt.setValue(ClinicalImpression.ClinicalImpressionStatus.NULL); 179 break; 180 } 181 } 182 return tgt; 183 } 184 185 public static org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent convertClinicalImpressionFindingComponent(org.hl7.fhir.r4b.model.ClinicalImpression.ClinicalImpressionFindingComponent src) throws FHIRException { 186 if (src == null) 187 return null; 188 org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent tgt = new org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent(); 189 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 190 if (src.hasItemCodeableConcept()) 191 tgt.setItem(CodeableConcept43_50.convertCodeableConceptToCodeableReference(src.getItemCodeableConcept())); 192 if (src.hasItemReference()) 193 tgt.setItem(Reference43_50.convertReferenceToCodeableReference(src.getItemReference())); 194 if (src.hasBasis()) 195 tgt.setBasisElement(String43_50.convertString(src.getBasisElement())); 196 return tgt; 197 } 198 199 public static org.hl7.fhir.r4b.model.ClinicalImpression.ClinicalImpressionFindingComponent convertClinicalImpressionFindingComponent(org.hl7.fhir.r5.model.ClinicalImpression.ClinicalImpressionFindingComponent src) throws FHIRException { 200 if (src == null) 201 return null; 202 org.hl7.fhir.r4b.model.ClinicalImpression.ClinicalImpressionFindingComponent tgt = new org.hl7.fhir.r4b.model.ClinicalImpression.ClinicalImpressionFindingComponent(); 203 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 204 if (src.hasItem() && src.getItem().hasConcept()) 205 tgt.setItemCodeableConcept(CodeableConcept43_50.convertCodeableConcept(src.getItem().getConcept())); 206 if (src.hasItem() && src.getItem().hasReference()) 207 tgt.setItemReference(Reference43_50.convertReference(src.getItem().getReference())); 208 if (src.hasBasis()) 209 tgt.setBasisElement(String43_50.convertString(src.getBasisElement())); 210 return tgt; 211 } 212}