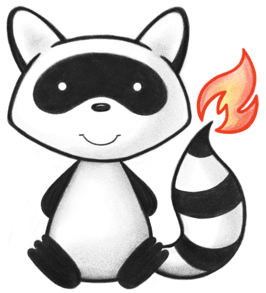
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import java.util.stream.Collectors; 004 005import org.hl7.fhir.convertors.context.ConversionContext43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Coding43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 013import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Code43_50; 014import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 015import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 016import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 017import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.UnsignedInt43_50; 018import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 019import org.hl7.fhir.exceptions.FHIRException; 020import org.hl7.fhir.r5.model.CodeSystem; 021import org.hl7.fhir.r5.model.Enumeration; 022import org.hl7.fhir.r5.model.Enumerations; 023 024/* 025 Copyright (c) 2011+, HL7, Inc. 026 All rights reserved. 027 028 Redistribution and use in source and binary forms, with or without modification, 029 are permitted provided that the following conditions are met: 030 031 * Redistributions of source code must retain the above copyright notice, this 032 list of conditions and the following disclaimer. 033 * Redistributions in binary form must reproduce the above copyright notice, 034 this list of conditions and the following disclaimer in the documentation 035 and/or other materials provided with the distribution. 036 * Neither the name of HL7 nor the names of its contributors may be used to 037 endorse or promote products derived from this software without specific 038 prior written permission. 039 040 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 041 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 042 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 043 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 044 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 045 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 046 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 047 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 048 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 049 POSSIBILITY OF SUCH DAMAGE. 050 051*/ 052// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 053public class CodeSystem43_50 { 054 055 public static org.hl7.fhir.r5.model.CodeSystem convertCodeSystem(org.hl7.fhir.r4b.model.CodeSystem src) throws FHIRException { 056 if (src == null) 057 return null; 058 org.hl7.fhir.r5.model.CodeSystem tgt = new org.hl7.fhir.r5.model.CodeSystem(); 059 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 060 if (src.hasUrl()) 061 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 062 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 063 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 064 if (src.hasVersion()) 065 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 066 if (src.hasName()) 067 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 068 if (src.hasTitle()) 069 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 070 if (src.hasStatus()) 071 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 072 if (src.hasExperimental()) 073 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 074 if (src.hasDate()) 075 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 076 if (src.hasPublisher()) 077 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 078 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 079 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 080 if (src.hasDescription()) 081 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 082 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 083 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 084 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getJurisdiction()) 085 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 086 if (src.hasPurpose()) 087 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 088 if (src.hasCopyright()) 089 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 090 if (src.hasCaseSensitive()) 091 tgt.setCaseSensitiveElement(Boolean43_50.convertBoolean(src.getCaseSensitiveElement())); 092 if (src.hasValueSet()) 093 tgt.setValueSetElement(Canonical43_50.convertCanonical(src.getValueSetElement())); 094 if (src.hasHierarchyMeaning()) 095 tgt.setHierarchyMeaningElement(convertCodeSystemHierarchyMeaning(src.getHierarchyMeaningElement())); 096 if (src.hasCompositional()) 097 tgt.setCompositionalElement(Boolean43_50.convertBoolean(src.getCompositionalElement())); 098 if (src.hasVersionNeeded()) 099 tgt.setVersionNeededElement(Boolean43_50.convertBoolean(src.getVersionNeededElement())); 100 if (src.hasContent()) 101 tgt.setContentElement(convertCodeSystemContentMode(src.getContentElement())); 102 if (src.hasSupplements()) 103 tgt.setSupplementsElement(Canonical43_50.convertCanonical(src.getSupplementsElement())); 104 if (src.hasCount()) 105 tgt.setCountElement(UnsignedInt43_50.convertUnsignedInt(src.getCountElement())); 106 for (org.hl7.fhir.r4b.model.CodeSystem.CodeSystemFilterComponent t : src.getFilter()) 107 tgt.addFilter(convertCodeSystemFilterComponent(t)); 108 for (org.hl7.fhir.r4b.model.CodeSystem.PropertyComponent t : src.getProperty()) 109 tgt.addProperty(convertPropertyComponent(t)); 110 for (org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionComponent t : src.getConcept()) 111 tgt.addConcept(convertConceptDefinitionComponent(t)); 112 return tgt; 113 } 114 115 public static org.hl7.fhir.r4b.model.CodeSystem convertCodeSystem(org.hl7.fhir.r5.model.CodeSystem src) throws FHIRException { 116 if (src == null) 117 return null; 118 org.hl7.fhir.r4b.model.CodeSystem tgt = new org.hl7.fhir.r4b.model.CodeSystem(); 119 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 120 if (src.hasUrl()) 121 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 122 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 123 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 124 if (src.hasVersion()) 125 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 126 if (src.hasName()) 127 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 128 if (src.hasTitle()) 129 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 130 if (src.hasStatus()) 131 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 132 if (src.hasExperimental()) 133 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 134 if (src.hasDate()) 135 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 136 if (src.hasPublisher()) 137 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 138 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 139 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 140 if (src.hasDescription()) 141 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 142 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 143 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 144 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getJurisdiction()) 145 tgt.addJurisdiction(CodeableConcept43_50.convertCodeableConcept(t)); 146 if (src.hasPurpose()) 147 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 148 if (src.hasCopyright()) 149 tgt.setCopyrightElement(MarkDown43_50.convertMarkdown(src.getCopyrightElement())); 150 if (src.hasCaseSensitive()) 151 tgt.setCaseSensitiveElement(Boolean43_50.convertBoolean(src.getCaseSensitiveElement())); 152 if (src.hasValueSet()) 153 tgt.setValueSetElement(Canonical43_50.convertCanonical(src.getValueSetElement())); 154 if (src.hasHierarchyMeaning()) 155 tgt.setHierarchyMeaningElement(convertCodeSystemHierarchyMeaning(src.getHierarchyMeaningElement())); 156 if (src.hasCompositional()) 157 tgt.setCompositionalElement(Boolean43_50.convertBoolean(src.getCompositionalElement())); 158 if (src.hasVersionNeeded()) 159 tgt.setVersionNeededElement(Boolean43_50.convertBoolean(src.getVersionNeededElement())); 160 if (src.hasContent()) 161 tgt.setContentElement(convertCodeSystemContentMode(src.getContentElement())); 162 if (src.hasSupplements()) 163 tgt.setSupplementsElement(Canonical43_50.convertCanonical(src.getSupplementsElement())); 164 if (src.hasCount()) 165 tgt.setCountElement(UnsignedInt43_50.convertUnsignedInt(src.getCountElement())); 166 for (org.hl7.fhir.r5.model.CodeSystem.CodeSystemFilterComponent t : src.getFilter()) 167 tgt.addFilter(convertCodeSystemFilterComponent(t)); 168 for (org.hl7.fhir.r5.model.CodeSystem.PropertyComponent t : src.getProperty()) 169 tgt.addProperty(convertPropertyComponent(t)); 170 for (org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent t : src.getConcept()) 171 tgt.addConcept(convertConceptDefinitionComponent(t)); 172 return tgt; 173 } 174 175 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CodeSystem.CodeSystemHierarchyMeaning> convertCodeSystemHierarchyMeaning(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaning> src) throws FHIRException { 176 if (src == null || src.isEmpty()) 177 return null; 178 Enumeration<CodeSystem.CodeSystemHierarchyMeaning> tgt = new Enumeration<>(new CodeSystem.CodeSystemHierarchyMeaningEnumFactory()); 179 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 180 if (src.getValue() == null) { 181 tgt.setValue(null); 182 } else { 183 switch (src.getValue()) { 184 case GROUPEDBY: 185 tgt.setValue(CodeSystem.CodeSystemHierarchyMeaning.GROUPEDBY); 186 break; 187 case ISA: 188 tgt.setValue(CodeSystem.CodeSystemHierarchyMeaning.ISA); 189 break; 190 case PARTOF: 191 tgt.setValue(CodeSystem.CodeSystemHierarchyMeaning.PARTOF); 192 break; 193 case CLASSIFIEDWITH: 194 tgt.setValue(CodeSystem.CodeSystemHierarchyMeaning.CLASSIFIEDWITH); 195 break; 196 default: 197 tgt.setValue(CodeSystem.CodeSystemHierarchyMeaning.NULL); 198 break; 199 } 200 } 201 return tgt; 202 } 203 204 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaning> convertCodeSystemHierarchyMeaning(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CodeSystem.CodeSystemHierarchyMeaning> src) throws FHIRException { 205 if (src == null || src.isEmpty()) 206 return null; 207 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaning> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaningEnumFactory()); 208 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 209 if (src.getValue() == null) { 210 tgt.setValue(null); 211 } else { 212 switch (src.getValue()) { 213 case GROUPEDBY: 214 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaning.GROUPEDBY); 215 break; 216 case ISA: 217 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaning.ISA); 218 break; 219 case PARTOF: 220 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaning.PARTOF); 221 break; 222 case CLASSIFIEDWITH: 223 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaning.CLASSIFIEDWITH); 224 break; 225 default: 226 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemHierarchyMeaning.NULL); 227 break; 228 } 229 } 230 return tgt; 231 } 232 233 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CodeSystemContentMode> convertCodeSystemContentMode(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode> src) throws FHIRException { 234 if (src == null || src.isEmpty()) 235 return null; 236 Enumeration<Enumerations.CodeSystemContentMode> tgt = new Enumeration<>(new Enumerations.CodeSystemContentModeEnumFactory()); 237 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 238 if (src.getValue() == null) { 239 tgt.setValue(null); 240 } else { 241 switch (src.getValue()) { 242 case NOTPRESENT: 243 tgt.setValue(Enumerations.CodeSystemContentMode.NOTPRESENT); 244 break; 245 case EXAMPLE: 246 tgt.setValue(Enumerations.CodeSystemContentMode.EXAMPLE); 247 break; 248 case FRAGMENT: 249 tgt.setValue(Enumerations.CodeSystemContentMode.FRAGMENT); 250 break; 251 case COMPLETE: 252 tgt.setValue(Enumerations.CodeSystemContentMode.COMPLETE); 253 break; 254 case SUPPLEMENT: 255 tgt.setValue(Enumerations.CodeSystemContentMode.SUPPLEMENT); 256 break; 257 default: 258 tgt.setValue(Enumerations.CodeSystemContentMode.NULL); 259 break; 260 } 261 } 262 return tgt; 263 } 264 265 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode> convertCodeSystemContentMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CodeSystemContentMode> src) throws FHIRException { 266 if (src == null || src.isEmpty()) 267 return null; 268 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentModeEnumFactory()); 269 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 270 if (src.getValue() == null) { 271 tgt.setValue(null); 272 } else { 273 switch (src.getValue()) { 274 case NOTPRESENT: 275 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode.NOTPRESENT); 276 break; 277 case EXAMPLE: 278 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode.EXAMPLE); 279 break; 280 case FRAGMENT: 281 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode.FRAGMENT); 282 break; 283 case COMPLETE: 284 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode.COMPLETE); 285 break; 286 case SUPPLEMENT: 287 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode.SUPPLEMENT); 288 break; 289 default: 290 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemContentMode.NULL); 291 break; 292 } 293 } 294 return tgt; 295 } 296 297 public static org.hl7.fhir.r5.model.CodeSystem.CodeSystemFilterComponent convertCodeSystemFilterComponent(org.hl7.fhir.r4b.model.CodeSystem.CodeSystemFilterComponent src) throws FHIRException { 298 if (src == null) 299 return null; 300 org.hl7.fhir.r5.model.CodeSystem.CodeSystemFilterComponent tgt = new org.hl7.fhir.r5.model.CodeSystem.CodeSystemFilterComponent(); 301 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 302 if (src.hasCode()) 303 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 304 if (src.hasDescription()) 305 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 306 tgt.setOperator(src.getOperator().stream() 307 .map(CodeSystem43_50::convertFilterOperator) 308 .collect(Collectors.toList())); 309 if (src.hasValue()) 310 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 311 return tgt; 312 } 313 314 public static org.hl7.fhir.r4b.model.CodeSystem.CodeSystemFilterComponent convertCodeSystemFilterComponent(org.hl7.fhir.r5.model.CodeSystem.CodeSystemFilterComponent src) throws FHIRException { 315 if (src == null) 316 return null; 317 org.hl7.fhir.r4b.model.CodeSystem.CodeSystemFilterComponent tgt = new org.hl7.fhir.r4b.model.CodeSystem.CodeSystemFilterComponent(); 318 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 319 if (src.hasCode()) 320 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 321 if (src.hasDescription()) 322 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 323 tgt.setOperator(src.getOperator().stream() 324 .map(CodeSystem43_50::convertFilterOperator) 325 .collect(Collectors.toList())); 326 if (src.hasValue()) 327 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 328 return tgt; 329 } 330 331 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> convertFilterOperator(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FilterOperator> src) throws FHIRException { 332 if (src == null || src.isEmpty()) 333 return null; 334 Enumeration<Enumerations.FilterOperator> tgt = new Enumeration<>(new Enumerations.FilterOperatorEnumFactory()); 335 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 336 if (src.getValue() == null) { 337 tgt.setValue(null); 338 } else { 339 switch (src.getValue()) { 340 case EQUAL: 341 tgt.setValue(Enumerations.FilterOperator.EQUAL); 342 break; 343 case ISA: 344 tgt.setValue(Enumerations.FilterOperator.ISA); 345 break; 346 case DESCENDENTOF: 347 tgt.setValue(Enumerations.FilterOperator.DESCENDENTOF); 348 break; 349 case ISNOTA: 350 tgt.setValue(Enumerations.FilterOperator.ISNOTA); 351 break; 352 case REGEX: 353 tgt.setValue(Enumerations.FilterOperator.REGEX); 354 break; 355 case IN: 356 tgt.setValue(Enumerations.FilterOperator.IN); 357 break; 358 case NOTIN: 359 tgt.setValue(Enumerations.FilterOperator.NOTIN); 360 break; 361 case GENERALIZES: 362 tgt.setValue(Enumerations.FilterOperator.GENERALIZES); 363 break; 364 case EXISTS: 365 tgt.setValue(Enumerations.FilterOperator.EXISTS); 366 break; 367 default: 368 tgt.setValue(Enumerations.FilterOperator.NULL); 369 break; 370 } 371 } 372 return tgt; 373 } 374 375 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FilterOperator> convertFilterOperator(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FilterOperator> src) throws FHIRException { 376 if (src == null || src.isEmpty()) 377 return null; 378 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FilterOperator> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.FilterOperatorEnumFactory()); 379 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 380 if (src.getValue() == null) { 381 tgt.setValue(null); 382 } else { 383 switch (src.getValue()) { 384 case EQUAL: 385 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.EQUAL); 386 break; 387 case ISA: 388 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.ISA); 389 break; 390 case DESCENDENTOF: 391 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.DESCENDENTOF); 392 break; 393 case ISNOTA: 394 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.ISNOTA); 395 break; 396 case REGEX: 397 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.REGEX); 398 break; 399 case IN: 400 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.IN); 401 break; 402 case NOTIN: 403 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.NOTIN); 404 break; 405 case GENERALIZES: 406 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.GENERALIZES); 407 break; 408 case EXISTS: 409 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.EXISTS); 410 break; 411 default: 412 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FilterOperator.NULL); 413 break; 414 } 415 } 416 return tgt; 417 } 418 419 public static org.hl7.fhir.r5.model.CodeSystem.PropertyComponent convertPropertyComponent(org.hl7.fhir.r4b.model.CodeSystem.PropertyComponent src) throws FHIRException { 420 if (src == null) 421 return null; 422 org.hl7.fhir.r5.model.CodeSystem.PropertyComponent tgt = new org.hl7.fhir.r5.model.CodeSystem.PropertyComponent(); 423 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 424 if (src.hasCode()) 425 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 426 if (src.hasUri()) 427 tgt.setUriElement(Uri43_50.convertUri(src.getUriElement())); 428 if (src.hasDescription()) 429 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 430 if (src.hasType()) 431 tgt.setTypeElement(convertPropertyType(src.getTypeElement())); 432 return tgt; 433 } 434 435 public static org.hl7.fhir.r4b.model.CodeSystem.PropertyComponent convertPropertyComponent(org.hl7.fhir.r5.model.CodeSystem.PropertyComponent src) throws FHIRException { 436 if (src == null) 437 return null; 438 org.hl7.fhir.r4b.model.CodeSystem.PropertyComponent tgt = new org.hl7.fhir.r4b.model.CodeSystem.PropertyComponent(); 439 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 440 if (src.hasCode()) 441 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 442 if (src.hasUri()) 443 tgt.setUriElement(Uri43_50.convertUri(src.getUriElement())); 444 if (src.hasDescription()) 445 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 446 if (src.hasType()) 447 tgt.setTypeElement(convertPropertyType(src.getTypeElement())); 448 return tgt; 449 } 450 451 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CodeSystem.PropertyType> convertPropertyType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.PropertyType> src) throws FHIRException { 452 if (src == null || src.isEmpty()) 453 return null; 454 Enumeration<CodeSystem.PropertyType> tgt = new Enumeration<>(new CodeSystem.PropertyTypeEnumFactory()); 455 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 456 if (src.getValue() == null) { 457 tgt.setValue(null); 458 } else { 459 switch (src.getValue()) { 460 case CODE: 461 tgt.setValue(CodeSystem.PropertyType.CODE); 462 break; 463 case CODING: 464 tgt.setValue(CodeSystem.PropertyType.CODING); 465 break; 466 case STRING: 467 tgt.setValue(CodeSystem.PropertyType.STRING); 468 break; 469 case INTEGER: 470 tgt.setValue(CodeSystem.PropertyType.INTEGER); 471 break; 472 case BOOLEAN: 473 tgt.setValue(CodeSystem.PropertyType.BOOLEAN); 474 break; 475 case DATETIME: 476 tgt.setValue(CodeSystem.PropertyType.DATETIME); 477 break; 478 case DECIMAL: 479 tgt.setValue(CodeSystem.PropertyType.DECIMAL); 480 break; 481 default: 482 tgt.setValue(CodeSystem.PropertyType.NULL); 483 break; 484 } 485 } 486 return tgt; 487 } 488 489 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.PropertyType> convertPropertyType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.CodeSystem.PropertyType> src) throws FHIRException { 490 if (src == null || src.isEmpty()) 491 return null; 492 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.CodeSystem.PropertyType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.CodeSystem.PropertyTypeEnumFactory()); 493 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 494 if (src.getValue() == null) { 495 tgt.setValue(null); 496 } else { 497 switch (src.getValue()) { 498 case CODE: 499 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.PropertyType.CODE); 500 break; 501 case CODING: 502 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.PropertyType.CODING); 503 break; 504 case STRING: 505 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.PropertyType.STRING); 506 break; 507 case INTEGER: 508 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.PropertyType.INTEGER); 509 break; 510 case BOOLEAN: 511 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.PropertyType.BOOLEAN); 512 break; 513 case DATETIME: 514 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.PropertyType.DATETIME); 515 break; 516 case DECIMAL: 517 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.PropertyType.DECIMAL); 518 break; 519 default: 520 tgt.setValue(org.hl7.fhir.r4b.model.CodeSystem.PropertyType.NULL); 521 break; 522 } 523 } 524 return tgt; 525 } 526 527 public static org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent convertConceptDefinitionComponent(org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionComponent src) throws FHIRException { 528 if (src == null) 529 return null; 530 org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent tgt = new org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent(); 531 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 532 if (src.hasCode()) 533 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 534 if (src.hasDisplay()) 535 tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 536 if (src.hasDefinition()) 537 tgt.setDefinitionElement(String43_50.convertString(src.getDefinitionElement())); 538 for (org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionDesignationComponent t : src.getDesignation()) 539 tgt.addDesignation(convertConceptDefinitionDesignationComponent(t)); 540 for (org.hl7.fhir.r4b.model.CodeSystem.ConceptPropertyComponent t : src.getProperty()) 541 tgt.addProperty(convertConceptPropertyComponent(t)); 542 for (org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionComponent t : src.getConcept()) 543 tgt.addConcept(convertConceptDefinitionComponent(t)); 544 return tgt; 545 } 546 547 public static org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionComponent convertConceptDefinitionComponent(org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent src) throws FHIRException { 548 if (src == null) 549 return null; 550 org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionComponent tgt = new org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionComponent(); 551 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 552 if (src.hasCode()) 553 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 554 if (src.hasDisplay()) 555 tgt.setDisplayElement(String43_50.convertString(src.getDisplayElement())); 556 if (src.hasDefinition()) 557 tgt.setDefinitionElement(String43_50.convertString(src.getDefinitionElement())); 558 for (org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent t : src.getDesignation()) 559 tgt.addDesignation(convertConceptDefinitionDesignationComponent(t)); 560 for (org.hl7.fhir.r5.model.CodeSystem.ConceptPropertyComponent t : src.getProperty()) 561 tgt.addProperty(convertConceptPropertyComponent(t)); 562 for (org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionComponent t : src.getConcept()) 563 tgt.addConcept(convertConceptDefinitionComponent(t)); 564 return tgt; 565 } 566 567 public static org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent convertConceptDefinitionDesignationComponent(org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionDesignationComponent src) throws FHIRException { 568 if (src == null) 569 return null; 570 org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent tgt = new org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent(); 571 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 572 if (src.hasLanguage()) 573 tgt.setLanguageElement(Code43_50.convertCode(src.getLanguageElement())); 574 if (src.hasUse()) 575 tgt.setUse(Coding43_50.convertCoding(src.getUse())); 576 if (src.hasValue()) 577 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 578 return tgt; 579 } 580 581 public static org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionDesignationComponent convertConceptDefinitionDesignationComponent(org.hl7.fhir.r5.model.CodeSystem.ConceptDefinitionDesignationComponent src) throws FHIRException { 582 if (src == null) 583 return null; 584 org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionDesignationComponent tgt = new org.hl7.fhir.r4b.model.CodeSystem.ConceptDefinitionDesignationComponent(); 585 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 586 if (src.hasLanguage()) 587 tgt.setLanguageElement(Code43_50.convertCode(src.getLanguageElement())); 588 if (src.hasUse()) 589 tgt.setUse(Coding43_50.convertCoding(src.getUse())); 590 if (src.hasValue()) 591 tgt.setValueElement(String43_50.convertString(src.getValueElement())); 592 return tgt; 593 } 594 595 public static org.hl7.fhir.r5.model.CodeSystem.ConceptPropertyComponent convertConceptPropertyComponent(org.hl7.fhir.r4b.model.CodeSystem.ConceptPropertyComponent src) throws FHIRException { 596 if (src == null) 597 return null; 598 org.hl7.fhir.r5.model.CodeSystem.ConceptPropertyComponent tgt = new org.hl7.fhir.r5.model.CodeSystem.ConceptPropertyComponent(); 599 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 600 if (src.hasCode()) 601 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 602 if (src.hasValue()) 603 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 604 return tgt; 605 } 606 607 public static org.hl7.fhir.r4b.model.CodeSystem.ConceptPropertyComponent convertConceptPropertyComponent(org.hl7.fhir.r5.model.CodeSystem.ConceptPropertyComponent src) throws FHIRException { 608 if (src == null) 609 return null; 610 org.hl7.fhir.r4b.model.CodeSystem.ConceptPropertyComponent tgt = new org.hl7.fhir.r4b.model.CodeSystem.ConceptPropertyComponent(); 611 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 612 if (src.hasCode()) 613 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 614 if (src.hasValue()) 615 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 616 return tgt; 617 } 618}