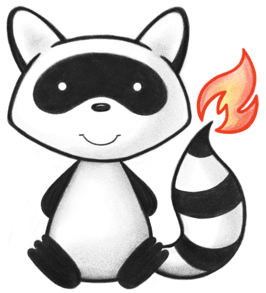
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Annotation43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Canonical43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r4b.model.StringType; 013import org.hl7.fhir.r5.model.CodeableConcept; 014import org.hl7.fhir.r5.model.CodeableReference; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class Communication43_50 { 046 047 public static org.hl7.fhir.r5.model.Communication convertCommunication(org.hl7.fhir.r4b.model.Communication src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.Communication tgt = new org.hl7.fhir.r5.model.Communication(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 052 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 053 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 054 for (org.hl7.fhir.r4b.model.CanonicalType t : src.getInstantiatesCanonical()) 055 tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 056 for (org.hl7.fhir.r4b.model.UriType t : src.getInstantiatesUri()) 057 tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 058 for (org.hl7.fhir.r4b.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 059 for (org.hl7.fhir.r4b.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference43_50.convertReference(t)); 060 for (org.hl7.fhir.r4b.model.Reference t : src.getInResponseTo()) 061 tgt.addInResponseTo(Reference43_50.convertReference(t)); 062 if (src.hasStatus()) 063 tgt.setStatusElement(convertCommunicationStatus(src.getStatusElement())); 064 if (src.hasStatusReason()) 065 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 066 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCategory()) 067 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 068 if (src.hasPriority()) 069 tgt.setPriorityElement(convertCommunicationPriority(src.getPriorityElement())); 070 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getMedium()) 071 tgt.addMedium(CodeableConcept43_50.convertCodeableConcept(t)); 072 if (src.hasSubject()) 073 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 074 if (src.hasTopic()) 075 tgt.setTopic(CodeableConcept43_50.convertCodeableConcept(src.getTopic())); 076 for (org.hl7.fhir.r4b.model.Reference t : src.getAbout()) tgt.addAbout(Reference43_50.convertReference(t)); 077 if (src.hasEncounter()) 078 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 079 if (src.hasSent()) 080 tgt.setSentElement(DateTime43_50.convertDateTime(src.getSentElement())); 081 if (src.hasReceived()) 082 tgt.setReceivedElement(DateTime43_50.convertDateTime(src.getReceivedElement())); 083 for (org.hl7.fhir.r4b.model.Reference t : src.getRecipient()) tgt.addRecipient(Reference43_50.convertReference(t)); 084 if (src.hasSender()) 085 tgt.setSender(Reference43_50.convertReference(src.getSender())); 086 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getReasonCode()) 087 tgt.addReason(CodeableConcept43_50.convertCodeableConceptToCodeableReference(t)); 088 for (org.hl7.fhir.r4b.model.Reference t : src.getReasonReference()) 089 tgt.addReason(Reference43_50.convertReferenceToCodeableReference(t)); 090 for (org.hl7.fhir.r4b.model.Communication.CommunicationPayloadComponent t : src.getPayload()) 091 tgt.addPayload(convertCommunicationPayloadComponent(t)); 092 for (org.hl7.fhir.r4b.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 093 return tgt; 094 } 095 096 public static org.hl7.fhir.r4b.model.Communication convertCommunication(org.hl7.fhir.r5.model.Communication src) throws FHIRException { 097 if (src == null) 098 return null; 099 org.hl7.fhir.r4b.model.Communication tgt = new org.hl7.fhir.r4b.model.Communication(); 100 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 101 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 102 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 103 for (org.hl7.fhir.r5.model.CanonicalType t : src.getInstantiatesCanonical()) 104 tgt.getInstantiatesCanonical().add(Canonical43_50.convertCanonical(t)); 105 for (org.hl7.fhir.r5.model.UriType t : src.getInstantiatesUri()) 106 tgt.getInstantiatesUri().add(Uri43_50.convertUri(t)); 107 for (org.hl7.fhir.r5.model.Reference t : src.getBasedOn()) tgt.addBasedOn(Reference43_50.convertReference(t)); 108 for (org.hl7.fhir.r5.model.Reference t : src.getPartOf()) tgt.addPartOf(Reference43_50.convertReference(t)); 109 for (org.hl7.fhir.r5.model.Reference t : src.getInResponseTo()) 110 tgt.addInResponseTo(Reference43_50.convertReference(t)); 111 if (src.hasStatus()) 112 tgt.setStatusElement(convertCommunicationStatus(src.getStatusElement())); 113 if (src.hasStatusReason()) 114 tgt.setStatusReason(CodeableConcept43_50.convertCodeableConcept(src.getStatusReason())); 115 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 116 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 117 if (src.hasPriority()) 118 tgt.setPriorityElement(convertCommunicationPriority(src.getPriorityElement())); 119 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getMedium()) 120 tgt.addMedium(CodeableConcept43_50.convertCodeableConcept(t)); 121 if (src.hasSubject()) 122 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 123 if (src.hasTopic()) 124 tgt.setTopic(CodeableConcept43_50.convertCodeableConcept(src.getTopic())); 125 for (org.hl7.fhir.r5.model.Reference t : src.getAbout()) tgt.addAbout(Reference43_50.convertReference(t)); 126 if (src.hasEncounter()) 127 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 128 if (src.hasSent()) 129 tgt.setSentElement(DateTime43_50.convertDateTime(src.getSentElement())); 130 if (src.hasReceived()) 131 tgt.setReceivedElement(DateTime43_50.convertDateTime(src.getReceivedElement())); 132 for (org.hl7.fhir.r5.model.Reference t : src.getRecipient()) tgt.addRecipient(Reference43_50.convertReference(t)); 133 if (src.hasSender()) 134 tgt.setSender(Reference43_50.convertReference(src.getSender())); 135 for (CodeableReference t : src.getReason()) 136 if (t.hasConcept()) 137 tgt.addReasonCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 138 for (CodeableReference t : src.getReason()) 139 if (t.hasReference()) 140 tgt.addReasonReference(Reference43_50.convertReference(t.getReference())); 141 for (org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent t : src.getPayload()) 142 tgt.addPayload(convertCommunicationPayloadComponent(t)); 143 for (org.hl7.fhir.r5.model.Annotation t : src.getNote()) tgt.addNote(Annotation43_50.convertAnnotation(t)); 144 return tgt; 145 } 146 147 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> convertCommunicationStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.EventStatus> src) throws FHIRException { 148 if (src == null || src.isEmpty()) 149 return null; 150 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.EventStatusEnumFactory()); 151 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 152 switch (src.getValue()) { 153 case PREPARATION: 154 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.PREPARATION); 155 break; 156 case INPROGRESS: 157 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.INPROGRESS); 158 break; 159 case NOTDONE: 160 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.NOTDONE); 161 break; 162 case ONHOLD: 163 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.ONHOLD); 164 break; 165 case STOPPED: 166 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.STOPPED); 167 break; 168 case COMPLETED: 169 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.COMPLETED); 170 break; 171 case ENTEREDINERROR: 172 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.ENTEREDINERROR); 173 break; 174 case UNKNOWN: 175 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.UNKNOWN); 176 break; 177 default: 178 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.EventStatus.NULL); 179 break; 180 } 181 return tgt; 182 } 183 184 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.EventStatus> convertCommunicationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.EventStatus> src) throws FHIRException { 185 if (src == null || src.isEmpty()) 186 return null; 187 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.EventStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.EventStatusEnumFactory()); 188 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 189 switch (src.getValue()) { 190 case PREPARATION: 191 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.PREPARATION); 192 break; 193 case INPROGRESS: 194 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.INPROGRESS); 195 break; 196 case NOTDONE: 197 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.NOTDONE); 198 break; 199 case ONHOLD: 200 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.ONHOLD); 201 break; 202 case STOPPED: 203 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.STOPPED); 204 break; 205 case COMPLETED: 206 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.COMPLETED); 207 break; 208 case ENTEREDINERROR: 209 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.ENTEREDINERROR); 210 break; 211 case UNKNOWN: 212 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.UNKNOWN); 213 break; 214 default: 215 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.EventStatus.NULL); 216 break; 217 } 218 return tgt; 219 } 220 221 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> convertCommunicationPriority(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> src) throws FHIRException { 222 if (src == null || src.isEmpty()) 223 return null; 224 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.RequestPriorityEnumFactory()); 225 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 226 switch (src.getValue()) { 227 case ROUTINE: 228 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.ROUTINE); 229 break; 230 case URGENT: 231 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.URGENT); 232 break; 233 case ASAP: 234 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.ASAP); 235 break; 236 case STAT: 237 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.STAT); 238 break; 239 default: 240 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.RequestPriority.NULL); 241 break; 242 } 243 return tgt; 244 } 245 246 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> convertCommunicationPriority(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.RequestPriority> src) throws FHIRException { 247 if (src == null || src.isEmpty()) 248 return null; 249 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.RequestPriority> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.RequestPriorityEnumFactory()); 250 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 251 switch (src.getValue()) { 252 case ROUTINE: 253 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ROUTINE); 254 break; 255 case URGENT: 256 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.URGENT); 257 break; 258 case ASAP: 259 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.ASAP); 260 break; 261 case STAT: 262 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.STAT); 263 break; 264 default: 265 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.RequestPriority.NULL); 266 break; 267 } 268 return tgt; 269 } 270 271 public static org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent convertCommunicationPayloadComponent(org.hl7.fhir.r4b.model.Communication.CommunicationPayloadComponent src) throws FHIRException { 272 if (src == null) 273 return null; 274 org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent tgt = new org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent(); 275 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 276 if (src.hasContent()) { 277 if (src.getContent() instanceof StringType) { 278 CodeableConcept tgtc = new CodeableConcept(); 279 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src.getContent(), tgtc); 280 tgtc.setText(src.getContentStringType().getValue()); 281 tgt.setContent(tgtc); 282 } else { 283 if (src.hasContent()) 284 tgt.setContent(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getContent())); 285 } 286 } 287 return tgt; 288 } 289 290 public static org.hl7.fhir.r4b.model.Communication.CommunicationPayloadComponent convertCommunicationPayloadComponent(org.hl7.fhir.r5.model.Communication.CommunicationPayloadComponent src) throws FHIRException { 291 if (src == null) 292 return null; 293 org.hl7.fhir.r4b.model.Communication.CommunicationPayloadComponent tgt = new org.hl7.fhir.r4b.model.Communication.CommunicationPayloadComponent(); 294 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 295 if (src.hasContent()) { 296 if (src.hasContentCodeableConcept()) { 297 StringType tgts = new StringType(); 298 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src.getContent(), tgts); 299 tgts.setValue(src.getContentCodeableConcept().getText()); 300 tgt.setContent(tgts); 301 } else { 302 if (src.hasContent()) 303 tgt.setContent(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getContent())); 304 } 305 } 306 return tgt; 307 } 308}