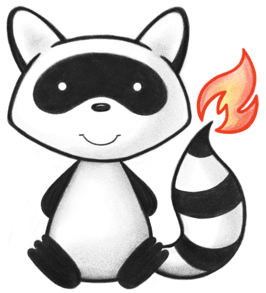
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.ContactDetail43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.metadata43_50.UsageContext43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Code43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Uri43_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.Enumeration; 014import org.hl7.fhir.r5.model.Enumerations; 015 016/* 017 Copyright (c) 2011+, HL7, Inc. 018 All rights reserved. 019 020 Redistribution and use in source and binary forms, with or without modification, 021 are permitted provided that the following conditions are met: 022 023 * Redistributions of source code must retain the above copyright notice, this 024 list of conditions and the following disclaimer. 025 * Redistributions in binary form must reproduce the above copyright notice, 026 this list of conditions and the following disclaimer in the documentation 027 and/or other materials provided with the distribution. 028 * Neither the name of HL7 nor the names of its contributors may be used to 029 endorse or promote products derived from this software without specific 030 prior written permission. 031 032 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 033 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 034 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 035 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 036 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 037 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 038 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 039 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 040 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 041 POSSIBILITY OF SUCH DAMAGE. 042 043*/ 044// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 045public class CompartmentDefinition43_50 { 046 047 public static org.hl7.fhir.r5.model.CompartmentDefinition convertCompartmentDefinition(org.hl7.fhir.r4b.model.CompartmentDefinition src) throws FHIRException { 048 if (src == null) 049 return null; 050 org.hl7.fhir.r5.model.CompartmentDefinition tgt = new org.hl7.fhir.r5.model.CompartmentDefinition(); 051 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 052 if (src.hasUrl()) 053 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 054 if (src.hasVersion()) 055 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 056 if (src.hasName()) 057 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 058 if (src.hasStatus()) 059 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 060 if (src.hasExperimental()) 061 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 062 if (src.hasDate()) 063 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 064 if (src.hasPublisher()) 065 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 066 for (org.hl7.fhir.r4b.model.ContactDetail t : src.getContact()) 067 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 068 if (src.hasDescription()) 069 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 070 for (org.hl7.fhir.r4b.model.UsageContext t : src.getUseContext()) 071 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 072 if (src.hasPurpose()) 073 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 074 if (src.hasCode()) 075 tgt.setCodeElement(convertCompartmentType(src.getCodeElement())); 076 if (src.hasSearch()) 077 tgt.setSearchElement(Boolean43_50.convertBoolean(src.getSearchElement())); 078 for (org.hl7.fhir.r4b.model.CompartmentDefinition.CompartmentDefinitionResourceComponent t : src.getResource()) 079 tgt.addResource(convertCompartmentDefinitionResourceComponent(t)); 080 return tgt; 081 } 082 083 public static org.hl7.fhir.r4b.model.CompartmentDefinition convertCompartmentDefinition(org.hl7.fhir.r5.model.CompartmentDefinition src) throws FHIRException { 084 if (src == null) 085 return null; 086 org.hl7.fhir.r4b.model.CompartmentDefinition tgt = new org.hl7.fhir.r4b.model.CompartmentDefinition(); 087 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 088 if (src.hasUrl()) 089 tgt.setUrlElement(Uri43_50.convertUri(src.getUrlElement())); 090 if (src.hasVersion()) 091 tgt.setVersionElement(String43_50.convertString(src.getVersionElement())); 092 if (src.hasName()) 093 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 094 if (src.hasStatus()) 095 tgt.setStatusElement(Enumerations43_50.convertPublicationStatus(src.getStatusElement())); 096 if (src.hasExperimental()) 097 tgt.setExperimentalElement(Boolean43_50.convertBoolean(src.getExperimentalElement())); 098 if (src.hasDate()) 099 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 100 if (src.hasPublisher()) 101 tgt.setPublisherElement(String43_50.convertString(src.getPublisherElement())); 102 for (org.hl7.fhir.r5.model.ContactDetail t : src.getContact()) 103 tgt.addContact(ContactDetail43_50.convertContactDetail(t)); 104 if (src.hasDescription()) 105 tgt.setDescriptionElement(MarkDown43_50.convertMarkdown(src.getDescriptionElement())); 106 for (org.hl7.fhir.r5.model.UsageContext t : src.getUseContext()) 107 tgt.addUseContext(UsageContext43_50.convertUsageContext(t)); 108 if (src.hasPurpose()) 109 tgt.setPurposeElement(MarkDown43_50.convertMarkdown(src.getPurposeElement())); 110 if (src.hasCode()) 111 tgt.setCodeElement(convertCompartmentType(src.getCodeElement())); 112 if (src.hasSearch()) 113 tgt.setSearchElement(Boolean43_50.convertBoolean(src.getSearchElement())); 114 for (org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent t : src.getResource()) 115 tgt.addResource(convertCompartmentDefinitionResourceComponent(t)); 116 return tgt; 117 } 118 119 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompartmentType> convertCompartmentType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompartmentType> src) throws FHIRException { 120 if (src == null || src.isEmpty()) 121 return null; 122 Enumeration<Enumerations.CompartmentType> tgt = new Enumeration<>(new Enumerations.CompartmentTypeEnumFactory()); 123 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 124 if (src.getValue() == null) { 125 tgt.setValue(null); 126 } else { 127 switch (src.getValue()) { 128 case PATIENT: 129 tgt.setValue(Enumerations.CompartmentType.PATIENT); 130 break; 131 case ENCOUNTER: 132 tgt.setValue(Enumerations.CompartmentType.ENCOUNTER); 133 break; 134 case RELATEDPERSON: 135 tgt.setValue(Enumerations.CompartmentType.RELATEDPERSON); 136 break; 137 case PRACTITIONER: 138 tgt.setValue(Enumerations.CompartmentType.PRACTITIONER); 139 break; 140 case DEVICE: 141 tgt.setValue(Enumerations.CompartmentType.DEVICE); 142 break; 143 default: 144 tgt.setValue(Enumerations.CompartmentType.NULL); 145 break; 146 } 147 } 148 return tgt; 149 } 150 151 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompartmentType> convertCompartmentType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompartmentType> src) throws FHIRException { 152 if (src == null || src.isEmpty()) 153 return null; 154 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompartmentType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.CompartmentTypeEnumFactory()); 155 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 156 if (src.getValue() == null) { 157 tgt.setValue(null); 158 } else { 159 switch (src.getValue()) { 160 case PATIENT: 161 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompartmentType.PATIENT); 162 break; 163 case ENCOUNTER: 164 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompartmentType.ENCOUNTER); 165 break; 166 case RELATEDPERSON: 167 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompartmentType.RELATEDPERSON); 168 break; 169 case PRACTITIONER: 170 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompartmentType.PRACTITIONER); 171 break; 172 case DEVICE: 173 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompartmentType.DEVICE); 174 break; 175 default: 176 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompartmentType.NULL); 177 break; 178 } 179 } 180 return tgt; 181 } 182 183 public static org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent convertCompartmentDefinitionResourceComponent(org.hl7.fhir.r4b.model.CompartmentDefinition.CompartmentDefinitionResourceComponent src) throws FHIRException { 184 if (src == null) 185 return null; 186 org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent tgt = new org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent(); 187 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 188 if (src.hasCode()) 189 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 190 for (org.hl7.fhir.r4b.model.StringType t : src.getParam()) tgt.getParam().add(String43_50.convertString(t)); 191 if (src.hasDocumentation()) 192 tgt.setDocumentationElement(String43_50.convertString(src.getDocumentationElement())); 193 return tgt; 194 } 195 196 public static org.hl7.fhir.r4b.model.CompartmentDefinition.CompartmentDefinitionResourceComponent convertCompartmentDefinitionResourceComponent(org.hl7.fhir.r5.model.CompartmentDefinition.CompartmentDefinitionResourceComponent src) throws FHIRException { 197 if (src == null) 198 return null; 199 org.hl7.fhir.r4b.model.CompartmentDefinition.CompartmentDefinitionResourceComponent tgt = new org.hl7.fhir.r4b.model.CompartmentDefinition.CompartmentDefinitionResourceComponent(); 200 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 201 if (src.hasCode()) 202 tgt.setCodeElement(Code43_50.convertCode(src.getCodeElement())); 203 for (org.hl7.fhir.r5.model.StringType t : src.getParam()) tgt.getParam().add(String43_50.convertString(t)); 204 if (src.hasDocumentation()) 205 tgt.setDocumentationElement(String43_50.convertString(src.getDocumentationElement())); 206 return tgt; 207 } 208}