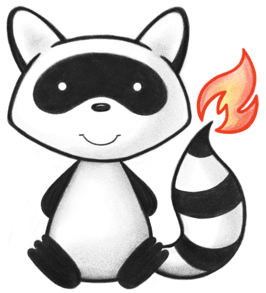
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Code43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.DateTime43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Narrative43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.*; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class Composition43_50 { 045 046 public static org.hl7.fhir.r5.model.Composition convertComposition(org.hl7.fhir.r4b.model.Composition src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.Composition tgt = new org.hl7.fhir.r5.model.Composition(); 050 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 051 if (src.hasIdentifier()) 052 tgt.addIdentifier(Identifier43_50.convertIdentifier(src.getIdentifier())); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertCompositionStatus(src.getStatusElement())); 055 if (src.hasType()) 056 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 057 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCategory()) 058 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 059 if (src.hasSubject()) 060 tgt.addSubject(Reference43_50.convertReference(src.getSubject())); 061 if (src.hasEncounter()) 062 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 063 if (src.hasDate()) 064 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 065 for (org.hl7.fhir.r4b.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference43_50.convertReference(t)); 066 if (src.hasTitle()) 067 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 068 if (src.hasConfidentiality()) 069 tgt.getMeta().addSecurity().setCodeElement(Code43_50.convertCode(src.getConfidentialityElement())); 070 for (org.hl7.fhir.r4b.model.Composition.CompositionAttesterComponent t : src.getAttester()) 071 tgt.addAttester(convertCompositionAttesterComponent(t)); 072 if (src.hasCustodian()) 073 tgt.setCustodian(Reference43_50.convertReference(src.getCustodian())); 074 for (org.hl7.fhir.r4b.model.Composition.CompositionRelatesToComponent t : src.getRelatesTo()) 075 tgt.addRelatesTo(convertCompositionRelatesToComponent(t)); 076 for (org.hl7.fhir.r4b.model.Composition.CompositionEventComponent t : src.getEvent()) 077 tgt.addEvent(convertCompositionEventComponent(t)); 078 for (org.hl7.fhir.r4b.model.Composition.SectionComponent t : src.getSection()) 079 tgt.addSection(convertSectionComponent(t)); 080 return tgt; 081 } 082 083 public static org.hl7.fhir.r4b.model.Composition convertComposition(org.hl7.fhir.r5.model.Composition src) throws FHIRException { 084 if (src == null) 085 return null; 086 org.hl7.fhir.r4b.model.Composition tgt = new org.hl7.fhir.r4b.model.Composition(); 087 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 088 if (src.hasIdentifier()) 089 tgt.setIdentifier(Identifier43_50.convertIdentifier(src.getIdentifierFirstRep())); 090 if (src.hasStatus()) 091 tgt.setStatusElement(convertCompositionStatus(src.getStatusElement())); 092 if (src.hasType()) 093 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 094 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 095 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 096 if (src.hasSubject()) 097 tgt.setSubject(Reference43_50.convertReference(src.getSubjectFirstRep())); 098 if (src.hasEncounter()) 099 tgt.setEncounter(Reference43_50.convertReference(src.getEncounter())); 100 if (src.hasDate()) 101 tgt.setDateElement(DateTime43_50.convertDateTime(src.getDateElement())); 102 for (org.hl7.fhir.r5.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference43_50.convertReference(t)); 103 if (src.hasTitle()) 104 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 105 if (src.getMeta().hasSecurity()) 106 tgt.setConfidentialityElement(Code43_50.convertCode(src.getMeta().getSecurityFirstRep().getCodeElement())); 107 for (org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent t : src.getAttester()) 108 tgt.addAttester(convertCompositionAttesterComponent(t)); 109 if (src.hasCustodian()) 110 tgt.setCustodian(Reference43_50.convertReference(src.getCustodian())); 111 for (RelatedArtifact t : src.getRelatesTo()) 112 tgt.addRelatesTo(convertCompositionRelatesToComponent(t)); 113 for (org.hl7.fhir.r5.model.Composition.CompositionEventComponent t : src.getEvent()) 114 tgt.addEvent(convertCompositionEventComponent(t)); 115 for (org.hl7.fhir.r5.model.Composition.SectionComponent t : src.getSection()) 116 tgt.addSection(convertSectionComponent(t)); 117 return tgt; 118 } 119 120 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> convertCompositionStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompositionStatus> src) throws FHIRException { 121 if (src == null || src.isEmpty()) 122 return null; 123 Enumeration<Enumerations.CompositionStatus> tgt = new Enumeration<>(new Enumerations.CompositionStatusEnumFactory()); 124 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 125 if (src.getValue() == null) { 126 tgt.setValue(null); 127 } else { 128 switch (src.getValue()) { 129 case PRELIMINARY: 130 tgt.setValue(Enumerations.CompositionStatus.PRELIMINARY); 131 break; 132 case FINAL: 133 tgt.setValue(Enumerations.CompositionStatus.FINAL); 134 break; 135 case AMENDED: 136 tgt.setValue(Enumerations.CompositionStatus.AMENDED); 137 break; 138 case ENTEREDINERROR: 139 tgt.setValue(Enumerations.CompositionStatus.ENTEREDINERROR); 140 break; 141 default: 142 tgt.setValue(Enumerations.CompositionStatus.NULL); 143 break; 144 } 145 } 146 return tgt; 147 } 148 149 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompositionStatus> convertCompositionStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> src) throws FHIRException { 150 if (src == null || src.isEmpty()) 151 return null; 152 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompositionStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.CompositionStatusEnumFactory()); 153 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 154 if (src.getValue() == null) { 155 tgt.setValue(null); 156 } else { 157 switch (src.getValue()) { 158 case PRELIMINARY: 159 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.PRELIMINARY); 160 break; 161 case FINAL: 162 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.FINAL); 163 break; 164 case AMENDED: 165 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.AMENDED); 166 break; 167 case ENTEREDINERROR: 168 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.ENTEREDINERROR); 169 break; 170 default: 171 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.NULL); 172 break; 173 } 174 } 175 return tgt; 176 } 177 178 public static org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent convertCompositionAttesterComponent(org.hl7.fhir.r4b.model.Composition.CompositionAttesterComponent src) throws FHIRException { 179 if (src == null) 180 return null; 181 org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent tgt = new org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent(); 182 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 183 if (src.hasMode()) 184 tgt.setMode(convertCompositionAttestationMode(src.getModeElement())); 185 if (src.hasTime()) 186 tgt.setTimeElement(DateTime43_50.convertDateTime(src.getTimeElement())); 187 if (src.hasParty()) 188 tgt.setParty(Reference43_50.convertReference(src.getParty())); 189 return tgt; 190 } 191 192 public static org.hl7.fhir.r4b.model.Composition.CompositionAttesterComponent convertCompositionAttesterComponent(org.hl7.fhir.r5.model.Composition.CompositionAttesterComponent src) throws FHIRException { 193 if (src == null) 194 return null; 195 org.hl7.fhir.r4b.model.Composition.CompositionAttesterComponent tgt = new org.hl7.fhir.r4b.model.Composition.CompositionAttesterComponent(); 196 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 197 if (src.hasMode()) 198 tgt.setModeElement(convertCompositionAttestationMode(src.getMode())); 199 if (src.hasTime()) 200 tgt.setTimeElement(DateTime43_50.convertDateTime(src.getTimeElement())); 201 if (src.hasParty()) 202 tgt.setParty(Reference43_50.convertReference(src.getParty())); 203 return tgt; 204 } 205 206 static public org.hl7.fhir.r5.model.CodeableConcept convertCompositionAttestationMode(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Composition.CompositionAttestationMode> src) throws FHIRException { 207 if (src == null || src.isEmpty()) 208 return null; 209 CodeableConcept tgt = new CodeableConcept(); 210 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 211 if (src.getValue() == null) { 212 // Add nothing 213 } else { 214 switch (src.getValue()) { 215 case PERSONAL: 216 tgt.addCoding().setSystem("http://hl7.org/fhir/composition-attestation-mode").setCode("personal"); 217 break; 218 case PROFESSIONAL: 219 tgt.addCoding().setSystem("http://hl7.org/fhir/composition-attestation-mode").setCode("professional"); 220 break; 221 case LEGAL: 222 tgt.addCoding().setSystem("http://hl7.org/fhir/composition-attestation-mode").setCode("legal"); 223 break; 224 case OFFICIAL: 225 tgt.addCoding().setSystem("http://hl7.org/fhir/composition-attestation-mode").setCode("official"); 226 break; 227 default: 228 break; 229 } 230 } 231 return tgt; 232 } 233 234 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Composition.CompositionAttestationMode> convertCompositionAttestationMode(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 235 if (src == null || src.isEmpty()) 236 return null; 237 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Composition.CompositionAttestationMode> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Composition.CompositionAttestationModeEnumFactory()); 238 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 239 switch (src.getCode("http://hl7.org/fhir/composition-attestation-mode")) { 240 case "personal": 241 tgt.setValue(org.hl7.fhir.r4b.model.Composition.CompositionAttestationMode.PERSONAL); 242 break; 243 case "professional": 244 tgt.setValue(org.hl7.fhir.r4b.model.Composition.CompositionAttestationMode.PROFESSIONAL); 245 break; 246 case "legal": 247 tgt.setValue(org.hl7.fhir.r4b.model.Composition.CompositionAttestationMode.LEGAL); 248 break; 249 case "official": 250 tgt.setValue(org.hl7.fhir.r4b.model.Composition.CompositionAttestationMode.OFFICIAL); 251 break; 252 default: 253 tgt.setValue(org.hl7.fhir.r4b.model.Composition.CompositionAttestationMode.NULL); 254 break; 255 } 256 return tgt; 257 } 258 259 public static org.hl7.fhir.r5.model.RelatedArtifact convertCompositionRelatesToComponent(org.hl7.fhir.r4b.model.Composition.CompositionRelatesToComponent src) throws FHIRException { 260 if (src == null) 261 return null; 262 org.hl7.fhir.r5.model.RelatedArtifact tgt = new org.hl7.fhir.r5.model.RelatedArtifact(); 263 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 264 if (src.hasCode()) 265 tgt.setTypeElement(convertDocumentRelationshipType(src.getCodeElement())); 266 if (src.hasTargetReference()) 267 tgt.setResourceReference(Reference43_50.convertReference(src.getTargetReference())); 268 return tgt; 269 } 270 271 public static org.hl7.fhir.r4b.model.Composition.CompositionRelatesToComponent convertCompositionRelatesToComponent(org.hl7.fhir.r5.model.RelatedArtifact src) throws FHIRException { 272 if (src == null) 273 return null; 274 org.hl7.fhir.r4b.model.Composition.CompositionRelatesToComponent tgt = new org.hl7.fhir.r4b.model.Composition.CompositionRelatesToComponent(); 275 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 276 if (src.hasType()) 277 tgt.setCodeElement(convertDocumentRelationshipType(src.getTypeElement())); 278 if (src.hasResourceReference()) 279 tgt.setTarget(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getResourceReference())); 280 return tgt; 281 } 282 283 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> convertDocumentRelationshipType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType> src) throws FHIRException { 284 if (src == null || src.isEmpty()) 285 return null; 286 Enumeration<RelatedArtifact.RelatedArtifactType> tgt = new Enumeration<>(new RelatedArtifact.RelatedArtifactTypeEnumFactory()); 287 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 288 if (src.getValue() == null) { 289 tgt.setValue(null); 290 } else { 291 switch (src.getValue()) { 292 case REPLACES: 293 tgt.setValue(RelatedArtifact.RelatedArtifactType.REPLACES); 294 break; 295 case TRANSFORMS: 296 tgt.setValue(RelatedArtifact.RelatedArtifactType.TRANSFORMS); 297 break; 298 case SIGNS: 299 tgt.setValue(RelatedArtifact.RelatedArtifactType.SIGNS); 300 break; 301 case APPENDS: 302 tgt.setValue(RelatedArtifact.RelatedArtifactType.APPENDS); 303 break; 304 default: 305 tgt.setValue(RelatedArtifact.RelatedArtifactType.NULL); 306 break; 307 } 308 } 309 return tgt; 310 } 311 312 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.RelatedArtifact.RelatedArtifactType> src) throws FHIRException { 313 if (src == null || src.isEmpty()) 314 return null; 315 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipTypeEnumFactory()); 316 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 317 if (src.getValue() == null) { 318 tgt.setValue(null); 319 } else { 320 switch (src.getValue()) { 321 case REPLACES: 322 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.REPLACES); 323 break; 324 case TRANSFORMS: 325 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.TRANSFORMS); 326 break; 327 case SIGNS: 328 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.SIGNS); 329 break; 330 case APPENDS: 331 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.APPENDS); 332 break; 333 default: 334 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.NULL); 335 break; 336 } 337 } 338 return tgt; 339 } 340 341 public static org.hl7.fhir.r5.model.Composition.CompositionEventComponent convertCompositionEventComponent(org.hl7.fhir.r4b.model.Composition.CompositionEventComponent src) throws FHIRException { 342 if (src == null) 343 return null; 344 org.hl7.fhir.r5.model.Composition.CompositionEventComponent tgt = new org.hl7.fhir.r5.model.Composition.CompositionEventComponent(); 345 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 346 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCode()) 347 tgt.addDetail().setConcept(CodeableConcept43_50.convertCodeableConcept(t)); 348 if (src.hasPeriod()) 349 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 350 for (org.hl7.fhir.r4b.model.Reference t : src.getDetail()) tgt.addDetail().setReference(Reference43_50.convertReference(t)); 351 return tgt; 352 } 353 354 public static org.hl7.fhir.r4b.model.Composition.CompositionEventComponent convertCompositionEventComponent(org.hl7.fhir.r5.model.Composition.CompositionEventComponent src) throws FHIRException { 355 if (src == null) 356 return null; 357 org.hl7.fhir.r4b.model.Composition.CompositionEventComponent tgt = new org.hl7.fhir.r4b.model.Composition.CompositionEventComponent(); 358 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 359 if (src.hasPeriod()) 360 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 361 for (CodeableReference t : src.getDetail()) { 362 if (t.hasConcept()) { 363 tgt.addCode(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 364 } 365 if (t.hasReference()) { 366 tgt.addDetail(Reference43_50.convertReference(t.getReference())); 367 } 368 } 369 return tgt; 370 } 371 372 public static org.hl7.fhir.r5.model.Composition.SectionComponent convertSectionComponent(org.hl7.fhir.r4b.model.Composition.SectionComponent src) throws FHIRException { 373 if (src == null) 374 return null; 375 org.hl7.fhir.r5.model.Composition.SectionComponent tgt = new org.hl7.fhir.r5.model.Composition.SectionComponent(); 376 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 377 if (src.hasTitle()) 378 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 379 if (src.hasCode()) 380 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 381 for (org.hl7.fhir.r4b.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference43_50.convertReference(t)); 382 if (src.hasFocus()) 383 tgt.setFocus(Reference43_50.convertReference(src.getFocus())); 384 if (src.hasText()) 385 tgt.setText(Narrative43_50.convertNarrative(src.getText())); 386// if (src.hasMode()) 387// tgt.setModeElement(convertSectionMode(src.getModeElement())); 388 if (src.hasOrderedBy()) 389 tgt.setOrderedBy(CodeableConcept43_50.convertCodeableConcept(src.getOrderedBy())); 390 for (org.hl7.fhir.r4b.model.Reference t : src.getEntry()) tgt.addEntry(Reference43_50.convertReference(t)); 391 if (src.hasEmptyReason()) 392 tgt.setEmptyReason(CodeableConcept43_50.convertCodeableConcept(src.getEmptyReason())); 393 for (org.hl7.fhir.r4b.model.Composition.SectionComponent t : src.getSection()) 394 tgt.addSection(convertSectionComponent(t)); 395 return tgt; 396 } 397 398 public static org.hl7.fhir.r4b.model.Composition.SectionComponent convertSectionComponent(org.hl7.fhir.r5.model.Composition.SectionComponent src) throws FHIRException { 399 if (src == null) 400 return null; 401 org.hl7.fhir.r4b.model.Composition.SectionComponent tgt = new org.hl7.fhir.r4b.model.Composition.SectionComponent(); 402 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 403 if (src.hasTitle()) 404 tgt.setTitleElement(String43_50.convertString(src.getTitleElement())); 405 if (src.hasCode()) 406 tgt.setCode(CodeableConcept43_50.convertCodeableConcept(src.getCode())); 407 for (org.hl7.fhir.r5.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference43_50.convertReference(t)); 408 if (src.hasFocus()) 409 tgt.setFocus(Reference43_50.convertReference(src.getFocus())); 410 if (src.hasText()) 411 tgt.setText(Narrative43_50.convertNarrative(src.getText())); 412// if (src.hasMode()) 413// tgt.setModeElement(convertSectionMode(src.getModeElement())); 414 if (src.hasOrderedBy()) 415 tgt.setOrderedBy(CodeableConcept43_50.convertCodeableConcept(src.getOrderedBy())); 416 for (org.hl7.fhir.r5.model.Reference t : src.getEntry()) tgt.addEntry(Reference43_50.convertReference(t)); 417 if (src.hasEmptyReason()) 418 tgt.setEmptyReason(CodeableConcept43_50.convertCodeableConcept(src.getEmptyReason())); 419 for (org.hl7.fhir.r5.model.Composition.SectionComponent t : src.getSection()) 420 tgt.addSection(convertSectionComponent(t)); 421 return tgt; 422 } 423 424 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ListMode> convertSectionMode(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ListMode> src) throws FHIRException { 425 if (src == null || src.isEmpty()) 426 return null; 427 Enumeration<Enumerations.ListMode> tgt = new Enumeration<>(new Enumerations.ListModeEnumFactory()); 428 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 429 if (src.getValue() == null) { 430 tgt.setValue(null); 431 } else { 432 switch (src.getValue()) { 433 case WORKING: 434 tgt.setValue(Enumerations.ListMode.WORKING); 435 break; 436 case SNAPSHOT: 437 tgt.setValue(Enumerations.ListMode.SNAPSHOT); 438 break; 439 case CHANGES: 440 tgt.setValue(Enumerations.ListMode.CHANGES); 441 break; 442 default: 443 tgt.setValue(Enumerations.ListMode.NULL); 444 break; 445 } 446 } 447 return tgt; 448 } 449 450 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ListMode> convertSectionMode(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.ListMode> src) throws FHIRException { 451 if (src == null || src.isEmpty()) 452 return null; 453 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.ListMode> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.ListModeEnumFactory()); 454 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 455 if (src.getValue() == null) { 456 tgt.setValue(null); 457 } else { 458 switch (src.getValue()) { 459 case WORKING: 460 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ListMode.WORKING); 461 break; 462 case SNAPSHOT: 463 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ListMode.SNAPSHOT); 464 break; 465 case CHANGES: 466 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ListMode.CHANGES); 467 break; 468 default: 469 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.ListMode.NULL); 470 break; 471 } 472 } 473 return tgt; 474 } 475}