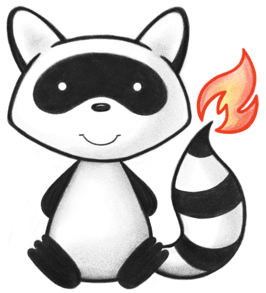
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Boolean43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.PositiveInt43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 011import org.hl7.fhir.exceptions.FHIRException; 012import org.hl7.fhir.r5.model.Enumeration; 013import org.hl7.fhir.r5.model.Enumerations; 014 015/* 016 Copyright (c) 2011+, HL7, Inc. 017 All rights reserved. 018 019 Redistribution and use in source and binary forms, with or without modification, 020 are permitted provided that the following conditions are met: 021 022 * Redistributions of source code must retain the above copyright notice, this 023 list of conditions and the following disclaimer. 024 * Redistributions in binary form must reproduce the above copyright notice, 025 this list of conditions and the following disclaimer in the documentation 026 and/or other materials provided with the distribution. 027 * Neither the name of HL7 nor the names of its contributors may be used to 028 endorse or promote products derived from this software without specific 029 prior written permission. 030 031 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 032 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 033 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 034 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 035 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 036 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 037 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 038 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 039 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 040 POSSIBILITY OF SUCH DAMAGE. 041 042*/ 043// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 044public class Coverage43_50 { 045 046 public static org.hl7.fhir.r5.model.Coverage convertCoverage(org.hl7.fhir.r4b.model.Coverage src) throws FHIRException { 047 if (src == null) 048 return null; 049 org.hl7.fhir.r5.model.Coverage tgt = new org.hl7.fhir.r5.model.Coverage(); 050 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 051 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 052 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 053 if (src.hasStatus()) 054 tgt.setStatusElement(convertCoverageStatus(src.getStatusElement())); 055 if (src.hasType()) 056 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 057 if (src.hasPolicyHolder()) 058 tgt.setPolicyHolder(Reference43_50.convertReference(src.getPolicyHolder())); 059 if (src.hasSubscriber()) 060 tgt.setSubscriber(Reference43_50.convertReference(src.getSubscriber())); 061 if (src.hasSubscriberId()) 062 tgt.getSubscriberIdFirstRep().setValueElement(String43_50.convertString(src.getSubscriberIdElement())); 063 if (src.hasBeneficiary()) 064 tgt.setBeneficiary(Reference43_50.convertReference(src.getBeneficiary())); 065 if (src.hasDependent()) 066 tgt.setDependentElement(String43_50.convertString(src.getDependentElement())); 067 if (src.hasRelationship()) 068 tgt.setRelationship(CodeableConcept43_50.convertCodeableConcept(src.getRelationship())); 069 if (src.hasPeriod()) 070 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 071 for (org.hl7.fhir.r4b.model.Reference t : src.getPayor()) tgt.setInsurer(Reference43_50.convertReference(t)); 072 for (org.hl7.fhir.r4b.model.Coverage.ClassComponent t : src.getClass_()) tgt.addClass_(convertClassComponent(t)); 073 if (src.hasOrder()) 074 tgt.setOrderElement(PositiveInt43_50.convertPositiveInt(src.getOrderElement())); 075 if (src.hasNetwork()) 076 tgt.setNetworkElement(String43_50.convertString(src.getNetworkElement())); 077 for (org.hl7.fhir.r4b.model.Coverage.CostToBeneficiaryComponent t : src.getCostToBeneficiary()) 078 tgt.addCostToBeneficiary(convertCostToBeneficiaryComponent(t)); 079 if (src.hasSubrogation()) 080 tgt.setSubrogationElement(Boolean43_50.convertBoolean(src.getSubrogationElement())); 081 for (org.hl7.fhir.r4b.model.Reference t : src.getContract()) tgt.addContract(Reference43_50.convertReference(t)); 082 return tgt; 083 } 084 085 public static org.hl7.fhir.r4b.model.Coverage convertCoverage(org.hl7.fhir.r5.model.Coverage src) throws FHIRException { 086 if (src == null) 087 return null; 088 org.hl7.fhir.r4b.model.Coverage tgt = new org.hl7.fhir.r4b.model.Coverage(); 089 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 090 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 091 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 092 if (src.hasStatus()) 093 tgt.setStatusElement(convertCoverageStatus(src.getStatusElement())); 094 if (src.hasType()) 095 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 096 if (src.hasPolicyHolder()) 097 tgt.setPolicyHolder(Reference43_50.convertReference(src.getPolicyHolder())); 098 if (src.hasSubscriber()) 099 tgt.setSubscriber(Reference43_50.convertReference(src.getSubscriber())); 100 if (src.hasSubscriberId()) 101 tgt.setSubscriberIdElement(String43_50.convertString(src.getSubscriberIdFirstRep().getValueElement())); 102 if (src.hasBeneficiary()) 103 tgt.setBeneficiary(Reference43_50.convertReference(src.getBeneficiary())); 104 if (src.hasDependent()) 105 tgt.setDependentElement(String43_50.convertString(src.getDependentElement())); 106 if (src.hasRelationship()) 107 tgt.setRelationship(CodeableConcept43_50.convertCodeableConcept(src.getRelationship())); 108 if (src.hasPeriod()) 109 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 110 tgt.addPayor(Reference43_50.convertReference(src.getInsurer())); 111 for (org.hl7.fhir.r5.model.Coverage.ClassComponent t : src.getClass_()) tgt.addClass_(convertClassComponent(t)); 112 if (src.hasOrder()) 113 tgt.setOrderElement(PositiveInt43_50.convertPositiveInt(src.getOrderElement())); 114 if (src.hasNetwork()) 115 tgt.setNetworkElement(String43_50.convertString(src.getNetworkElement())); 116 for (org.hl7.fhir.r5.model.Coverage.CostToBeneficiaryComponent t : src.getCostToBeneficiary()) 117 tgt.addCostToBeneficiary(convertCostToBeneficiaryComponent(t)); 118 if (src.hasSubrogation()) 119 tgt.setSubrogationElement(Boolean43_50.convertBoolean(src.getSubrogationElement())); 120 for (org.hl7.fhir.r5.model.Reference t : src.getContract()) tgt.addContract(Reference43_50.convertReference(t)); 121 return tgt; 122 } 123 124 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FinancialResourceStatusCodes> convertCoverageStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes> src) throws FHIRException { 125 if (src == null || src.isEmpty()) 126 return null; 127 Enumeration<Enumerations.FinancialResourceStatusCodes> tgt = new Enumeration<>(new Enumerations.FinancialResourceStatusCodesEnumFactory()); 128 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 129 if (src.getValue() == null) { 130 tgt.setValue(null); 131 } else { 132 switch (src.getValue()) { 133 case ACTIVE: 134 tgt.setValue(Enumerations.FinancialResourceStatusCodes.ACTIVE); 135 break; 136 case CANCELLED: 137 tgt.setValue(Enumerations.FinancialResourceStatusCodes.CANCELLED); 138 break; 139 case DRAFT: 140 tgt.setValue(Enumerations.FinancialResourceStatusCodes.DRAFT); 141 break; 142 case ENTEREDINERROR: 143 tgt.setValue(Enumerations.FinancialResourceStatusCodes.ENTEREDINERROR); 144 break; 145 default: 146 tgt.setValue(Enumerations.FinancialResourceStatusCodes.NULL); 147 break; 148 } 149 } 150 return tgt; 151 } 152 153 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes> convertCoverageStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FinancialResourceStatusCodes> src) throws FHIRException { 154 if (src == null || src.isEmpty()) 155 return null; 156 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodesEnumFactory()); 157 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 158 if (src.getValue() == null) { 159 tgt.setValue(null); 160 } else { 161 switch (src.getValue()) { 162 case ACTIVE: 163 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.ACTIVE); 164 break; 165 case CANCELLED: 166 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.CANCELLED); 167 break; 168 case DRAFT: 169 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.DRAFT); 170 break; 171 case ENTEREDINERROR: 172 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.ENTEREDINERROR); 173 break; 174 default: 175 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FinancialResourceStatusCodes.NULL); 176 break; 177 } 178 } 179 return tgt; 180 } 181 182 public static org.hl7.fhir.r5.model.Coverage.ClassComponent convertClassComponent(org.hl7.fhir.r4b.model.Coverage.ClassComponent src) throws FHIRException { 183 if (src == null) 184 return null; 185 org.hl7.fhir.r5.model.Coverage.ClassComponent tgt = new org.hl7.fhir.r5.model.Coverage.ClassComponent(); 186 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 187 if (src.hasType()) 188 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 189 if (src.hasValue()) 190 tgt.getValue().setValueElement(String43_50.convertString(src.getValueElement())); 191 if (src.hasName()) 192 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 193 return tgt; 194 } 195 196 public static org.hl7.fhir.r4b.model.Coverage.ClassComponent convertClassComponent(org.hl7.fhir.r5.model.Coverage.ClassComponent src) throws FHIRException { 197 if (src == null) 198 return null; 199 org.hl7.fhir.r4b.model.Coverage.ClassComponent tgt = new org.hl7.fhir.r4b.model.Coverage.ClassComponent(); 200 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 201 if (src.hasType()) 202 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 203 if (src.hasValue()) 204 tgt.setValueElement(String43_50.convertString(src.getValue().getValueElement())); 205 if (src.hasName()) 206 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 207 return tgt; 208 } 209 210 public static org.hl7.fhir.r5.model.Coverage.CostToBeneficiaryComponent convertCostToBeneficiaryComponent(org.hl7.fhir.r4b.model.Coverage.CostToBeneficiaryComponent src) throws FHIRException { 211 if (src == null) 212 return null; 213 org.hl7.fhir.r5.model.Coverage.CostToBeneficiaryComponent tgt = new org.hl7.fhir.r5.model.Coverage.CostToBeneficiaryComponent(); 214 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 215 if (src.hasType()) 216 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 217 if (src.hasValue()) 218 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 219 for (org.hl7.fhir.r4b.model.Coverage.ExemptionComponent t : src.getException()) 220 tgt.addException(convertExemptionComponent(t)); 221 return tgt; 222 } 223 224 public static org.hl7.fhir.r4b.model.Coverage.CostToBeneficiaryComponent convertCostToBeneficiaryComponent(org.hl7.fhir.r5.model.Coverage.CostToBeneficiaryComponent src) throws FHIRException { 225 if (src == null) 226 return null; 227 org.hl7.fhir.r4b.model.Coverage.CostToBeneficiaryComponent tgt = new org.hl7.fhir.r4b.model.Coverage.CostToBeneficiaryComponent(); 228 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 229 if (src.hasType()) 230 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 231 if (src.hasValue()) 232 tgt.setValue(ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().convertType(src.getValue())); 233 for (org.hl7.fhir.r5.model.Coverage.ExemptionComponent t : src.getException()) 234 tgt.addException(convertExemptionComponent(t)); 235 return tgt; 236 } 237 238 public static org.hl7.fhir.r5.model.Coverage.ExemptionComponent convertExemptionComponent(org.hl7.fhir.r4b.model.Coverage.ExemptionComponent src) throws FHIRException { 239 if (src == null) 240 return null; 241 org.hl7.fhir.r5.model.Coverage.ExemptionComponent tgt = new org.hl7.fhir.r5.model.Coverage.ExemptionComponent(); 242 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 243 if (src.hasType()) 244 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 245 if (src.hasPeriod()) 246 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 247 return tgt; 248 } 249 250 public static org.hl7.fhir.r4b.model.Coverage.ExemptionComponent convertExemptionComponent(org.hl7.fhir.r5.model.Coverage.ExemptionComponent src) throws FHIRException { 251 if (src == null) 252 return null; 253 org.hl7.fhir.r4b.model.Coverage.ExemptionComponent tgt = new org.hl7.fhir.r4b.model.Coverage.ExemptionComponent(); 254 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 255 if (src.hasType()) 256 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 257 if (src.hasPeriod()) 258 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 259 return tgt; 260 } 261}