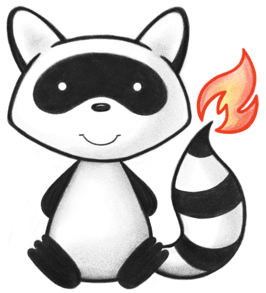
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Attachment43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Instant43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.MarkDown43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 012import org.hl7.fhir.exceptions.FHIRException; 013import org.hl7.fhir.r5.model.CodeableConcept; 014import org.hl7.fhir.r5.model.CodeableReference; 015import org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceAttesterComponent; 016import org.hl7.fhir.r5.model.Enumeration; 017import org.hl7.fhir.r5.model.Enumerations; 018 019/* 020 Copyright (c) 2011+, HL7, Inc. 021 All rights reserved. 022 023 Redistribution and use in source and binary forms, with or without modification, 024 are permitted provided that the following conditions are met: 025 026 * Redistributions of source code must retain the above copyright notice, this 027 list of conditions and the following disclaimer. 028 * Redistributions in binary form must reproduce the above copyright notice, 029 this list of conditions and the following disclaimer in the documentation 030 and/or other materials provided with the distribution. 031 * Neither the name of HL7 nor the names of its contributors may be used to 032 endorse or promote products derived from this software without specific 033 prior written permission. 034 035 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 036 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 037 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 038 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 039 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 040 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 041 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 042 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 043 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 044 POSSIBILITY OF SUCH DAMAGE. 045 046*/ 047// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 048public class DocumentReference43_50 { 049 050 public static org.hl7.fhir.r5.model.DocumentReference convertDocumentReference(org.hl7.fhir.r4b.model.DocumentReference src) throws FHIRException { 051 if (src == null) 052 return null; 053 org.hl7.fhir.r5.model.DocumentReference tgt = new org.hl7.fhir.r5.model.DocumentReference(); 054 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 055 if (src.hasMasterIdentifier()) 056 tgt.addIdentifier(Identifier43_50.convertIdentifier(src.getMasterIdentifier())); 057 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 058 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 059 if (src.hasStatus()) 060 tgt.setStatusElement(Enumerations43_50.convertDocumentReferenceStatus(src.getStatusElement())); 061 if (src.hasDocStatus()) 062 tgt.setDocStatusElement(convertReferredDocumentStatus(src.getDocStatusElement())); 063 if (src.hasType()) 064 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 065 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getCategory()) 066 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 067 if (src.hasSubject()) 068 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 069 if (src.hasDate()) 070 tgt.setDateElement(Instant43_50.convertInstant(src.getDateElement())); 071 for (org.hl7.fhir.r4b.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference43_50.convertReference(t)); 072 if (src.hasAuthenticator()) 073 tgt.addAttester().setMode(new org.hl7.fhir.r5.model.CodeableConcept().addCoding(new org.hl7.fhir.r5.model.Coding("http://hl7.org/fhir/composition-attestation-mode","official", "Official"))) 074 .setParty(Reference43_50.convertReference(src.getAuthenticator())); 075 if (src.hasCustodian()) 076 tgt.setCustodian(Reference43_50.convertReference(src.getCustodian())); 077 for (org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 078 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 079 if (src.hasDescription()) 080 tgt.setDescriptionElement(MarkDown43_50.convertStringToMarkdown(src.getDescriptionElement())); 081 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getSecurityLabel()) 082 tgt.addSecurityLabel(CodeableConcept43_50.convertCodeableConcept(t)); 083 for (org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 084 tgt.addContent(convertDocumentReferenceContentComponent(t)); 085 if (src.hasContext()) 086 convertDocumentReferenceContextComponent(src.getContext(), tgt); 087 for (org.hl7.fhir.r4b.model.Reference t : src.getAuthor()) 088 tgt.addAuthor(Reference43_50.convertReference(t)); 089 return tgt; 090 } 091 092 public static org.hl7.fhir.r4b.model.DocumentReference convertDocumentReference(org.hl7.fhir.r5.model.DocumentReference src) throws FHIRException { 093 if (src == null) 094 return null; 095 org.hl7.fhir.r4b.model.DocumentReference tgt = new org.hl7.fhir.r4b.model.DocumentReference(); 096 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 097// if (src.hasMasterIdentifier()) 098// tgt.setMasterIdentifier(convertIdentifier(src.getMasterIdentifier())); 099 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 100 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 101 if (src.hasStatus()) 102 tgt.setStatusElement(Enumerations43_50.convertDocumentReferenceStatus(src.getStatusElement())); 103 if (src.hasDocStatus()) 104 tgt.setDocStatusElement(convertReferredDocumentStatus(src.getDocStatusElement())); 105 if (src.hasType()) 106 tgt.setType(CodeableConcept43_50.convertCodeableConcept(src.getType())); 107 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getCategory()) 108 tgt.addCategory(CodeableConcept43_50.convertCodeableConcept(t)); 109 if (src.hasSubject()) 110 tgt.setSubject(Reference43_50.convertReference(src.getSubject())); 111 if (src.hasDate()) 112 tgt.setDateElement(Instant43_50.convertInstant(src.getDateElement())); 113 for (org.hl7.fhir.r5.model.Reference t : src.getAuthor()) tgt.addAuthor(Reference43_50.convertReference(t)); 114 for (DocumentReferenceAttesterComponent t : src.getAttester()) { 115 if (t.getMode().hasCoding("http://hl7.org/fhir/composition-attestation-mode", "official")) 116 tgt.setAuthenticator(Reference43_50.convertReference(t.getParty())); 117 } 118 if (src.hasCustodian()) 119 tgt.setCustodian(Reference43_50.convertReference(src.getCustodian())); 120 for (org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent t : src.getRelatesTo()) 121 tgt.addRelatesTo(convertDocumentReferenceRelatesToComponent(t)); 122 if (src.hasDescription()) 123 tgt.setDescriptionElement(String43_50.convertString(src.getDescriptionElement())); 124 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getSecurityLabel()) 125 tgt.addSecurityLabel(CodeableConcept43_50.convertCodeableConcept(t)); 126 for (org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent t : src.getContent()) 127 tgt.addContent(convertDocumentReferenceContentComponent(t)); 128 convertDocumentReferenceContextComponent(src, tgt.getContext()); 129 for (org.hl7.fhir.r5.model.Reference t : src.getAuthor()) 130 tgt.addAuthor(Reference43_50.convertReference(t)); 131 return tgt; 132 } 133 134 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> convertReferredDocumentStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompositionStatus> src) throws FHIRException { 135 if (src == null || src.isEmpty()) 136 return null; 137 Enumeration<Enumerations.CompositionStatus> tgt = new Enumeration<>(new Enumerations.CompositionStatusEnumFactory()); 138 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 139 if (src.getValue() == null) { 140 tgt.setValue(null); 141 } else { 142 switch (src.getValue()) { 143 case PRELIMINARY: 144 tgt.setValue(Enumerations.CompositionStatus.PRELIMINARY); 145 break; 146 case FINAL: 147 tgt.setValue(Enumerations.CompositionStatus.FINAL); 148 break; 149 case AMENDED: 150 tgt.setValue(Enumerations.CompositionStatus.AMENDED); 151 break; 152 case ENTEREDINERROR: 153 tgt.setValue(Enumerations.CompositionStatus.ENTEREDINERROR); 154 break; 155 default: 156 tgt.setValue(Enumerations.CompositionStatus.NULL); 157 break; 158 } 159 } 160 return tgt; 161 } 162 163 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompositionStatus> convertReferredDocumentStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.CompositionStatus> src) throws FHIRException { 164 if (src == null || src.isEmpty()) 165 return null; 166 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.CompositionStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.CompositionStatusEnumFactory()); 167 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 168 if (src.getValue() == null) { 169 tgt.setValue(null); 170 } else { 171 switch (src.getValue()) { 172 case PRELIMINARY: 173 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.PRELIMINARY); 174 break; 175 case FINAL: 176 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.FINAL); 177 break; 178 case AMENDED: 179 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.AMENDED); 180 break; 181 case ENTEREDINERROR: 182 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.ENTEREDINERROR); 183 break; 184 default: 185 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.CompositionStatus.NULL); 186 break; 187 } 188 } 189 return tgt; 190 } 191 192 public static org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 193 if (src == null) 194 return null; 195 org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent(); 196 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 197 if (src.hasCode()) 198 tgt.setCode(convertDocumentRelationshipType(src.getCodeElement())); 199 if (src.hasTarget()) 200 tgt.setTarget(Reference43_50.convertReference(src.getTarget())); 201 return tgt; 202 } 203 204 public static org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceRelatesToComponent convertDocumentReferenceRelatesToComponent(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceRelatesToComponent src) throws FHIRException { 205 if (src == null) 206 return null; 207 org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceRelatesToComponent tgt = new org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceRelatesToComponent(); 208 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 209 if (src.hasCode()) 210 tgt.setCodeElement(convertDocumentRelationshipType(src.getCode())); 211 if (src.hasTarget()) 212 tgt.setTarget(Reference43_50.convertReference(src.getTarget())); 213 return tgt; 214 } 215 216 static public org.hl7.fhir.r5.model.CodeableConcept convertDocumentRelationshipType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType> src) throws FHIRException { 217 if (src == null || src.isEmpty()) 218 return null; 219 CodeableConcept tgt = new CodeableConcept(); 220 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 221 if (src.getValue() == null) { 222 // Add nothing 223 } else { 224 switch (src.getValue()) { 225 case REPLACES: 226 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("replaces"); 227 break; 228 case TRANSFORMS: 229 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("transforms"); 230 break; 231 case SIGNS: 232 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("signs"); 233 break; 234 case APPENDS: 235 tgt.addCoding().setSystem("http://hl7.org/fhir/document-relationship-type").setCode("appends"); 236 break; 237 default: 238 break; 239 } 240 } 241 return tgt; 242 } 243 244 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType> convertDocumentRelationshipType(org.hl7.fhir.r5.model.CodeableConcept src) throws FHIRException { 245 if (src == null || src.isEmpty()) 246 return null; 247 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipTypeEnumFactory()); 248 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 249 switch (src.getCode("http://hl7.org/fhir/document-relationship-type")) { 250 case "replaces": 251 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.REPLACES); 252 break; 253 case "transforms": 254 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.TRANSFORMS); 255 break; 256 case "signs": 257 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.SIGNS); 258 break; 259 case "appends": 260 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.APPENDS); 261 break; 262 default: 263 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentRelationshipType.NULL); 264 break; 265 } 266 return tgt; 267 } 268 269 public static org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 270 if (src == null) 271 return null; 272 org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent(); 273 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 274 if (src.hasAttachment()) 275 tgt.setAttachment(Attachment43_50.convertAttachment(src.getAttachment())); 276 return tgt; 277 } 278 279 public static org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceContentComponent convertDocumentReferenceContentComponent(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceContentComponent src) throws FHIRException { 280 if (src == null) 281 return null; 282 org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceContentComponent tgt = new org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceContentComponent(); 283 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 284 if (src.hasAttachment()) 285 tgt.setAttachment(Attachment43_50.convertAttachment(src.getAttachment())); 286 return tgt; 287 } 288 289 public static void convertDocumentReferenceContextComponent(org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceContextComponent src, org.hl7.fhir.r5.model.DocumentReference tgt) throws FHIRException { 290 for (org.hl7.fhir.r4b.model.Reference t : src.getEncounter()) tgt.addContext(Reference43_50.convertReference(t)); 291 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getEvent()) 292 tgt.addEvent(new CodeableReference().setConcept(CodeableConcept43_50.convertCodeableConcept(t))); 293 if (src.hasPeriod()) 294 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 295 if (src.hasFacilityType()) 296 tgt.setFacilityType(CodeableConcept43_50.convertCodeableConcept(src.getFacilityType())); 297 if (src.hasPracticeSetting()) 298 tgt.setPracticeSetting(CodeableConcept43_50.convertCodeableConcept(src.getPracticeSetting())); 299// if (src.hasSourcePatientInfo()) 300// tgt.setSourcePatientInfo(Reference43_50.convertReference(src.getSourcePatientInfo())); 301// for (org.hl7.fhir.r4b.model.Reference t : src.getRelated()) tgt.addRelated(Reference43_50.convertReference(t)); 302 } 303 304 public static void convertDocumentReferenceContextComponent(org.hl7.fhir.r5.model.DocumentReference src, org.hl7.fhir.r4b.model.DocumentReference.DocumentReferenceContextComponent tgt) throws FHIRException { 305 for (org.hl7.fhir.r5.model.Reference t : src.getContext()) tgt.addEncounter(Reference43_50.convertReference(t)); 306 for (CodeableReference t : src.getEvent()) 307 if (t.hasConcept()) 308 tgt.addEvent(CodeableConcept43_50.convertCodeableConcept(t.getConcept())); 309 if (src.hasPeriod()) 310 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 311 if (src.hasFacilityType()) 312 tgt.setFacilityType(CodeableConcept43_50.convertCodeableConcept(src.getFacilityType())); 313 if (src.hasPracticeSetting()) 314 tgt.setPracticeSetting(CodeableConcept43_50.convertCodeableConcept(src.getPracticeSetting())); 315// if (src.hasSourcePatientInfo()) 316// tgt.setSourcePatientInfo(Reference43_50.convertReference(src.getSourcePatientInfo())); 317// for (org.hl7.fhir.r5.model.Reference t : src.getRelated()) tgt.addRelated(Reference43_50.convertReference(t)); 318 } 319}