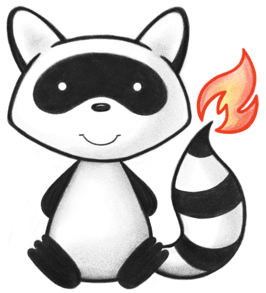
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Coding43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.ContactPoint43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 008import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 009import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Code43_50; 010import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.String43_50; 011import org.hl7.fhir.convertors.conv43_50.datatypes43_50.primitive43_50.Url43_50; 012import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 013import org.hl7.fhir.exceptions.FHIRException; 014import org.hl7.fhir.r5.model.Endpoint; 015import org.hl7.fhir.r5.model.Endpoint.EndpointPayloadComponent; 016import org.hl7.fhir.r5.model.Enumeration; 017 018/* 019 Copyright (c) 2011+, HL7, Inc. 020 All rights reserved. 021 022 Redistribution and use in source and binary forms, with or without modification, 023 are permitted provided that the following conditions are met: 024 025 * Redistributions of source code must retain the above copyright notice, this 026 list of conditions and the following disclaimer. 027 * Redistributions in binary form must reproduce the above copyright notice, 028 this list of conditions and the following disclaimer in the documentation 029 and/or other materials provided with the distribution. 030 * Neither the name of HL7 nor the names of its contributors may be used to 031 endorse or promote products derived from this software without specific 032 prior written permission. 033 034 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 035 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 036 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 037 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 038 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 039 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 040 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 041 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 042 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 043 POSSIBILITY OF SUCH DAMAGE. 044 045*/ 046// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 047public class Endpoint43_50 { 048 049 public static org.hl7.fhir.r5.model.Endpoint convertEndpoint(org.hl7.fhir.r4b.model.Endpoint src) throws FHIRException { 050 if (src == null) 051 return null; 052 org.hl7.fhir.r5.model.Endpoint tgt = new org.hl7.fhir.r5.model.Endpoint(); 053 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 054 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 055 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 056 if (src.hasStatus()) 057 tgt.setStatusElement(convertEndpointStatus(src.getStatusElement())); 058 if (src.hasConnectionType()) 059 tgt.addConnectionType(Coding43_50.convertCodingToCodeableConcept(src.getConnectionType())); 060 if (src.hasName()) 061 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 062 if (src.hasManagingOrganization()) 063 tgt.setManagingOrganization(Reference43_50.convertReference(src.getManagingOrganization())); 064 for (org.hl7.fhir.r4b.model.ContactPoint t : src.getContact()) 065 tgt.addContact(ContactPoint43_50.convertContactPoint(t)); 066 if (src.hasPeriod()) 067 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 068 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getPayloadType()) 069 tgt.addPayload().addType(CodeableConcept43_50.convertCodeableConcept(t)); 070 for (org.hl7.fhir.r4b.model.CodeType t : src.getPayloadMimeType()) 071 tgt.addPayload().getMimeType().add(Code43_50.convertCode(t)); 072 if (src.hasAddress()) 073 tgt.setAddressElement(Url43_50.convertUrl(src.getAddressElement())); 074 for (org.hl7.fhir.r4b.model.StringType t : src.getHeader()) tgt.getHeader().add(String43_50.convertString(t)); 075 return tgt; 076 } 077 078 public static org.hl7.fhir.r4b.model.Endpoint convertEndpoint(org.hl7.fhir.r5.model.Endpoint src) throws FHIRException { 079 if (src == null) 080 return null; 081 org.hl7.fhir.r4b.model.Endpoint tgt = new org.hl7.fhir.r4b.model.Endpoint(); 082 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 083 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 084 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 085 if (src.hasStatus()) 086 tgt.setStatusElement(convertEndpointStatus(src.getStatusElement())); 087 if (src.hasConnectionType()) 088 tgt.setConnectionType(Coding43_50.convertCoding(src.getConnectionTypeFirstRep())); 089 if (src.hasName()) 090 tgt.setNameElement(String43_50.convertString(src.getNameElement())); 091 if (src.hasManagingOrganization()) 092 tgt.setManagingOrganization(Reference43_50.convertReference(src.getManagingOrganization())); 093 for (org.hl7.fhir.r5.model.ContactPoint t : src.getContact()) 094 tgt.addContact(ContactPoint43_50.convertContactPoint(t)); 095 if (src.hasPeriod()) 096 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 097 for (EndpointPayloadComponent t : src.getPayload()) 098 if (t.hasType()) 099 tgt.addPayloadType(CodeableConcept43_50.convertCodeableConcept(t.getTypeFirstRep())); 100 for (EndpointPayloadComponent t : src.getPayload()) 101 if (t.hasMimeType()) 102 tgt.getPayloadMimeType().add(Code43_50.convertCode(t.getMimeType().get(0))); 103 if (src.hasAddress()) 104 tgt.setAddressElement(Url43_50.convertUrl(src.getAddressElement())); 105 for (org.hl7.fhir.r5.model.StringType t : src.getHeader()) tgt.getHeader().add(String43_50.convertString(t)); 106 return tgt; 107 } 108 109 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Endpoint.EndpointStatus> convertEndpointStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Endpoint.EndpointStatus> src) throws FHIRException { 110 if (src == null || src.isEmpty()) 111 return null; 112 Enumeration<Endpoint.EndpointStatus> tgt = new Enumeration<>(new Endpoint.EndpointStatusEnumFactory()); 113 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 114 if (src.getValue() == null) { 115 tgt.setValue(null); 116 } else { 117 switch (src.getValue()) { 118 case ACTIVE: 119 tgt.setValue(Endpoint.EndpointStatus.ACTIVE); 120 break; 121 case SUSPENDED: 122 tgt.setValue(Endpoint.EndpointStatus.SUSPENDED); 123 break; 124 case ERROR: 125 tgt.setValue(Endpoint.EndpointStatus.ERROR); 126 break; 127 case OFF: 128 tgt.setValue(Endpoint.EndpointStatus.OFF); 129 break; 130 case ENTEREDINERROR: 131 tgt.setValue(Endpoint.EndpointStatus.ENTEREDINERROR); 132 break; 133 default: 134 tgt.setValue(Endpoint.EndpointStatus.NULL); 135 break; 136 } 137 } 138 return tgt; 139 } 140 141 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Endpoint.EndpointStatus> convertEndpointStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Endpoint.EndpointStatus> src) throws FHIRException { 142 if (src == null || src.isEmpty()) 143 return null; 144 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Endpoint.EndpointStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Endpoint.EndpointStatusEnumFactory()); 145 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 146 if (src.getValue() == null) { 147 tgt.setValue(null); 148 } else { 149 switch (src.getValue()) { 150 case ACTIVE: 151 tgt.setValue(org.hl7.fhir.r4b.model.Endpoint.EndpointStatus.ACTIVE); 152 break; 153 case SUSPENDED: 154 tgt.setValue(org.hl7.fhir.r4b.model.Endpoint.EndpointStatus.SUSPENDED); 155 break; 156 case ERROR: 157 tgt.setValue(org.hl7.fhir.r4b.model.Endpoint.EndpointStatus.ERROR); 158 break; 159 case OFF: 160 tgt.setValue(org.hl7.fhir.r4b.model.Endpoint.EndpointStatus.OFF); 161 break; 162 case ENTEREDINERROR: 163 tgt.setValue(org.hl7.fhir.r4b.model.Endpoint.EndpointStatus.ENTEREDINERROR); 164 break; 165 default: 166 tgt.setValue(org.hl7.fhir.r4b.model.Endpoint.EndpointStatus.NULL); 167 break; 168 } 169 } 170 return tgt; 171 } 172}