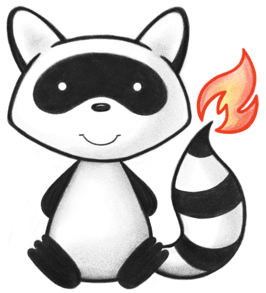
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Extension43_50; 005import org.hl7.fhir.exceptions.FHIRException; 006 007/* 008 Copyright (c) 2011+, HL7, Inc. 009 All rights reserved. 010 011 Redistribution and use in source and binary forms, with or without modification, 012 are permitted provided that the following conditions are met: 013 014 * Redistributions of source code must retain the above copyright notice, this 015 list of conditions and the following disclaimer. 016 * Redistributions in binary form must reproduce the above copyright notice, 017 this list of conditions and the following disclaimer in the documentation 018 and/or other materials provided with the distribution. 019 * Neither the name of HL7 nor the names of its contributors may be used to 020 endorse or promote products derived from this software without specific 021 prior written permission. 022 023 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 024 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 025 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 026 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 027 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 028 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 029 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 030 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 031 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 032 POSSIBILITY OF SUCH DAMAGE. 033 034*/ 035// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 036public class Enumerations43_50 { 037 038 public static void copyEnumeration(org.hl7.fhir.r4b.model.Enumeration<?> src, org.hl7.fhir.r5.model.Enumeration<?> tgt) throws FHIRException { 039 if (src.hasId()) tgt.setId(src.getId()); 040 for (org.hl7.fhir.r4b.model.Extension e : src.getExtension()) { 041 tgt.addExtension(Extension43_50.convertExtension(e)); 042 } 043 } 044 045 public static void copyEnumeration(org.hl7.fhir.r5.model.Enumeration<?> src, org.hl7.fhir.r4b.model.Enumeration<?> tgt) throws FHIRException { 046 if (src.hasId()) tgt.setId(src.getId()); 047 for (org.hl7.fhir.r5.model.Extension e : src.getExtension()) { 048 tgt.addExtension(Extension43_50.convertExtension(e)); 049 } 050 } 051 052 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.BindingStrength> src) throws FHIRException { 053 if (src == null || src.isEmpty()) 054 return null; 055 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.BindingStrengthEnumFactory()); 056 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 057 switch (src.getValue()) { 058 case REQUIRED: 059 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.BindingStrength.REQUIRED); 060 break; 061 case EXTENSIBLE: 062 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.BindingStrength.EXTENSIBLE); 063 break; 064 case PREFERRED: 065 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.BindingStrength.PREFERRED); 066 break; 067 case EXAMPLE: 068 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.BindingStrength.EXAMPLE); 069 break; 070 default: 071 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.BindingStrength.NULL); 072 break; 073 } 074 return tgt; 075 } 076 077 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.BindingStrength> convertBindingStrength(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.BindingStrength> src) throws FHIRException { 078 if (src == null || src.isEmpty()) 079 return null; 080 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.BindingStrength> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.BindingStrengthEnumFactory()); 081 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 082 switch (src.getValue()) { 083 case REQUIRED: 084 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.BindingStrength.REQUIRED); 085 break; 086 case EXTENSIBLE: 087 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.BindingStrength.EXTENSIBLE); 088 break; 089 case PREFERRED: 090 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.BindingStrength.PREFERRED); 091 break; 092 case EXAMPLE: 093 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.BindingStrength.EXAMPLE); 094 break; 095 default: 096 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.BindingStrength.NULL); 097 break; 098 } 099 return tgt; 100 } 101 102 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> convertPublicationStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.PublicationStatus> src) throws FHIRException { 103 if (src == null || src.isEmpty()) 104 return null; 105 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.PublicationStatusEnumFactory()); 106 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 107 switch (src.getValue()) { 108 case DRAFT: 109 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.PublicationStatus.DRAFT); 110 break; 111 case ACTIVE: 112 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.PublicationStatus.ACTIVE); 113 break; 114 case RETIRED: 115 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.PublicationStatus.RETIRED); 116 break; 117 case UNKNOWN: 118 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.PublicationStatus.UNKNOWN); 119 break; 120 default: 121 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.PublicationStatus.NULL); 122 break; 123 } 124 return tgt; 125 } 126 127 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.PublicationStatus> convertPublicationStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.PublicationStatus> src) throws FHIRException { 128 if (src == null || src.isEmpty()) 129 return null; 130 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.PublicationStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.PublicationStatusEnumFactory()); 131 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 132 switch (src.getValue()) { 133 case DRAFT: 134 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.PublicationStatus.DRAFT); 135 break; 136 case ACTIVE: 137 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.PublicationStatus.ACTIVE); 138 break; 139 case RETIRED: 140 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.PublicationStatus.RETIRED); 141 break; 142 case UNKNOWN: 143 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.PublicationStatus.UNKNOWN); 144 break; 145 default: 146 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.PublicationStatus.NULL); 147 break; 148 } 149 return tgt; 150 } 151 152 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FHIRVersion> convertFHIRVersion(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FHIRVersion> src) throws FHIRException { 153 if (src == null || src.isEmpty()) 154 return null; 155 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FHIRVersion> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.FHIRVersionEnumFactory()); 156 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 157 switch (src.getValue()) { 158 case _0_01: 159 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_01); 160 break; 161 case _0_05: 162 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_05); 163 break; 164 case _0_06: 165 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_06); 166 break; 167 case _0_11: 168 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_11); 169 break; 170 case _0_0_80: 171 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_0_80); 172 break; 173 case _0_0_81: 174 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_0_81); 175 break; 176 case _0_0_82: 177 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_0_82); 178 break; 179 case _0_4_0: 180 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_4_0); 181 break; 182 case _0_5_0: 183 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._0_5_0); 184 break; 185 case _1_0_0: 186 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._1_0_0); 187 break; 188 case _1_0_1: 189 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._1_0_1); 190 break; 191 case _1_0_2: 192 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._1_0_2); 193 break; 194 case _1_1_0: 195 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._1_1_0); 196 break; 197 case _1_4_0: 198 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._1_4_0); 199 break; 200 case _1_6_0: 201 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._1_6_0); 202 break; 203 case _1_8_0: 204 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._1_8_0); 205 break; 206 case _3_0_0: 207 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._3_0_0); 208 break; 209 case _3_0_1: 210 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._3_0_1); 211 break; 212 case _3_0_2: 213 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._3_0_2); 214 break; 215 case _3_3_0: 216 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._3_3_0); 217 break; 218 case _3_5_0: 219 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._3_5_0); 220 break; 221 case _4_0_0: 222 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._4_0_0); 223 break; 224 case _4_0_1: 225 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._4_0_1); 226 break; 227 case _4_1_0: 228 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._4_1_0); 229 break; 230 case _4_3_0: 231 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion._4_3_0); 232 break; 233 default: 234 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.FHIRVersion.NULL); 235 break; 236 } 237 return tgt; 238 } 239 240 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FHIRVersion> convertFHIRVersion(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.FHIRVersion> src) throws FHIRException { 241 if (src == null || src.isEmpty()) 242 return null; 243 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.FHIRVersion> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.FHIRVersionEnumFactory()); 244 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 245 switch (src.getValue()) { 246 case _0_01: 247 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_01); 248 break; 249 case _0_05: 250 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_05); 251 break; 252 case _0_06: 253 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_06); 254 break; 255 case _0_11: 256 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_11); 257 break; 258 case _0_0_80: 259 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_0_80); 260 break; 261 case _0_0_81: 262 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_0_81); 263 break; 264 case _0_0_82: 265 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_0_82); 266 break; 267 case _0_4_0: 268 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_4_0); 269 break; 270 case _0_5_0: 271 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._0_5_0); 272 break; 273 case _1_0_0: 274 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._1_0_0); 275 break; 276 case _1_0_1: 277 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._1_0_1); 278 break; 279 case _1_0_2: 280 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._1_0_2); 281 break; 282 case _1_1_0: 283 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._1_1_0); 284 break; 285 case _1_4_0: 286 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._1_4_0); 287 break; 288 case _1_6_0: 289 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._1_6_0); 290 break; 291 case _1_8_0: 292 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._1_8_0); 293 break; 294 case _3_0_0: 295 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._3_0_0); 296 break; 297 case _3_0_1: 298 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._3_0_1); 299 break; 300 case _3_0_2: 301 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._3_0_2); 302 break; 303 case _3_3_0: 304 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._3_3_0); 305 break; 306 case _3_5_0: 307 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._3_5_0); 308 break; 309 case _4_0_0: 310 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._4_0_0); 311 break; 312 case _4_0_1: 313 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._4_0_1); 314 break; 315 case _4_1_0: 316 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._4_1_0); 317 break; 318 case _4_3_0: 319 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion._4_3_0); 320 break; 321 default: 322 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.FHIRVersion.NULL); 323 break; 324 } 325 return tgt; 326 } 327 328 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.SearchParamType> src) throws FHIRException { 329 if (src == null || src.isEmpty()) 330 return null; 331 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.SearchParamTypeEnumFactory()); 332 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 333 switch (src.getValue()) { 334 case NUMBER: 335 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.NUMBER); 336 break; 337 case DATE: 338 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.DATE); 339 break; 340 case STRING: 341 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.STRING); 342 break; 343 case TOKEN: 344 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.TOKEN); 345 break; 346 case REFERENCE: 347 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.REFERENCE); 348 break; 349 case COMPOSITE: 350 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.COMPOSITE); 351 break; 352 case QUANTITY: 353 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.QUANTITY); 354 break; 355 case URI: 356 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.URI); 357 break; 358 case SPECIAL: 359 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.SPECIAL); 360 break; 361 default: 362 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.SearchParamType.NULL); 363 break; 364 } 365 return tgt; 366 } 367 368 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.SearchParamType> convertSearchParamType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.SearchParamType> src) throws FHIRException { 369 if (src == null || src.isEmpty()) 370 return null; 371 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.SearchParamType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.SearchParamTypeEnumFactory()); 372 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 373 switch (src.getValue()) { 374 case NUMBER: 375 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.NUMBER); 376 break; 377 case DATE: 378 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.DATE); 379 break; 380 case STRING: 381 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.STRING); 382 break; 383 case TOKEN: 384 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.TOKEN); 385 break; 386 case REFERENCE: 387 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.REFERENCE); 388 break; 389 case COMPOSITE: 390 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.COMPOSITE); 391 break; 392 case QUANTITY: 393 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.QUANTITY); 394 break; 395 case URI: 396 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.URI); 397 break; 398 case SPECIAL: 399 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.SPECIAL); 400 break; 401 default: 402 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.SearchParamType.NULL); 403 break; 404 } 405 return tgt; 406 } 407 408 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.PaymentReconciliation.NoteType> convertNoteType(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.NoteType> src) throws FHIRException { 409 if (src == null || src.isEmpty()) 410 return null; 411 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.PaymentReconciliation.NoteType> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.PaymentReconciliation.NoteTypeEnumFactory()); 412 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 413 switch (src.getValue()) { 414 case DISPLAY: 415 tgt.setValue(org.hl7.fhir.r5.model.PaymentReconciliation.NoteType.DISPLAY); 416 break; 417 case PRINT: 418 tgt.setValue(org.hl7.fhir.r5.model.PaymentReconciliation.NoteType.PRINT); 419 break; 420 case PRINTOPER: 421 tgt.setValue(org.hl7.fhir.r5.model.PaymentReconciliation.NoteType.PRINTOPER); 422 break; 423 default: 424 tgt.setValue(org.hl7.fhir.r5.model.PaymentReconciliation.NoteType.NULL); 425 break; 426 } 427 return tgt; 428 } 429 430 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.NoteType> convertNoteType(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.PaymentReconciliation.NoteType> src) throws FHIRException { 431 if (src == null || src.isEmpty()) 432 return null; 433 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.NoteType> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.NoteTypeEnumFactory()); 434 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 435 switch (src.getValue()) { 436 case DISPLAY: 437 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.NoteType.DISPLAY); 438 break; 439 case PRINT: 440 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.NoteType.PRINT); 441 break; 442 case PRINTOPER: 443 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.NoteType.PRINTOPER); 444 break; 445 default: 446 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.NoteType.NULL); 447 break; 448 } 449 return tgt; 450 } 451 452 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentReferenceStatus> src) throws FHIRException { 453 if (src == null || src.isEmpty()) 454 return null; 455 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatusEnumFactory()); 456 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 457 switch (src.getValue()) { 458 case CURRENT: 459 tgt.setValue(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus.CURRENT); 460 break; 461 case SUPERSEDED: 462 tgt.setValue(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus.SUPERSEDED); 463 break; 464 case ENTEREDINERROR: 465 tgt.setValue(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus.ENTEREDINERROR); 466 break; 467 default: 468 tgt.setValue(org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus.NULL); 469 break; 470 } 471 return tgt; 472 } 473 474 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentReferenceStatus> convertDocumentReferenceStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.DocumentReference.DocumentReferenceStatus> src) throws FHIRException { 475 if (src == null || src.isEmpty()) 476 return null; 477 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.DocumentReferenceStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.DocumentReferenceStatusEnumFactory()); 478 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 479 switch (src.getValue()) { 480 case CURRENT: 481 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentReferenceStatus.CURRENT); 482 break; 483 case SUPERSEDED: 484 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentReferenceStatus.SUPERSEDED); 485 break; 486 case ENTEREDINERROR: 487 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentReferenceStatus.ENTEREDINERROR); 488 break; 489 default: 490 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.DocumentReferenceStatus.NULL); 491 break; 492 } 493 return tgt; 494 } 495 496 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.AdministrativeGender> src) throws FHIRException { 497 if (src == null || src.isEmpty()) 498 return null; 499 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.Enumerations.AdministrativeGenderEnumFactory()); 500 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 501 switch (src.getValue()) { 502 case MALE: 503 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.AdministrativeGender.MALE); 504 break; 505 case FEMALE: 506 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.AdministrativeGender.FEMALE); 507 break; 508 case OTHER: 509 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.AdministrativeGender.OTHER); 510 break; 511 case UNKNOWN: 512 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.AdministrativeGender.UNKNOWN); 513 break; 514 default: 515 tgt.setValue(org.hl7.fhir.r5.model.Enumerations.AdministrativeGender.NULL); 516 break; 517 } 518 return tgt; 519 } 520 521 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.AdministrativeGender> convertAdministrativeGender(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.Enumerations.AdministrativeGender> src) throws FHIRException { 522 if (src == null || src.isEmpty()) 523 return null; 524 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.Enumerations.AdministrativeGender> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.Enumerations.AdministrativeGenderEnumFactory()); 525 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 526 switch (src.getValue()) { 527 case MALE: 528 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.AdministrativeGender.MALE); 529 break; 530 case FEMALE: 531 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.AdministrativeGender.FEMALE); 532 break; 533 case OTHER: 534 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.AdministrativeGender.OTHER); 535 break; 536 case UNKNOWN: 537 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.AdministrativeGender.UNKNOWN); 538 break; 539 default: 540 tgt.setValue(org.hl7.fhir.r4b.model.Enumerations.AdministrativeGender.NULL); 541 break; 542 } 543 return tgt; 544 } 545 546}