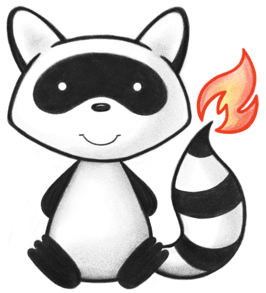
001package org.hl7.fhir.convertors.conv43_50.resources43_50; 002 003import org.hl7.fhir.convertors.context.ConversionContext43_50; 004import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.CodeableConcept43_50; 005import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Identifier43_50; 006import org.hl7.fhir.convertors.conv43_50.datatypes43_50.general43_50.Period43_50; 007import org.hl7.fhir.convertors.conv43_50.datatypes43_50.special43_50.Reference43_50; 008import org.hl7.fhir.exceptions.FHIRException; 009 010/* 011 Copyright (c) 2011+, HL7, Inc. 012 All rights reserved. 013 014 Redistribution and use in source and binary forms, with or without modification, 015 are permitted provided that the following conditions are met: 016 017 * Redistributions of source code must retain the above copyright notice, this 018 list of conditions and the following disclaimer. 019 * Redistributions in binary form must reproduce the above copyright notice, 020 this list of conditions and the following disclaimer in the documentation 021 and/or other materials provided with the distribution. 022 * Neither the name of HL7 nor the names of its contributors may be used to 023 endorse or promote products derived from this software without specific 024 prior written permission. 025 026 THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND 027 ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED 028 WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE DISCLAIMED. 029 IN NO EVENT SHALL THE COPYRIGHT HOLDER OR CONTRIBUTORS BE LIABLE FOR ANY DIRECT, 030 INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL DAMAGES (INCLUDING, BUT 031 NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR SERVICES; LOSS OF USE, DATA, OR 032 PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED AND ON ANY THEORY OF LIABILITY, 033 WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT (INCLUDING NEGLIGENCE OR OTHERWISE) 034 ARISING IN ANY WAY OUT OF THE USE OF THIS SOFTWARE, EVEN IF ADVISED OF THE 035 POSSIBILITY OF SUCH DAMAGE. 036 037*/ 038// Generated on Sun, Feb 24, 2019 11:37+1100 for FHIR v4.0.0 039public class EpisodeOfCare43_50 { 040 041 public static org.hl7.fhir.r5.model.EpisodeOfCare convertEpisodeOfCare(org.hl7.fhir.r4b.model.EpisodeOfCare src) throws FHIRException { 042 if (src == null) 043 return null; 044 org.hl7.fhir.r5.model.EpisodeOfCare tgt = new org.hl7.fhir.r5.model.EpisodeOfCare(); 045 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 046 for (org.hl7.fhir.r4b.model.Identifier t : src.getIdentifier()) 047 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 048 if (src.hasStatus()) 049 tgt.setStatusElement(convertEpisodeOfCareStatus(src.getStatusElement())); 050 for (org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent t : src.getStatusHistory()) 051 tgt.addStatusHistory(convertEpisodeOfCareStatusHistoryComponent(t)); 052 for (org.hl7.fhir.r4b.model.CodeableConcept t : src.getType()) 053 tgt.addType(CodeableConcept43_50.convertCodeableConcept(t)); 054 for (org.hl7.fhir.r4b.model.EpisodeOfCare.DiagnosisComponent t : src.getDiagnosis()) 055 tgt.addDiagnosis(convertDiagnosisComponent(t)); 056 if (src.hasPatient()) 057 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 058 if (src.hasManagingOrganization()) 059 tgt.setManagingOrganization(Reference43_50.convertReference(src.getManagingOrganization())); 060 if (src.hasPeriod()) 061 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 062 for (org.hl7.fhir.r4b.model.Reference t : src.getReferralRequest()) 063 tgt.addReferralRequest(Reference43_50.convertReference(t)); 064 if (src.hasCareManager()) 065 tgt.setCareManager(Reference43_50.convertReference(src.getCareManager())); 066 for (org.hl7.fhir.r4b.model.Reference t : src.getTeam()) tgt.addCareTeam(Reference43_50.convertReference(t)); 067 for (org.hl7.fhir.r4b.model.Reference t : src.getAccount()) tgt.addAccount(Reference43_50.convertReference(t)); 068 return tgt; 069 } 070 071 public static org.hl7.fhir.r4b.model.EpisodeOfCare convertEpisodeOfCare(org.hl7.fhir.r5.model.EpisodeOfCare src) throws FHIRException { 072 if (src == null) 073 return null; 074 org.hl7.fhir.r4b.model.EpisodeOfCare tgt = new org.hl7.fhir.r4b.model.EpisodeOfCare(); 075 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyDomainResource(src, tgt); 076 for (org.hl7.fhir.r5.model.Identifier t : src.getIdentifier()) 077 tgt.addIdentifier(Identifier43_50.convertIdentifier(t)); 078 if (src.hasStatus()) 079 tgt.setStatusElement(convertEpisodeOfCareStatus(src.getStatusElement())); 080 for (org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent t : src.getStatusHistory()) 081 tgt.addStatusHistory(convertEpisodeOfCareStatusHistoryComponent(t)); 082 for (org.hl7.fhir.r5.model.CodeableConcept t : src.getType()) 083 tgt.addType(CodeableConcept43_50.convertCodeableConcept(t)); 084 for (org.hl7.fhir.r5.model.EpisodeOfCare.DiagnosisComponent t : src.getDiagnosis()) 085 tgt.addDiagnosis(convertDiagnosisComponent(t)); 086 if (src.hasPatient()) 087 tgt.setPatient(Reference43_50.convertReference(src.getPatient())); 088 if (src.hasManagingOrganization()) 089 tgt.setManagingOrganization(Reference43_50.convertReference(src.getManagingOrganization())); 090 if (src.hasPeriod()) 091 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 092 for (org.hl7.fhir.r5.model.Reference t : src.getReferralRequest()) 093 tgt.addReferralRequest(Reference43_50.convertReference(t)); 094 if (src.hasCareManager()) 095 tgt.setCareManager(Reference43_50.convertReference(src.getCareManager())); 096 for (org.hl7.fhir.r5.model.Reference t : src.getCareTeam()) tgt.addTeam(Reference43_50.convertReference(t)); 097 for (org.hl7.fhir.r5.model.Reference t : src.getAccount()) tgt.addAccount(Reference43_50.convertReference(t)); 098 return tgt; 099 } 100 101 static public org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus> convertEpisodeOfCareStatus(org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus> src) throws FHIRException { 102 if (src == null || src.isEmpty()) 103 return null; 104 org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus> tgt = new org.hl7.fhir.r5.model.Enumeration<>(new org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatusEnumFactory()); 105 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 106 switch (src.getValue()) { 107 case PLANNED: 108 tgt.setValue(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus.PLANNED); 109 break; 110 case WAITLIST: 111 tgt.setValue(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus.WAITLIST); 112 break; 113 case ACTIVE: 114 tgt.setValue(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus.ACTIVE); 115 break; 116 case ONHOLD: 117 tgt.setValue(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus.ONHOLD); 118 break; 119 case FINISHED: 120 tgt.setValue(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus.FINISHED); 121 break; 122 case CANCELLED: 123 tgt.setValue(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus.CANCELLED); 124 break; 125 case ENTEREDINERROR: 126 tgt.setValue(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus.ENTEREDINERROR); 127 break; 128 default: 129 tgt.setValue(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus.NULL); 130 break; 131 } 132 return tgt; 133 } 134 135 static public org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus> convertEpisodeOfCareStatus(org.hl7.fhir.r5.model.Enumeration<org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatus> src) throws FHIRException { 136 if (src == null || src.isEmpty()) 137 return null; 138 org.hl7.fhir.r4b.model.Enumeration<org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus> tgt = new org.hl7.fhir.r4b.model.Enumeration<>(new org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatusEnumFactory()); 139 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyElement(src, tgt); 140 switch (src.getValue()) { 141 case PLANNED: 142 tgt.setValue(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus.PLANNED); 143 break; 144 case WAITLIST: 145 tgt.setValue(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus.WAITLIST); 146 break; 147 case ACTIVE: 148 tgt.setValue(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus.ACTIVE); 149 break; 150 case ONHOLD: 151 tgt.setValue(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus.ONHOLD); 152 break; 153 case FINISHED: 154 tgt.setValue(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus.FINISHED); 155 break; 156 case CANCELLED: 157 tgt.setValue(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus.CANCELLED); 158 break; 159 case ENTEREDINERROR: 160 tgt.setValue(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus.ENTEREDINERROR); 161 break; 162 default: 163 tgt.setValue(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatus.NULL); 164 break; 165 } 166 return tgt; 167 } 168 169 public static org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent convertEpisodeOfCareStatusHistoryComponent(org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent src) throws FHIRException { 170 if (src == null) 171 return null; 172 org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent tgt = new org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent(); 173 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 174 if (src.hasStatus()) 175 tgt.setStatusElement(convertEpisodeOfCareStatus(src.getStatusElement())); 176 if (src.hasPeriod()) 177 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 178 return tgt; 179 } 180 181 public static org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent convertEpisodeOfCareStatusHistoryComponent(org.hl7.fhir.r5.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent src) throws FHIRException { 182 if (src == null) 183 return null; 184 org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent tgt = new org.hl7.fhir.r4b.model.EpisodeOfCare.EpisodeOfCareStatusHistoryComponent(); 185 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 186 if (src.hasStatus()) 187 tgt.setStatusElement(convertEpisodeOfCareStatus(src.getStatusElement())); 188 if (src.hasPeriod()) 189 tgt.setPeriod(Period43_50.convertPeriod(src.getPeriod())); 190 return tgt; 191 } 192 193 public static org.hl7.fhir.r5.model.EpisodeOfCare.DiagnosisComponent convertDiagnosisComponent(org.hl7.fhir.r4b.model.EpisodeOfCare.DiagnosisComponent src) throws FHIRException { 194 if (src == null) 195 return null; 196 org.hl7.fhir.r5.model.EpisodeOfCare.DiagnosisComponent tgt = new org.hl7.fhir.r5.model.EpisodeOfCare.DiagnosisComponent(); 197 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 198 if (src.hasCondition()) 199 tgt.addCondition(Reference43_50.convertReferenceToCodeableReference(src.getCondition())); 200 if (src.hasRole()) 201 tgt.setUse(CodeableConcept43_50.convertCodeableConcept(src.getRole())); 202// if (src.hasRank()) 203// tgt.setRankElement(PositiveInt43_50.convertPositiveInt(src.getRankElement())); 204 return tgt; 205 } 206 207 public static org.hl7.fhir.r4b.model.EpisodeOfCare.DiagnosisComponent convertDiagnosisComponent(org.hl7.fhir.r5.model.EpisodeOfCare.DiagnosisComponent src) throws FHIRException { 208 if (src == null) 209 return null; 210 org.hl7.fhir.r4b.model.EpisodeOfCare.DiagnosisComponent tgt = new org.hl7.fhir.r4b.model.EpisodeOfCare.DiagnosisComponent(); 211 ConversionContext43_50.INSTANCE.getVersionConvertor_43_50().copyBackboneElement(src, tgt); 212 if (src.hasCondition()) 213 tgt.setCondition(Reference43_50.convertCodeableReferenceToReference(src.getConditionFirstRep())); 214 if (src.hasUse()) 215 tgt.setRole(CodeableConcept43_50.convertCodeableConcept(src.getUse())); 216// if (src.hasRank()) 217// tgt.setRankElement(PositiveInt43_50.convertPositiveInt(src.getRankElement())); 218 return tgt; 219 } 220}